The "llama.cpp" project on GitHub provides a streamlined implementation of the LLaMA (Large Language Model Meta AI) architecture using C++, allowing developers to efficiently utilize and modify the model for their applications.
Here's a code snippet to demonstrate how to include the llama.cpp library in your C++ project:
#include "llama.h"
int main() {
LlamaModel model("path/to/model");
model.load();
model.generate("Hello, world!");
return 0;
}
What is Llama.cpp?
Llama.cpp is an innovative open-source project aimed at simplifying the learning curve associated with C++ programming. Often seen as a complex and intimidating language, C++ can be made more approachable through structured guidance and examples, which is precisely what Llama.cpp offers. This project provides both beginners and seasoned developers with practical insights into C++ commands and concepts, facilitating an effective learning environment.
Understanding the functionalities encapsulated within Llama.cpp is crucial for anyone looking to master C++. The repository offers real-world examples and a clear layout that guides users through various aspects of C++ programming.
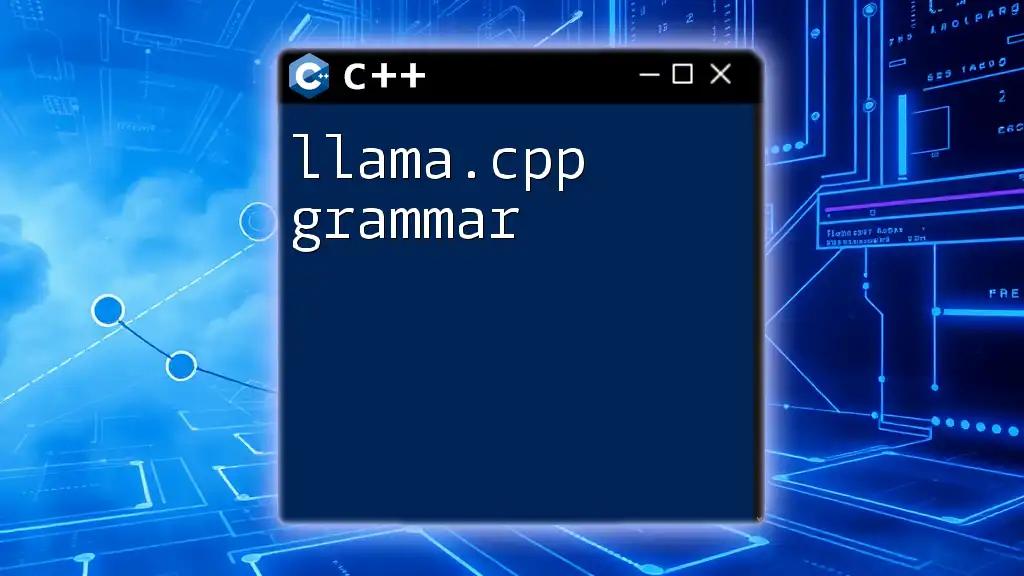
Why Explore Llama.cpp on GitHub?
GitHub serves as the central hub for developers to host, manage, and collaborate on their code projects. Exploring Llama.cpp on GitHub provides an opportunity to dive into a well-structured codebase. By analyzing this repository, you can gain immediate insights into:
- Best Practices: How to effectively organize and write C++ code.
- Real-World Applications: Understand common patterns that appear in real-world codebases.
- Community Contributions: Engage with a community of developers who are equally passionate about C++.
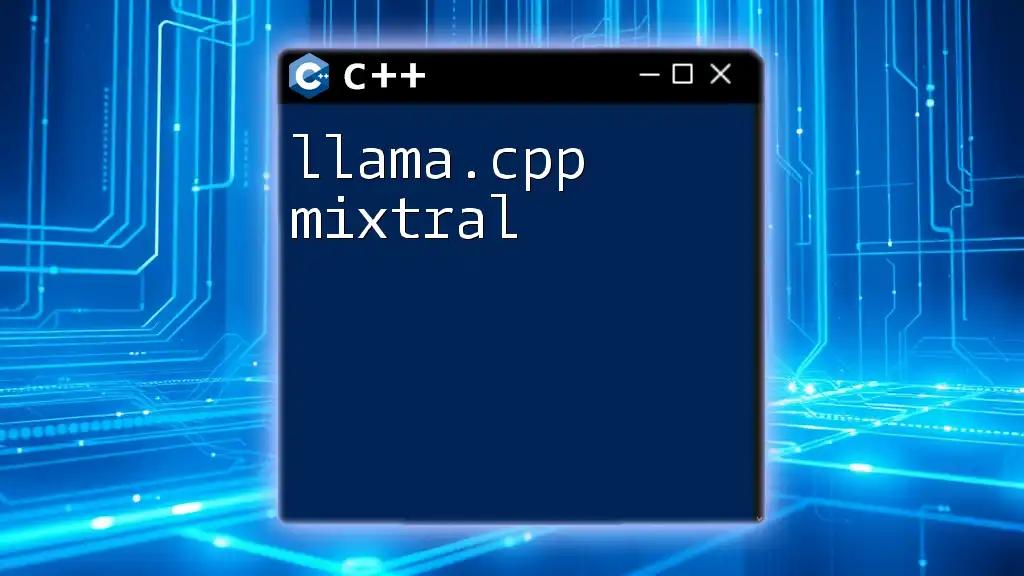
Setting Up Your Environment for Llama.cpp
Prerequisites for Working with Llama.cpp
Before diving into Llama.cpp, ensure that your development environment is equipped with the following tools:
- C++ Compiler: Install a suitable C++ compiler like GCC or Clang.
- Git: Essential for version control and collaboration.
- Integrated Development Environment (IDE): Consider using IDEs like Visual Studio, JetBrains CLion, or Code::Blocks for a more user-friendly coding experience.
How to Clone the Llama.cpp Repository
To get started, you will need to clone the repository to your local machine. Open your command-line interface and run the following command:
git clone https://github.com/username/llama.cpp.git
This command allows you to download all the files from the Llama.cpp GitHub repository, placing them in a new directory on your system. After cloning, navigate into the directory with:
cd llama.cpp

Understanding the Structure of Llama.cpp
Project Directory Breakdown
One of the first steps to effectively use Llama.cpp is to familiarize yourself with its directory structure. This will not only help you navigate the project smoothly but also understand where to find specific functionalities. Key folders and files include:
- `/src` Directory: Contains the source code files responsible for core functionalities. This is where the primary logic of the application resides.
- `/include` Directory: Holds the header files that declare functions and classes, crucial for the compilation of the project.
Key Components of Llama.cpp
Source Files
In the `/src` directory, you'll find various `.cpp` files that implement the core features of the Llama.cpp project. Each source file is designed to encapsulate specific functionalities. For example:
// Example functionality in source file
#include "example.h"
void performExample() {
// Function implementation
std::cout << "Hello from example!" << std::endl;
}
This snippet showcases a simple function defined within a source file, demonstrating how functionalities are implemented.
Header Files
Header files located in the `/include` directory serve a vital role in C++ projects. By containing declarations of classes and functions, they enable code organization and modularity. Below is an example of a header file:
// example.h
#ifndef EXAMPLE_H
#define EXAMPLE_H
void performExample();
#endif
In this header file, the declaration of the `performExample` function is provided. Proper use of header files ensures code readability and reusability.
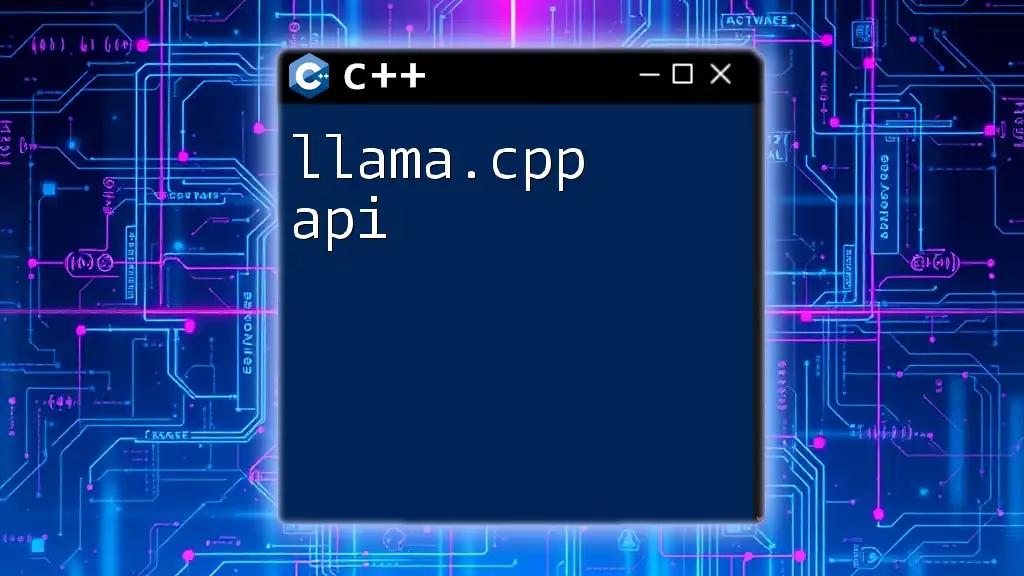
Core Concepts in Llama.cpp
Key Features of Llama.cpp
Llama.cpp brings several unique features to the forefront, aiding in both learning and application development. These features include intuitive command implementations, well-commented code, and a focus on modular programming concepts, which are essential for writing maintainable and scalable C++ code.
Using CPP Commands Effectively
Understanding and using C++ commands within the context of Llama.cpp is crucial for learning. The project leverages specific cpp commands to showcase their functionalities effectively. For instance, the use of loops, conditionals, and functions are demonstrated throughout the codebase.
A practical example of utilizing a loop can be shown as follows:
for (int i = 0; i < 10; ++i) {
std::cout << "Iteration: " << i << std::endl;
}
This snippet illustrates a simple loop that prints out the iteration count, serving as a basic yet important concept in C++ programming.
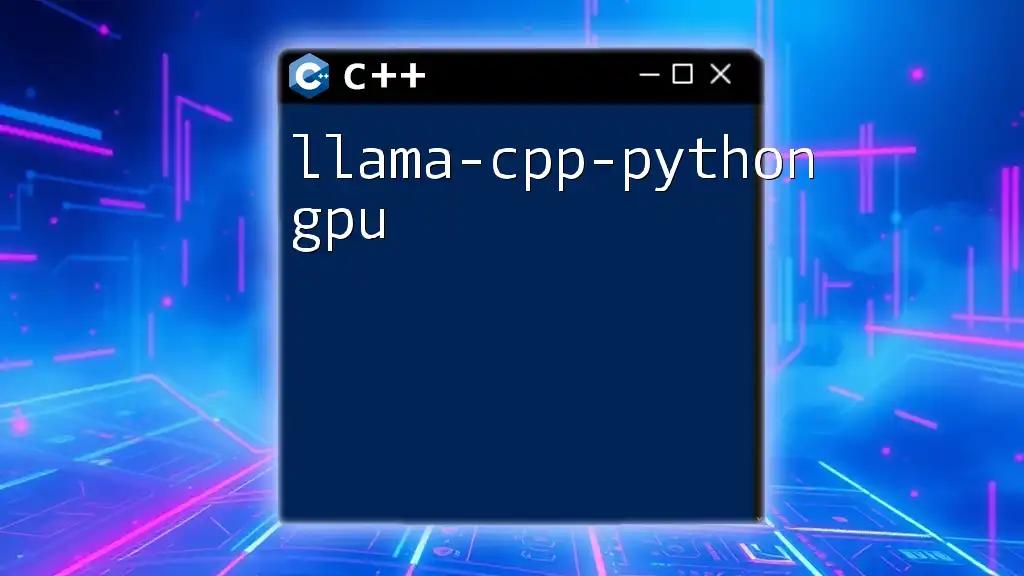
Contributing to Llama.cpp
How to Contribute to the Project
Engaging with the Llama.cpp community can enhance your learning experience. Here’s how you can contribute:
- Fork the Repository: Create your own copy of the project by forking the repository on GitHub.
- Make Changes: Implement new features, fix bugs, or improve documentation in your local version.
- Submit a Pull Request: Propose your changes by submitting a pull request to the original repository. Always ensure that your changes follow the project's coding standards and guidelines.
Best Practices for Contributions
When contributing, adhere to the following best practices:
- Write Clean and Maintainable Code: Ensure your code is well-structured and follows conventions. Use meaningful variable names and maintain consistent formatting.
- Document Your Code: Providing comments and documentation enhances the readability and usability of your code for others.
- Follow Code Review Etiquette: Be open to feedback and actively participate in discussions about your contributions.
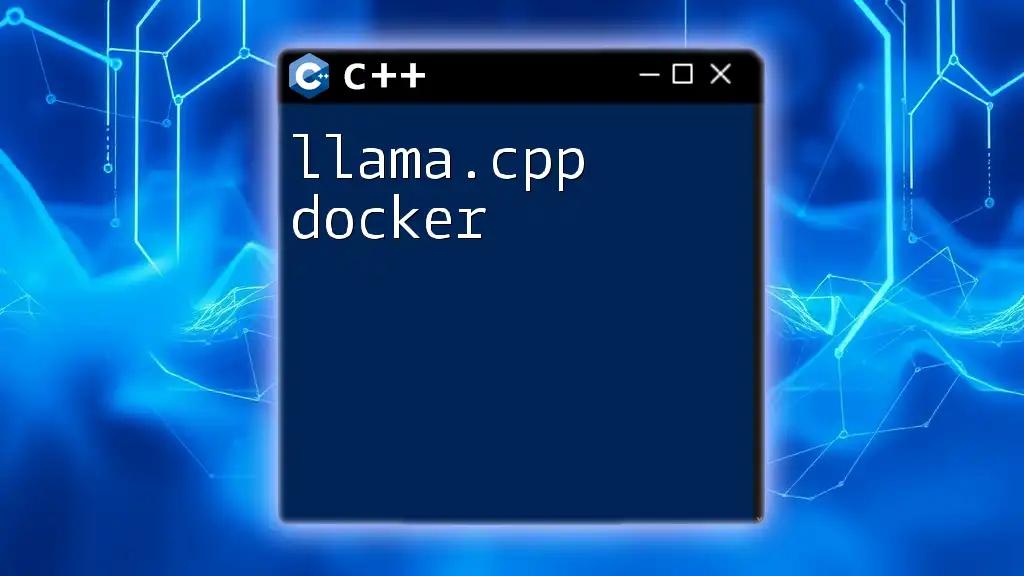
Learning Resources for Llama.cpp
Additional Learning Material
To further deepen your understanding of C++, consider seeking additional learning materials. Books like "C++ Primer" and online platforms offering courses (Coursera, Udemy) can effectively supplement your learning journey.
Community and Support
Joining forums or discussion groups focused on C++ development can be beneficial for networking and knowledge sharing. Platforms like Stack Overflow, Reddit, and even GitHub Discussions can provide valuable insights and answers to your questions.
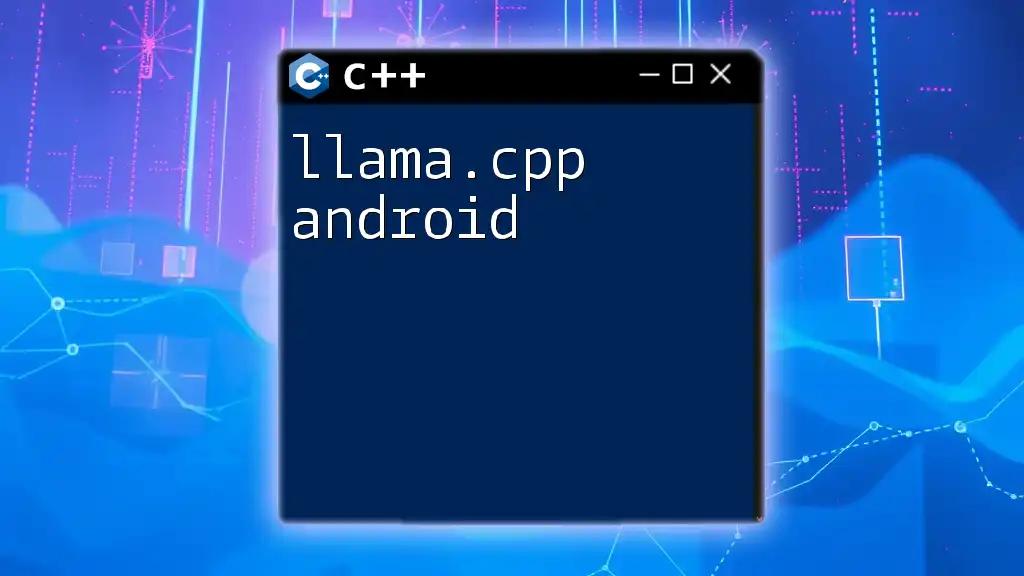
Conclusion
By exploring Llama.cpp on GitHub, you can accelerate your journey in learning C++. Utilize this resource to understand core C++ commands, engage with the community, and apply best practices in your coding endeavors. Each step taken within this project paves the way for greater comfort and proficiency in C++ programming.
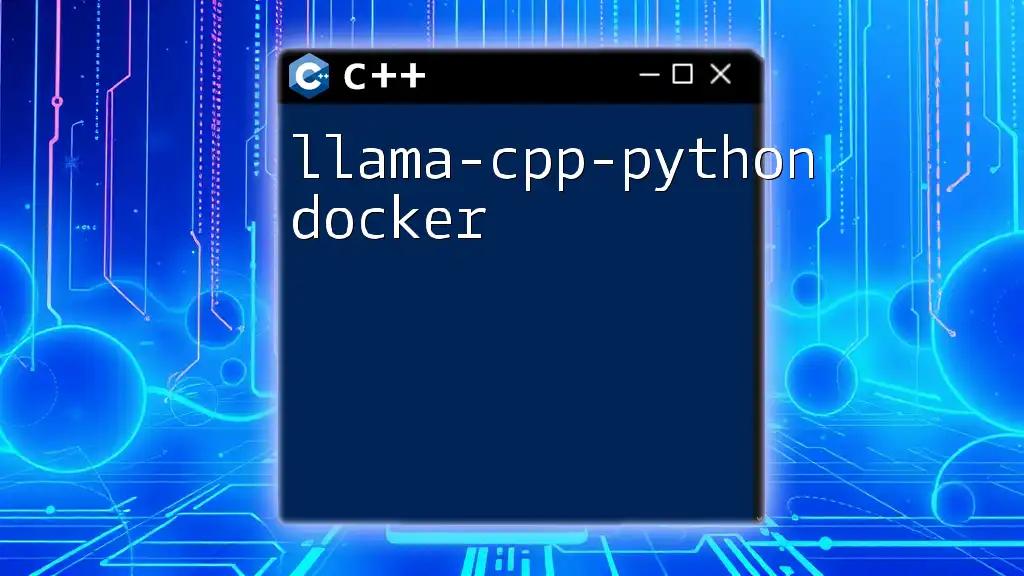
FAQs
Common Questions About Llama.cpp and GitHub
What are the prerequisites to understanding Llama.cpp?
A basic understanding of programming concepts and a desire to learn C++ is essential. Familiarity with Git and version control will enhance your experience.
How can I best utilize GitHub when working on C++ projects?
Utilize GitHub's features for collaboration—forking repositories, creating branches, and submitting pull requests are key aspects of working effectively on open-source projects.