To run `llama.cpp`, you need to compile the code and execute the generated binary, like this:
g++ llama.cpp -o llama && ./llama
What is Llama.cpp?
Llama.cpp is a C++ file designed to perform specific functions within the realm of programming. It encapsulates a variety of operations that can enhance applications or serve particular use cases. The primary purpose of `llama.cpp` is to streamline tasks within your C++ projects, making programming not only easier but also more efficient.
Key Features
- Modularity: Through its specific functions, `llama.cpp` promotes code reuse and better organization by separating concerns effectively.
- Performance: Optimized for speed and efficiency, it leverages C++'s strengths to execute tasks swiftly.
- Flexibility: It integrates seamlessly with other libraries and frameworks, allowing for broader application in various contexts.
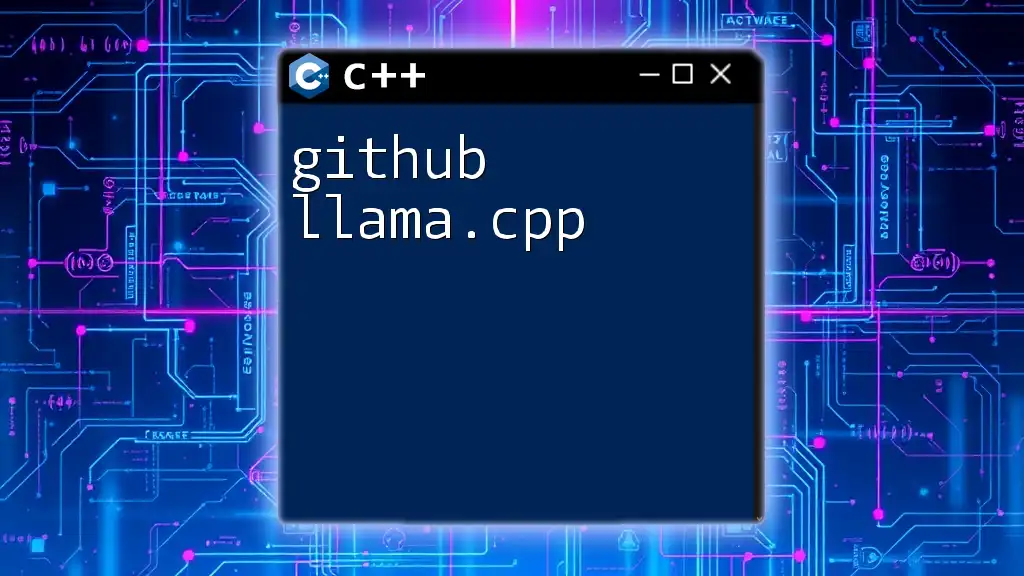
Setting Up Your Environment
To run llama.cpp, you first need to ensure that your development environment is ready. That means installing the necessary tools and following specific setup steps.
Required Tools
Before diving into coding, make sure you have the right tools in your toolkit:
- C++ Compiler: You can choose from several options. Two popular ones include GCC and Clang for Linux/Mac, and Visual Studio for Windows.
Installation Steps
Installing the necessary software is straightforward:
- For Linux users: You can install GCC using the following command in your terminal:
sudo apt-get install g++
- For Windows users: Install Visual Studio with C++ development tools enabled. It's user-friendly and provides a great IDE for beginners.
- For Mac users: You can install Xcode Command Line Tools by running:
xcode-select --install
Once your environment is set up, you're ready to run `llama.cpp`.
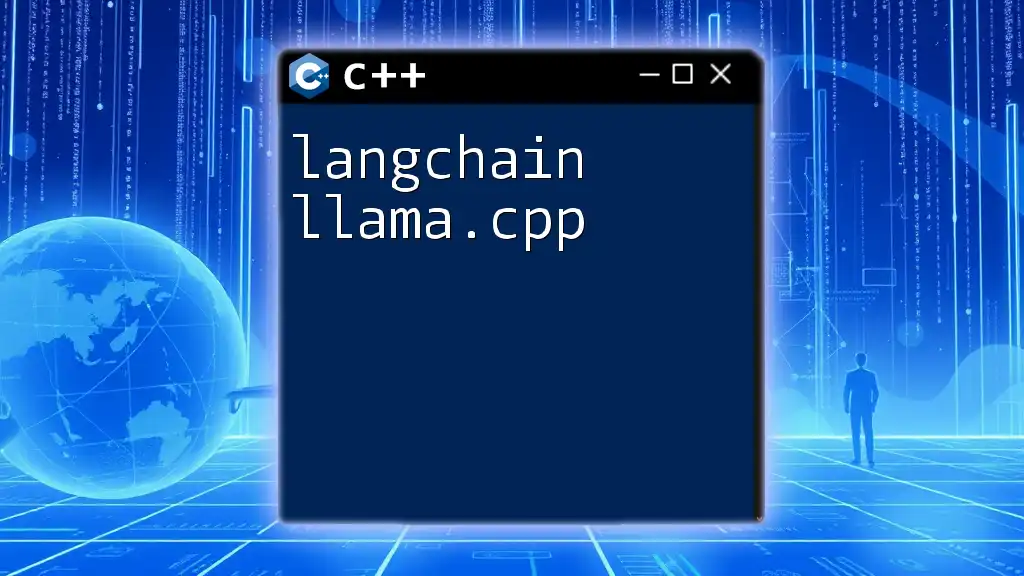
Running Llama.cpp
The next step is understanding how to execute `llama.cpp`. This involves compiling the code and running the compiled program.
Basic Execution Flow
A C++ program generally follows a specific structure. To execute `llama.cpp`, you start by compiling it into an executable file.
Compiling Llama.cpp
To compile `llama.cpp`, you can use the `g++` command. This key command transforms your C++ source code into a runnable executable.
- Basic Compilation Command
To compile the program, execute:
g++ llama.cpp -o llama
The `-o` option specifies the output file's name, which in this case is `llama`.
- Debugging Options
If you want to include debugging information, you can compile it with the `-g` flag:
g++ -g llama.cpp -o llama
Executing the Compiled Program
After compiling, the next step is to run the program. Executing the compiled file is simple and can be done using the following command:
./llama
This command tells your operating system to run the `llama` executable you just created.
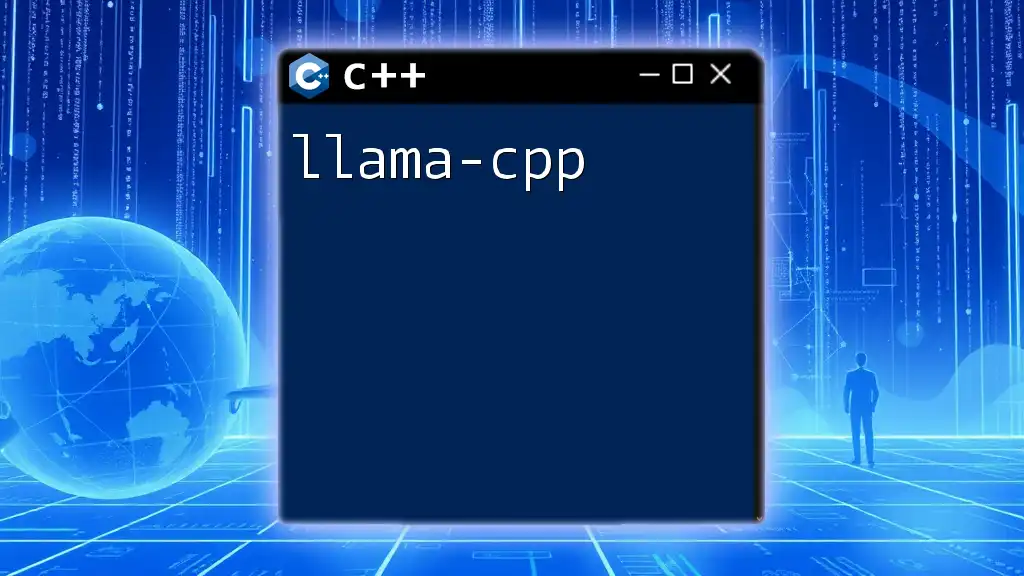
Code Snippets in Llama.cpp
Understanding the functions within `llama.cpp` is crucial for leveraging its capabilities.
Understanding Key Functions
Let's explore some significant functions commonly found in `llama.cpp`:
- Function Definition: Here’s a basic function that prints a message to the console:
void sayHello() {
std::cout << "Hello, Llama!" << std::endl;
}
- Main Function: Here’s how you can employ the `sayHello` function in the main program loop:
int main() {
sayHello();
return 0;
}
In this example, the `sayHello` function is called within the `main` function, showcasing a simple task execution.
Example Code Walkthrough
The previous snippets highlight the typical structure of a C++ program. The use of `std::cout` for console output and `return 0;` indicates successful execution are standard practices. These core components are essential in grasping how to effectively run llama.cpp.
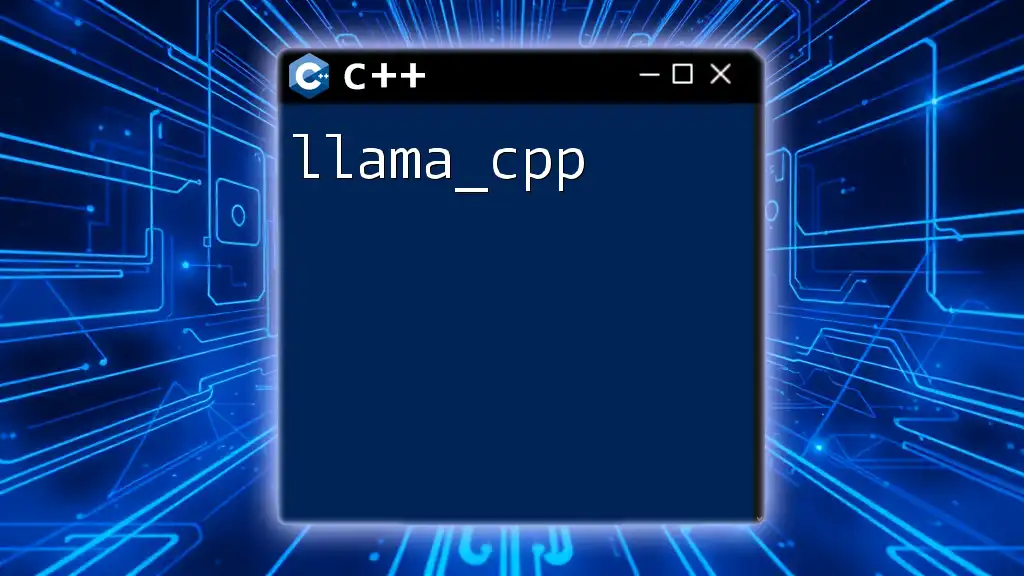
Common Errors and Troubleshooting
Even experienced programmers encounter errors. It's vital to know common pitfalls when working with C++.
Compilation Errors
Compilation errors often stem from syntax mistakes or undeclared variables. Examples include:
-
Undefined Reference Errors: This occurs when the compiler can't find a function's definition. The solution is to ensure all functions are properly declared and defined.
-
Syntax Errors: These commonly arise from missing semi-colons or mismatched braces. Carefully cross-checking your syntax can often resolve these issues.
Runtime Errors
When you run the program, you might encounter runtime errors, such as:
-
Segmentation Faults: These occur when your program attempts to access invalid memory. Always verify array bounds and pointer dereferences.
-
Logical Errors: Your code runs without producing an error, but the output is incorrect. Debugging tools like GDB can be helpful here for stepping through your code.
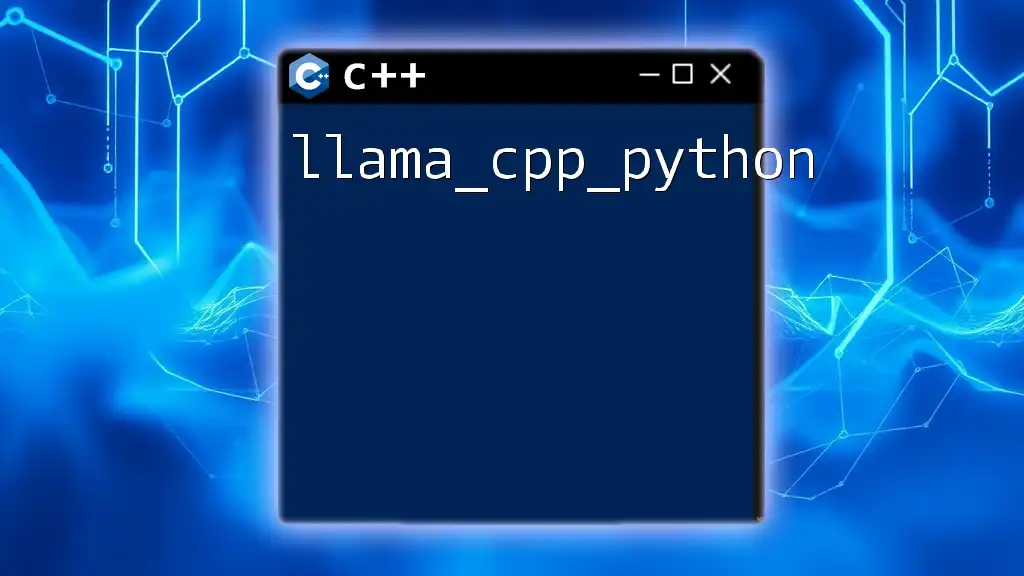
Advanced Tips and Tricks
To maximize the power of `llama.cpp`, consider implementing some advanced strategies.
Optimizing Llama.cpp
To ensure that your program runs efficiently, you may take the following steps:
-
Use of Constants: Replace magic numbers with named constants. This enhances readability and maintainability.
-
Compiler Optimizations: You can enable optimizations during compilation by adding flags like `-O2`:
g++ -O2 llama.cpp -o llama
Integrating with Other Libraries
You can enhance `llama.cpp` by integrating it with popular libraries, such as Boost or SDL. This can broaden its capabilities significantly.
To link against a library, use the `-l` option followed by the library name in your compile command, like so:
g++ llama.cpp -o llama -lboost_system
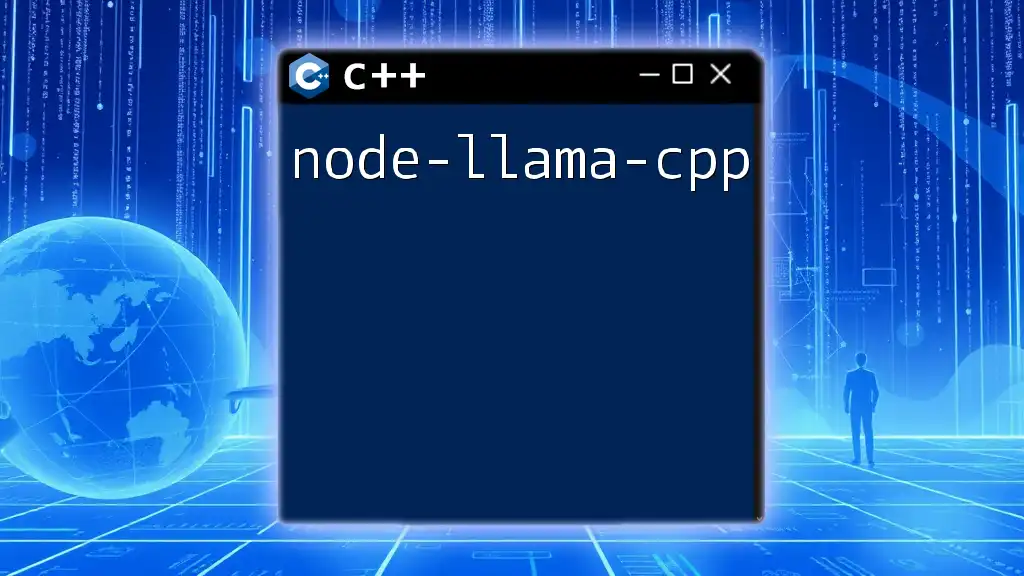
Conclusion
Understanding how to run llama.cpp is a gateway into the world of C++. With proper setup, coding practices, and an awareness of troubleshooting, you can leverage this powerful programming language effectively.
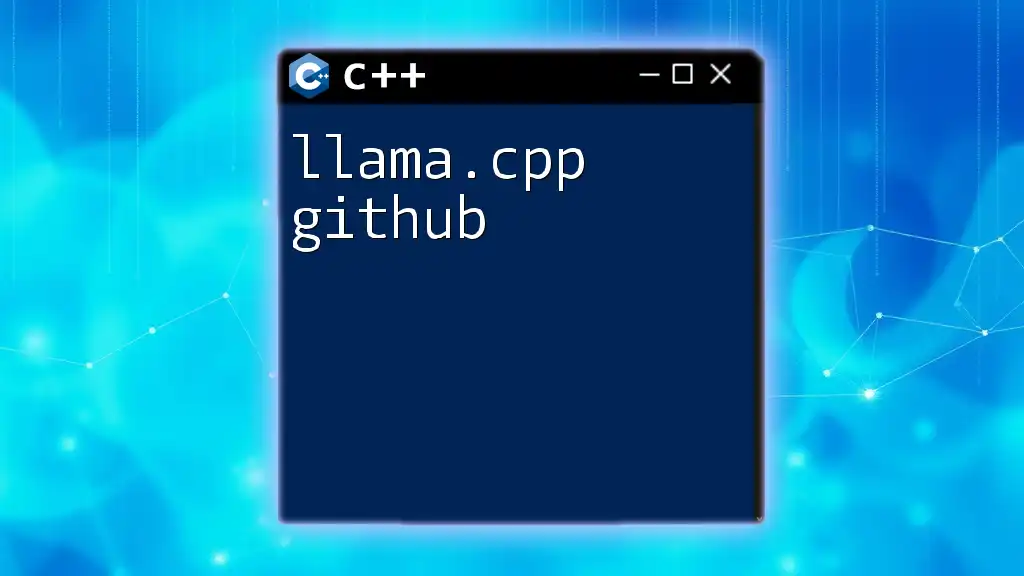
Call to Action
Feel free to reach out for more tips on executing C++ commands. Stay tuned for future articles that will further enrich your programming journey!
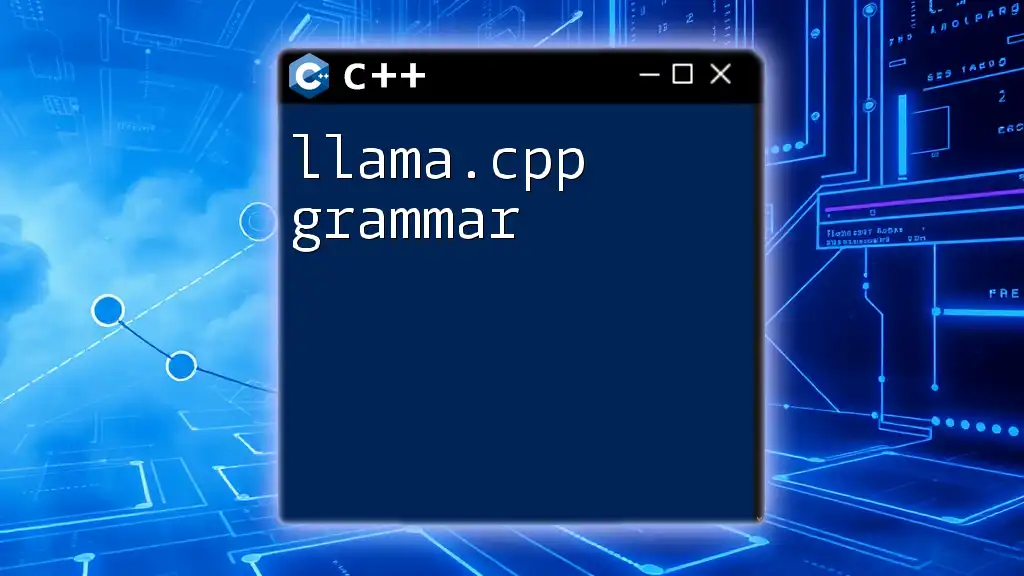
Additional Resources
For further reading, check out other related articles and tutorials that delve into C++ programming, along with recommended books and online courses that can enhance your coding proficiency.