C++ alternatives include programming languages like Rust and Python, which offer different paradigms and features for system-level programming and scripting, respectively.
Here's a simple example of a C++ command for printing a message:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Alternatives
What Are Programming Language Alternatives?
Programming language alternatives refer to other languages that can be used to achieve similar outcomes as C++. While C++ is a powerful language for systems programming, there are scenarios where other languages may provide advantages that make them more suitable. The choice of programming language can affect the ease of development, performance, and even the maintenance of the codebase.
Why Seek Alternatives to C++?
There are numerous reasons why developers might look for a C++ alternative:
- Performance Demands: Certain applications may require rapid development cycles, where the efficiency of coding in a different language helps meet deadlines.
- Ease of Learning for Newcomers: Languages like Python or JavaScript are often seen as more beginner-friendly, making them appealing for new programmers.
- Targeting Different Ecosystems: Depending on the project requirements, you may wish to focus on web, mobile, or embedded systems where specific languages excel.
- Industry Demand and Job Market Considerations: The popularity of languages evolves, and understanding the current trends may guide your choice of a programming language.
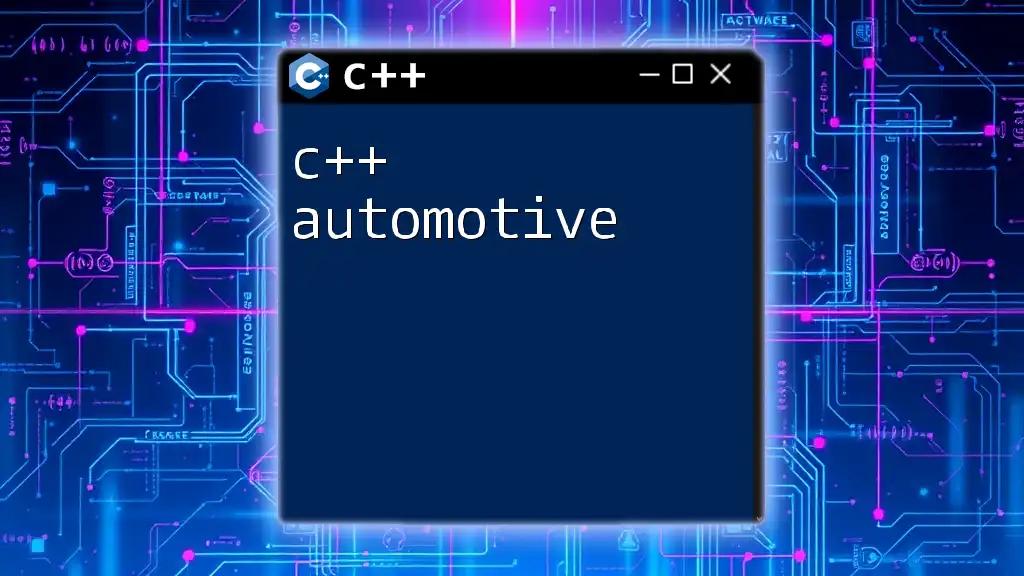
Popular Alternatives to C++
C#
Overview
C# is a high-level language developed by Microsoft as part of its .NET framework. It provides a versatile platform for developing a variety of applications, from web services to desktop software. C# has gained traction for its ease of use and powerful features that resemble C++ in terms of structure but simplify many tasks.
Key Features
C# comes with several noteworthy features that make it a compelling alternative:
- Strong Type-Checking: Helps catch errors at compile time, reducing runtime bugs.
- Garbage Collection: Manages memory automatically, allowing developers to focus more on their logic rather than memory management.
- Rich Class Libraries: Provides a broad range of built-in functionality that accelerates development.
Example Code Snippet
using System;
class Program
{
static void Main()
{
Console.WriteLine("Hello, World!");
}
}
This simple program demonstrates the straightforward syntax of C#, making it accessible for new developers.
Java
Overview
Java is an established language that commands a robust ecosystem. Known for its "Write Once, Run Anywhere" philosophy, Java applications are compiled into bytecode that runs on any platform equipped with a Java Virtual Machine (JVM). This cross-platform capability makes it a popular choice for enterprise applications.
Advantages Over C++
Java offers several benefits when compared to C++:
- Automatic Memory Management: Java’s garbage collector automatically frees up unused memory, allowing developers to avoid the complexities of manual memory handling.
- Robust Standard Libraries: It comes with a rich set of libraries that provide numerous functionalities, allowing developers to implement complex solutions without reinventing the wheel.
Example Code Snippet
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
This example showcases Java’s syntax, which prioritizes clarity and simplicity.
Rust
Overview
Rust is a modern systems programming language designed with safety and performance in mind. One of its primary goals is to provide memory safety—ensuring that developers can write concurrent code without fear of data races or crashes.
Key Benefits
Rust sets itself apart through:
- Memory Safety Without Garbage Collection: Utilizes ownership rules that help ensure memory is managed safely without the need for traditional garbage collection.
- Zero-Cost Abstractions: Promises that higher-level features won’t incur additional runtime costs, allowing developers to write expressive yet efficient code.
Example Code Snippet
fn main() {
println!("Hello, World!");
}
This simple Rust program highlights the language’s focus on safety and performance while maintaining readability.
Go
Overview
Developed by Google, Go (or Golang) is known for its simplicity and efficiency, particularly in cloud services and distributed systems. Its design focuses on fast compilation times and ease of use, particularly for web services.
Strengths
Go stands out for its:
- Small Syntax: Simplicity in syntax makes it quick to learn and implement.
- Concurrency Features: Utilizes goroutines and channels, making it easy to manage multiple tasks simultaneously—a key asset for networked applications.
Example Code Snippet
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
The straightforward syntax of Go supports rapid development, especially for programmers familiar with simpler languages.
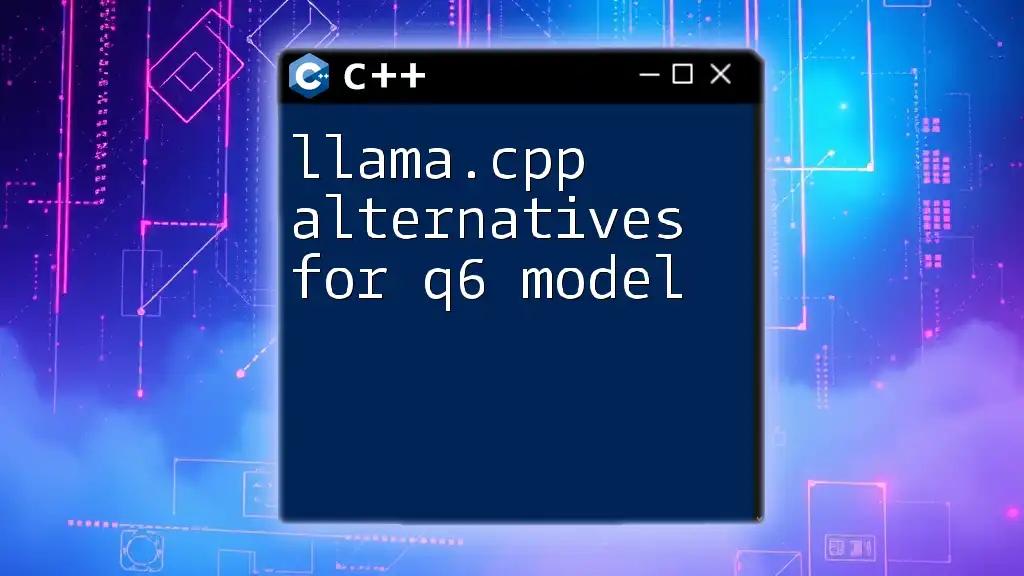
Comparing C++ and Its Alternatives
Performance
Performance comparison often depends on the specific use case. While C++ is traditionally favored for low-level system tasks and performance-intensive applications, languages like Rust and Go bring a modern approach to concurrency and memory safety. In the scenarios where runtime and memory efficiency are crucial, C++ may still outperform its alternatives.
Ease of Use
Ease of use is subjective and varies by individual experience. Generally, languages such as Python and JavaScript offer a gentler learning curve due to their straightforward syntax and rich documentation. Additionally, C# and Java come with extensive resources, making them easy to adopt for beginners transitioning from C++.
Ecosystem and Tooling
The ecosystem plays a significant role in language popularity. While C++ boasts a mature ecosystem with extensive libraries, alternatives like Java and C# have also developed solid community support and tooling. Each alternative language has IDEs and development tools that streamline programming tasks, often surpassing the tooling available for C++.
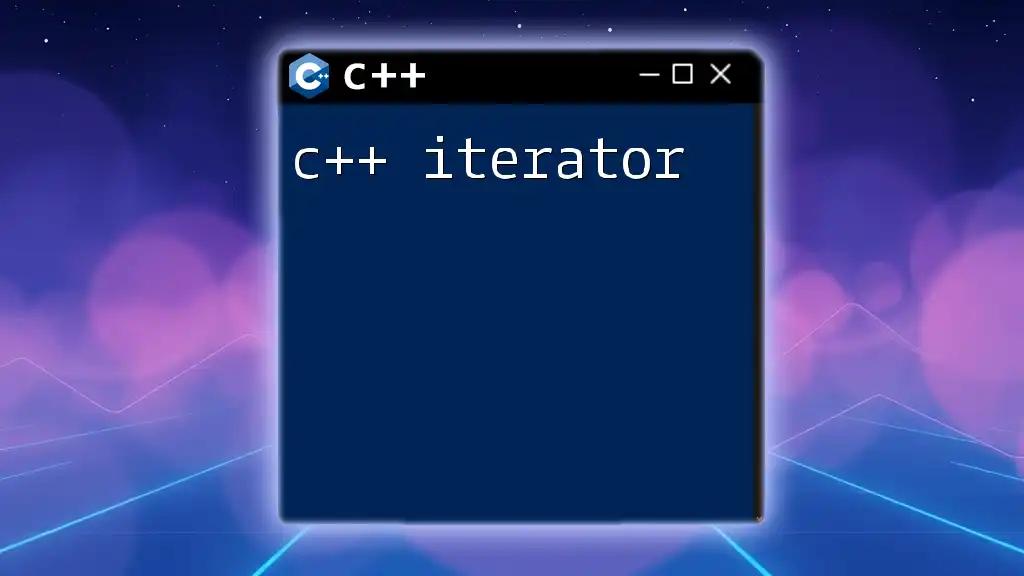
Evaluating Your Project’s Needs
Choosing the Right Alternative
When selecting a C++ alternative, consider the following criteria:
- Performance requirements: If execution speed is paramount, C++ or Rust may be suitable.
- Ease of deployment: For web applications, Java or C# could provide advantages in deployment and scaling.
- Team expertise: Ensure your team's familiarity and comfort with the language to avoid steep learning curves.
Real-World Use Cases
Case Studies
There are numerous instances of companies that moved away from C++ to languages such as Rust or Go to take advantage of their flexible concurrency models and safety features. For example, Mozilla uses Rust for performance-critical applications, while Dropbox has adopted Go for its backend services to enhance development speed and maintainability. Each migration possesses its challenges, including the need for retraining developers and managing legacy codebases.
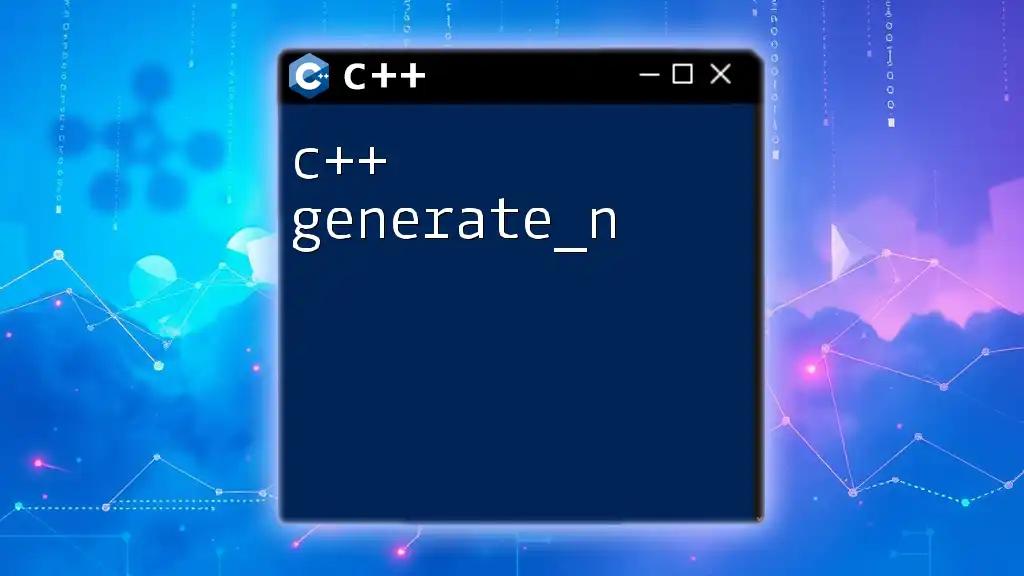
Conclusion
Exploring alternatives to C++ is essential for adapting to evolving project needs and programming landscapes. Choosing the best language is context-dependent; thus, understanding these alternatives can empower developers to make informed decisions.
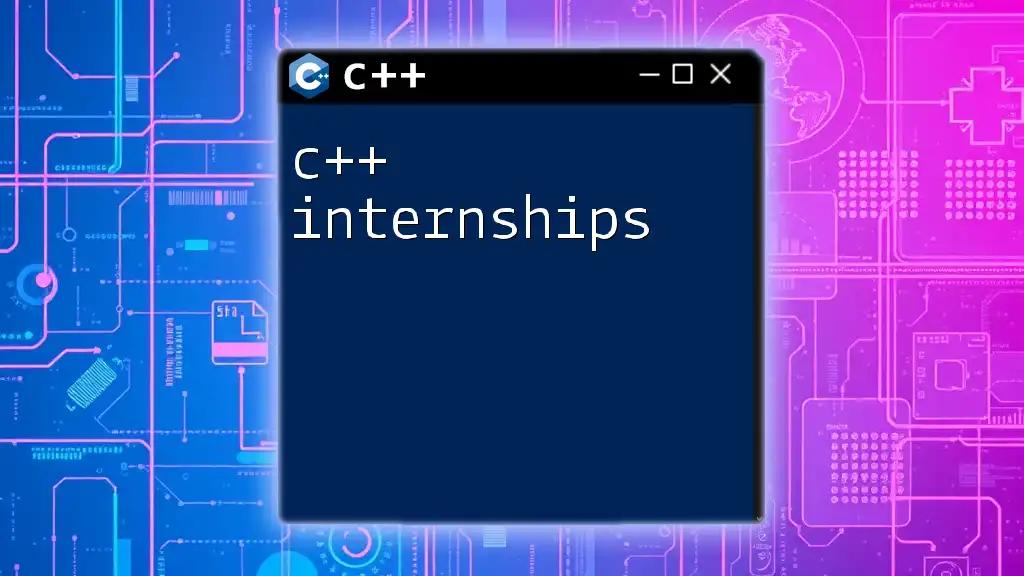
Call to Action
Experiment with these alternatives and discover which one best suits your project or development style. Share your experiences or seek assistance within programming communities to enhance your learning journey.

Additional Resources
Books and Online Courses
Seek out books and online platforms that cover each alternative language in-depth, allowing you to bolster your skills and understanding comprehensively.
Community Forums and Groups
Engagement with online communities can provide real-time help and foster discussions about best practices within specific languages.
Tools and Libraries
Take advantage of necessary tools and libraries in these languages that can streamline your development workflow and expand your capabilities.