The `llama-cpp-python` library enables users to leverage GPU capabilities when executing LLaMA models with minimal setup in Python.
Here's a code snippet demonstrating how to use the `llama-cpp-python` library with GPU support:
# First, ensure you have llama-cpp-python installed with GPU support
# Then, use the following code to load a model and perform inference
from llama_cpp import Llama
# Load the model with GPU support
llm = Llama(model="/path/to/your/model", use_gpu=True)
# Generate text based on a prompt
response = llm("Once upon a time")
print(response)
What is Llama-cpp-python?
Llama-cpp-python is a powerful package that seamlessly integrates the capabilities of C++ directly into Python. This combination allows developers to exploit the optimized performance of C++ while enjoying the simplicity and flexibility of Python.
Definition and Purpose
Llama-cpp-python serves as a bridge for those who want to harness C++’s speed and efficiency without diving deep into C++ programming. It is particularly beneficial for data-intensive applications where performance is critical.
Key Features
- Speed Optimization: By utilizing C++ under the hood, Llama-cpp-python enhances execution speed, making it faster than pure Python approaches.
- Flexibility with C++ Commands: You can directly call C++ functions and commands from Python, giving you access to a wide range of performance-sensitive algorithms and computational routines.
- Integration with Python Libraries: Llama-cpp-python works smoothly with popular Python libraries such as NumPy and TensorFlow, allowing for easy integration into existing workflows.
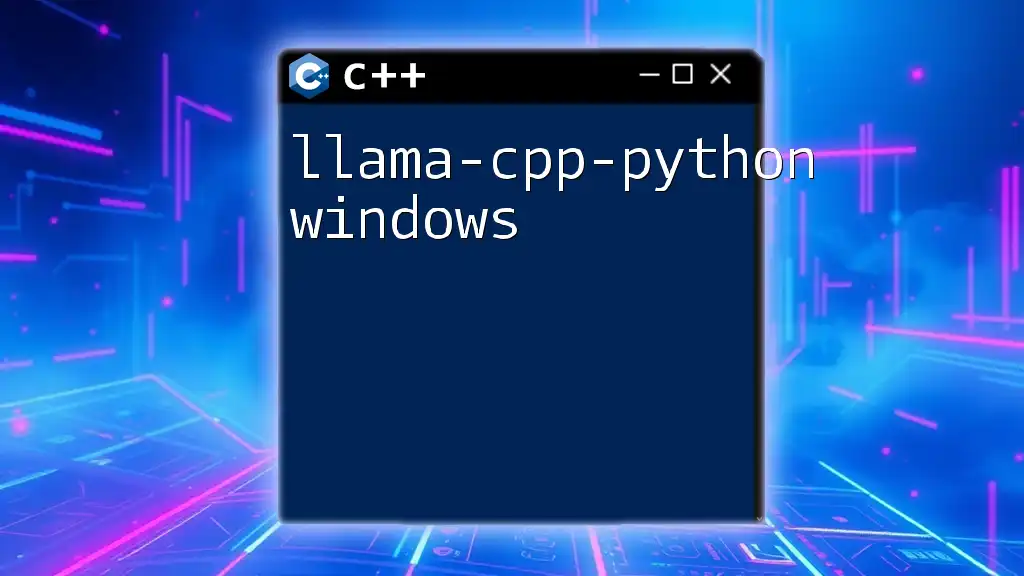
Understanding GPU Computing
What is GPU Computing?
GPU computing refers to the use of a graphics processing unit (GPU) to perform computations traditionally handled by the central processing unit (CPU). Whereas CPUs are designed for sequential task processing, GPUs excel in parallel processing, which is particularly beneficial for tasks involving large datasets.
Advantages of Using GPUs
Using GPUs comes with notable advantages:
- Increased Performance for Parallel Tasks: With thousands of cores operating simultaneously, GPUs can dramatically speed up processes that can be parallelized, such as matrix operations and deep learning training.
- Improved Energy Efficiency: GPUs can perform more calculations per watt compared to CPUs, making them an attractive choice for energy-conscious applications.
How GPUs Work
Basic Architecture of a GPU
A GPU's architecture is different from that of a CPU. It consists of a larger number of smaller cores designed for high-throughput computing. Each core is optimized for executing many threads simultaneously, which is essential for tasks like graphics rendering and scientific computations.
Common Applications of GPU Computing
GPU computing is not confined to gaming; it has expanded into various sectors such as:
- Scientific Simulations: Running complex simulations requiring vast computational resources.
- Machine Learning and AI: Training models faster with parallel computations.
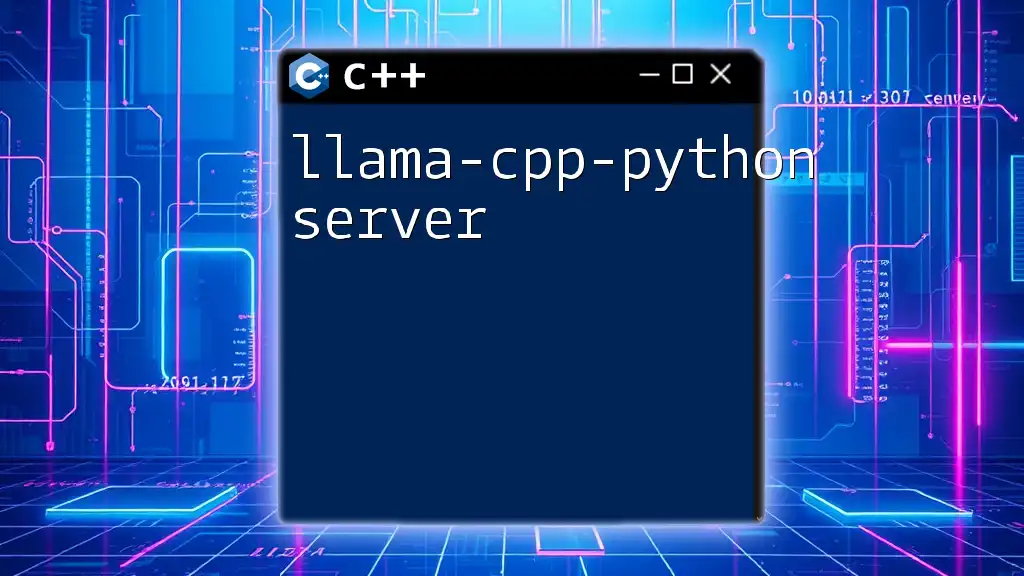
Setting Up Llama-cpp-python with GPU
System Requirements
Before beginning installation, you need to ensure that your system meets the following requirements:
- Hardware Requirements: A compatible GPU from NVIDIA is recommended due to CUDA's widespread support. Ensure the GPU is appropriate for your intended computational tasks.
- Software Prerequisites: Install the latest drivers for your GPU and ensure you have Python and pip on your system.
Installation Process
To install Llama-cpp-python, follow these steps:
-
Open your terminal.
-
Run the following command to install via pip:
pip install llama-cpp-python
After the installation completes, ensure that CUDA (Compute Unified Device Architecture) is also installed on your system for optimal GPU functionality.
Configuration for GPU
Configuring Llama-cpp-python for GPU Use
After installation, you must configure Llama-cpp-python to utilize the GPU effectively. You may need to modify specific configuration files found in the installation folder. For instance:
llama_config.json
In this file, specify the GPU settings, including memory allocation and device ID.
Testing the Setup
To verify that your setup is correctly configured for GPU usage, run a simple test script:
import llama_cpp
def test_gpu():
print("Testing GPU capabilities...")
# Example function from Llama that checks device
llama_cpp.check_gpu()
if __name__ == "__main__":
test_gpu()
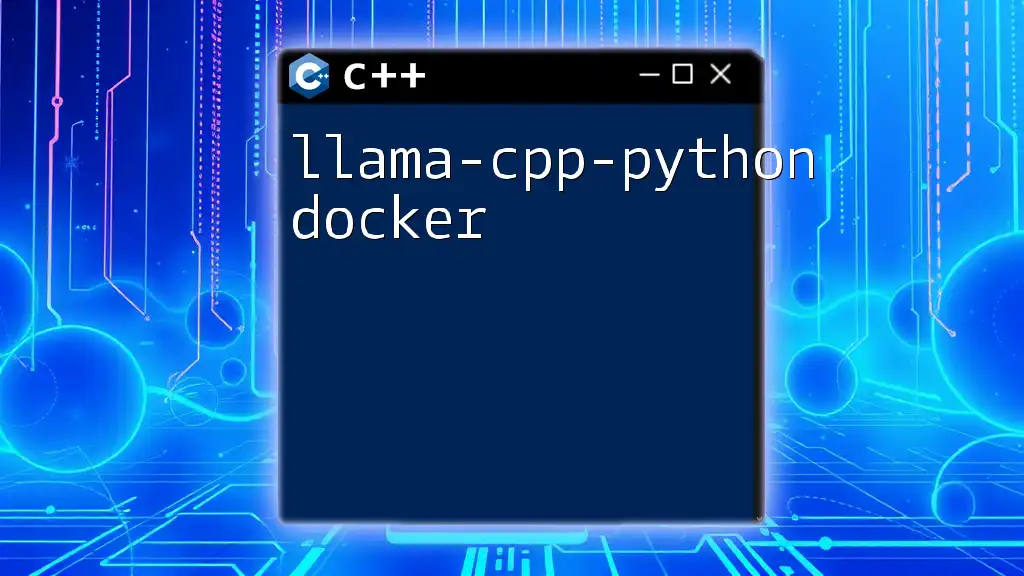
Using Llama-cpp-python with GPU
Basic Commands and Implementation
Llama-cpp-python provides an array of commands that allow access to GPU functionalities.
For example, with Llama’s `compute` function, you can perform a simple vector addition:
import llama_cpp
def vector_addition(vec_a, vec_b):
result = llama_cpp.compute(vec_a, vec_b)
return result
vec_a = [1, 2, 3]
vec_b = [4, 5, 6]
print("Result:", vector_addition(vec_a, vec_b))
Performance Comparisons
Benchmarking code performance showcases the immense benefits of leveraging a GPU. By conducting a comparison with and without GPU utilization, you can clearly observe the execution time differences. Here’s a conceptual example of measuring time:
import time
start_cpu = time.time()
# Run a CPU-bound operation here
end_cpu = time.time()
start_gpu = time.time()
# Run a GPU-optimized operation here
end_gpu = time.time()
print("CPU Time:", end_cpu - start_cpu)
print("GPU Time:", end_gpu - start_gpu)
Advanced Features
Optimizing Your Code for GPU Performance
To make the most out of your GPU, consider the following guidelines:
- Memory Management: Efficient management of GPU memory can prevent bottlenecks and improve speed.
- Batch Processing: Where possible, process data in batches to maximize parallel execution.
For those utilizing multiple GPUs, be aware of the pros and cons. While multiple GPUs can increase performance for large-scale tasks, managing synchronization and data transfer between GPUs can introduce additional complexity.
Handling Errors and Troubleshooting
Common issues that users might face when using Llama-cpp-python with GPU include installation problems, configuration settings, or runtime errors. Here are a few frequently encountered errors along with their solutions:
- CUDA Not Found: Ensure that CUDA is correctly installed and that environment variables are set.
- Insufficient memory: Monitor your memory usage and adjust your code or hardware resources accordingly.
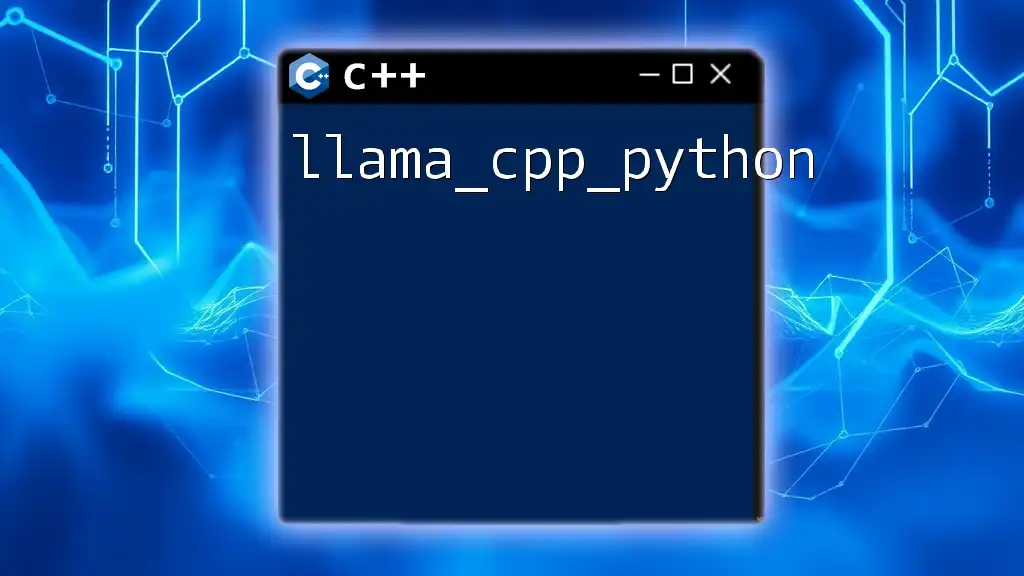
Real-World Applications
Case Studies Using Llama-cpp-python with GPU
Real-world applications of Llama-cpp-python combined with GPU power include:
- Machine Learning Model Training: Rapid prototyping of models with vastly improved training times can be achieved using Llama-cpp-python to access optimized libraries.
- Data Analysis and Visualization Projects: Handling large datasets and applying complex algorithms in real-time can be dramatically enhanced.
Community Contributions and Resources
Llama-cpp-python benefits from an active community. Many users share their projects, insights, and optimization techniques. Engaging with platforms like GitHub or community forums can provide valuable resources and allow you to tap into existing knowledge.
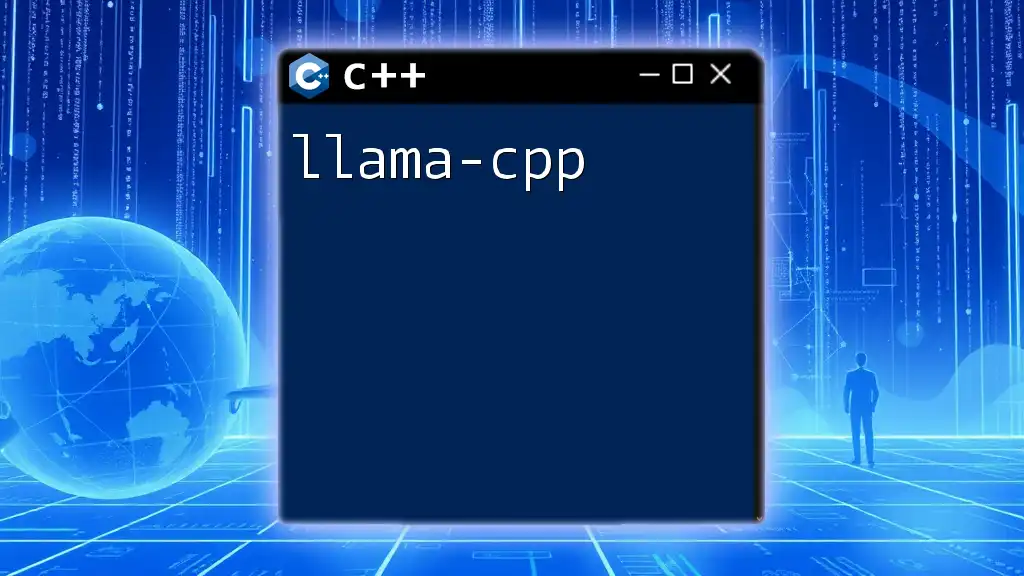
Conclusion
Llama-cpp-python, when coupled with GPU capabilities, opens up endless possibilities for rapid and efficient computation. The advantages of increased performance and flexibility in coding make it a compelling choice for developers. Embracing this powerful combination not only improves productivity but also enhances the potential for innovation in various applications.
By leveraging the shared knowledge, available resources, and community support, you can embark on an exciting journey into the world of GPU computing with Llama-cpp-python!