The "llama cpp cpu only" refers to the usage of the LLaMA model implemented in C++ that is designed to run exclusively on CPU without the need for GPU acceleration.
Here's a simple code snippet demonstrating how to load and use a LLaMA model with CPU support in C++:
#include <llama.h> // Hypothetical LLaMA library header
int main() {
LlamaModel model("path/to/model"); // Load LLaMA model from specified path
model.setDevice("cpu"); // Set the execution device to CPU
auto result = model.predict("Your input text here"); // Make predictions
std::cout << result << std::endl; // Output the results
return 0;
}
What is Llama CPP?
Llama CPP is an innovative framework designed for developing and deploying models efficiently. It caters primarily to the needs of AI practitioners by providing a robust platform for integrating machine learning models. The CPU-only version of Llama CPP enables users to run models without requiring dedicated GPU hardware, making it accessible for developers working on less powerful systems.
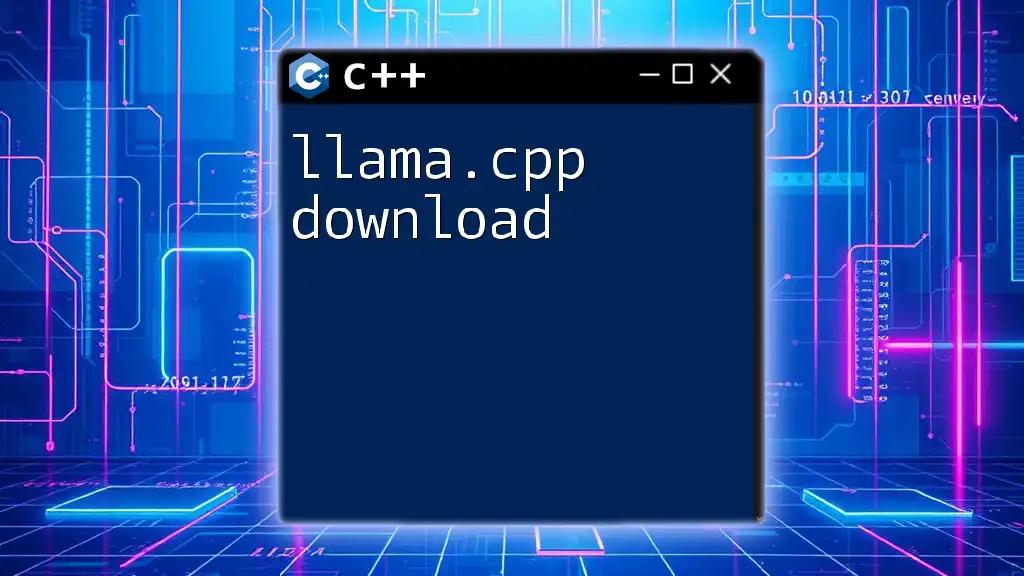
Importance of CPU-Only Implementations
CPU-only implementations of Llama CPP are essential because they broaden access to powerful AI tools without necessitating expensive hardware. In situations where budget constraints exist or when deploying applications on devices where a GPU is unavailable, the CPU-only functionality ensures that users can still leverage the capabilities of Llama CPP effectively. Furthermore, CPU processing can often be more than sufficient for lightweight applications or during the development phase.

Setting Up the Environment
System Requirements
To run Llama CPP CPU only, ensure the following system requirements are met:
- Operating System Compatibility: Llama CPP supports various operating systems including Windows, macOS, and Linux.
- Libraries and Dependencies: Necessary dependencies must be installed for Llama CPP to function optimally, including libraries such as CPU optimizations from vendor-specific tools.
Installation Instructions
Installing via Package Managers
For those who prefer quick installations, Llama CPP can be easily installed using popular package managers. For example, on Ubuntu, you can use:
sudo apt install llama-cpp
Building from Source
If you want the latest features or prefer building from the source, follow these steps:
-
Download the Source Code: Clone the repository from the official Llama CPP GitHub page.
-
Build with the following commands:
git clone https://github.com/llama-cpp/llama-cpp.git cd llama-cpp mkdir build && cd build cmake .. make
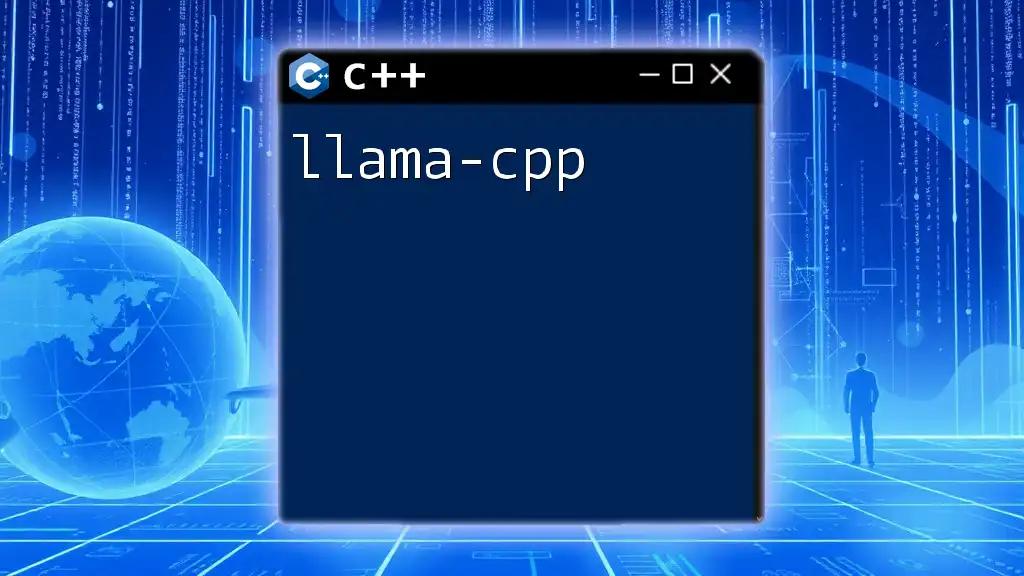
Core Concepts of Llama CPP
Understanding CPU Processing
When utilizing Llama CPP on a CPU, it's vital to understand how CPUs differ from GPUs in processing tasks. CPUs handle a limited number of threads but execute complex instructions more efficiently. This makes them suitable for tasks that require high single-thread performance. However, they may struggle with data-parallel workloads as compared to GPUs, which can execute thousands of threads simultaneously.
Key Features of Llama CPP CPU Only
The CPU-only version retains many of the powerful features of Llama CPP while focusing on optimizations for CPU architecture. This includes:
- Efficient Memory Management: It optimizes how memory is allocated and freed, ensuring minimal overhead.
- Performance Optimization Techniques: Specific optimizations make the most of CPU cache hierarchies, which significantly enhances execution speed.
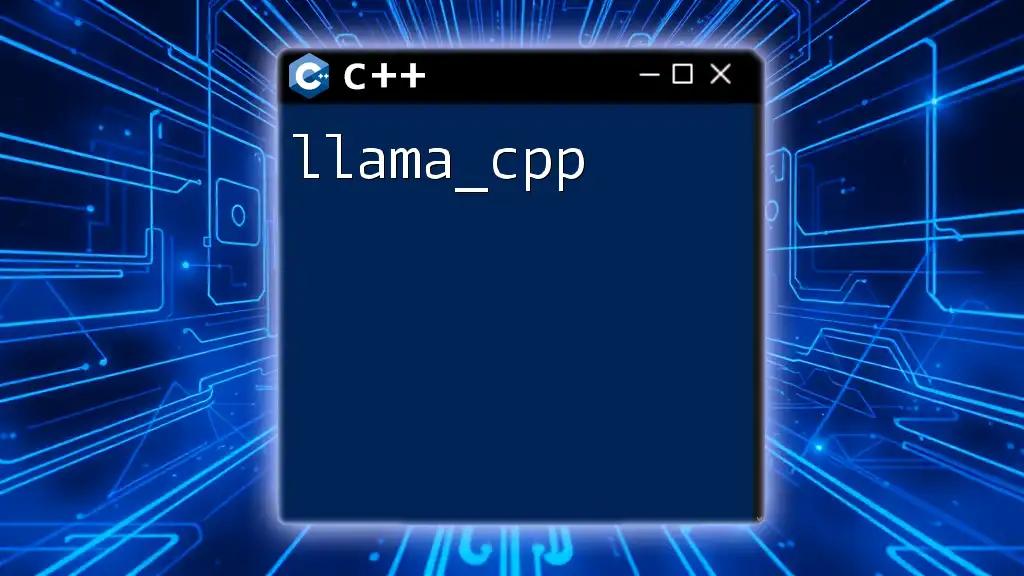
Basic Usage of Llama CPP CPU Only
Running A Simple Example
To get started with Llama CPP CPU only, create a basic example that initializes the pipeline and runs a simple operation. Here’s how you can do it:
#include <iostream>
#include "llama.hpp" // Placeholder for the actual include
int main() {
LlamaCPUPipeline pipeline;
pipeline.initialize();
pipeline.run();
std::cout << "Llama CPP Running on CPU!" << std::endl;
return 0;
}
This code sets up the Llama CPP pipeline flawlessly and displays a confirmation message when executed successfully.
Functionalities Overview
Input and Output Handling
Llama CPP allows users to work with various input formats, including text and binary data. Here’s a simple example of how to handle input and output in Llama CPP:
std::string input = "Hello, Llama CPP!";
pipeline.set_input(input);
std::string output = pipeline.get_output();
std::cout << "Output: " << output << std::endl;
This shows how to set inputs and retrieve outputs seamlessly.
Debugging and Logging
To help developers troubleshoot their applications, Llama CPP includes built-in logging features. Utilize these tools effectively to gain insights during the development process. You can enable logging using a simple configuration option:
pipeline.enable_logging(true);
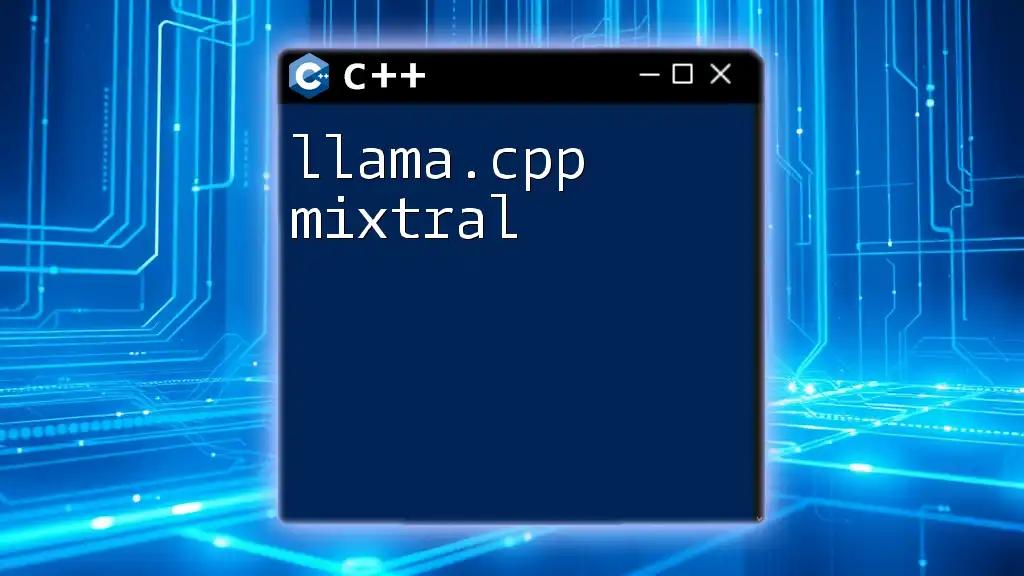
Advanced Techniques
Performance Optimization
Multi-threading Capabilities
Although CPUs have limitations on the number of threads they can execute, Llama CPP enables effective use of multi-threading. You can utilize threading to enhance performance on CPU-only implementations. Here’s an example of implementing threading:
#include <thread>
void run_in_parallel() {
// Your processing code here
}
for (int i = 0; i < number_of_threads; ++i) {
std::thread t(run_in_parallel);
t.join();
}
By dividing the workload among threads, you can significantly improve processing time.
Memory Management Strategies
Memory optimization is critical for performance in CPU-based processing. Effective strategies include pre-allocating memory and using data structures that minimize fragmentation. Here’s a code snippet demonstrating memory allocation optimization:
// Optimizing memory allocation
std::vector<double> data;
data.reserve(1000); // Allocating memory upfront
This reserved approach reduces the overhead of dynamic allocations during runtime.
Customizing Configuration
Configuration Files
Users can customize Llama CPP functionality using configuration files to streamline their experience. Typically, these files can include parameters for model settings, input formats, and output options. An example configuration file could look like this:
[Model]
Type = "CPU"
InputFormat = "JSON"
OutputFormat = "Text"
This flexibility allows users to tailor the environment according to specific project requirements.
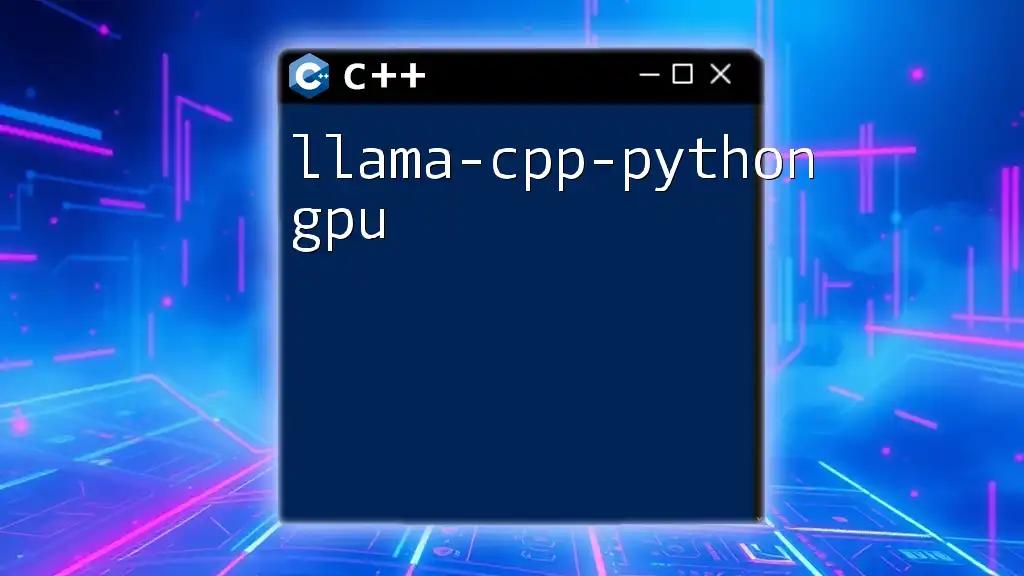
Real-World Applications
Use Cases for Llama CPP CPU Only
Llama CPP CPU only isn't limited to a specific domain; it is versatile and finds applications in various industries such as data analysis, natural language processing, and even IoT devices where computational resources are constrained.
Comparison with GPU Implementations
While GPU implementations can handle more extensive datasets and complex computations, CPU-only solutions can suffice when dealing with lighter workloads. If your application requires quick testing and development without a major hardware investment, opting for Llama CPP CPU only may be the best choice.
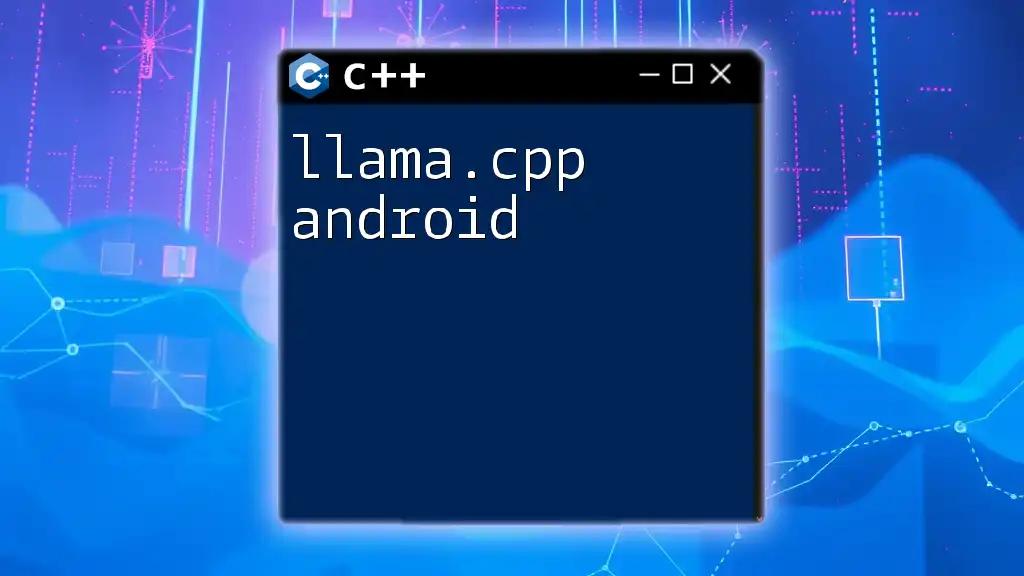
Conclusion
Summary of Key Points
In summary, Llama CPP CPU only enables developers to leverage AI functionalities without demanding GPU resources. Its effective memory management, multi-threading capabilities, and ease of use make it a compelling choice for a wide range of applications.
Future of Llama CPP
As Llama CPP continues to evolve, expect to see more features aimed at enhancing CPU performance and usability. Keep an eye on updates and improvements in future releases to maximize your development potential.
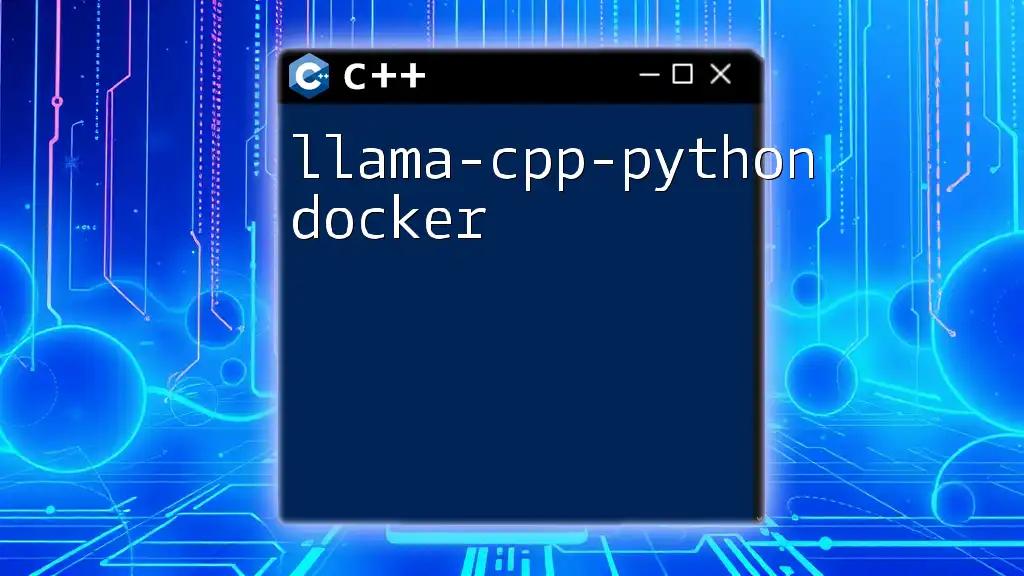
Additional Resources
Documentation and Community
For comprehensive information, refer to the official Llama CPP documentation. Engage with the community through forums and social media for support, tips, and best practices.
Tutorials and Further Reading
Delve into additional tutorials and articles to deepen your understanding of Llama CPP and optimize your applications.