If you're seeking alternatives to `llama.cpp` for implementing Q6 models, consider using lightweight libraries that simplify the process while maintaining efficiency.
Here's a code snippet as an example:
#include <iostream>
#include <q6Model.h>
int main() {
Q6Model model("model_config.json");
model.load();
model.run();
std::cout << "Q6 model executed successfully!" << std::endl;
return 0;
}
Understanding the Q6 Model
What is the Q6 Model?
The Q6 model stands as a significant advancement in programming paradigms, providing a structured environment for developers to create efficient applications. It leverages advanced algorithms and frameworks to streamline processes like data manipulation, analysis, and machine learning.
This model is widely used in fields such as data science, computer vision, and natural language processing, offering a robust platform for building sophisticated applications. However, users often seek effective integrations with libraries that can facilitate better performance, and that's where examining llama.cpp alternatives for Q6 model becomes crucial.
Integration with Llama.cpp
Llama.cpp is a versatile C++ library designed to provide tools for handling complex computational tasks. When integrated with the Q6 model, it allows for efficient processing of large datasets. Nonetheless, users have reported certain limitations, such as reduced flexibility in certain operations, which prompts the exploration of alternatives.
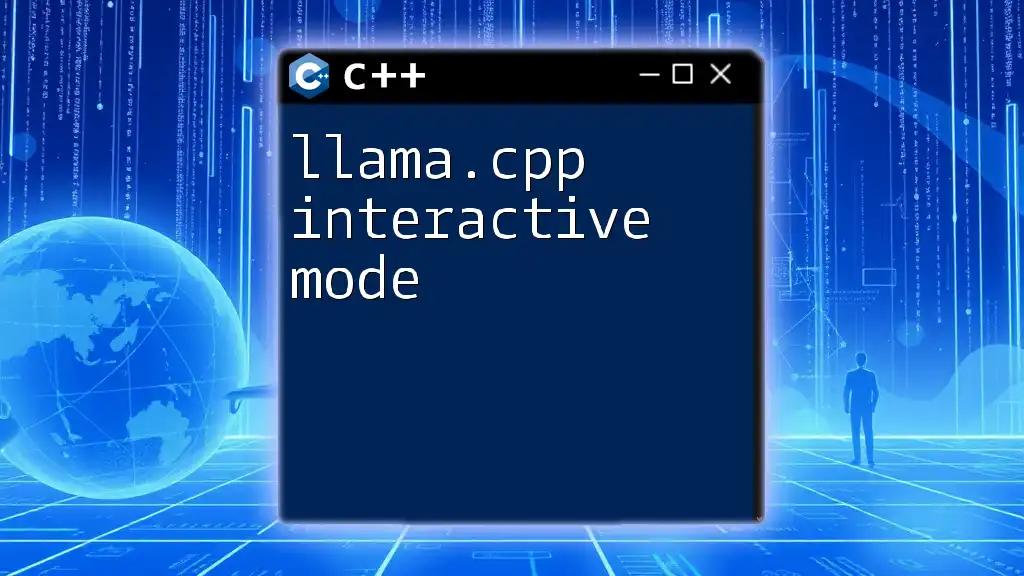
Exploring Alternatives to Llama.cpp
Why Consider Alternatives?
While Llama.cpp certainly serves its purpose, it might not fulfill every requirement for the Q6 model, particularly regarding performance and compatibility. The following aspects shed light on why exploring alternatives may be beneficial:
- Performance: Other libraries may offer faster execution times for specific tasks.
- Functionality: Some alternatives provide functionalities that Llama.cpp lacks.
- Ease of Use: Depending on the user’s familiarity with certain libraries, switching could lead to easier implementations and faster learning curves.
Alternative Libraries and Frameworks
OpenBLAS
OpenBLAS is a highly optimized implementation of the Basic Linear Algebra Subprograms (BLAS). It takes full advantage of modern CPU features and provides an effective way to accelerate computations.
Pros:
- Excellent performance for matrix operations
- Optimized for many CPU architectures
Cons:
- Complexity in installation for some users
- May require additional tuning for optimal performance
Code Example: Integrating OpenBLAS in Q6
#include <cblas.h>
// Example of matrix multiplication using OpenBLAS
void matrixMultiplication(double *A, double *B, double *C, int N) {
cblas_dgemm(CblasRowMajor, CblasNoTrans, CblasNoTrans, N, N, N, 1.0, A, N, B, N, 0.0, C, N);
}
This snippet establishes a basic matrix multiplication algorithm that showcases how easy it is to perform complex mathematical operations with OpenBLAS.
Eigen
Eigen is another powerful library designed for linear algebra operations. It is well-known for its user-friendly API and supports various operations needed to work seamlessly with the Q6 model.
Pros:
- Strong emphasis on usability and simplicity
- Excellent documentation and community support
Cons:
- Potentially slower than specialized libraries for specific tasks
Code Example: Using Eigen with Q6
#include <Eigen/Dense>
using namespace Eigen;
void exampleFunction() {
MatrixXd A(2, 2);
A << 1, 2, 3, 4;
MatrixXd B = A.transpose();
}
Here, Eigen captures the essence of matrix operations, providing an intuitive approach to defining and manipulating matrices.
TensorFlow C++ API
TensorFlow's C++ API allows developers to implement machine learning models in C++ while harnessing the full power of TensorFlow.
Pros:
- State-of-the-art performance for deep learning applications
- High-level and low-level APIs for versatility
Cons:
- Steeper learning curve compared to simpler libraries
- Larger overhead than smaller alternatives
Code Example: TensorFlow C++ Integration
#include "tensorflow/cc/client/client_session.h"
#include "tensorflow/cc/ops/standard_ops.h"
using namespace tensorflow;
void runTensorFlow() {
Scope root = Scope::NewRootScope();
auto A = ops::Const(root.WithOpName("A"), {{3.0, 2.0, -1.0}});
// Additional TensorFlow operations can be added here
}
This example illustrates how TensorFlow can be integrated into projects utilizing the Q6 model, particularly focusing on easy scalability for machine learning applications.
Comparing Performance and Usability
When venturing into the realm of llama.cpp alternatives for Q6 model, it is vital to assess performance against usability. Selecting the right library should be based on careful benchmarking.
Benchmarking Techniques
To ensure that your chosen alternative surpasses Llama.cpp, consider using tools like Google Benchmark or similar profilers that enable detailed performance reports. This will allow you to measure execution times, memory usage, and other essential performance metrics rigorously.
Ease of Integration
Integration is another significant factor; a library that seamlessly integrates with your existing code and structure saves development time. Make sure to check the compatibility with other tools and libraries that you might be using in conjunction with the Q6 model. Be on the lookout for common integration pitfalls and consider the support available for each alternative.
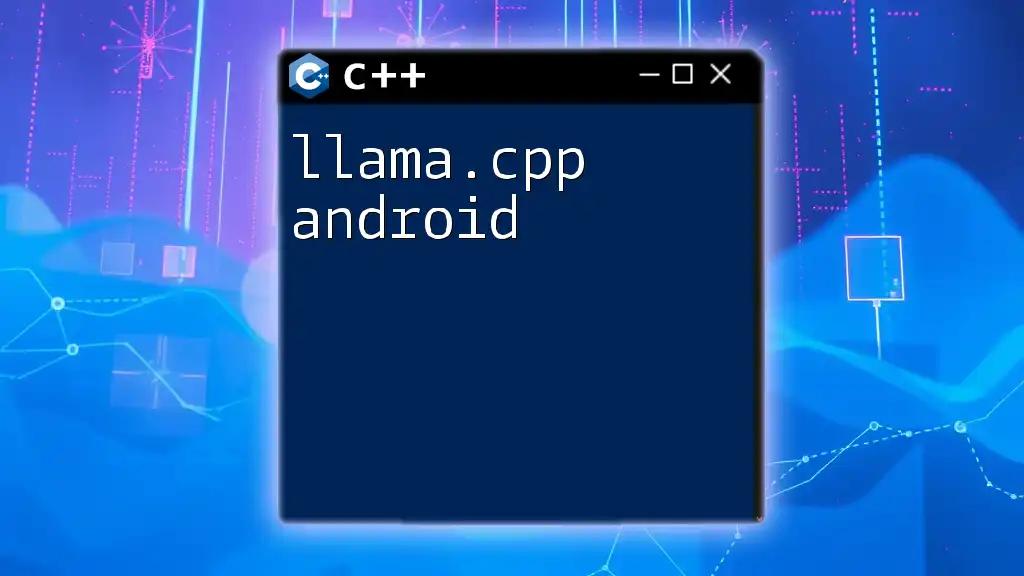
Additional Libraries Worth Considering
MKL (Intel Math Kernel Library)
MKL is vital for performance-critical applications. It provides highly-efficient implementations of mathematical functions and is optimized specifically for Intel architectures.
Code Example: Below is a concise snippet that demonstrates using MKL for matrix-vector multiplication, showcasing its potential performance benefits.
#include <mkl.h>
// Example for matrix-vector multiplication with MKL
void mklExample(const double *A, const double *x, double *y, int N) {
cblas_dgemv(CblasRowMajor, CblasNoTrans, N, N, 1.0, A, N, x, 1, 0.0, y, 1);
}
Armadillo
Armadillo is another library that excels in linear algebra. It combines ease of use with performance and is especially convenient for applications that require rapid development.
Usage with Q6: Armadillo's matrix manipulation capabilities are particularly beneficial for Q6 model developers who require a straightforward approach to handle complex data structures.
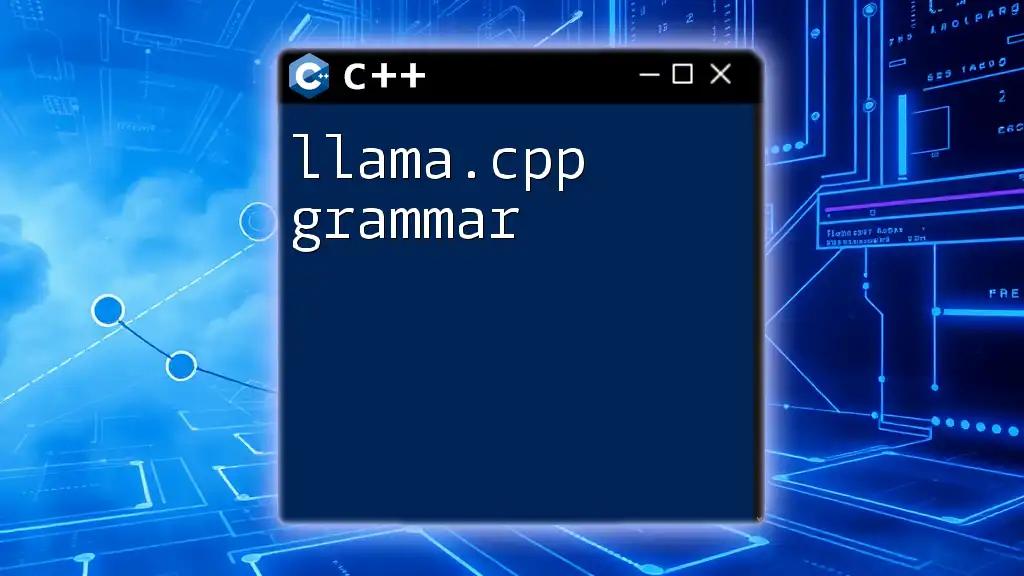
Best Practices When Choosing Alternatives
Considerations for Selection
When defining your criteria for the best llama.cpp alternatives for your Q6 model, consider:
- Performance Needs: Will the alternative provide a tangible boost in speed or efficiency?
- Development Speed: Is the time invested in integrating a particular library worth the benefits gained?
- Community and Documentation: Strong documentation and community support can significantly ease the learning curve, offering guidance when necessary.
Trial Runs and Prototyping
Always engage in trial runs with any alternative library. Creating small prototypes allows you to experiment with functionality while assessing ease of integration without fully committing to adoption. It’s your opportunity to explore what works best for your specific requirements.
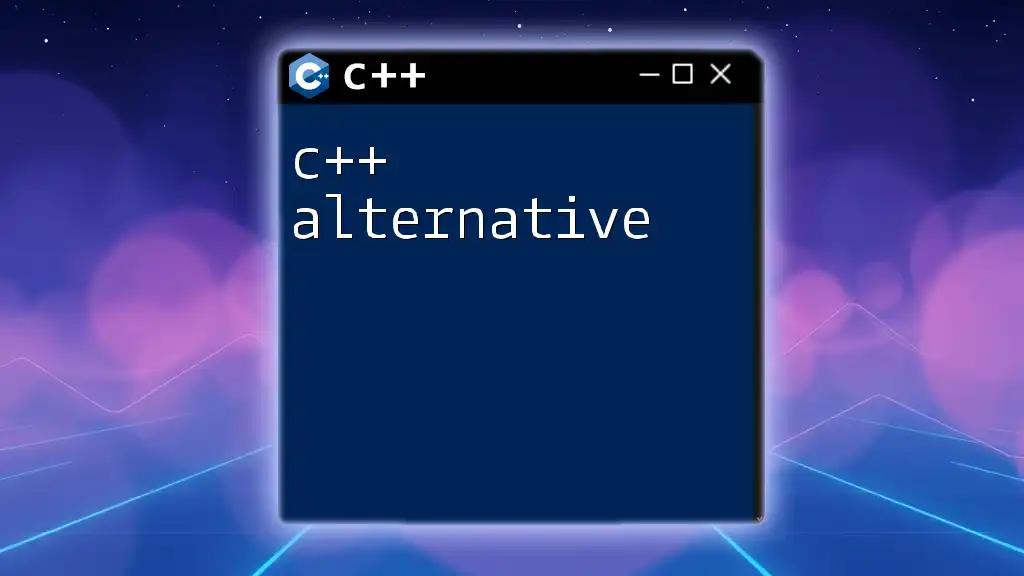
Conclusion
Exploring llama.cpp alternatives for the Q6 model can significantly enhance your development experience, provide alternative functionalities, and improve performance. Libraries such as OpenBLAS, Eigen, TensorFlow, MKL, and Armadillo offer a range of tools that could become integral to your projects. By carefully considering your unique needs and testing various options available, you can create a more efficient coding environment. Don’t hesitate to experiment with these alternative libraries to discover what best suits your needs in the Q6 model.