You can iterate through a string in C++ using a simple range-based for loop to access each character individually. Here's an example:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
for (char c : text) {
std::cout << c << ' ';
}
return 0;
}
Understanding Strings in C++
What is a String?
A string in C++ is a sequence of characters that can be manipulated as a single entity. It is an essential data type in programming used for handling textual data. C++ offers two main types of strings:
-
C-style strings: These are arrays of characters terminated by a null character (`'\0'`). They have limitations that include manual memory management and the lack of built-in methods for manipulation.
-
C++ string class: Introduced in the C++ Standard Library, the `std::string` class overcomes the limitations of C-style strings. It provides various member functions for easier string manipulation and automatic memory management.
C++ String Class
The `std::string` class is part of the C++ Standard Library and provides a rich set of functionalities. Some key methods include:
- `length()`: Returns the length of the string.
- `append()`: Adds a string to the end.
- `substr()`: Extracts a substring.
- `find()`: Locates a character or substring within the string.
- `replace()`: Replaces a section of the string with another.
Understanding these functionalities allows programmers to manipulate strings effectively.
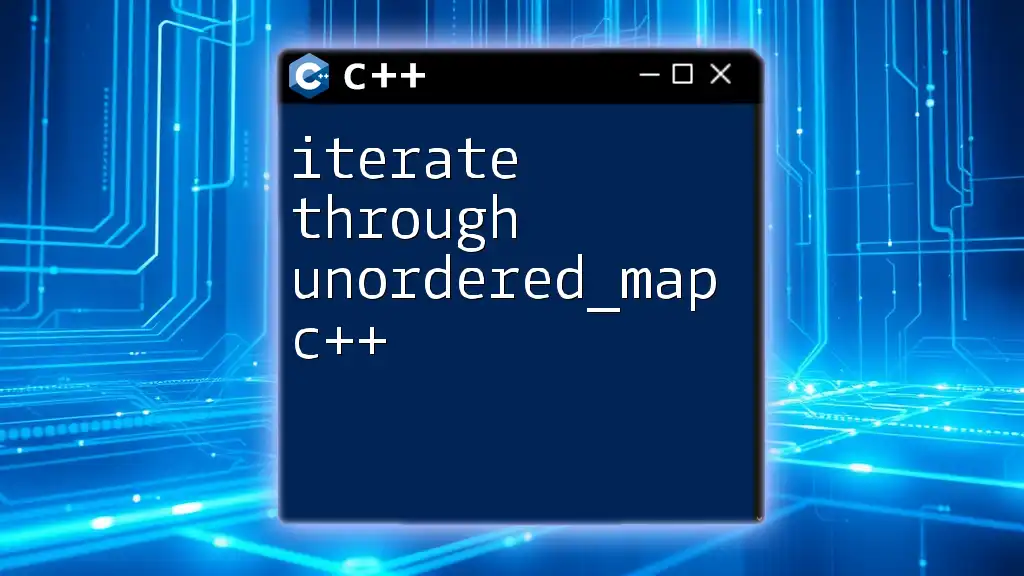
Methods to Iterate Through Strings in C++
Using a Standard for Loop
The traditional for loop is one of the most common methods to iterate over strings in C++. It provides precise control over the loop's execution.
Example: Iterating with a Standard For Loop
std::string myString = "Hello, World!";
for (int i = 0; i < myString.length(); i++) {
std::cout << myString[i] << std::endl;
}
In this example, we manually track the index `i`, which allows us to access each character in the string using `myString[i]`. This method, while straightforward, can lead to potential issues, such as off-by-one errors if not managed correctly.
Using a Range-Based For Loop
Introduced in C++11, the range-based for loop simplifies the syntax and eliminates the need for an index variable when iterating over strings.
Example: Iterating with Range-Based For Loop
std::string myString = "Hello, World!";
for (char character : myString) {
std::cout << character << std::endl;
}
In this example, each character in `myString` is directly assigned to the variable `character` in each iteration. This method not only improves readability but also minimizes errors related to indexing.
Using Iterators
Iterators provide a powerful way to traverse strings. They act as pointers to the elements of the container, enabling more complex manipulations.
Example: Iterating with String Iterators
std::string myString = "Hello, World!";
for (std::string::iterator it = myString.begin(); it != myString.end(); ++it) {
std::cout << *it << std::endl;
}
Here, we initialize the iterator `it` to the beginning of `myString` and continue until we reach the end. The `*it` syntax accesses the value at the current position. This method is particularly useful for modifying elements during iteration or when using algorithms that rely on iterators.
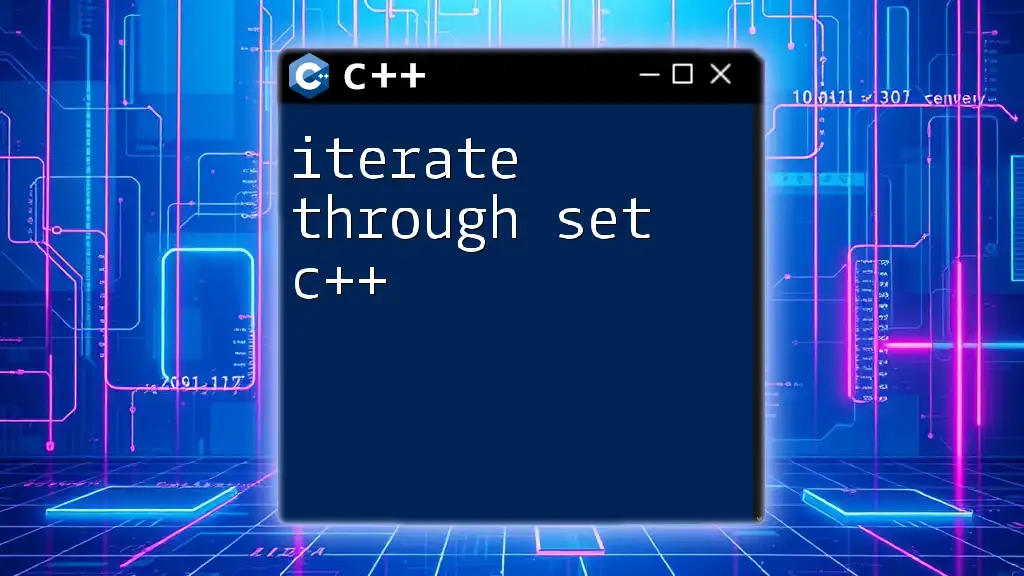
Advanced Iteration Techniques
Using std::for_each with Lambdas
C++ offers the `std::for_each` algorithm, which can be paired with lambda functions for even more succinct and expressive code.
Example: Using std::for_each
#include <algorithm>
std::string myString = "Hello, World!";
std::for_each(myString.begin(), myString.end(), [](char character) {
std::cout << character << std::endl;
});
In this example, we utilize `std::for_each` to apply a lambda expression to every character in the string. This approach increases flexibility for complex operations, such as conditional checks or transformations.
Using std::string Methods for Iteration
Manipulating strings often requires finding characters or substrings, and methods such as `find()`, `substr()`, and `replace()` come in handy.
Example: Finding Characters in a String
std::string myString = "Hello, World!";
size_t position = myString.find('o');
while (position != std::string::npos) {
std::cout << "Found 'o' at: " << position << std::endl;
position = myString.find('o', position + 1);
}
In this example, we use `find()` to locate the position of the character `'o'` within `myString`. The loop continues until no more occurrences are found (indicated by `std::string::npos`). This example showcases how to efficiently iterate through string contents by searching for specific characters.
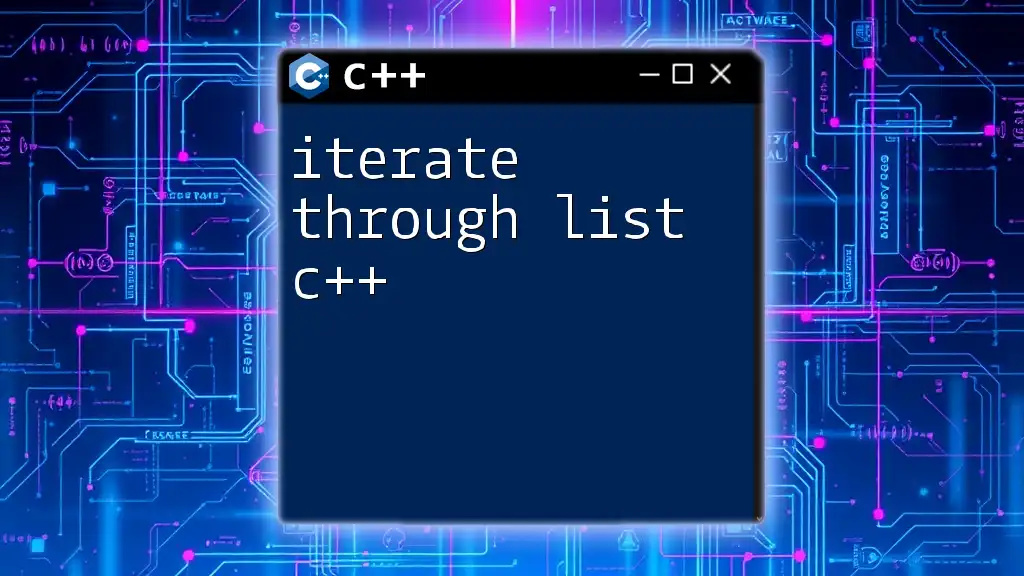
Common Iteration Mistakes to Avoid
Off-By-One Errors
One of the most prevalent issues when iterating through strings is the off-by-one error, where the index goes out of bounds. Such mistakes can lead to runtime errors or unexpected behavior, especially in arrays. To avoid these errors, it's crucial to use the `.length()` method or similar functions correctly to determine loop boundaries.
Invalid Iterator Use
When working with iterators, it’s important to avoid invalidating them inadvertently. For example, modifying the string while iterating through it can lead to undefined behavior. Always ensure that the same string object isn't modified during iteration.
Mismatching String Types
Mixing C-style strings and C++ strings can lead to several issues, including memory leaks and access violations. Always convert C-style strings to `std::string` when performing manipulations to enjoy the benefits of C++'s built-in safety features.
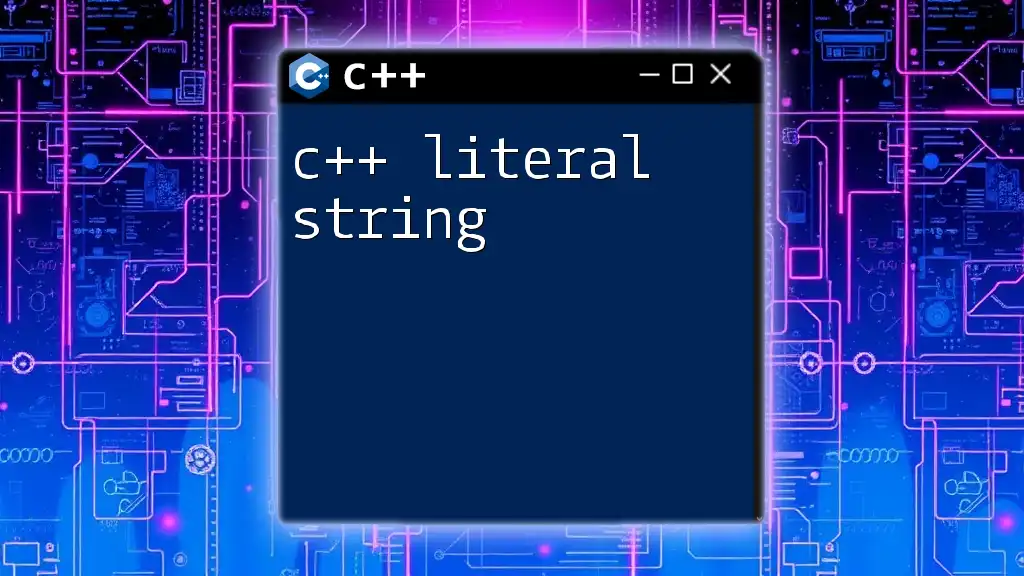
Conclusion
Mastering the various ways to c++ iterate through string effectively enhances your programming skill set, especially for tasks involving text data. Understanding the nuances of iterators, control structures, and string methods fosters better coding practices and leads to more efficient programs. Practice implementing these techniques to solidify your skills and add versatility to your C++ toolbox.
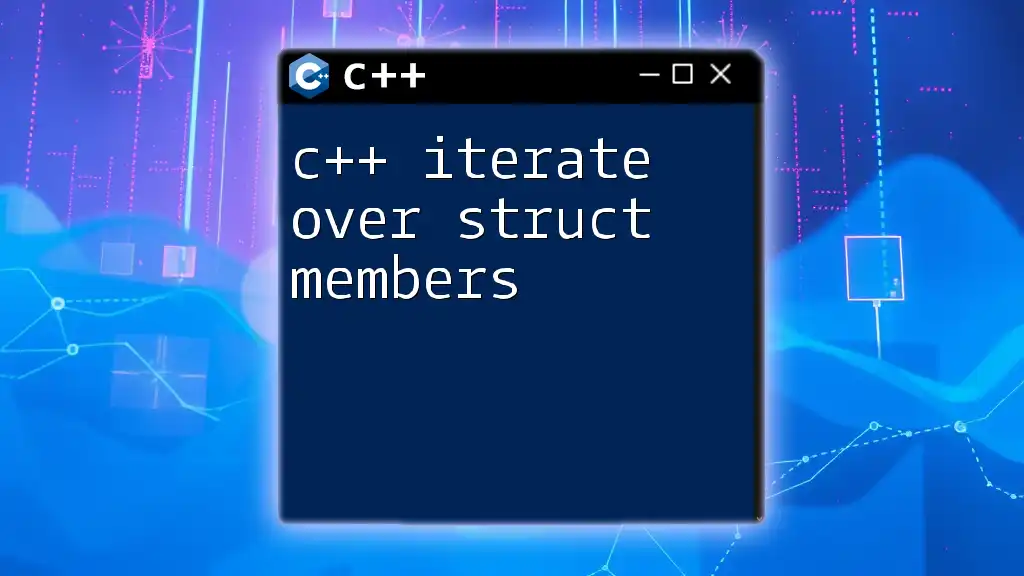
Additional Resources
For further exploration, consider checking the official C++ documentation and various programming tutorials to deepen your understanding of strings and their manipulation in C++.
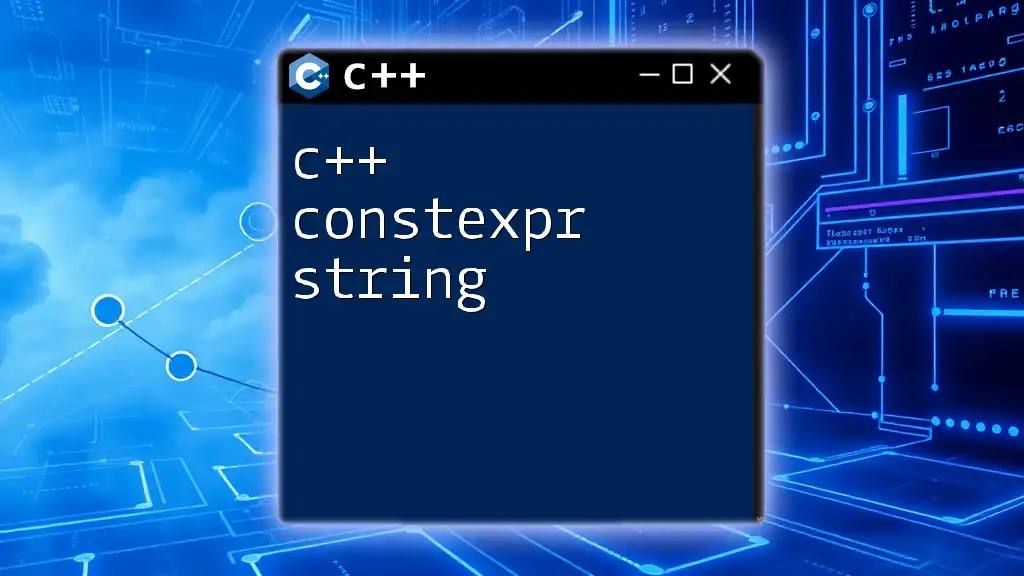
Call to Action
We encourage you to share your experiences or questions regarding string iteration in C++. Join our community and subscribe for more programming tips and tutorials focused on C++ and beyond!