In C++, the heap is a region of memory used for dynamic memory allocation, allowing programmers to request and release memory at runtime using pointers.
Here's a simple example of allocating and deallocating memory on the heap:
#include <iostream>
int main() {
// Allocate memory for an integer on the heap
int* ptr = new int(42);
// Output the value
std::cout << "Value: " << *ptr << std::endl;
// Deallocate memory
delete ptr;
return 0;
}
What is the Heap?
Definition of Heap Memory
The heap is an area of memory used for dynamic memory allocation in C++. Unlike the stack, which operates in a last-in, first-out manner and is managed automatically, the heap allows you to manually allocate and deallocate memory as needed. This flexibility is crucial for programs that require variable-sized data structures and memory management beyond the scope of stack limitations.
Characteristics of Heap Memory
Heap memory exhibits several key characteristics that distinguish it from stack memory:
- Dynamic Memory Allocation: Memory can be allocated and deallocated at any time during program execution, allowing for more flexible data structures.
- Longer Lifetime: Unlike stack variables, which are automatically destroyed when they go out of scope, heap-allocated memory exists until it is explicitly deallocated. This feature is critical when you need to maintain data across different function calls.
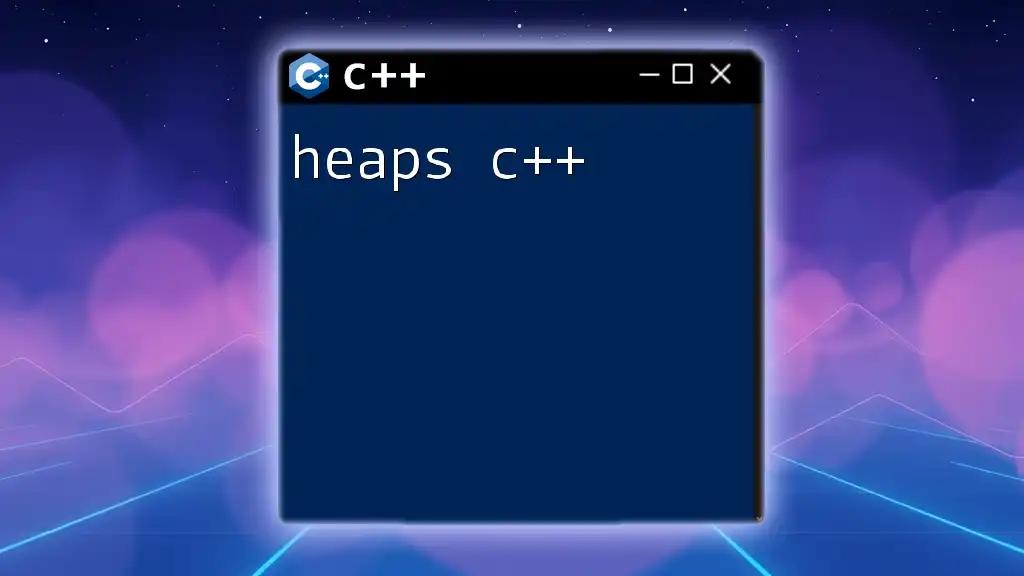
How to Allocate Memory on the Heap
Using `new` Keyword
In C++, you can allocate memory on the heap using the `new` keyword. This operator allows you to create variables and objects dynamically.
Basic Syntax
int* ptr = new int;
In this example, `ptr` is a pointer to an integer variable allocated on the heap. Using `new` ensures that the memory is allocated out of the stack frame, meaning it will not be released automatically when the function scope ends.
Allocating Arrays
Heap allocation is particularly useful for dynamic arrays. You can allocate an array on the heap easily by extending the `new` keyword.
Code Snippet
int* arr = new int[10];
This code snippet allocates an array of 10 integers on the heap. Remember that heap-allocated arrays do not have a predefined size; they can grow or shrink as the program executes, provided that you manage the memory correctly.
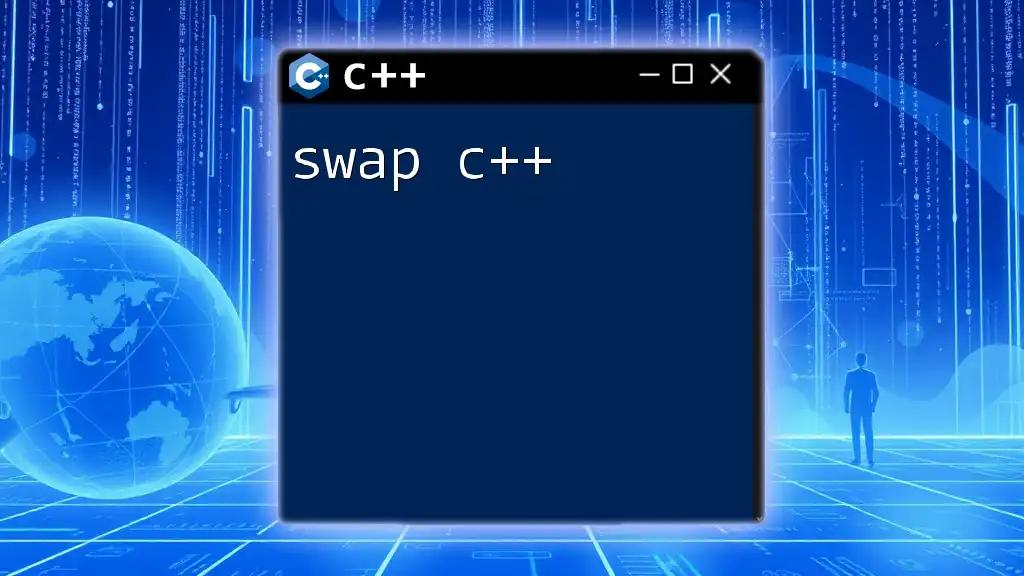
Deallocating Heap Memory
The Importance of Memory Management
Failing to manage heap memory properly can lead to memory leaks, where allocated memory blocks are no longer accessible or deallocated, causing the program to consume increasing amounts of memory over time.
Using `delete` Keyword
To free up memory allocated on the heap, you use the `delete` operator for single variables and `delete[]` for arrays.
Syntax and Usage of `delete`
delete ptr;
This line deallocates the memory pointed to by `ptr`. If you forget to call `delete`, you will create a memory leak.
Array Deletion
When dealing with heap-allocated arrays, you must use the following syntax:
delete[] arr;
This ensures that the entire array is properly deallocated, preventing memory leaks.
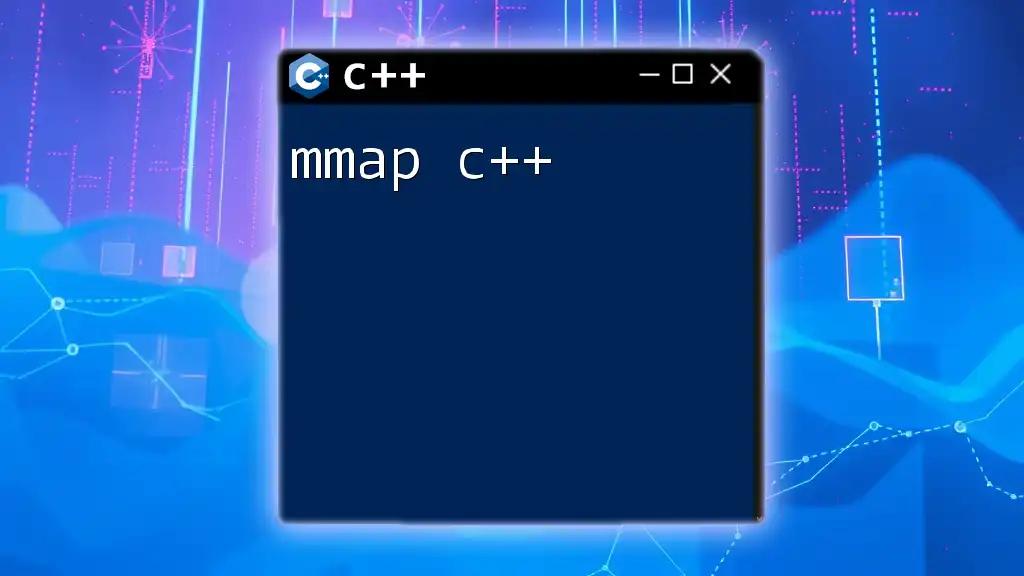
Best Practices for Heap Memory Management
Avoiding Memory Leaks
To avoid memory leaks, always ensure that every `new` operation has a corresponding `delete`. Track your allocations, and make it a practice to deallocate memory in the reverse order of allocation.
Using Smart Pointers
C++11 introduced smart pointers, which simplify memory management and help prevent common memory issues. Smart pointers automatically manage memory, meaning you don’t explicitly need to call `delete`.
Types of Smart Pointers
-
`unique_ptr`: Represents exclusive ownership of a dynamically allocated object. When the `unique_ptr` goes out of scope, the memory is automatically deallocated.
std::unique_ptr<int> smartPtr(new int);
-
`shared_ptr`: Represents shared ownership of a dynamically allocated object, allowing multiple pointers to share the same memory. The memory is automatically deallocated when the last `shared_ptr` pointing to it is destroyed.
-
`weak_ptr`: Does not affect the reference count of `shared_ptr`, providing a non-owning reference to an object managed by `shared_ptr`.
Using smart pointers means your program can handle memory more safely with fewer explicit delete calls, reducing the risk of leaks and dangling pointers.
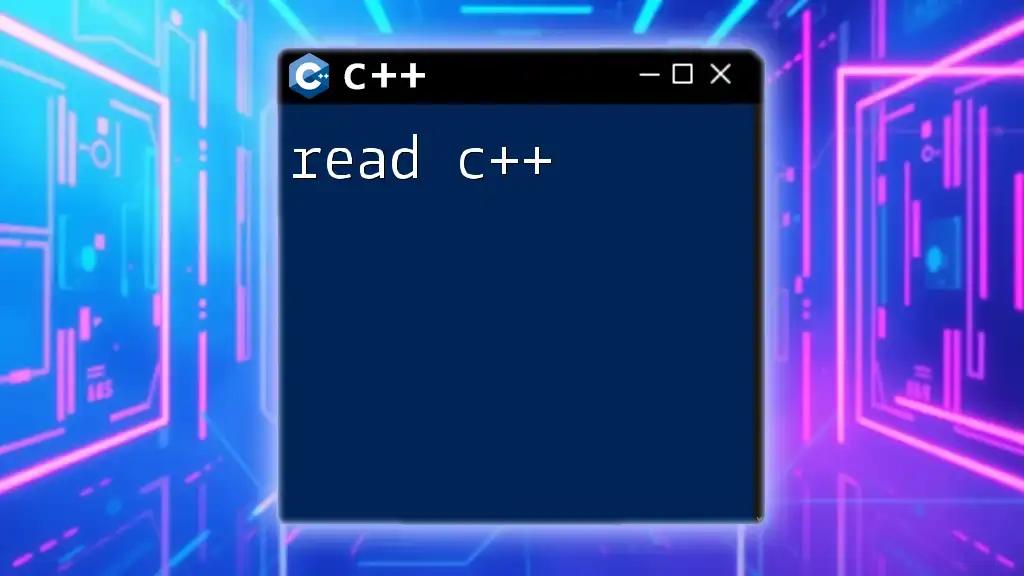
When to Use Heap over Stack
Performance Considerations
Heap memory allocation can offer significant performance advantages in specific scenarios, especially when dealing with large data structures. Unlike stack allocation, which is limited by fixed size, the heap allows you to allocate variable-sized data structures that can grow as needed.
Use Cases
Consider these situations where heap allocation is beneficial:
- Large Data Structures: Data structures like linked lists, trees, or graphs often require dynamic memory allocation.
- Dynamic Data Needs: If the size or number of objects is not known at compile time, heap allocation permits adaptability.
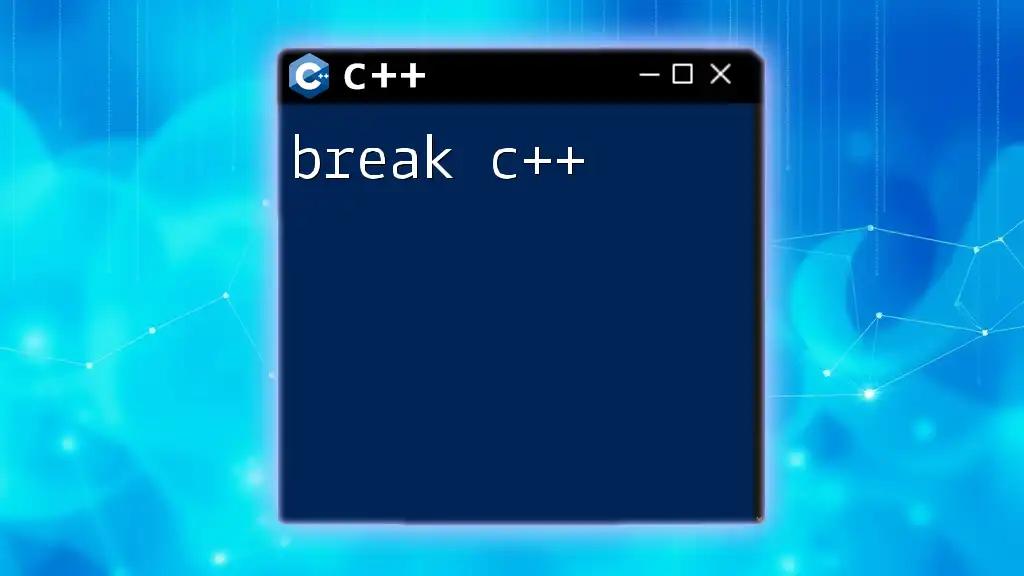
Common Mistakes in Heap Memory Management
Forgetting to Deallocate Memory
One of the most prevalent issues is neglecting to deallocate memory after use. This often leads to memory leaks, which can significantly affect the performance of long-running applications.
Double Deleting
Double deleting occurs when you attempt to delete the same memory twice. This can lead to undefined behavior, including crashes. Avoid this by always setting pointers to `nullptr` after deletion:
delete ptr;
ptr = nullptr; // Prevent double delete
Mismanagement of Pointers
Ensure to perform null checks before deletion to prevent dereferencing null pointers. Here's how:
if(ptr != nullptr) {
delete ptr;
}
This practice helps maintain program stability and reduces the risk of crashes.

Conclusion
Understanding the heap in C++ is crucial for effective memory management in programming. By mastering dynamic memory allocation and emphasizing best practices—such as using smart pointers and preventing memory leaks—you will write more efficient and safer code. As you grow in your programming journey, continue to practice and explore advanced techniques in heap memory management.