To iterate through an `unordered_map` in C++, you can use a range-based for loop to access each key-value pair efficiently. Here's a code snippet demonstrating this:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> myMap = {{"one", 1}, {"two", 2}, {"three", 3}};
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
What is an unordered_map?
An unordered_map is a part of the C++ Standard Library that stores key-value pairs. Unlike a `std::map`, which maintains elements in a sorted order, `unordered_map` organizes elements using hash tables. This means that the data elements are stored in an arbitrary order, optimizing performance for lookup operations.
Why Use unordered_map?
The primary advantages of using `unordered_map` are its average constant time complexity for search, insert, and delete operations. This efficiency makes it ideal for scenarios where quick data retrieval is essential. Common use cases include:
- Implementing caches.
- Counting frequency of elements (such as words in a text).
- Building look-up tables for algorithms.
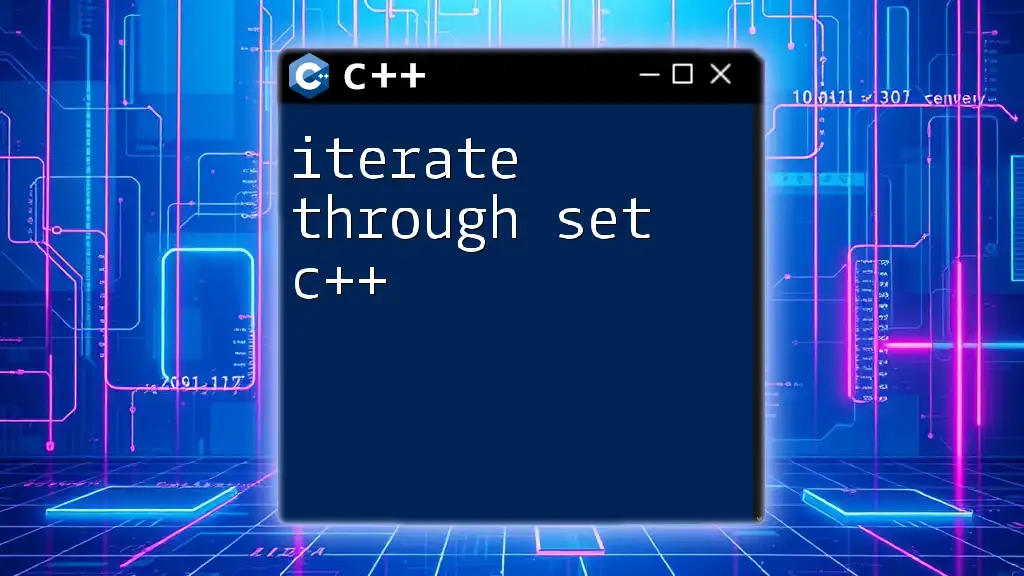
Setting Up Your C++ Environment
Installing Necessary Tools
To get started with C++, you should install an Integrated Development Environment (IDE) or a text editor with a C++ compiler. Some popular choices include Visual Studio, Code::Blocks, and CLion. Ensure that your environment supports C++11 or later for the best compatibility.
Including Necessary Libraries
To begin using `unordered_map`, include the relevant header file in your code:
#include <iostream>
#include <unordered_map>
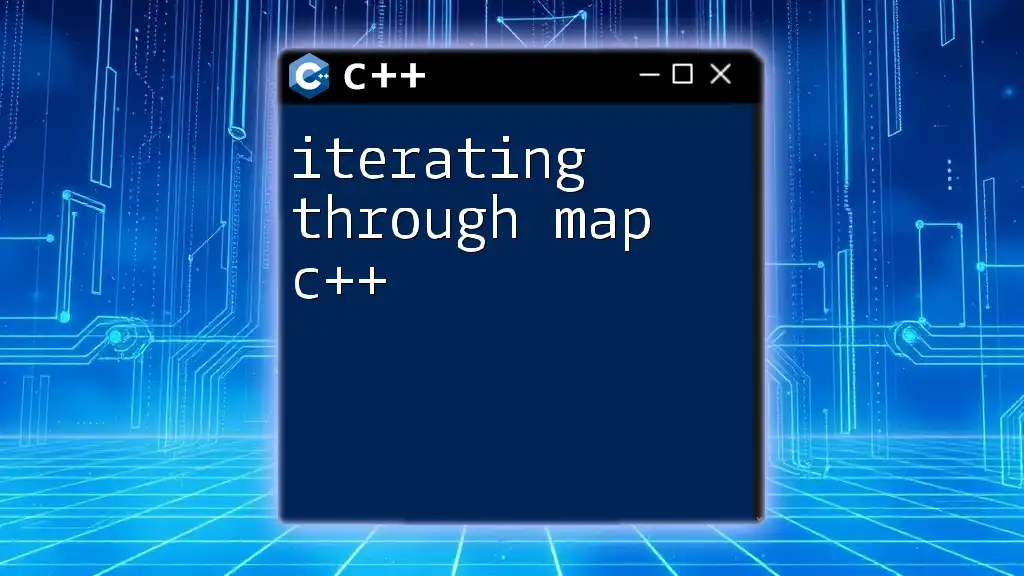
Understanding the Structure of unordered_map
Key-Value Pairs
In `unordered_map`, each element consists of a key and a value. The key is unique, while values can be duplicated. The functionality of `unordered_map` is heavily dependent on the underlying hash function that maps the keys to their respective buckets.
Default Allocator and Bucket Count
The default allocator in `unordered_map` allows for dynamic memory management. Bucket count is the number of initial storage locations for the key-value pairs, which can affect performance. Optimizing bucket count is vital for minimizing collisions and improving access speeds.
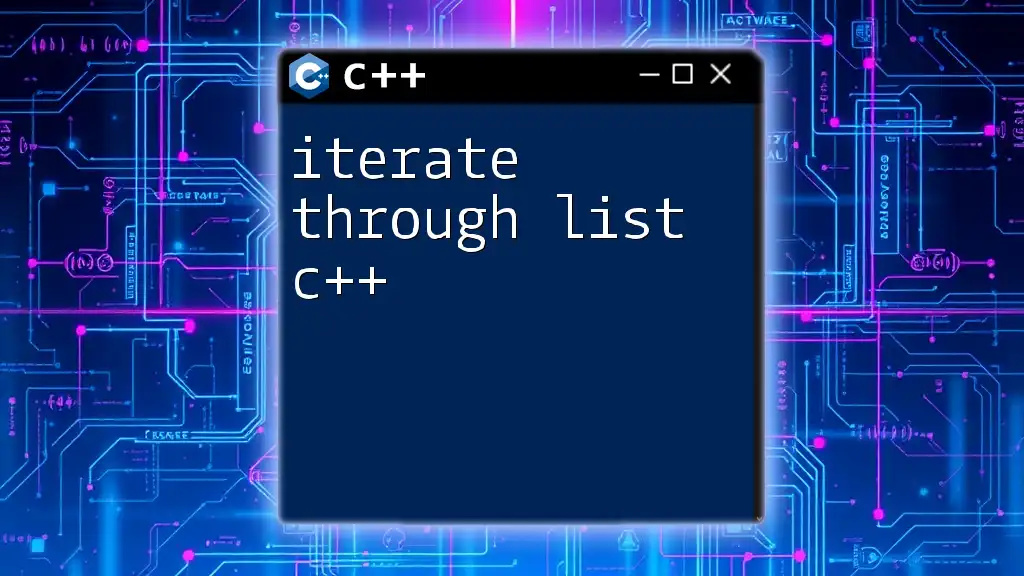
Basic Operations on unordered_map
Insertion of Elements
You can easily insert elements into an `unordered_map` using the following syntax:
std::unordered_map<int, std::string> myMap;
myMap[1] = "Apple";
myMap[2] = "Banana";
Accessing Elements
There are two primary ways to access elements in `unordered_map`. You can either use the subscript operator or the `find` method.
Using the subscript operator:
std::cout << myMap[1]; // Outputs: Apple
Using `find` is safer as it allows you to check if the element exists:
auto it = myMap.find(1);
if (it != myMap.end()) {
std::cout << it->second; // Outputs: Apple
}
Removal of Elements
To delete elements from an `unordered_map`, utilize the `erase` method:
myMap.erase(1); // Removes the element with key '1'
Searching for Elements
Searching can be more efficient using the `find` method, which returns an iterator. If the key exists, the iterator points to its location; otherwise, it points to `end()`:
auto it = myMap.find(1);
if (it != myMap.end()) {
// Element found
}
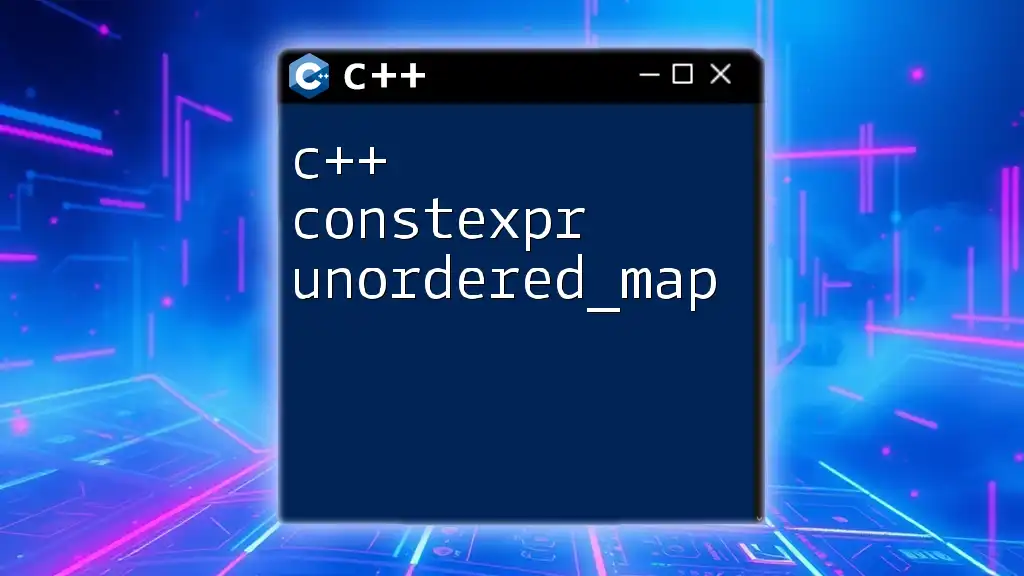
Iterating Through unordered_map
Why and When to Iterate?
Iterating through an `unordered_map` is essential for accessing all key-value pairs to perform operations like printing elements, transforming data, or performing calculations based on the data.
Iteration Methods
Using Range-Based For Loop
The range-based for loop is the simplest and most readable way to iterate through an `unordered_map`. Here's how it works:
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
This method automatically handles the underlying iterator and provides easy access to key-value pairs.
Using Iterators
Iterators provide more control during iteration, allowing you to manipulate the iteration process if needed. Here's an iteration example using iterators:
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
This approach gives explicit access to the iterator and lets you perform conditional checks or complex logic within the loop.
Using for_each with Lambda Functions
C++11 introduced lambda functions, providing a modern way to iterate through collections. You can use `std::for_each` along with a lambda function for clear and concise iteration:
std::for_each(myMap.begin(), myMap.end(), [](const auto& pair) {
std::cout << pair.first << ": " << pair.second << std::endl;
});
This method enhances readability, especially when applying transformations or filtering.
Performance Considerations
When iterating through `unordered_map`, be aware that performance can vary based on the underlying implementation and bucket count. A well-chosen bucket count minimizes collisions and potentially speeds up access times.
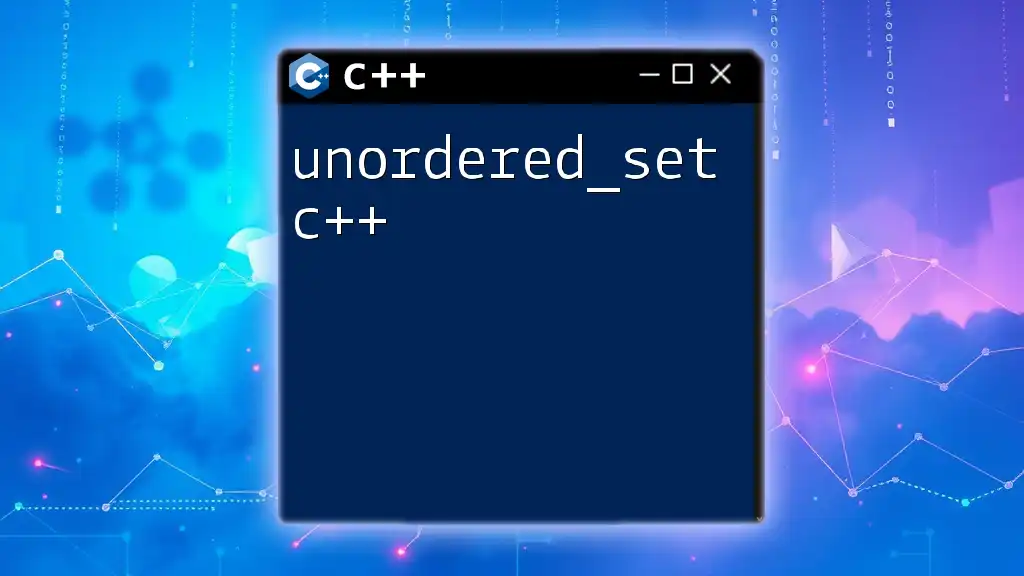
Common Pitfalls and Best Practices
Avoiding Invalid Access
A common mistake when using `unordered_map` is attempting to access a key that does not exist, which can lead to unexpected behavior. Ensure to check for existence before access. Using the `find` method is a great way to avoid this issue:
if (myMap.find(1) != myMap.end()) {
std::cout << myMap[1];
}
Custom Hash Function
In scenarios with complex key types or specific performance requirements, implementing a custom hash function can be beneficial. It allows for more effective distribution of elements across buckets. Here’s a basic example of a custom hash function:
struct CustomHash {
size_t operator()(const std::string& key) const {
return std::hash<std::string>()(key);
}
};
You can then use this custom hash function when declaring your `unordered_map`.
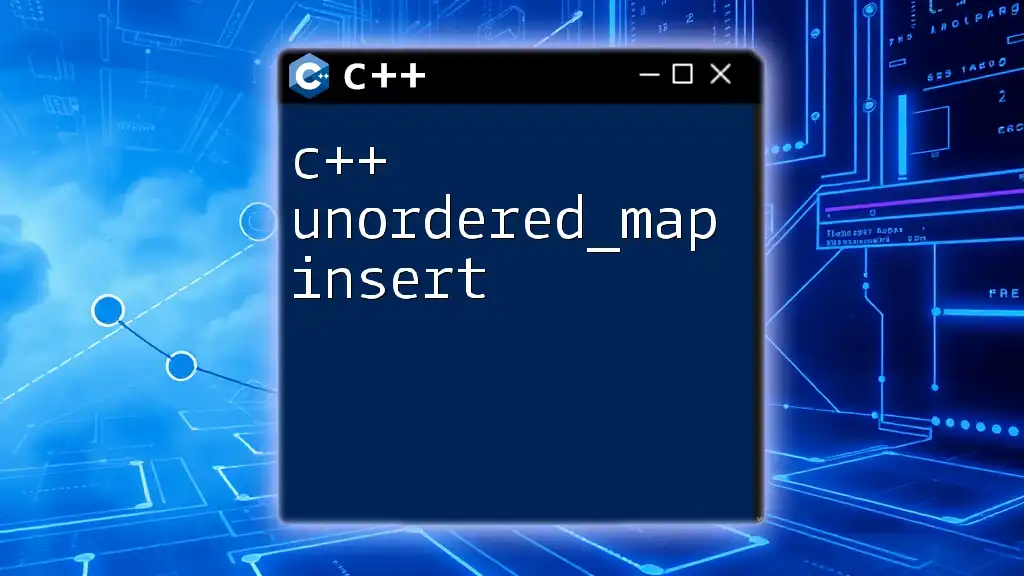
Conclusion
Mastering how to iterate through unordered_map c++ is crucial for leveraging its capabilities effectively. From understanding basic operations to exploring advanced iteration methods, mastering this topic will enable you to write more efficient and powerful C++ code.
As you explore further, practice implementing these concepts to solidify your understanding. Don't hesitate to seek additional resources and engage with the programming community for continuous learning.
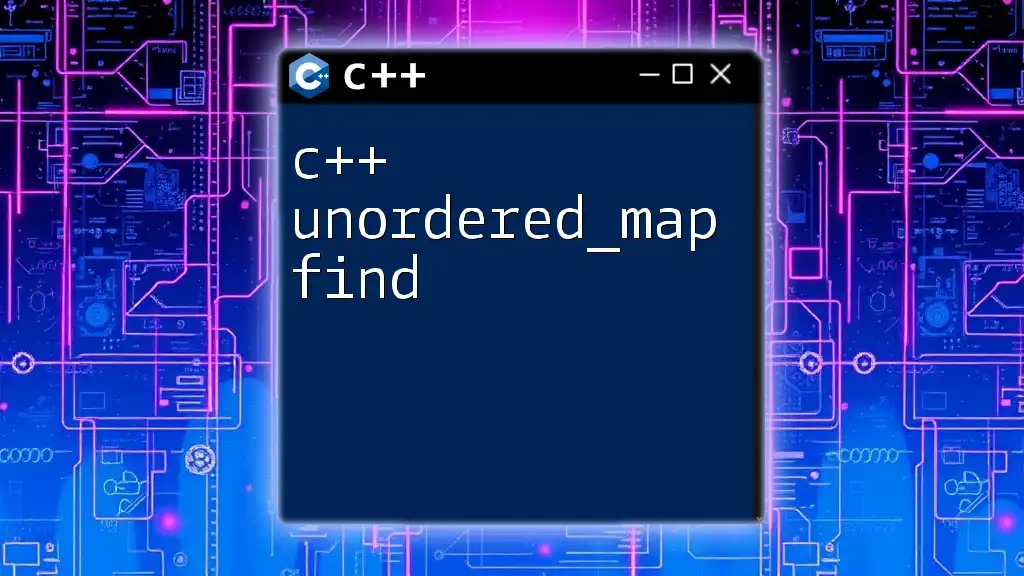
Call to Action
We encourage you to try out these examples on your own system. Experiment with different methods of iteration, and share your findings with the community. Your experiences can guide others on their journey with C++.