Node-llama-cpp is a Node.js wrapper that allows developers to leverage LLaMA model functionalities with C++ efficiency for fast and effective AI applications.
Here’s a simple code snippet demonstrating how to use node-llama-cpp to initialize a LLaMA model:
#include <llama.h>
int main() {
LLaMA model("path/to/model");
model.load();
model.generate("Hello, World!");
return 0;
}
What Is Node-Llama-CPP?
Node-Llama-CPP is a powerful integration framework that enables developers to harness the speed and efficiency of C++ while leveraging the asynchronous capabilities of Node.js. This fusion allows developers to write performance-critical applications where high computational tasks can be offloaded to C++, while still enjoying the event-driven architecture that Node.js provides. By bridging the gap between these two programming environments, Node-Llama-CPP streamlines workflow and enhances application responsiveness.
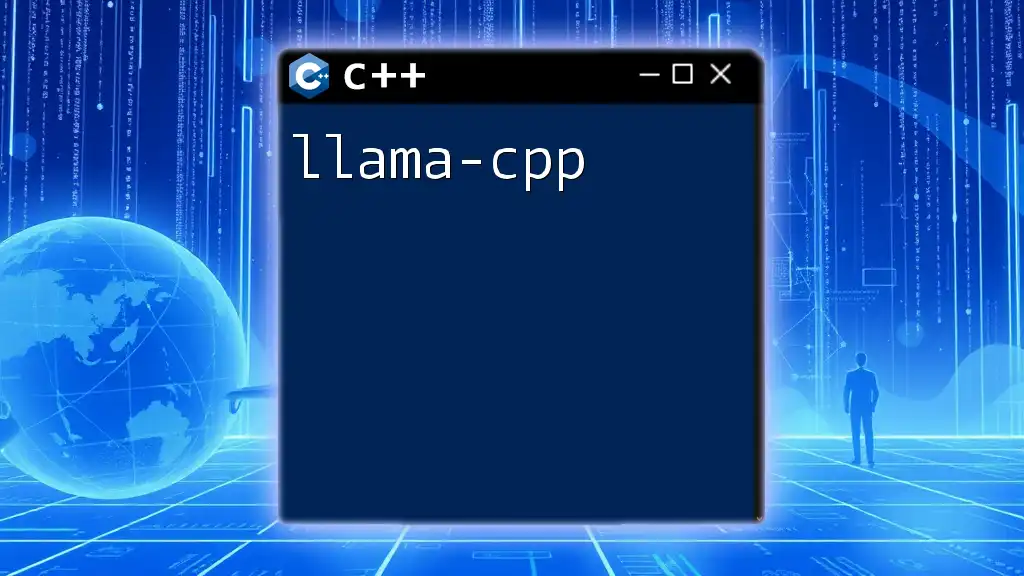
Why Use Node-Llama-CPP?
Using Node-Llama-CPP comes with numerous advantages. It allows developers to write performance-sensitive code in C++, while retaining the simplicity and flexibility of Node.js for handling I/O operations. This is particularly beneficial for applications that require heavy computation, such as data processing, image manipulation, or real-time analytics.
Real-world applications of Node-Llama-CPP range from game development, where performance and speed are paramount, to web applications that process large datasets in real time. By integrating C++ code into a Node.js environment, developers achieve a more efficient application architecture that is not only faster but also more resource-friendly.
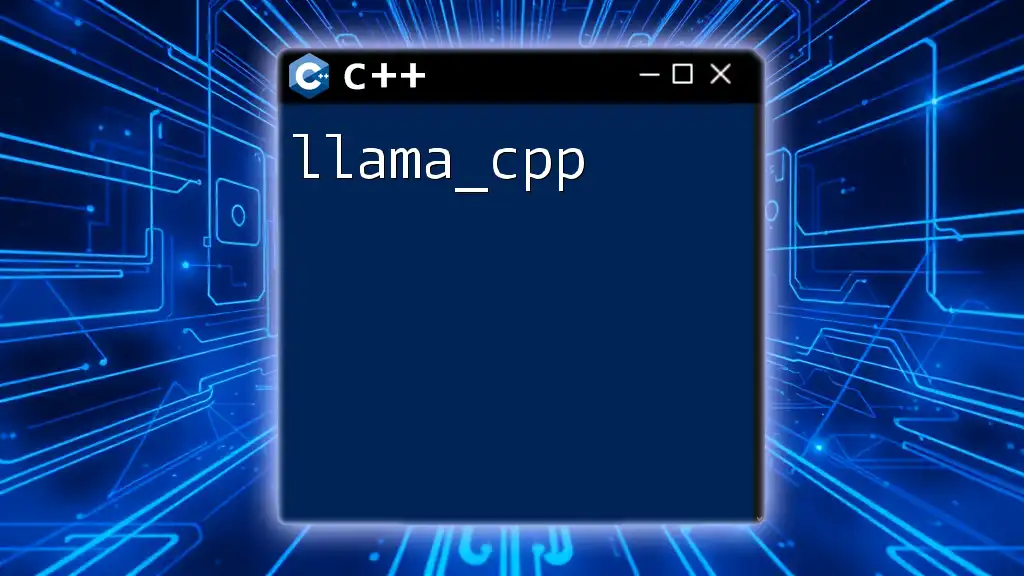
Setting Up Node-Llama-CPP
Prerequisites
Before diving into Node-Llama-CPP, ensure you have the following software installed:
- Node.js: Required for running JavaScript code on the server.
- C++ Compiler: Necessary for compiling your C++ code (such as GCC for Linux, Xcode for macOS, or MSVC for Windows).
Recommended IDEs for this setup include Visual Studio Code, JetBrains CLion, or any code editor that supports both C++ and JavaScript development.
Installation Steps
Installing Node.js
Follow these steps based on your operating system:
- Windows: Download the installer from the official Node.js website and run it.
- macOS: Use Homebrew by running `brew install node` in the terminal.
- Linux: Use your package manager, e.g., `sudo apt install nodejs` for Debian-based systems.
Installing C++ Compiler
- Windows: Install Visual Studio and select the C++ development tools during installation.
- macOS: Install Xcode from the App Store and its command line tools.
- Linux: Install GCC using `sudo apt install g++` for Debian-based systems.
Installing Node-Llama-CPP
To install Node-Llama-CPP, simply run the following command in your terminal:
npm install node-llama-cpp
This command will fetch the package and add it to your `node_modules` directory, making it ready for use in your applications.
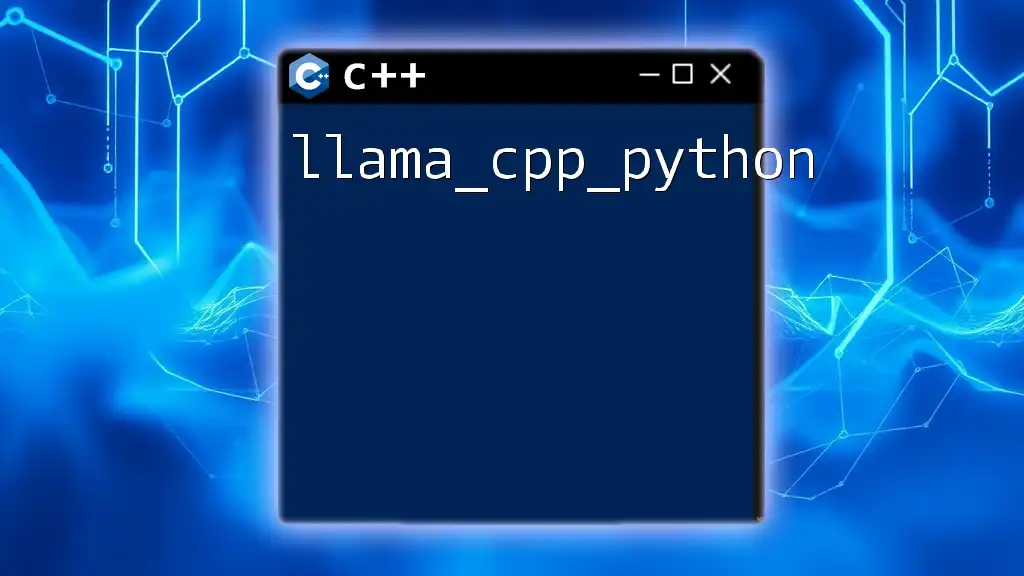
Understanding the Basics of Node-Llama-CPP
Core Concepts of Node-Llama-CPP
Node-Llama-CPP primarily revolves around binding C++ code to JavaScript. This integration allows JavaScript to leverage C++ functionality, resulting in faster execution for resource-intensive tasks. Understanding the operational framework of Node-Llama-CPP is critical for effective utilization.
Getting Started with Basic Commands
Creating Your First Node-Llama-CPP Project
To start a new project, create a folder for your application, navigate into it, and initialize a new Node.js project:
mkdir my-llama-project
cd my-llama-project
npm init -y
This will create a `package.json` file necessary for managing your project dependencies.
Writing Your First C++ Command
Once your environment is set, you can create and compile your first C++ command. Below is an example of a simple C++ function that returns a string:
#include <n-api.h>
Napi::String HelloWorld(const Napi::CallbackInfo& info) {
Napi::Env env = info.Env();
return Napi::String::New(env, "Hello, World!");
}
Explanation: This code snippet includes the necessary N-API header to interface with Node.js. The function `HelloWorld` takes no parameters and returns a string. The interaction with Node.js is facilitated through the concept of an environment, which is passed to the function.
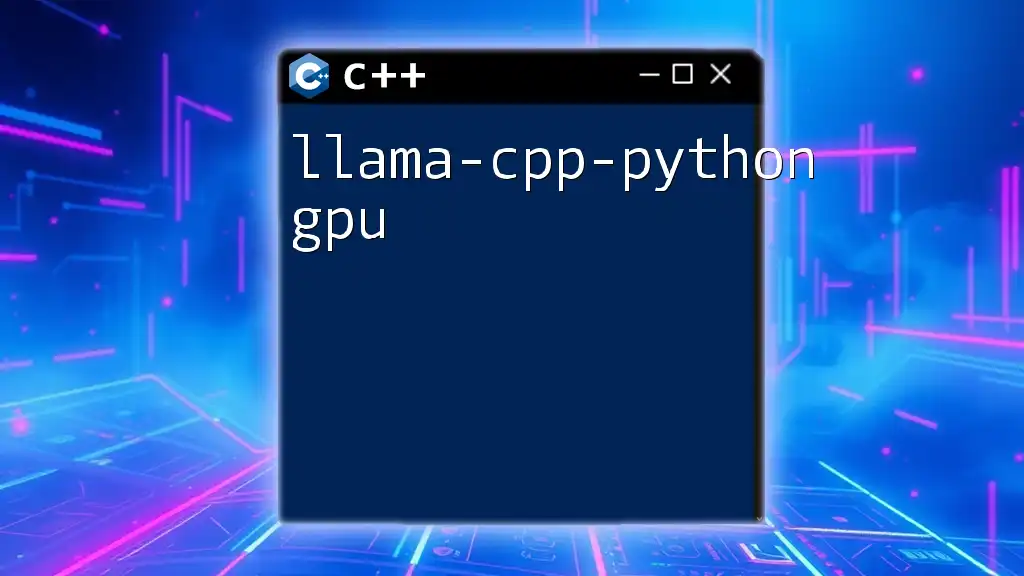
Advanced Features of Node-Llama-CPP
Working with C++ Classes and Objects
Defining complex data structures can drastically simplify your code. By exposing C++ classes to JavaScript, you gain greater control over the application logic. Here is how you can define a simple C++ class:
class MyClass {
public:
MyClass() {}
Napi::String GetMessage(const Napi::CallbackInfo& info) {
return Napi::String::New(info.Env(), "Hello from C++!");
}
};
You can then wrap this class in a N-API function to make it available in your Node.js code.
Error Handling in Node-Llama-CPP
Robust applications need excellent error handling mechanisms. In C++, errors can arise due to various reasons, including memory access violations. Below is an example of effective error handling:
if (someErrorCondition) {
Napi::TypeError::New(env, "An error occurred").ThrowAsJavaScriptException();
}
This code demonstrates how to throw a JavaScript exception from C++ when a specific condition is met, ensuring that the JavaScript layer can react accordingly.
Asynchronous Programming with Node-Llama-CPP
Node.js thrives on asynchronous programming, and Node-Llama-CPP allows you to maintain this paradigm in C++. Below is an example of creating an asynchronous function:
Napi::Promise MyAsyncFunction(const Napi::CallbackInfo& info) {
// Implementation here
}
Implementing asynchronous behavior involves scaffolding with JavaScript promises, allowing you to seamlessly integrate C++ heavy-lifting tasks into your Node.js application without blocking the event loop.
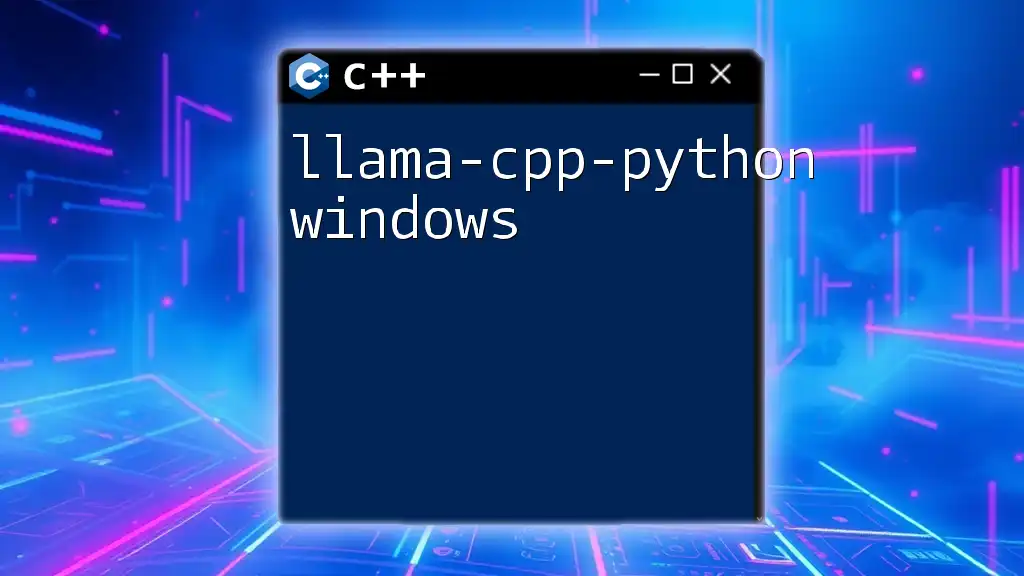
Practical Applications
Creating a Simple Web Server with Node-Llama-CPP
You can kickstart a web server using Node-Llama-CPP by initializing an HTTP server and employing C++ to process requests efficiently. This allows you to handle large datasets or perform complex calculations without compromising performance.
Building a Data Processing Tool
Leveraging the computational capabilities of C++, you can construct a data processing tool that interfaces with Node.js to handle I/O efficiently. This setup enables you to process large volumes of data rapidly, making your application both powerful and responsive.
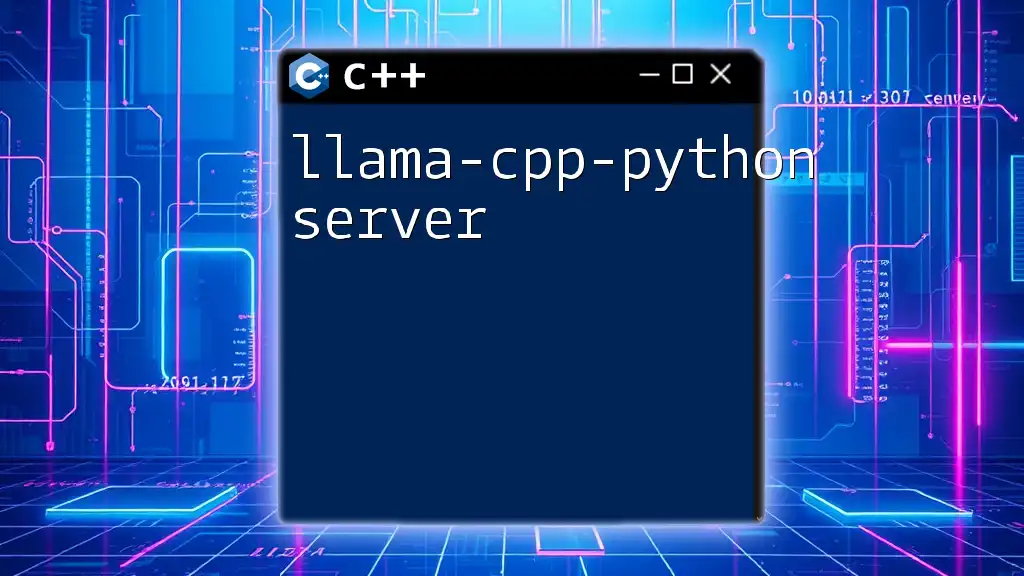
Testing and Debugging in Node-Llama-CPP
Best Practices for Testing C++ Code
Maintaining code quality is fundamental in development. Utilize frameworks like Google Test for writing unit tests in your C++ portions of Node-Llama-CPP. Unit tests ensure that your code behaves as expected and helps detect issues early.
Debugging Techniques
Common debugging strategies such as logging, breakpoints, and assertions are crucial for diagnosing issues within your C++ code. Tools like `gdb` for Linux or the built-in debugger in Visual Studio can help trace problems effectively.
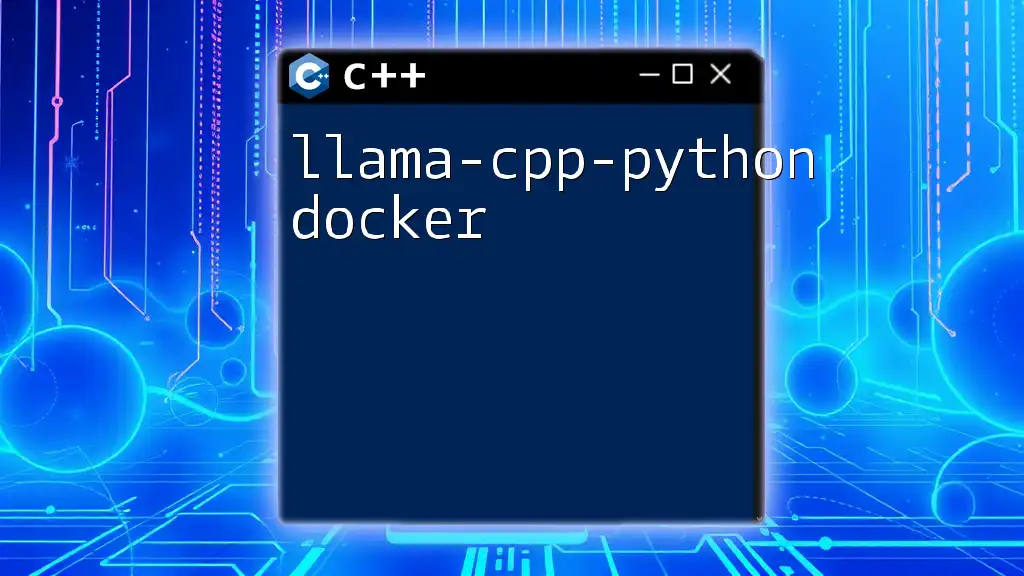
Performance Optimization Tips
Profiling Your Application
Use profiling tools to identify bottlenecks in both JavaScript and C++ portions of your application. Tools like `node --inspect` or `perf` can help collect detailed performance metrics, guiding your optimization efforts.
Optimizing C++ Code
Enhancing the efficiency of your C++ code is paramount. Some techniques include:
- Utilizing memory management effectively to reduce overheads.
- Preferring algorithmic efficiency—opt for O(n log n) algorithms when dealing with huge datasets.
- Employing inlining and template metaprogramming where applicable to improve execution speed.
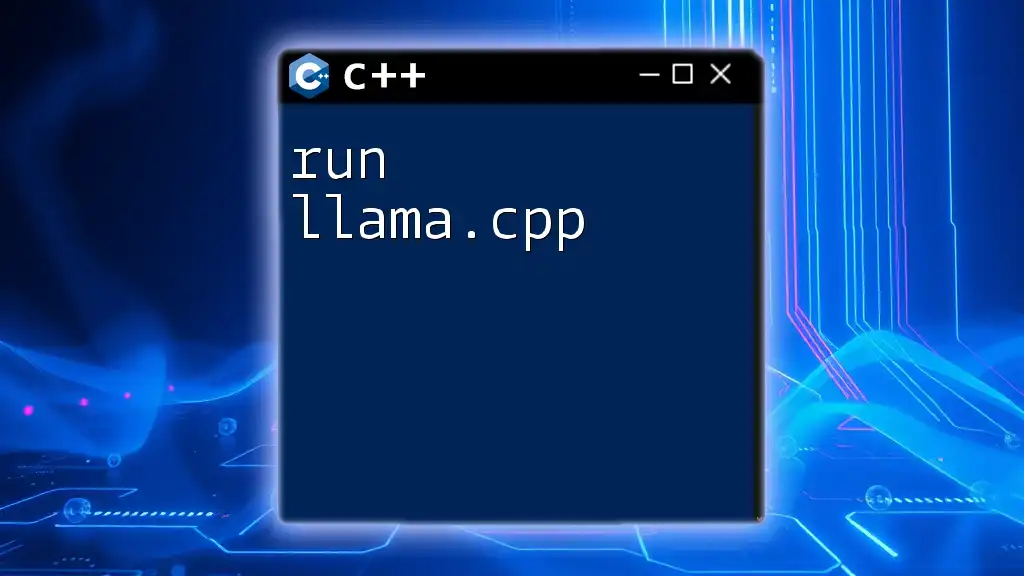
Conclusion
Node-Llama-CPP serves as a powerful tool in the modern development landscape, combining the best of both C++ performance and Node.js flexibility. By understanding the framework's core concepts and applications, you can develop applications that are not only efficient but also maintainable and scalable. As you experiment with this integration, you'll discover innovative solutions to performance challenges, ultimately enhancing the user experience of your applications.
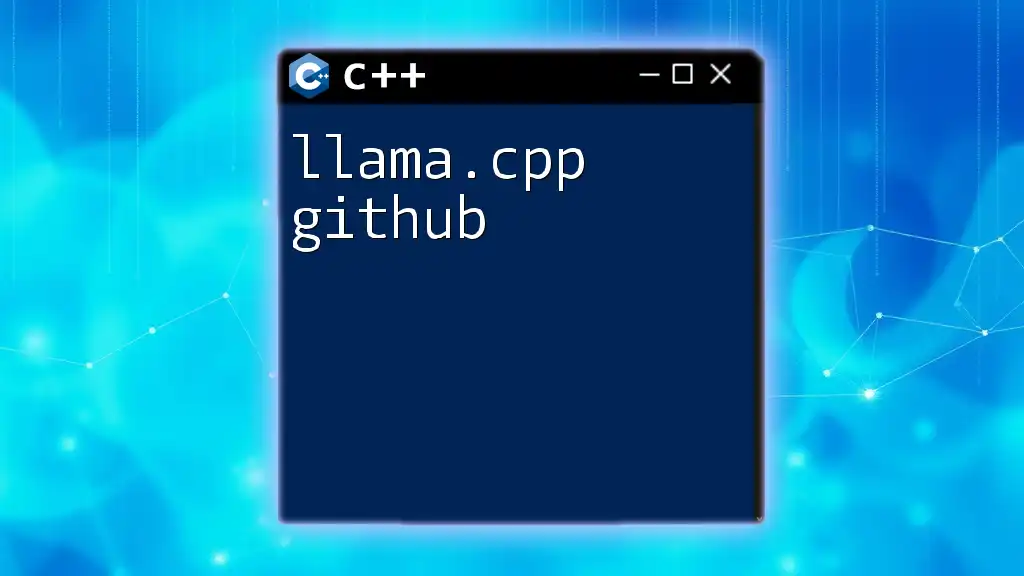
Additional Resources
For ongoing support and additional learning, refer to the official Node-Llama-CPP documentation, browse GitHub repositories related to your projects, and engage with community forums where you can ask questions and share insights. With these resources, you can continue to deepen your understanding of this remarkable integration framework.