To convert a binary number to decimal in C++, you can use the following simple code snippet:
#include <iostream>
#include <cmath>
using namespace std;
int binaryToDecimal(int binary) {
int decimal = 0, base = 1;
while (binary > 0) {
int lastDigit = binary % 10;
binary = binary / 10;
decimal += lastDigit * base;
base = base * 2;
}
return decimal;
}
int main() {
int binaryNumber = 1011; // Example binary number
cout << "Decimal of " << binaryNumber << " is " << binaryToDecimal(binaryNumber) << endl;
return 0;
}
Understanding Binary Number System
What is a Binary Number?
Binary is a base-2 numeral system that consists of only two digits: 0 and 1. This system is fundamental to computer technology because digital devices operate on binary logic. In contrast, the decimal system, which we commonly use, is base-10 and consists of ten digits ranging from 0 to 9.
Why Convert Binary to Decimal?
Understanding the process of converting binary to decimal is crucial in programming and computer science. It helps in data representation, manipulation, and algorithm design. For instance, network addresses and hardware interfaces often rely on binary values, and converting them to a decimal format makes interpretation easier for developers.
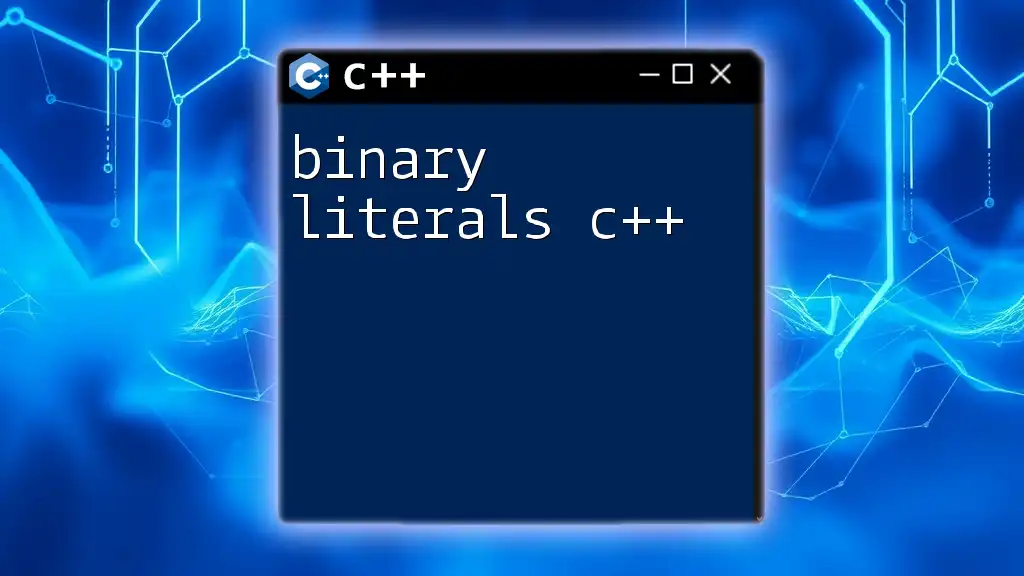
Decimal Number System Overview
The Decimal System Explained
The decimal system operates on ten digits and offers an intuitive way for humans to perform arithmetic. Each digit's place value increases by a factor of ten as you move from right to left. This base-10 system is used in everyday calculations and makes up the numeric foundation for most modern calculations.
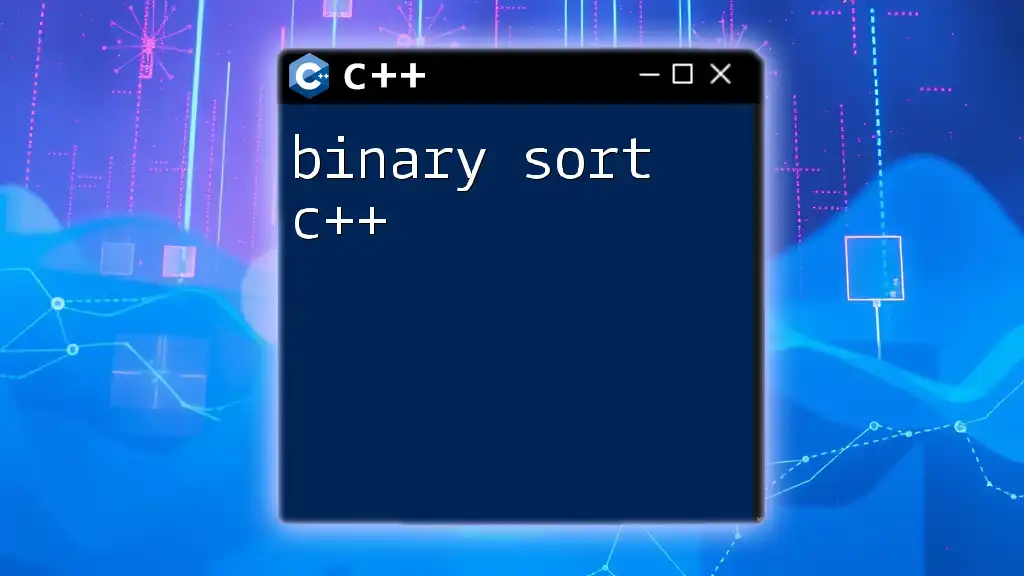
The Conversion Process
How Binary to Decimal Conversion Works
The conversion from binary to decimal involves calculating the sum of the products of each binary digit and its respective positional value. The formula can be represented as:
\[ \text{Decimal} = b_n \times 2^n + b_{n-1} \times 2^{n-1} + \cdots + b_0 \times 2^0 \]
where \( b \) represents each binary digit and \( n \) is its position from the right, starting from 0.
Example Breakdown of Binary to Decimal
Converting Binary 1011 to Decimal
Let’s take the binary number 1011 and break down the conversion process:
-
Step 1: Identify each digit:
- From right to left: \( 1, 1, 0, 1 \).
-
Step 2: Calculate positional values:
- \( 1 \times 2^3 = 8 \)
- \( 0 \times 2^2 = 0 \)
- \( 1 \times 2^1 = 2 \)
- \( 1 \times 2^0 = 1 \)
-
Step 3: Sum the products:
- \( 8 + 0 + 2 + 1 = 11 \)
Thus, the binary number 1011 converts to the decimal number 11.
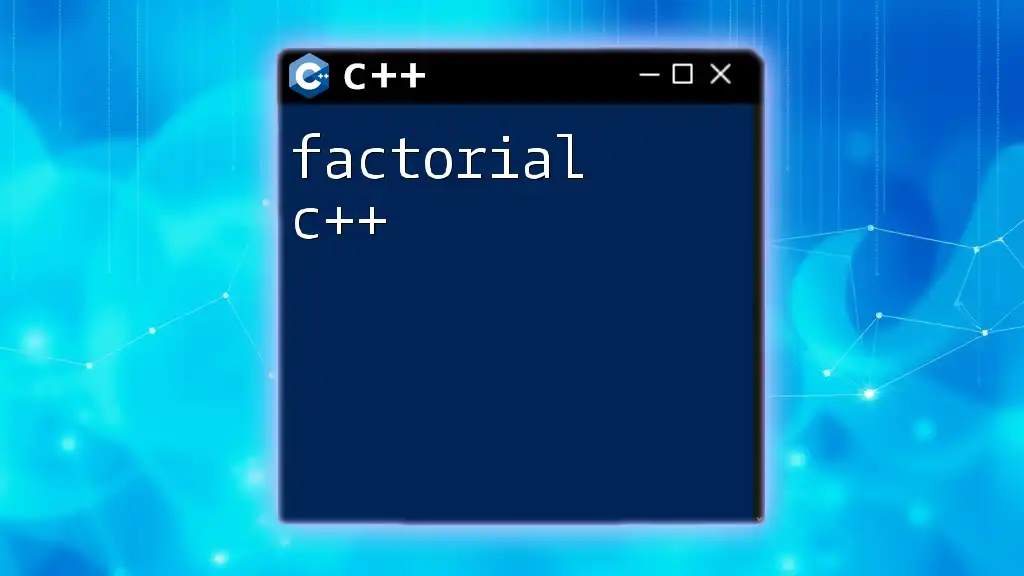
Implementing Binary to Decimal Conversion in C++
Standard Method to Convert Binary to Decimal
To convert binary numbers to decimal in C++, you can utilize a straightforward function. Here’s an example implementation:
int binaryToDecimal(int binaryNumber) {
int decimalNumber = 0, base = 1, lastDigit;
while (binaryNumber > 0) {
lastDigit = binaryNumber % 10;
binaryNumber = binaryNumber / 10;
decimalNumber += lastDigit * base;
base = base * 2;
}
return decimalNumber;
}
Detailed Explanation of the Code
- Initialization: The function starts by initializing `decimalNumber` to 0, `base` to 1, and `lastDigit** to hold the value of the current binary digit.
- Looping through Binary Digits: Within the `while` loop, as long as `binaryNumber` remains greater than zero:
- The last digit is isolated using the modulus operation.
- The binary number is then reduced by dividing it by 10 (dropping the last digit).
- The `decimalNumber` is updated by adding the product of the `lastDigit` and the current `base`.
- The `base` is subsequently multiplied by 2 for the next positional value.
Input and Output Example
To see this function in action, you can add a main function to test the conversion:
#include <iostream>
using namespace std;
// Function definition here
int main() {
int binaryInput = 1011;
cout << "Decimal of " << binaryInput << " is " << binaryToDecimal(binaryInput) << endl;
return 0;
}
When run, this program will output:
Decimal of 1011 is 11
This result confirms the conversion we performed earlier.
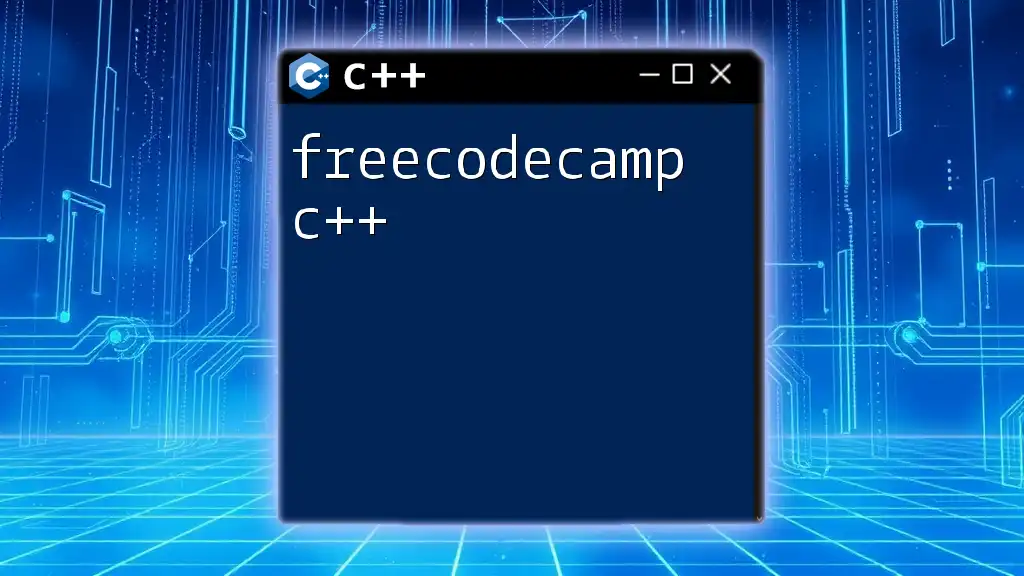
Alternative Methods for Conversion
Using C++ Standard Library Functions
For a more streamlined approach, you can utilize the C++ Standard Library. The `std::stoi` function can help convert binary strings directly:
int binaryToDecimalString(string binary) {
return stoi(binary, nullptr, 2);
}
This method is particularly useful when you receive binary input as a string rather than an integer.
Recursive Approach to Binary to Decimal Conversion
For those who favor recursion, here’s a recursive function to carry out the conversion:
int binaryToDecimalRecursive(int binary, int power = 0) {
if (binary == 0)
return 0;
return (binary % 10 * pow(2, power)) + binaryToDecimalRecursive(binary / 10, power + 1);
}
Step-by-step Explanation of Recursion
- The function checks if the binary value has reached 0; if so, it returns 0.
- For each call, it calculates the current digit's contribution by applying the power of two based on its position (given by `power`), and then recursively calls itself with the binary number divided by 10.
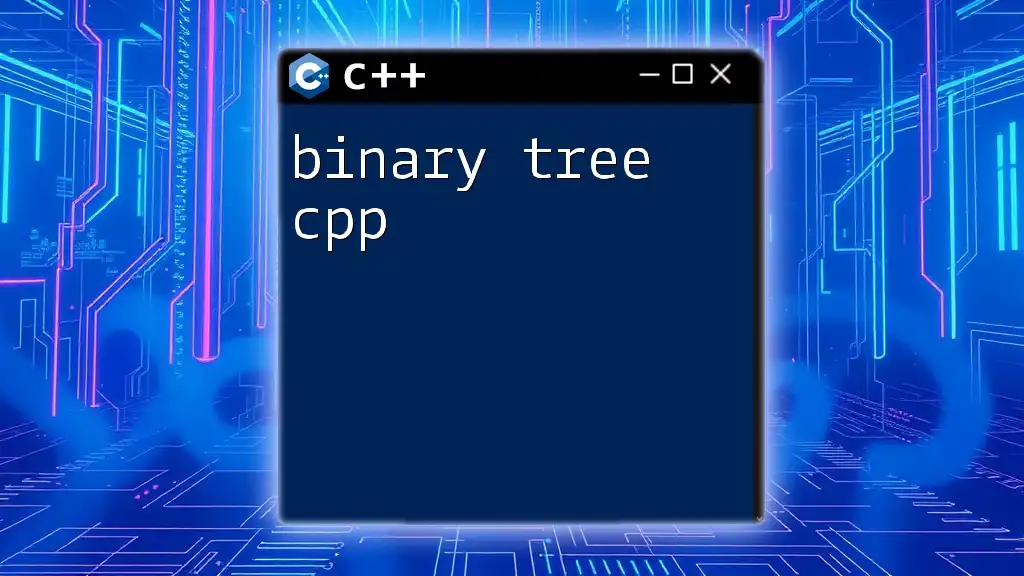
Common Errors and Debugging Tips
Handling Invalid Input
When performing binary to decimal conversions, it's crucial to ensure the input is valid binary (only contains 0s and 1s). Invalid inputs may lead to incorrect calculations or runtime errors.
Debugging Common Issues
- Ensure that the modulus and division operations are appropriately applied to extract binary digits.
- Utilize debugging tools available in most IDEs to step through your code and verify calculations at each stage.
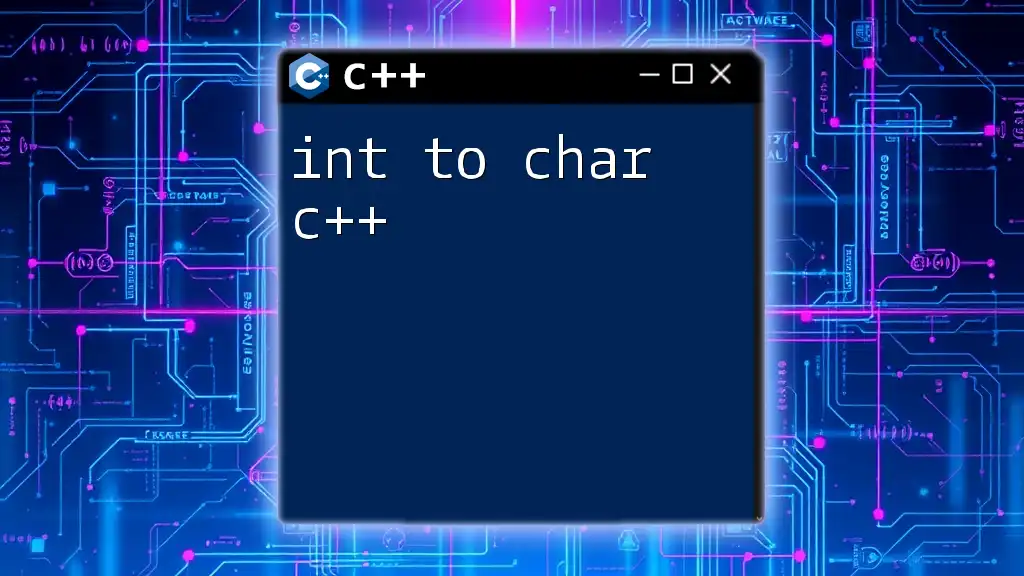
Real-world Applications of Binary to Decimal Conversion
User Input and Data Processing
Binary to decimal conversion is often encountered in various application scenarios. For example, user input can be used to gather binary values, which must then be converted for processing and representation.
Networking and Computer Systems
In networking, IP addresses are often represented in binary. Understanding how to convert these values to decimal not only aids in comprehension but is also vital in network configuration tasks.
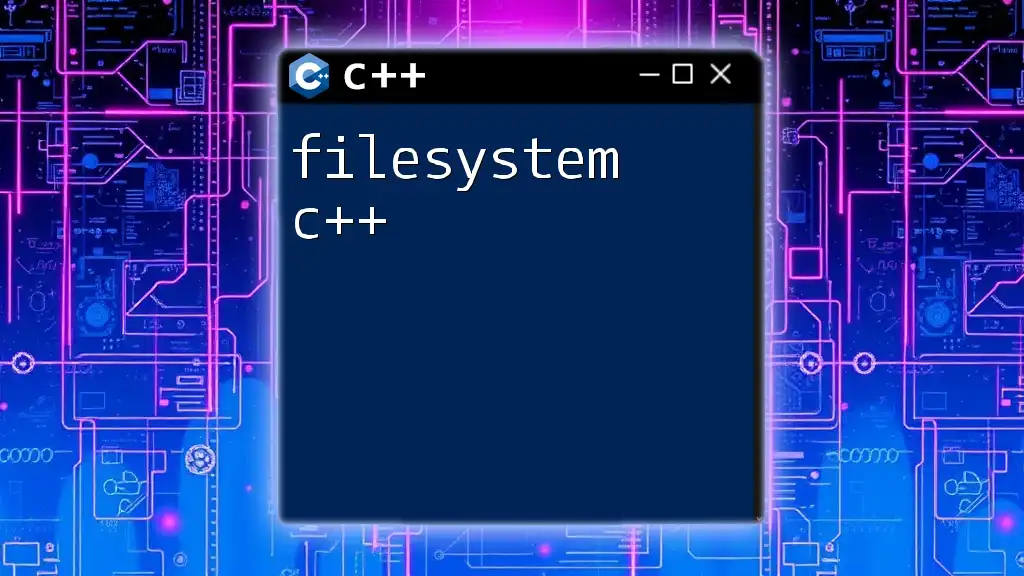
Conclusion
In this guide, we've explored the process of converting binary to decimal in C++ thoroughly. From understanding the underlying principles of binary numbers to implementing multiple methods for conversion, you now have the tools to handle this essential programming task efficiently.
Encouraging Further Learning
For further exploration, you might want to delve into related topics like converting decimal back to binary or understanding the nuances of other numeral systems. The world of programming is vast, and mastery of these concepts lays a strong foundation for future endeavors.
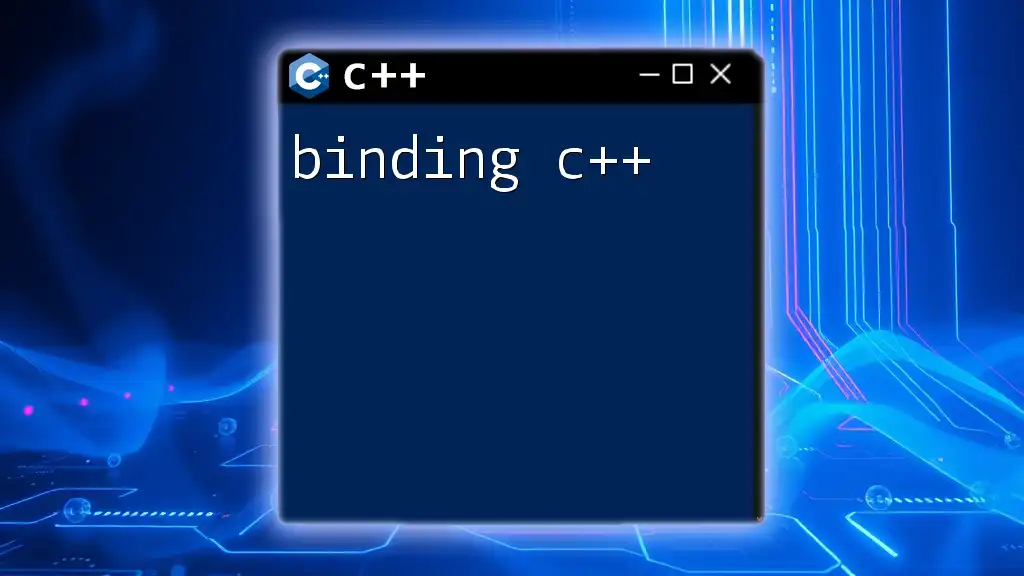
FAQs
What is the difference between binary and decimal?
The primary difference lies in the base: binary is base-2 (using only digits 0 and 1), while decimal is base-10 (using digits 0 to 9).
Can you convert a decimal number back to binary?
Yes! You can convert decimal numbers to binary using techniques such as repeated division by 2 and tracking remainders.
What is the maximum binary number that can be converted to decimal in C++?
The maximum binary number depends on the integer limits defined by the C++ data types in use. For standard integers, this is often up to 31 bits for positive numbers, depending on the implementation.