In C++, negative infinity can be represented using the `-std::numeric_limits<double>::infinity()` function from the <limits> header, allowing for calculations that need to incorporate a negative infinite value.
Here’s a code snippet demonstrating this:
#include <iostream>
#include <limits>
int main() {
double negativeInfinity = -std::numeric_limits<double>::infinity();
std::cout << "Negative Infinity: " << negativeInfinity << std::endl;
return 0;
}
Understanding Infinity in C++
In programming, infinity typically refers to a value that exceeds the maximum representable number in a given data type. In C++, this concept is particularly relevant in floating-point arithmetic, where a value can be effectively limitless in either direction.
The IEEE 754 Standard governs how floating-point numbers are represented in most programming languages, including C++. This standard specifies how to model positive and negative infinity as well as how to handle computations involving these values. Understanding this foundational concept is essential for working with computations that may exceed the limits of normal numeric representation.
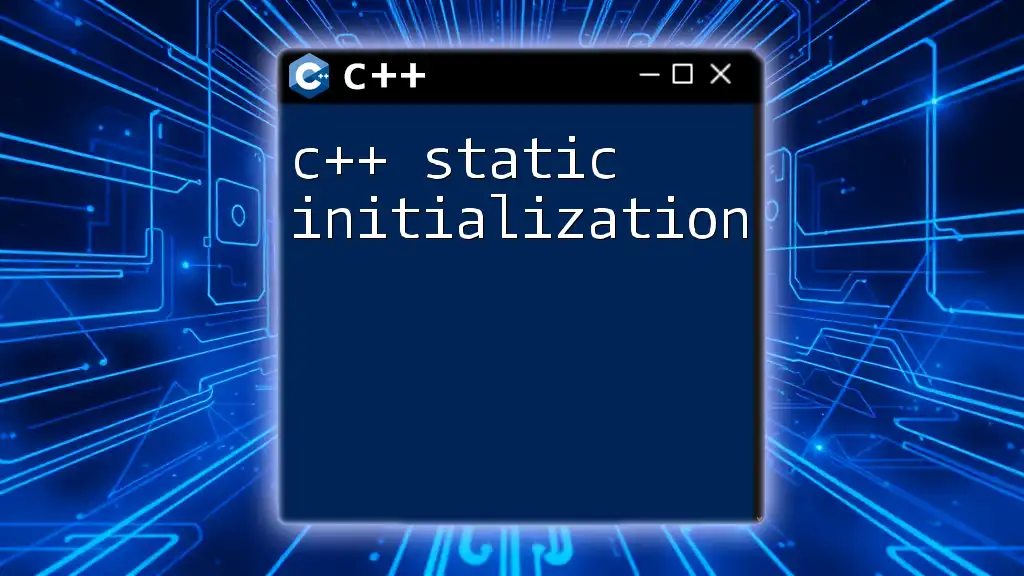
What is Negative Infinity in C++?
Negative infinity refers to a value that approaches negative limitless magnitude. It is crucial to recognize that negative infinity is not merely a very large negative number. Instead, it represents a mathematical concept that allows for comparisons and operations that intuitively understand numbers extending indefinitely in the negative direction.
For example, in mathematical operations:
- Any number, when added to negative infinity, results in negative infinity.
- Multiplying any positive number by negative infinity yields negative infinity, while multiplying by a negative number results in positive infinity.
This behavior allows developers to work effectively with values at the extremes, particularly in algorithms and data comparisons.
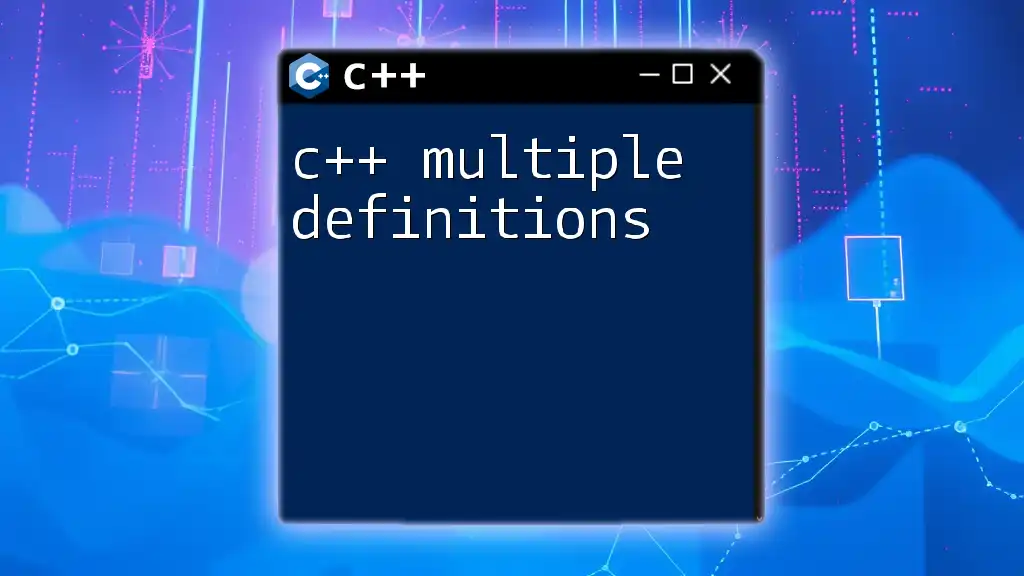
Creating Negative Infinity in C++
To create negative infinity in C++, you typically use the `<limits>` header, which provides a standardized way to access these special values.
Here’s how you can define negative infinity:
#include <limits>
double negInfinity = -std::numeric_limits<double>::infinity();
In this code snippet:
- We include `<limits>`, which is necessary to access the numeric limits provided by C++.
- The `std::numeric_limits<double>::infinity()` function fetches the positive infinity value, and we simply negate it to create negative infinity.
This elegant approach makes it easy to use negative infinity in your calculations.
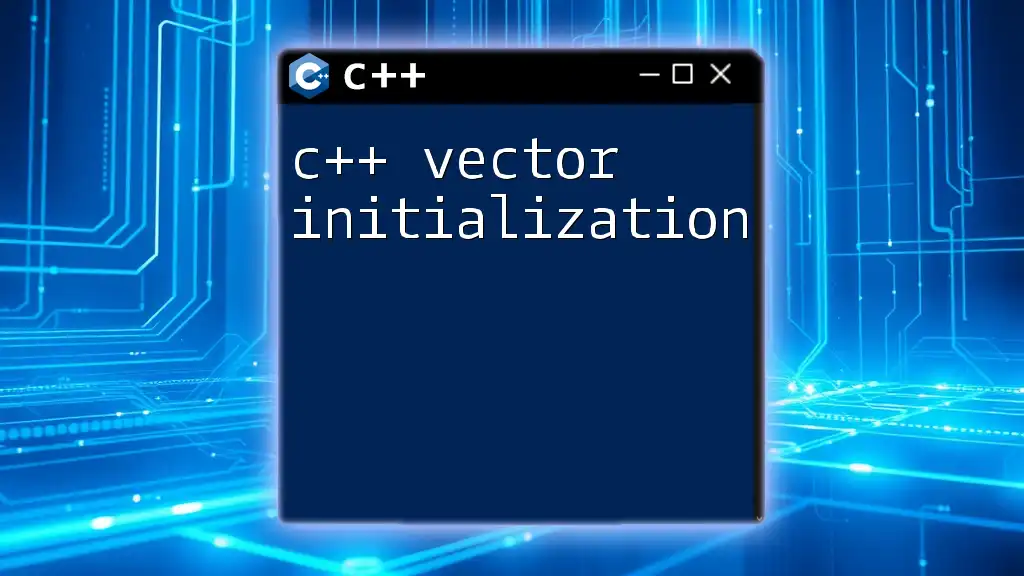
Practical Applications of Negative Infinity
Negative infinity has several practical applications in programming:
-
Algorithm Initialization: In algorithms, particularly those involved in comparisons like finding minimum values, initializing a variable with negative infinity sets a benchmark that will be reliably surpassed.
-
Data Structures: It is useful in priority queues where the minimum needs to be tracked or when laying out bounds for recursive functions.
For example, consider a simple algorithm designed to find the minimum value in a list:
double minValue = std::numeric_limits<double>::infinity();
for (const auto& elem : arr) {
minValue = std::min(minValue, elem);
}
In this example, initializing `minValue` to positive infinity ensures that any number in `arr` will be lower, allowing the algorithm to correctly identify and return the minimum value.
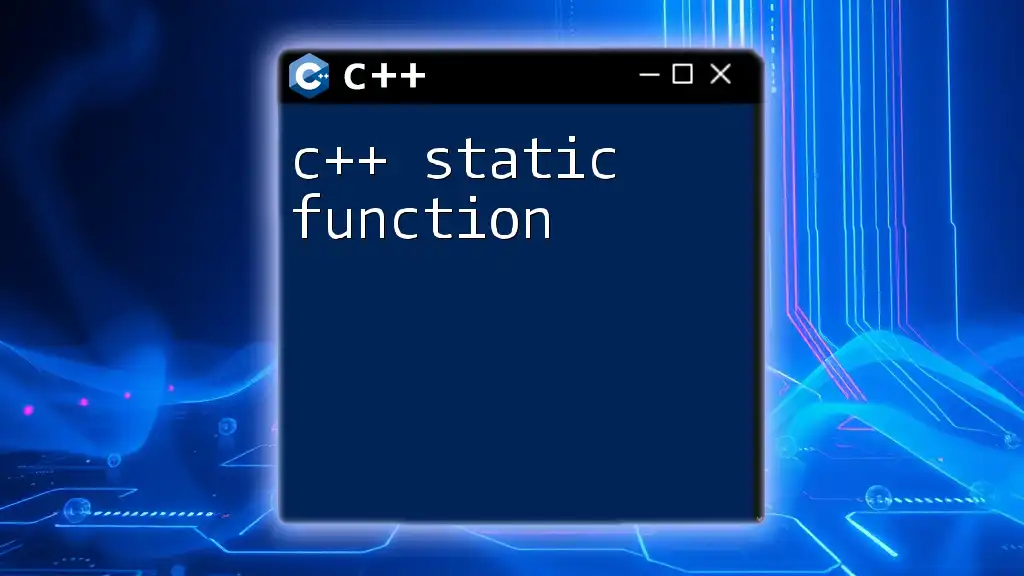
Comparison with Other Special Values
Positive Infinity
While we often think about negative infinity, its counterpart—positive infinity—is equally crucial. Positive infinity is represented as `std::numeric_limits<double>::infinity()` in C++. The behaviors of positive and negative infinity are analogous but opposite; positive infinity represents values greater than all finite numbers.
NaN (Not a Number)
Another important special value in C++ is NaN (Not a Number). This value occurs in floating-point operations that are undefined, such as dividing zero by zero.
Key Differences:
- Negative Infinity: Exists as a valid number and can participate in arithmetic.
- NaN: Represents an undefined or unrepresentable quantity and does not behave as a numeric type.
When working with both negative infinity and NaN, it's vital to handle them properly to prevent unexpected results in computations.
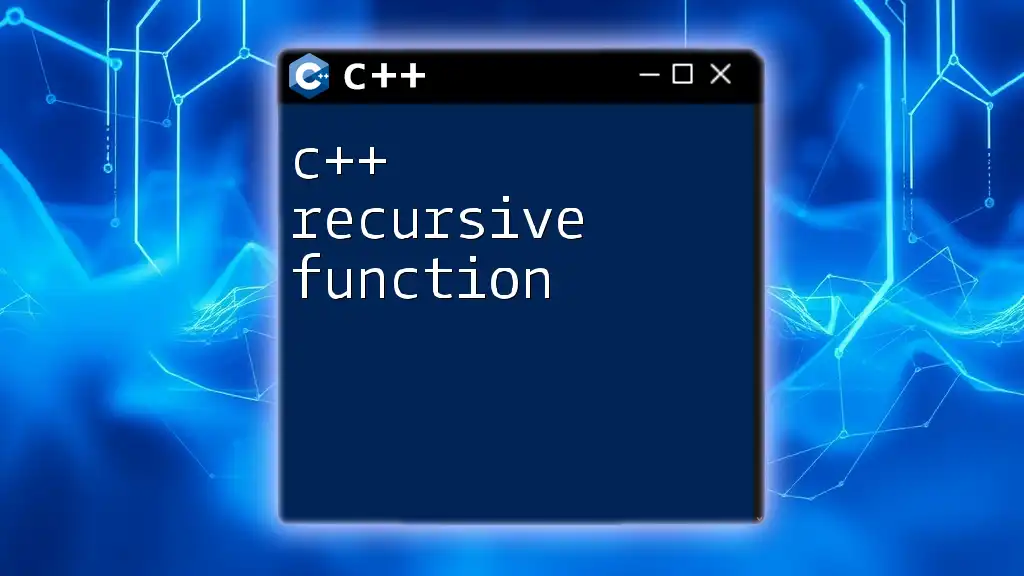
Handling Negative Infinity in C++
Comparisons involving negative infinity are straightforward but require careful attention.
For instance, you can perform a comparison like so:
if (x < -std::numeric_limits<double>::infinity()) {
// Process accordingly
}
This code checks whether `x` is less than negative infinity. However, it’s important to note that any finite value will never be less than negative infinity. Thus, this condition will never be true in practical use.
Furthermore, to check if a value is negative infinity in your program, you can use the `std::isinf` function:
if (std::isinf(negInfinity) && negInfinity < 0) {
// Handle negative infinity case
}
In this case, we first verify that the variable is indeed an infinite value and then check that it is negative.
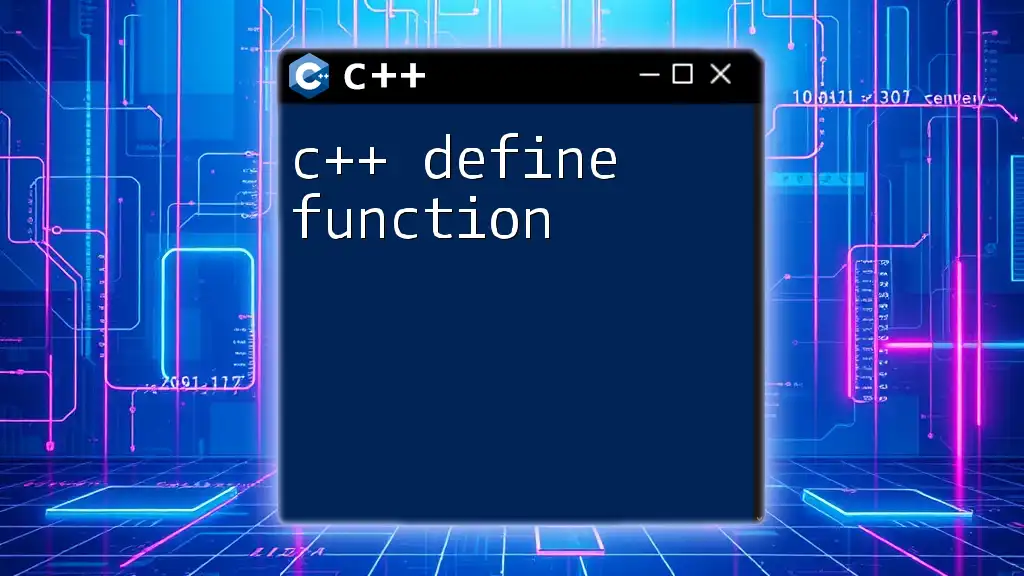
Common Pitfalls and Best Practices
As with any special values, using negative infinity in C++ can lead to potential pitfalls:
-
Misunderstanding Operations: Developers may mistakenly assume arithmetic with negative infinity behaves like regular numbers. It’s essential to remember that operations involving negative infinity follow specific mathematical rules.
-
Confusion with NaN: Mixing up negative infinity with NaN can cause logic errors in your programs. Always validate whether you are working with NaN or an infinite value.
By adhering to best practices, such as initializing variables with negative infinity for comparisons and checking conditions carefully, you can effectively utilize this concept in your coding projects.
Here’s an example of how to implement negative infinity cleanly in a function:
double findMin(const std::vector<double>& values) {
double minVal = std::numeric_limits<double>::infinity();
for (double value : values) {
minVal = std::min(minVal, value);
}
return minVal;
}
In this `findMin` function, we start with `minVal` initialized to positive infinity, then proceed to find and return the minimum value in the provided vector.
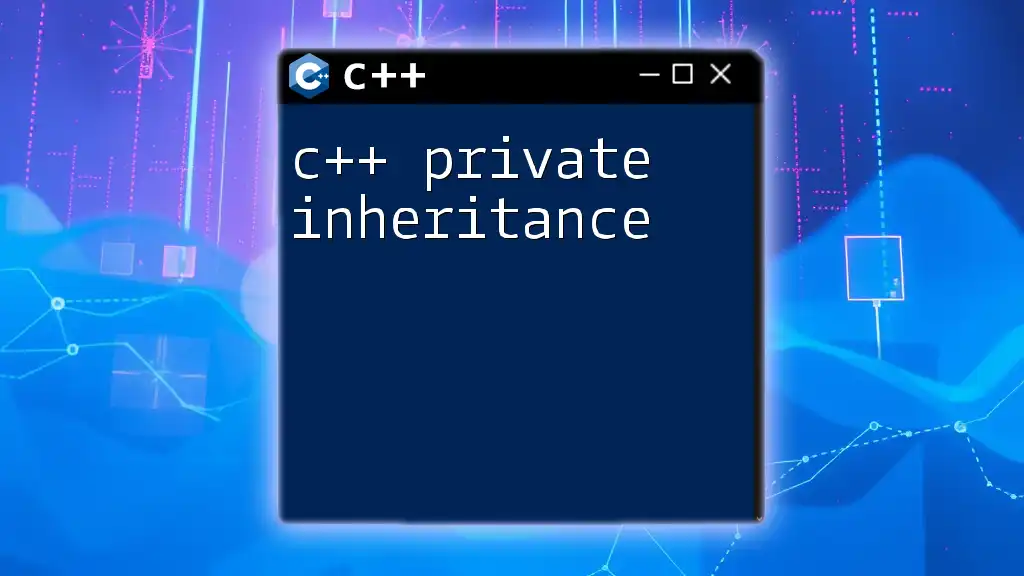
Conclusion
Understanding and using C++ negative infinity is crucial for efficient programming, particularly in cases involving comparison algorithms and extreme value management. By mastering this concept, developers can enhance their C++ coding skills, leading to more robust and error-resistant applications.
As you explore C++, practice using negative infinity in various coding challenges to solidify your understanding. Familiarizing yourself with this important mathematical concept will serve you well in your programming journey.
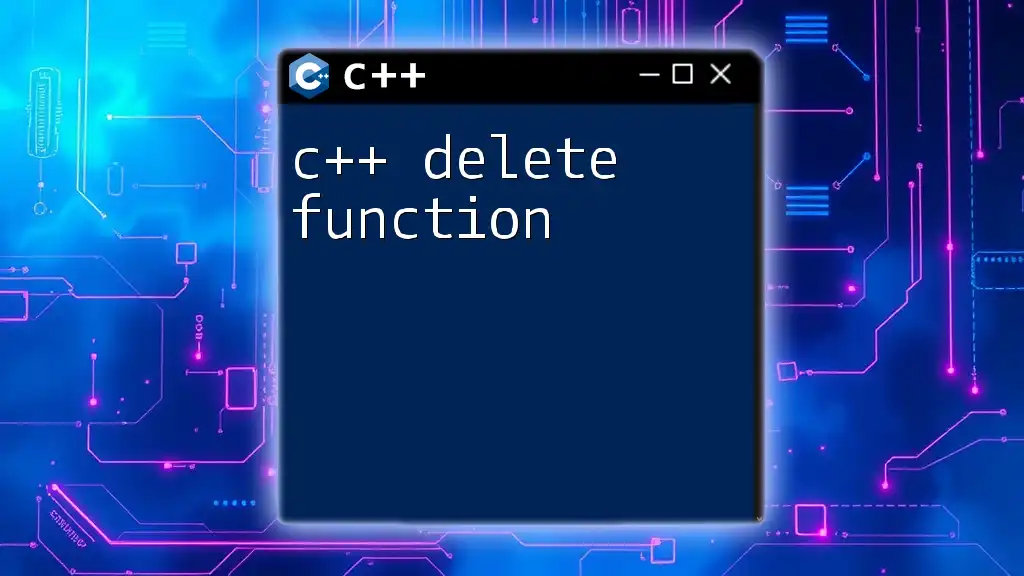
Further Resources
For those wishing to delve deeper into C++ programming, consider reviewing the official C++ documentation or exploring recommended books and tutorials for a broader understanding of special values and program architecture.
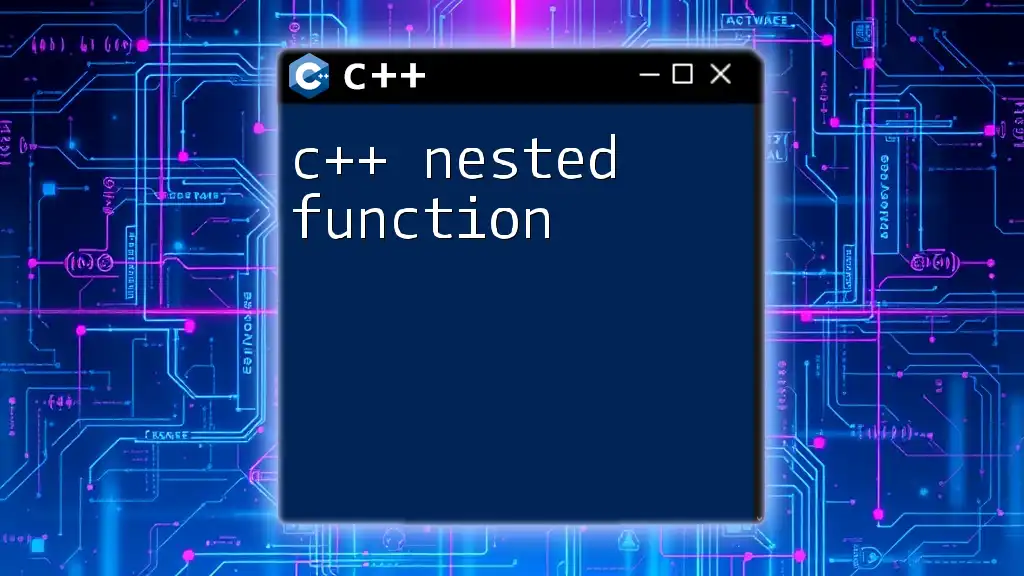
FAQs
-
What happens if I add a number to negative infinity?
Adding any number to negative infinity will yield negative infinity. -
Can negative infinity be used in integer operations?
Negative infinity is defined for floating-point arithmetic. Integer types do not support infinity directly. -
How to debug issues related to negative infinity in C++?
Use debug prints to track the state of variables and verify if any invalid operations have led to unexpected behavior.