`alpaca.cpp` is a C++ program that demonstrates a simple implementation of an Alpaca class, allowing you to create and manipulate Alpaca objects using basic functionality.
#include <iostream>
#include <string>
class Alpaca {
public:
Alpaca(std::string name) : name(name) {}
void speak() {
std::cout << name << " says: *bleat*" << std::endl;
}
private:
std::string name;
};
int main() {
Alpaca alpaca("Alfred");
alpaca.speak();
return 0;
}
Understanding `alpaca.cpp`
What is `alpaca.cpp`?
`alpaca.cpp` is a modern C++ library designed for ease of use and rapid development. It allows developers to leverage a variety of commands in a streamlined manner, enhancing productivity and reducing the learning curve associated with complex C++ commands. This library has evolved over time, inspired by the need for simpler, more accessible C++ functionalities.
Why Use `alpaca.cpp`?
The advantages of using `alpaca.cpp` are manifold. Primarily, it provides a concise API that lets developers execute commands without delving into the often intricate details of standard C++. Compared to other libraries, `alpaca.cpp` offers a more intuitive design, making it an ideal choice for both novice and experienced programmers.
One of the notable applications of `alpaca.cpp` is in data processing, where its simplicity can drastically reduce development time. Moreover, it is used in various fields like game development, system automation, and web scripting, which underscores its versatility.
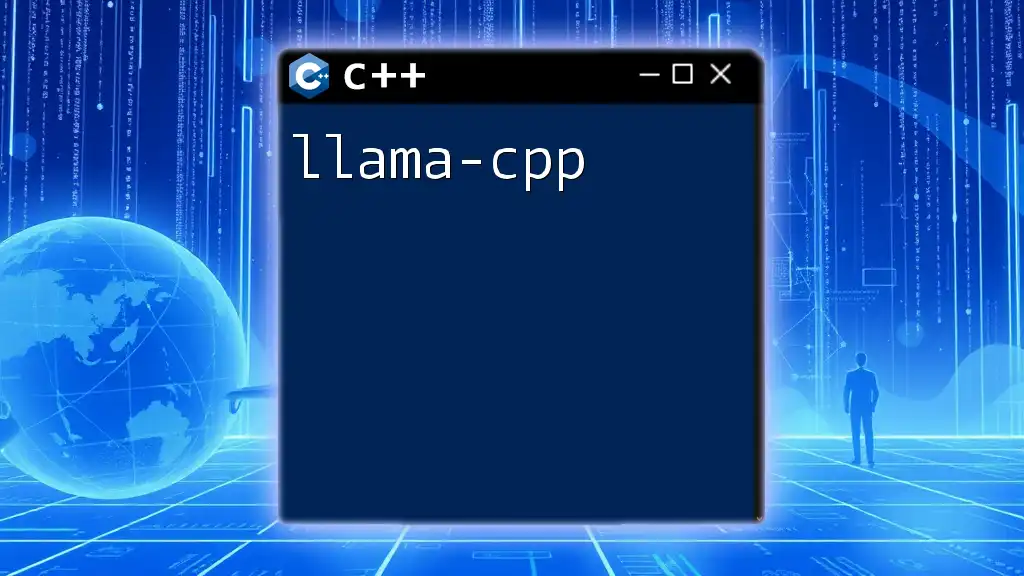
Getting Started with `alpaca.cpp`
Installation and Setup
Setting up `alpaca.cpp` is a straightforward process. First, ensure that your development environment meets the following requirements:
- A C++ compiler (GCC, Clang, or MSVC)
- CMake for building the library
To install `alpaca.cpp`:
- Clone the repository: Use Git to pull the latest version.
git clone https://github.com/your-repo/alpaca.cpp.git cd alpaca.cpp
- Build the project:
mkdir build cd build cmake .. make
- Link to your project: Ensure your CMake or IDE settings point to the `alpaca.cpp` installation.
Basic Structure of `alpaca.cpp`
`alpaca.cpp` is organized into several key components, each serving specific functions:
- Core commands: These are the primary functions you'll call to perform tasks with `alpaca.cpp`.
- Helpers: A collection of utility functions that simplify work with core commands.
- Modules: Specialized components that address specific domains or functionalities, such as arithmetic operations or string handling.
Understanding this structure is crucial for leveraging `alpaca.cpp` effectively.
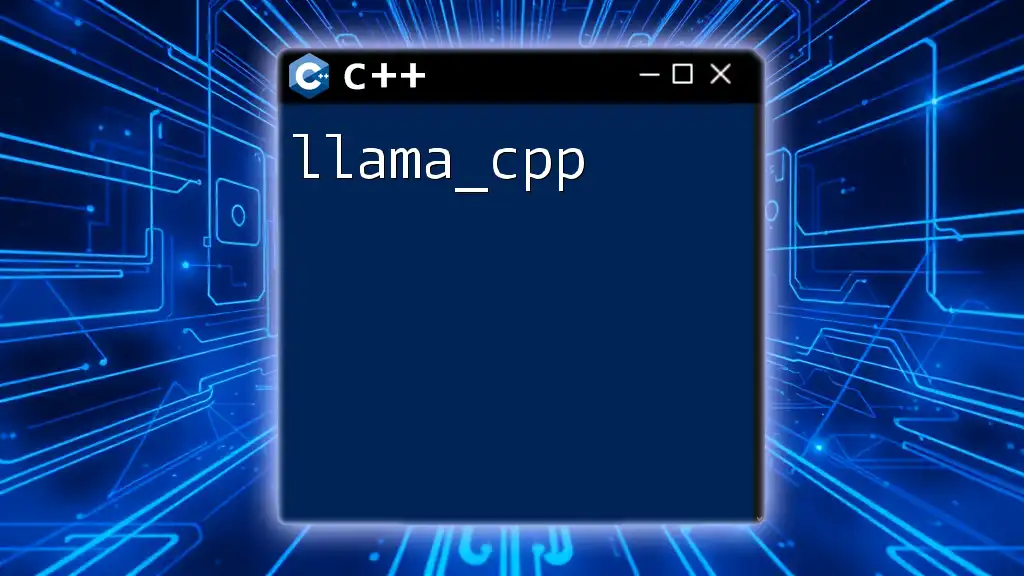
Core Concepts in `alpaca.cpp`
Commands and Syntax
At the heart of `alpaca.cpp` are its commands. These commands follow a simple syntax that makes them easy to remember and implement. Here’s an overview of common commands:
- Initialization: Start using the library.
#include "alpaca.cpp" alpaca::init();
- Execution: Run a command.
alpaca::execute("Hello, World!");
This basic example highlights the command structure and shows how straightforward it is to utilize the library.
Variables and Data Types
Variables in `alpaca.cpp` are declared in a manner consistent with C++. The library supports various data types, including:
- Integers
- Floats
- Strings
Here’s how you can declare and use variables:
int count = 10;
float average = 5.5;
std::string message = "Count is ";
alpaca::execute(message + std::to_string(count));
Functions in `alpaca.cpp`
Defining functions is essential for developing modular code. `alpaca.cpp` makes it easy to create and use functions. Here’s a simple example of a function that prints a welcome message:
void sayHello() {
std::cout << "Hello from alpaca.cpp!" << std::endl;
}
// Call the function
sayHello();
This illustrates how you can encapsulate functionality, promoting code reuse and clarity.
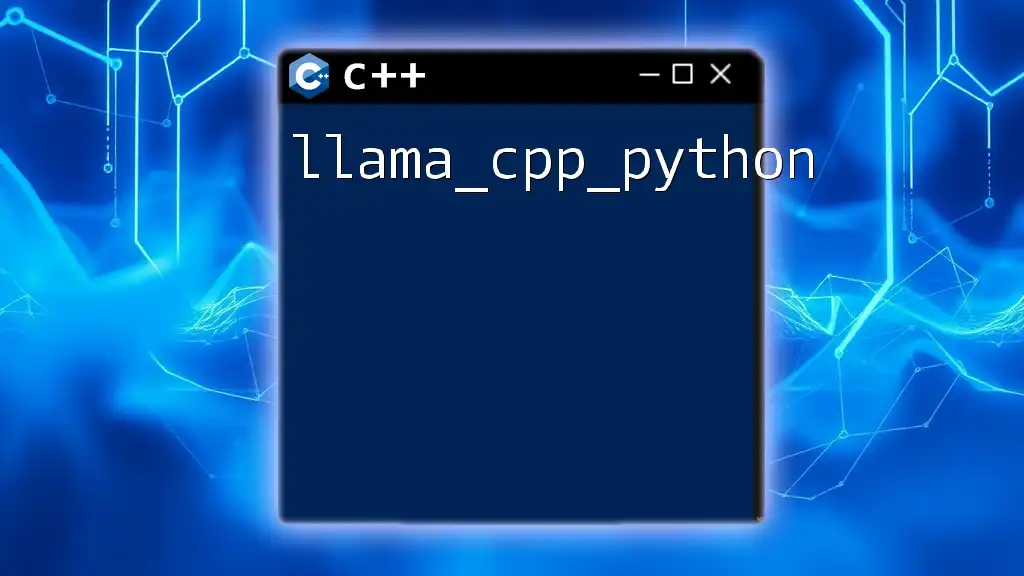
Advanced Topics in `alpaca.cpp`
Error Handling and Debugging
Error handling is imperative in programming. `alpaca.cpp` utilizes exceptions to manage errors gracefully. Common errors include incorrect command syntax or invalid function calls.
Here’s a sample code demonstrating error handling:
try {
alpaca::execute("some risky command");
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
This implementation helps maintain program stability by providing meaningful feedback when something goes wrong.
Integrating `alpaca.cpp` with Other Libraries
`alpaca.cpp` is designed to work well with other C++ libraries. For instance, let's consider integrating `alpaca.cpp` with a hypothetical library called `otherLibrary`:
#include "otherLibrary.h"
#include "alpaca.cpp"
otherLibrary::function();
alpaca::execute("Combined functionality!");
This showcases how you can extend the capabilities of your application by leveraging multiple libraries.
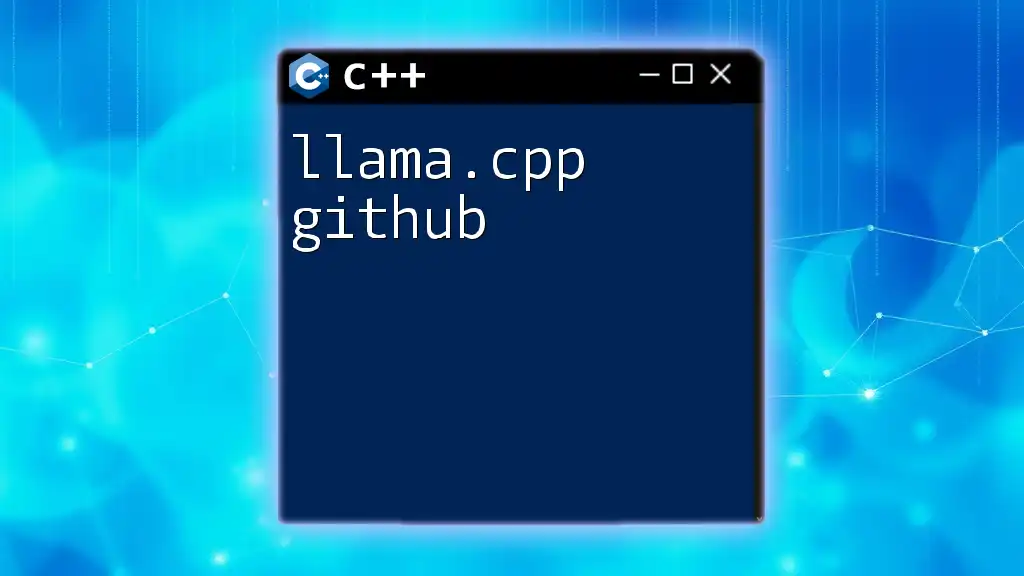
Best Practices for Using `alpaca.cpp`
Coding Standards and Style
Adhering to coding standards enhances readability and maintainability. Here are some recommended practices:
- Use descriptive names for variables and functions.
- Keep functions short and focused on a single task.
- Comment on complex sections of your code.
Performance Optimization
Optimize your code by:
- Minimizing unnecessary calculations.
- Using `const` where appropriate to prevent modifications.
- Profiling your code to identify bottlenecks.
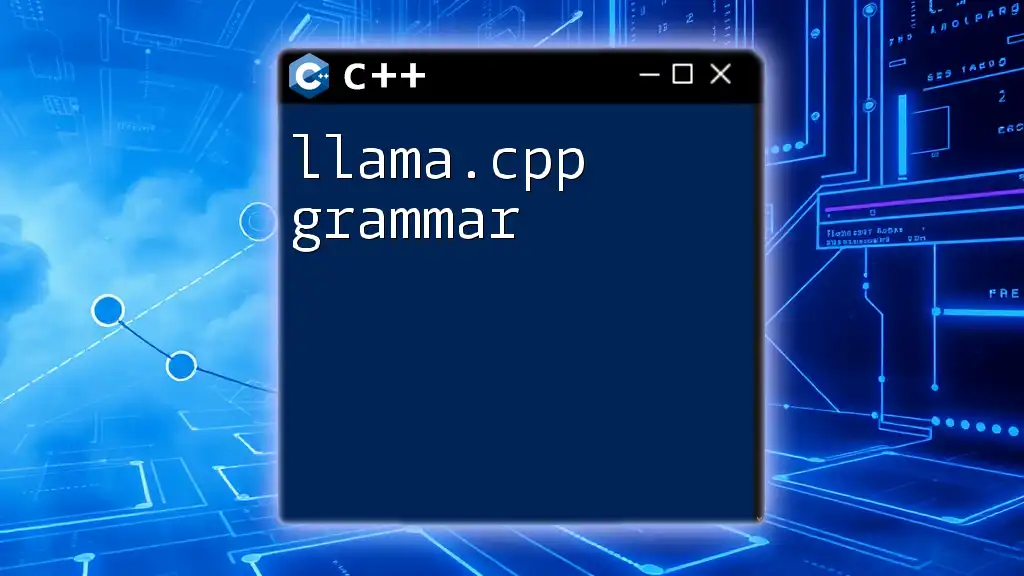
Troubleshooting Common Issues
Frequently Encountered Problems
While using `alpaca.cpp`, you may run into some common issues:
- Installation errors: Ensure you have the correct environment set up.
- Command execution issues: Always check for typos or syntax errors in your commands.
If you encounter issues, consult the community forums or the official documentation for solutions.
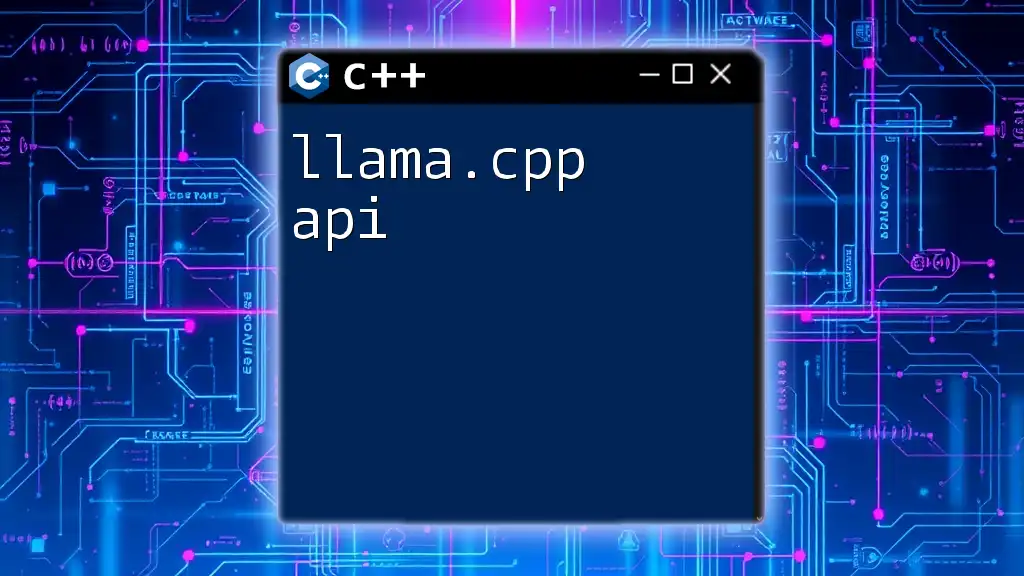
Conclusion
Throughout this guide, we explored the functionalities, advantages, and best practices associated with `alpaca.cpp`. This library offers a powerful yet accessible platform for C++ developers, providing a simplified approach to executing commands. Dive into `alpaca.cpp` today, implement the examples provided, and join our community for ongoing support and resources!
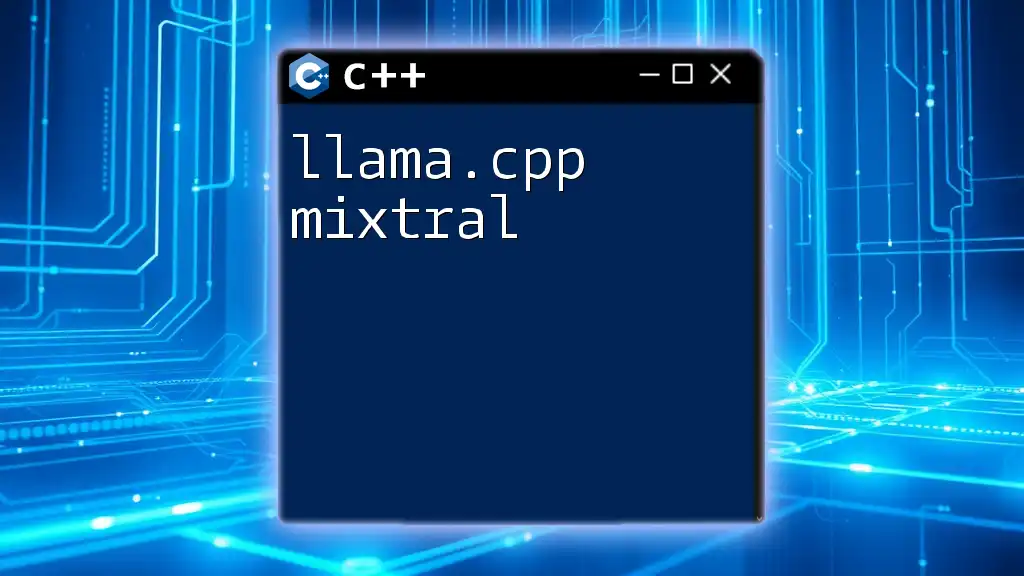
Additional Resources
For further learning:
- Refer to well-known C++ programming books that cover broader concepts.
- Join online forums like Stack Overflow or dedicated C++ communities.
- Always check the reference documentation for the latest features and updates in `alpaca.cpp`.
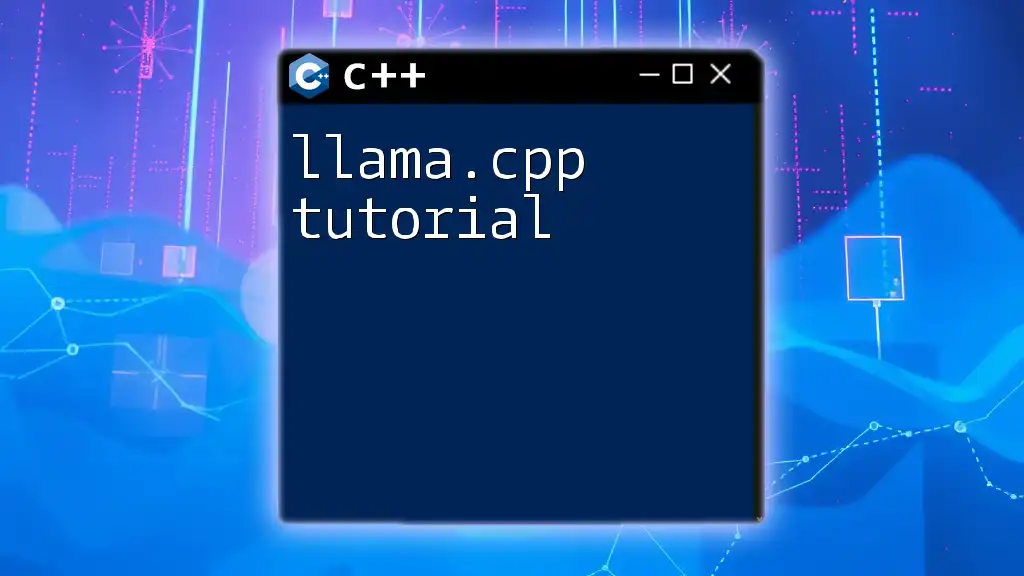
FAQs
Frequently Asked Questions
-
What are the prerequisites for using `alpaca.cpp`? Users should have a basic understanding of C++ programming and familiarity with using libraries.
-
Can `alpaca.cpp` handle advanced data types? Yes, it supports custom and complex data types, allowing for advanced programming techniques.