The `isalpha` function in C++ checks whether a given character is an alphabetic letter (either uppercase or lowercase). Here’s a simple example:
#include <iostream>
#include <cctype>
int main() {
char ch = 'A';
if (isalpha(ch)) {
std::cout << ch << " is an alphabetic character." << std::endl;
} else {
std::cout << ch << " is not an alphabetic character." << std::endl;
}
return 0;
}
What is `isalpha`?
The `isalpha` function is a part of the C++ Standard Library, specifically found in the `<cctype>` header file. It serves a critical role in character classification. This function checks whether a given character is an alphabetic letter—a key functionality in many programming scenarios, such as input validation and data processing. By utilizing `isalpha`, developers can ensure their applications handle user input more reliably and accurately.

How to Use `isalpha` in C++
Syntax of `isalpha`
The syntax for the `isalpha` function is straightforward:
int isalpha(int c);
In this declaration:
- The parameter `c` is an integer that represents a character (typically passed in as its ASCII value).
- The return value is a non-zero integer (true) if the character is alphabetic (A-Z or a-z) and zero (false) otherwise.
Example of `isalpha` in Action
Consider the following simple example that demonstrates how to use `isalpha`:
#include <iostream>
#include <cctype>
int main() {
char ch = 'A';
if (isalpha(ch)) {
std::cout << ch << " is an alphabetic character." << std::endl;
} else {
std::cout << ch << " is not an alphabetic character." << std::endl;
}
return 0;
}
In this instance, since `ch` is ‘A’, it is an alphabetic character. As expected, the output will state, "A is an alphabetic character." This code snippet effectively showcases how `isalpha` can check individual characters.

Practical Applications of `isalpha`
Input Validation
One of the critical applications of `isalpha` is in input validation scenarios. When accepting user input—especially in forms or command-line interfaces—it's essential to verify that users enter appropriate characters.
Here’s an example where we use `isalpha` to filter user input:
std::string input;
std::cout << "Enter a string: ";
std::cin >> input;
for (char c : input) {
if (isalpha(c)) {
std::cout << c << " is a letter." << std::endl;
} else {
std::cout << c << " is not a letter." << std::endl;
}
}
In this code, after capturing user input into `input`, each character is checked with `isalpha`. This establishes a clear way to provide feedback on the user’s input, indicating which characters are letters and which are not.
Data Processing
Another prevalent use of `isalpha` is in data processing, where you may need to parse strings or files and filter out unwanted characters. For instance, suppose you wanted to count the number of alphabetic characters in a string. You could implement the following function:
int countAlphaCharacters(const std::string& str) {
int count = 0;
for (char c : str) {
if (isalpha(c)) count++;
}
return count;
}
Here, the function `countAlphaCharacters` iterates through each character of the input string. It employs `isalpha` to check if the character is alphabetic, incrementing `count` each time it encounters a letter. This approach serves as a practical example of how `isalpha` can help in data analysis and manipulation.

Related Functions: `ischar` in C++
What is `ischar`?
There may be some confusion surrounding the term `ischar`. In the context of C++, `ischar` is not a standard function. Developers often mean to refer to `isalpha` or other similar functions for character classification purposes.
Usage of `ischar` (if applicable)
If you're looking to implement functionality similar to an assumed `ischar`, you would typically rely on `isalpha`, `isdigit`, or `isalnum` depending on your specific requirements. For instance, `isdigit` checks if a character is a numeral, while `isalnum` checks for alphanumeric characters. Understanding these distinctions helps prevent confusion and streamlines character evaluations in your code.
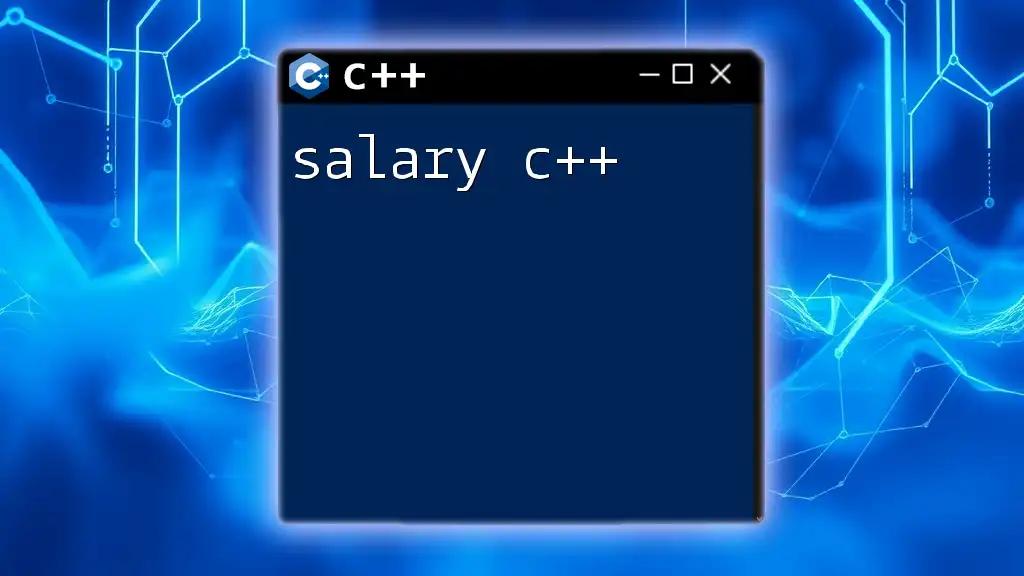
Best Practices for Using `isalpha` in C++
Performance Considerations
While character classification functions like `isalpha` are generally efficient, it's vital to use them judiciously, especially in performance-critical applications. Minimize unnecessary calls by first checking if the input character is in an expected range (for instance, within the ASCII values for alphabetic characters) before invoking `isalpha`. This can help avoid potential overhead.
Error Handling
When utilizing `isalpha`, pay careful attention to input validation and error handling. Always ensure that the characters passed to `isalpha` are valid to avoid undefined behavior. Wrapping the character checks with appropriate error-handling mechanisms can prevent your application from crashing or reacting unpredictably.
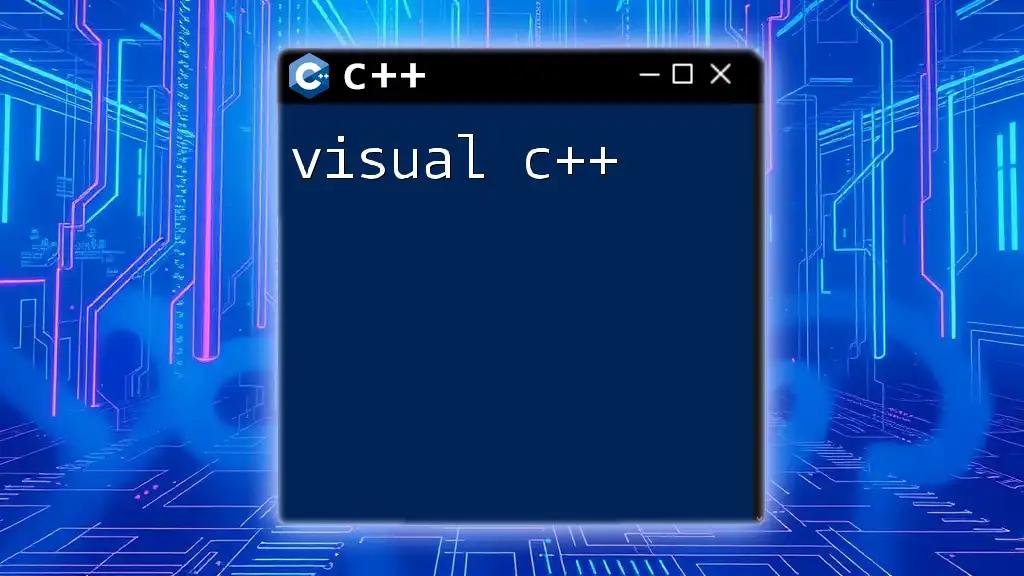
Conclusion
In summary, mastering the `isalpha` function in C++ is invaluable for validating character input, performing data processing tasks, and ensuring safer user interactions. This function exemplifies the ease with which C++ can handle character classifications, providing a solid foundation for more complex programming tasks.
Encourage continued exploration and practice with `isalpha` and similar functions, as they are indispensable tools in any C++ developer's toolkit. If you have questions or experiences to share regarding character classification in your projects, feel free to leave them in the comments!
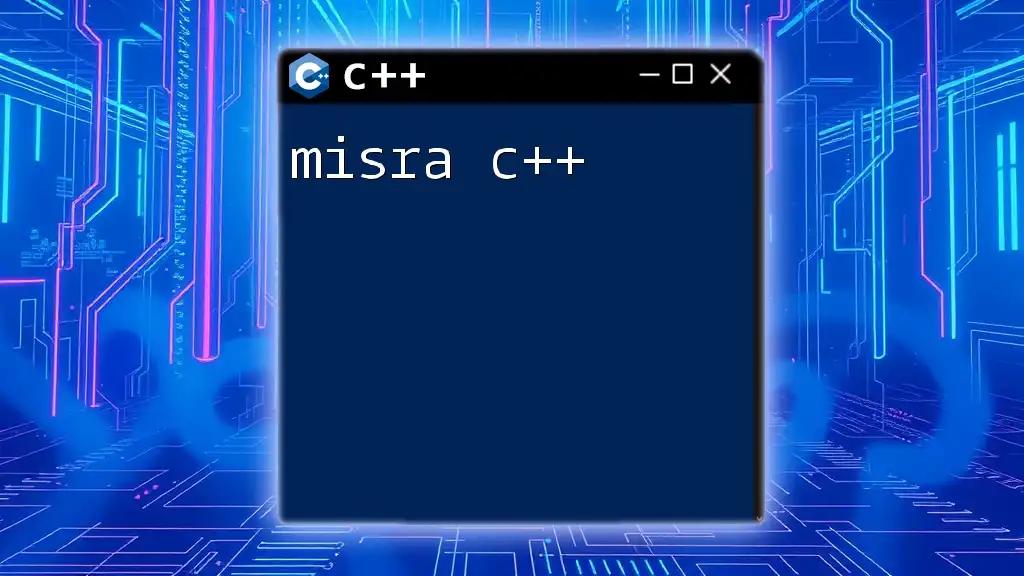
Additional Resources
For more in-depth study, refer to the official C++ documentation for the `<cctype>` header and its character classification functions. Consider seeking out additional resources and tutorials that cover character handling and input validation to further strengthen your skills in C++.