The "llama-cpp-python server" refers to a server setup that enables the use of Llama C++ models within Python applications to facilitate efficient model deployment and interaction.
Here’s a simple code snippet to demonstrate how to initialize and run a Llama C++ model server in Python:
#include <iostream>
#include "llama_cpp_model.h"
int main() {
llama_cpp::Model model("path/to/model");
model.load();
std::cout << "Llama CPP model server is running..." << std::endl;
model.run();
return 0;
}
What is Llama-CPP-Python?
Llama-CPP-Python is a high-performance library that bridges the gap between C++ and Python, enabling developers to leverage the speed of C++ programming within the flexibility of Python. This powerful combination allows for rapid development cycles while still maintaining efficient execution.
Key Features
The Llama-CPP-Python server is designed with several advantageous features:
-
Fast Execution: By utilizing C++ code, which is compiled to machine language, the Llama server can execute tasks significantly faster than pure Python scripts.
-
Easy Integration with Python Scripts: The library simplifies complex C++ functionalities, wrapping them in a Pythonic interface that makes them accessible to developers familiar only with Python.
-
Flexible Architecture: The modular approach of Llama allows for easy customization and extension, making it suitable for various applications, from data processing to machine learning.
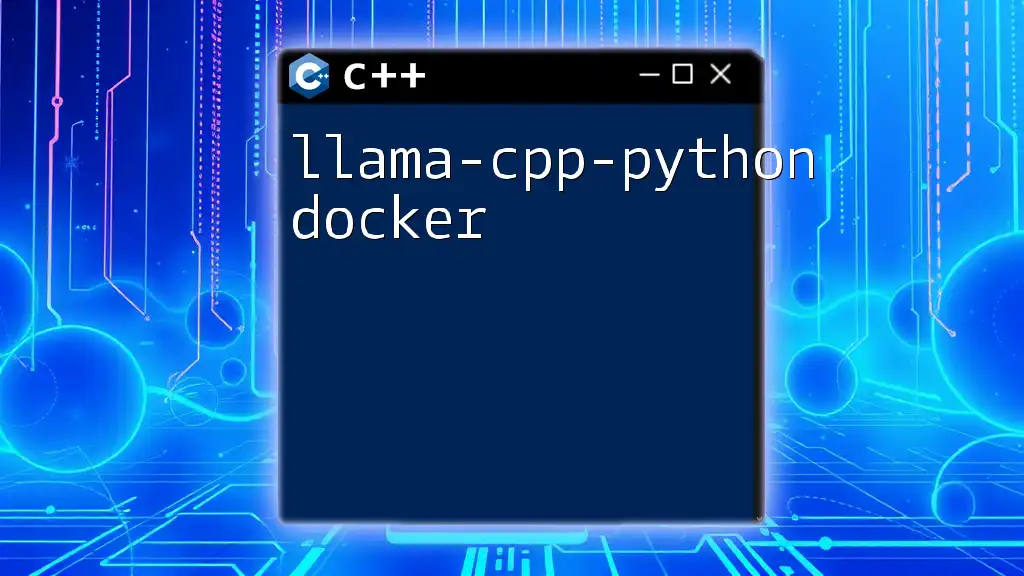
Setting Up Llama-CPP-Python Server
Prerequisites
Before diving into the installation of the Llama-CPP-Python server, ensure that you meet the following prerequisites:
-
System Requirements: Make sure your hardware specifications are sufficient for the tasks you plan on running. A standard modern computer should suffice.
-
Installation Requirements: Llama-CPP-Python requires a compatible Python version (preferably Python 3.x) and a capable C++ compiler such as GCC or Clang to compile any necessary C++ code.
Installation Steps
Installing Llama-CPP-Python
The installation process is straightforward. Use the terminal or command line window to execute the following command:
pip install llama-cpp-python
This command will fetch and install the Llama-CPP-Python library along with any dependencies.
Verifying the Installation
Once installation is complete, it’s essential to verify the installation's success. Open a Python shell or your preferred IDE, and execute:
import llama_cpp
print(llama_cpp.__version__)
If the output displays the version number without any import errors, your installation is successful.
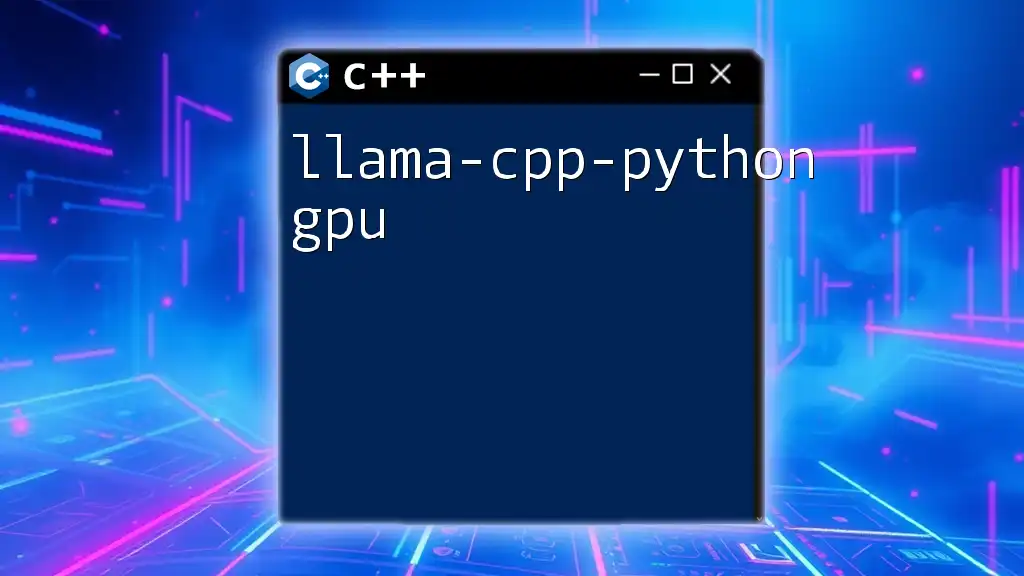
Creating Your First Llama-CPP-Python Server
Understanding Server Architecture
To effectively use the Llama-CPP-Python server, it’s crucial to understand its architecture. The server comprises several key components, including:
- Core Module: The heart of the server, managing requests and responses.
- Communication Interface: Handles interactions with Python scripts for request processing.
- Data Management Layer: Manages data storage and retrieval for efficient operations.
Writing Your First Server Script
Setting up your first Llama-CPP-Python server is simple. Here’s how to get started:
Setting Up the Environment
Choose a code editor like VSCode or PyCharm to write your script. Make sure to set your Python environment correctly to avoid any path issues.
Sample Code Snippet
Here is a basic server setup to kick off your project:
from llama_cpp import LlamaServer
server = LlamaServer()
server.start()
print("Server is running...")
This script imports the Llama server class, initializes it, and starts the server. The printed confirmation indicates that your server is now active.
Testing Your Server
To test the server’s functionality, you can send a request and observe the response:
response = server.process_request({'input': 'Hello, Llama!'})
print(response)
In this example, the server processes a simple input string and returns the output, demonstrating that your server setup is functioning correctly.
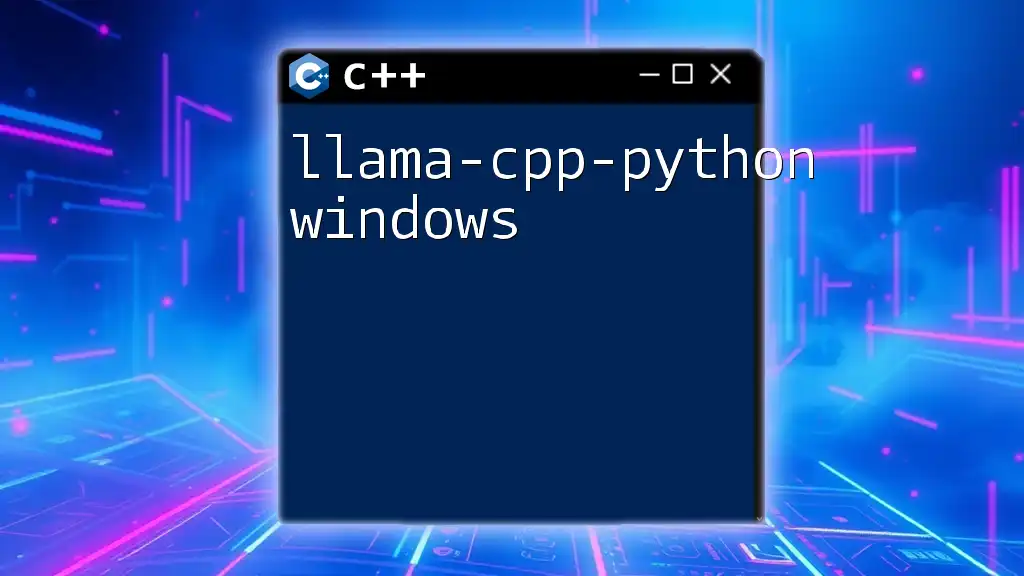
Advanced Usage of Llama-CPP-Python Server
Optimizing Performance
For performance-critical applications, consider implementing cache management strategies to reduce processing times, particularly for repeated requests. Additionally, managing concurrent requests can significantly enhance the server's responsiveness:
- Cache Management: Utilize appropriate caching techniques to store frequent responses.
- Concurrency: Incorporate threading or asynchronous programming to handle multiple requests simultaneously.
Integration with Other Libraries
One of the strengths of the Llama-CPP-Python server is its ability to integrate seamlessly with popular libraries, like TensorFlow or Flask.
Using Llama with TensorFlow
When integrating Llama with TensorFlow, you can leverage its computational power for deep learning applications. Here’s a simple integration example:
import tensorflow as tf
from llama_cpp import LlamaServer
server = LlamaServer()
# TensorFlow model processing logic here
Using Llama with Flask
To create a web service using Flask and the Llama-CPP-Python server, follow this example:
from flask import Flask, request
from llama_cpp import LlamaServer
app = Flask(__name__)
server = LlamaServer()
@app.route('/process', methods=['POST'])
def process():
input_data = request.json['input']
return server.process_request({'input': input_data})
if __name__ == '__main__':
app.run(debug=True)
In this setup, you define a Flask application that listens for POST requests to the `/process` endpoint, which in turn communicates with the Llama server for processing.
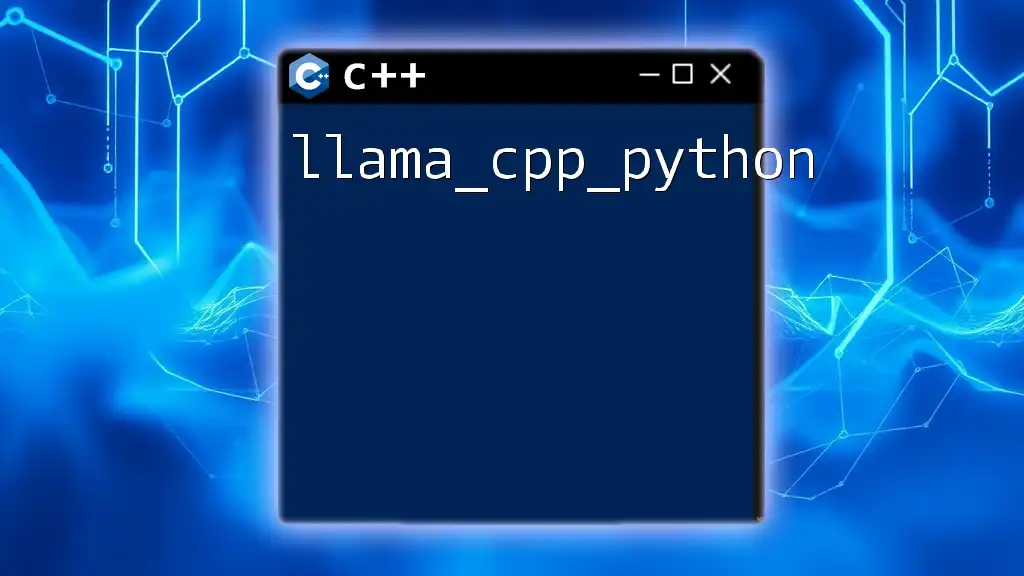
Troubleshooting Common Issues
Installation Failures
As with any library, installation issues may arise. Common errors may include missing dependencies or permissions issues. Ensure that you have installed all required libraries and have the necessary permissions to install packages.
Runtime Exceptions
When running your server, you might encounter runtime exceptions. Debugging these can be made simpler by reading the stack traces carefully. Implementing a logging mechanism within your server can also provide better visibility into runtime events and help diagnose issues quickly.
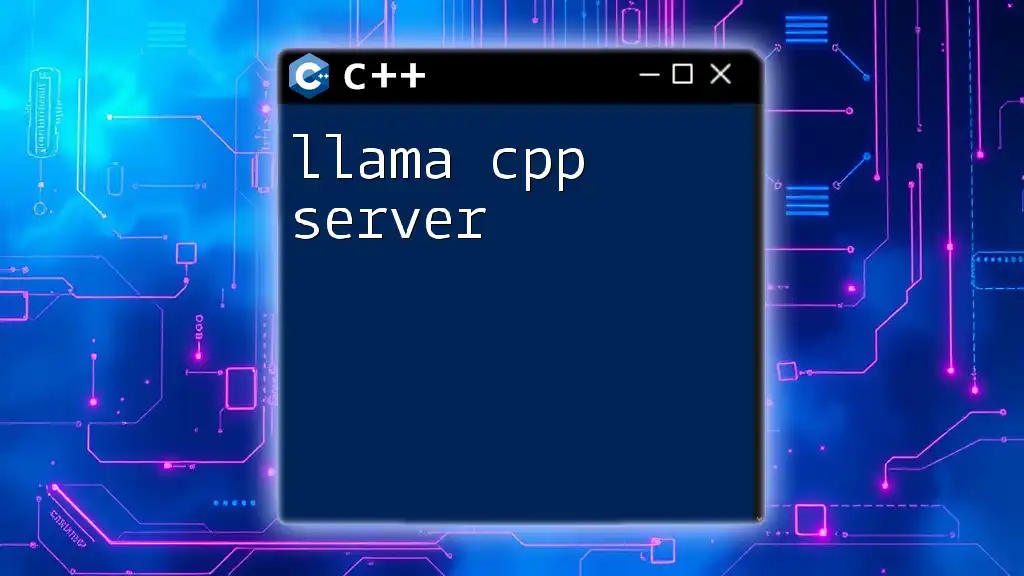
Best Practices for Using Llama-CPP-Python
Code Organization
Keeping your project organized is crucial for maintainability. Adopting a modular structure allows for easier updates and testing of individual components without affecting the entire application.
Documentation and Comments
Good documentation is vital. Ensure that your code is well-commented, explaining complex logic or specific decisions made within your scripts. This practice assists not only your future self but also team members or contributors who may work on the project later.
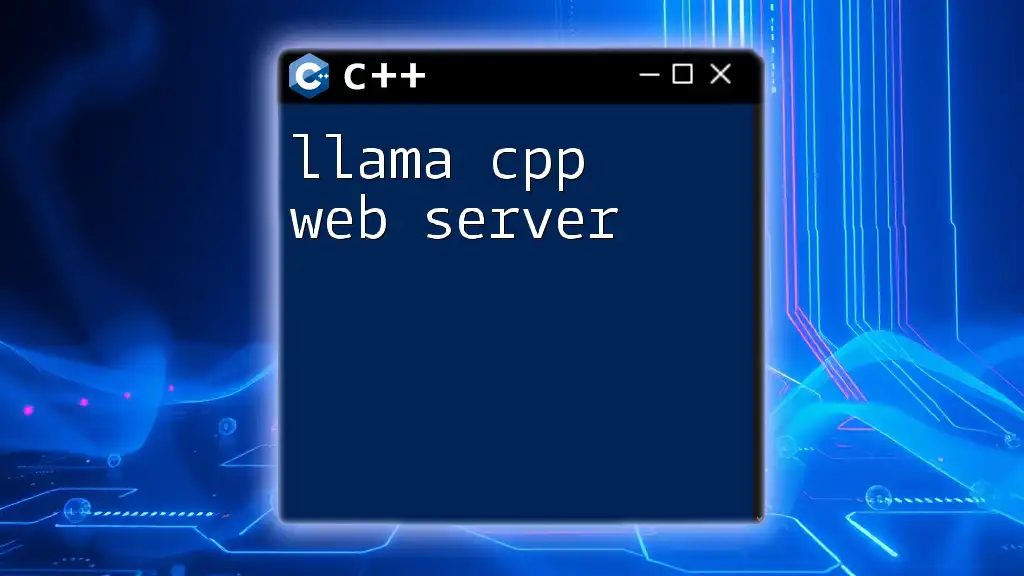
Conclusion
In this guide, we explored the Llama-CPP-Python server, highlighting its capabilities, installation process, and how to create and run servers efficiently. By integrating C++ with Python, developers can harness the best of both worlds—speed and accessibility—enhancing their projects and applications.
With a strong foundation now laid out, you are encouraged to continue exploring and experimenting with this powerful server to unlock its full potential in your development endeavors.
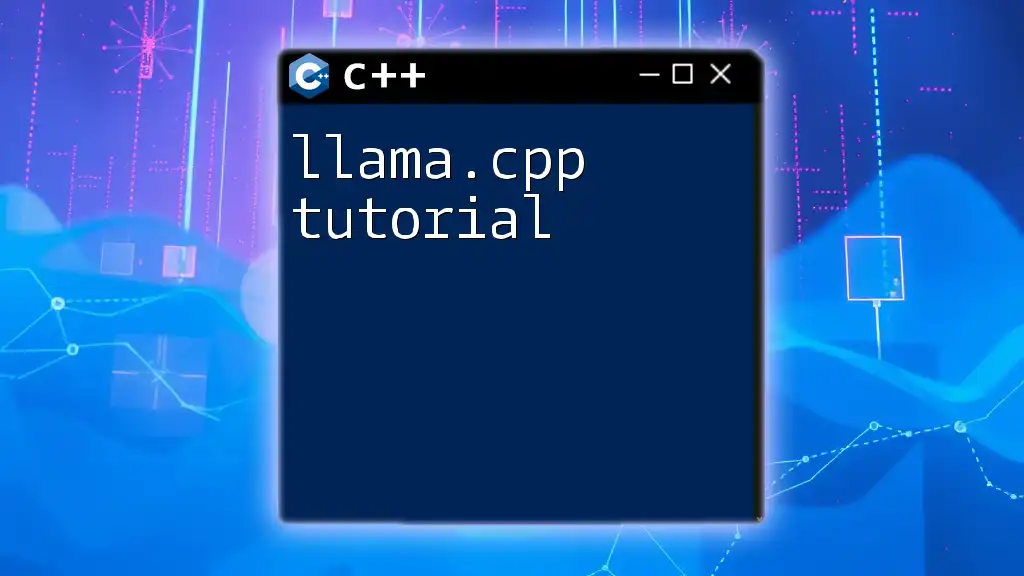
Further Resources
For those looking to dive deeper into the Llama-CPP-Python library, consider reviewing the official documentation, participating in community forums, or enrolling in additional learning resources tailored to enhance your programming skills and knowledge on C++ and Python integration.
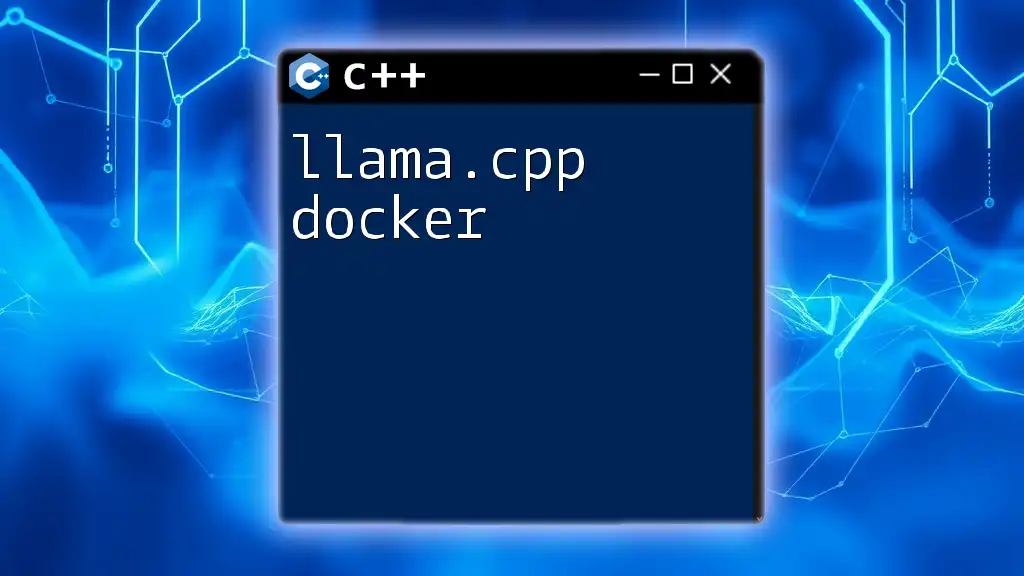
Call to Action
We invite you to share your own experiences using the Llama-CPP-Python server! What challenges did you face, and how did you overcome them? Don't forget to subscribe for future tutorials and tips on effectively using C++ commands in Python!