The LLaMA C++ server is designed to streamline the process of serving large language models using C++ commands, allowing for efficient deployment and interaction with machine learning models.
Here's a simple code snippet demonstrating how to set up a basic LLaMA C++ server:
#include <iostream>
#include "llama_server.h"
int main() {
LlamaServer server("0.0.0.0", 8080);
server.start();
std::cout << "LLaMA Server is running on port 8080..." << std::endl;
return 0;
}
Understanding llama.cpp Server
What is Llama?
Llama, within the context of programming, refers to a powerful set of abstractions and functionality that allows developers to create efficient and concise server applications using C++. It is built with modern programming paradigms in mind, offering features that facilitate quick development and maintainability. The llama.cpp server leverages these concepts to provide a stalwart solution for web servers.
Exploring the Role of C++
C++ is renowned for its efficiency and flexibility, making it an excellent choice for server applications. Originally created in the early 1980s, the language has evolved significantly, incorporating features that support both high-level abstractions and low-level memory manipulation. This duality empowers developers to create robust server applications that can perform under high loads and scale with ease.
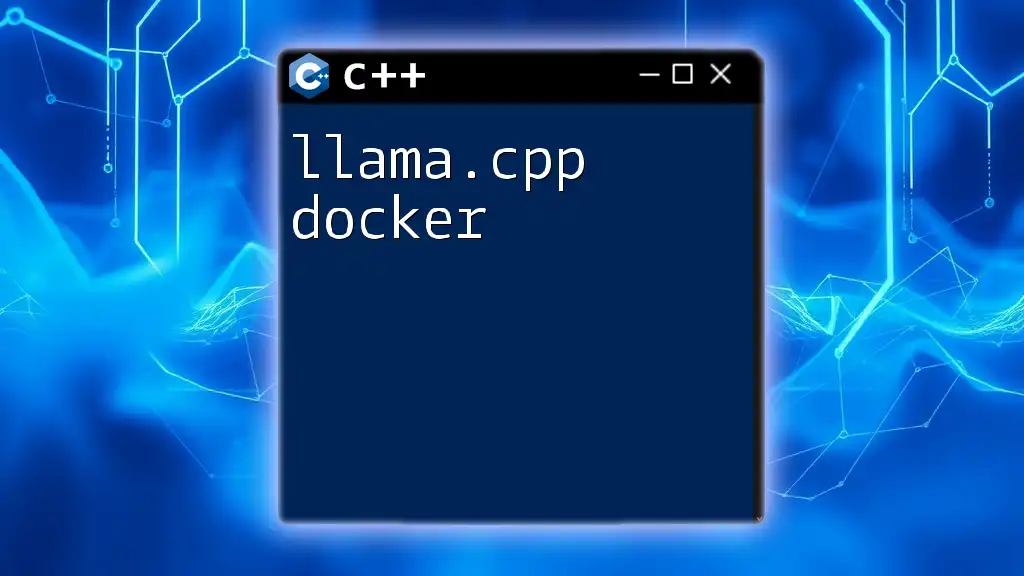
Setting Up Your Development Environment
Prerequisites
Before you can start building a llama.cpp server, you need to set up your development environment. This includes ensuring you have a C++ compiler installed, such as GCC or Clang, as well as a development environment, which could range from a full-fledged IDE like Visual Studio to a lightweight editor such as Visual Studio Code.
Installing llama.cpp
To utilize llama.cpp, follow these steps:
-
Download the llama.cpp library from its official repository on GitHub. It usually comes in a `.zip` or as a cloneable Git repository.
-
Build llama.cpp from source by following the installation instructions provided in the repository's README file. This often involves using CMake or Makefiles, depending on the project setup.
-
Be prepared to troubleshoot common installation issues, such as library path problems or missing dependencies. FAQs or community discussions often address these problems.
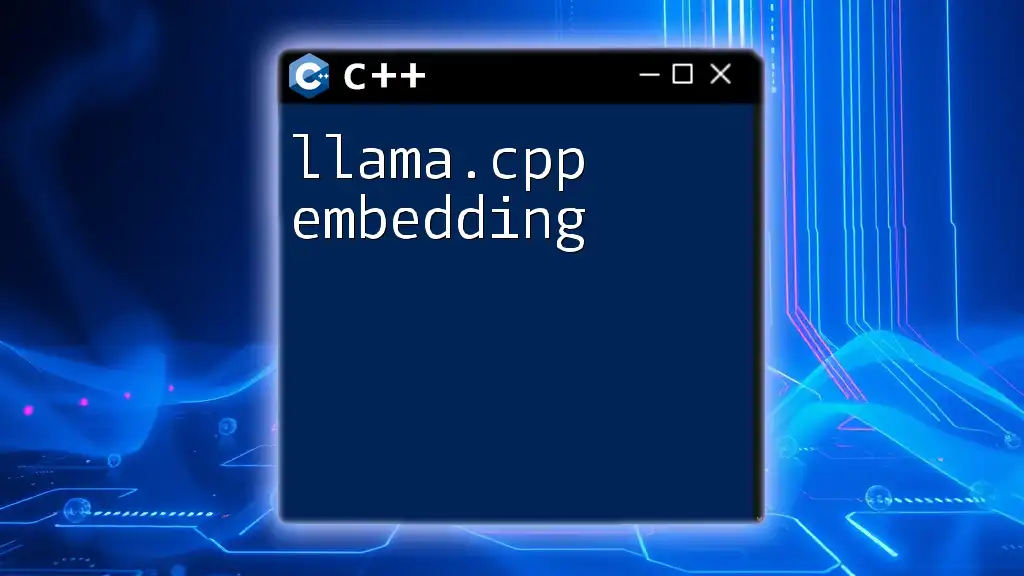
Structuring Your llama.cpp Server Application
Basic Framework of a llama.cpp Server
Understanding the architecture of a llama.cpp server is crucial for effective development. A typical architecture involves defining routes, handling requests, and returning responses.
Example: Your First llama.cpp Server
Creating your first server is straightforward and will provide you with hands-on experience.
#include <iostream>
#include "llama.h"
int main() {
Llama::Server server(8080);
server.handle("/hello", [](const Llama::Request& req, Llama::Response& res) {
res.set_content("Hello, Llama!", "text/plain");
});
server.start();
return 0;
}
In this example, we first include the necessary headers, where `llama.h` is the core header for llama functionalities. The server is initialized to listen on port `8080`. The route `/hello` is defined to respond with "Hello, Llama!" in plain text format. Finally, the `server.start();` call initializes the listening loop to process incoming requests.
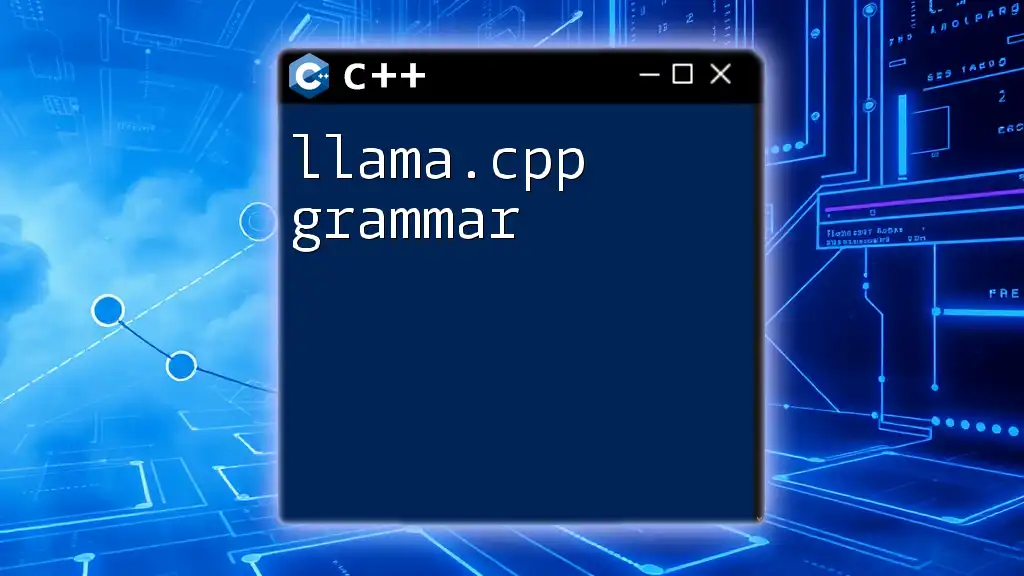
Key Features of llama.cpp Server
Performance Efficiency
One of the primary advantages of using llama.cpp is its performance efficiency. The library has been designed with minimal overhead, ensuring rapid request handling and low latency. The lightweight nature of llama means that you can handle tens of thousands of simultaneous connections without significant performance degradation.
Scalability
Building scalable applications is essential, especially for projects expecting rapid growth or those supporting a large user base. The llama.cpp server is structured to easily accommodate horizontal scaling, allowing for multiple instances to run across different servers or even in cloud environments. It's beneficial to design your server to be stateless wherever possible, optimizing resource utilization and simplifying scaling operations.
Error Handling
Handling errors effectively is crucial for any server application. The llama.cpp server provides built-in mechanisms to respond to unexpected conditions gracefully. Here's how to implement robust error responses:
server.handle("/error", [](const Llama::Request& req, Llama::Response& res) {
res.set_status(404);
res.set_content("Error: Resource not found", "text/plain");
});
This example sets a route for error handling if a requested resource is not found. By setting the status to `404` and providing an informative message, the server communicates clearly with clients about the issue.
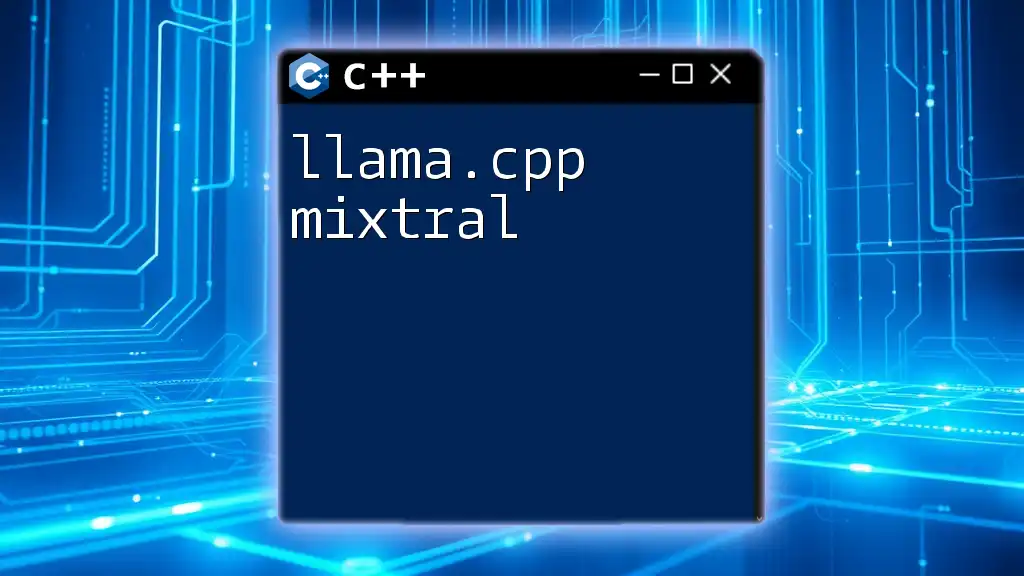
Advanced Topics in llama.cpp Server Development
Middleware Implementation
Middleware is a powerful concept that allows for the addition of processing requirements at various stages of request handling in a llama.cpp server. For instance, you might want to implement Cross-Origin Resource Sharing (CORS) middleware to manage resource access across different origins.
Here’s a simple way to create a CORS middleware:
void cors_middleware(const Llama::Request& req, Llama::Response& res) {
res.add_header("Access-Control-Allow-Origin", "*");
}
server.use(cors_middleware);
Working with Databases
Integrating databases into your llama.cpp server can enhance its capabilities significantly. If you decide to use SQLite, for example, you would typically do so by installing the SQLite library and linking it to your project. Here's a brief illustration of how to connect to SQLite:
#include <sqlite3.h>
// Rest of your server setup code
sqlite3* db;
int exit = sqlite3_open("database.db", &db);
if (exit) {
std::cerr << "Error open DB: " << sqlite3_errmsg(db) << std::endl;
}
Utilizing Asynchronous Processing
Asynchronous processing is essential for efficient server operations. It allows your application to handle multiple requests concurrently without blocking the main thread. You can leverage asynchronous routes and handlers within llama:
server.handle_async("/async", [](const Llama::Request& req, Llama::Response& res) {
// Perform time-consuming operations, like database queries or external API calls
res.set_content("Asynchronous operation completed!", "text/plain");
});
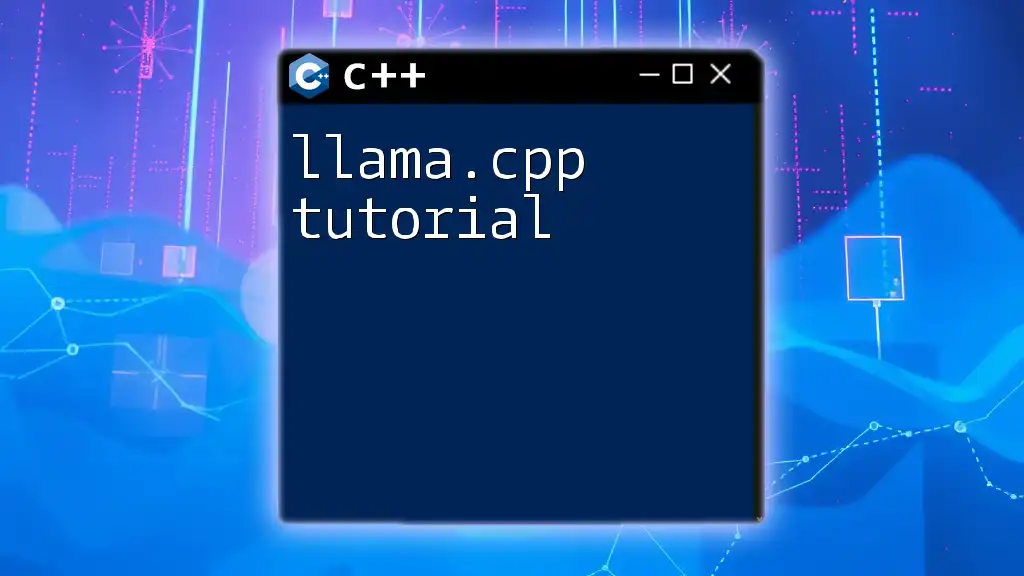
Testing and Debugging Your llama.cpp Server
Writing Tests for Your Server
Quality assurance can be achieved by writing tests for your llama.cpp server applications. Utilizing a unit testing framework allows you to ensure your routes and handlers respond as expected. Implement tests similar to the following:
#include <assert.h>
void test_hello_route() {
Llama::Request req;
Llama::Response res;
server.handle("/hello", req, res);
assert(res.get_content() == "Hello, Llama!");
}
Debugging Techniques
When issues arise, having robust debugging techniques at your disposal is crucial. Take advantage of debugging tools built into your IDE, such as breakpoints and variable watches, to trace through your methods and identify problems efficiently.
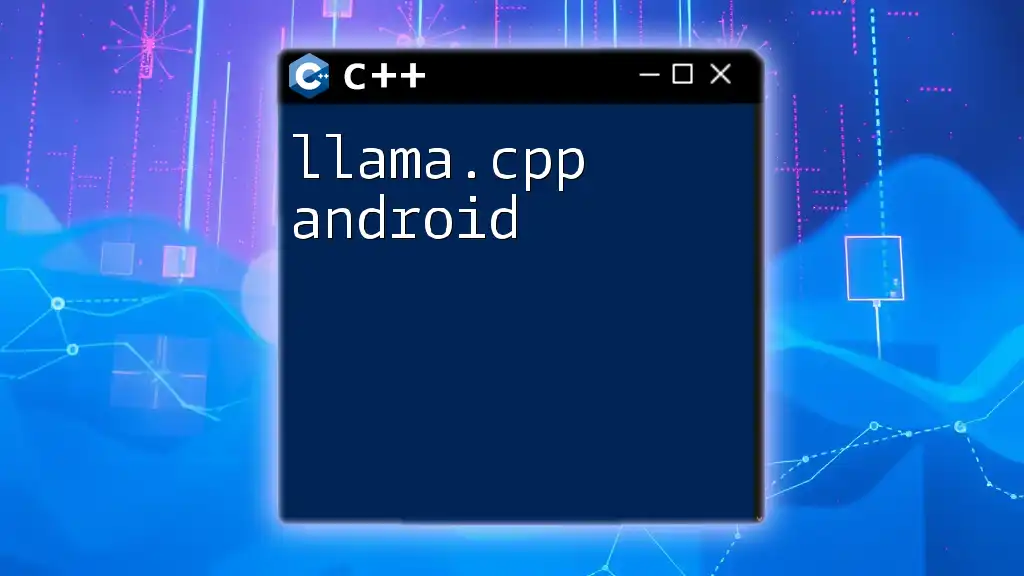
Deployment of llama.cpp Server Applications
Preparing for Production
Preparing a server for production involves meticulous attention to configuration and optimization. Key areas include:
-
Configuration: Ensure all settings align with production needs, such as database credentials and environment variables.
-
Security: Implement security best practices, including validating user input and ensuring the server is resilient to attacks.
Hosting Options
When it comes time to deploy, you'll have a variety of hosting options available, from cloud services like AWS or DigitalOcean to on-premise solutions. The deployment process varies but usually involves provisioning resources, deploying your server application, and configuring firewalls or proxies for securing and managing network traffic.
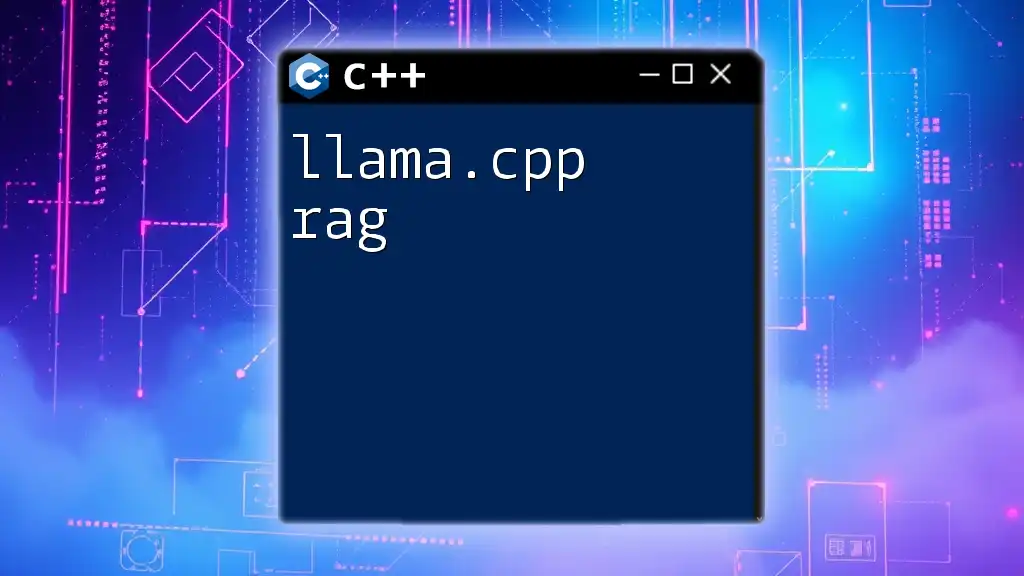
Conclusion
The journey to mastering llama.cpp server applications is filled with opportunities to explore modern C++ features and techniques. By understanding the foundational concepts, setting up your environment, and applying advanced features, you can build efficient, scalable, and resilient server applications.
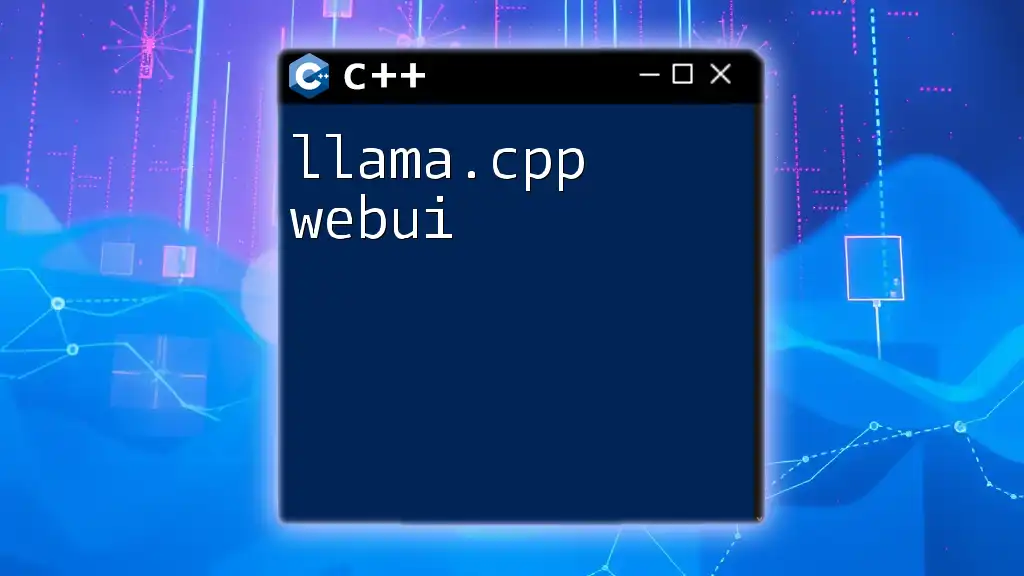
Call to Action
Take your first steps toward mastering llama.cpp by experimenting with the examples provided. If you're interested in enhancing your skills further, consider signing up for courses or tutorials that dive deeper into C++ server development. Don't hesitate to share your projects or questions in the comments – the community is here to support you on your journey!