A search binary tree (or binary search tree) in C++ is a data structure that maintains sorted data in a hierarchical order, allowing for efficient search, insertion, and deletion operations.
Here's a simple code snippet demonstrating the insertion and search operations in a binary search tree:
#include <iostream>
using namespace std;
struct Node {
int value;
Node* left;
Node* right;
};
Node* insert(Node* root, int value) {
if (!root) {
Node* newNode = new Node{value, nullptr, nullptr};
return newNode;
}
if (value < root->value) {
root->left = insert(root->left, value);
} else {
root->right = insert(root->right, value);
}
return root;
}
bool search(Node* root, int value) {
if (!root) return false;
if (root->value == value) return true;
return value < root->value ? search(root->left, value) : search(root->right, value);
}
int main() {
Node* root = nullptr;
root = insert(root, 5);
insert(root, 3);
insert(root, 7);
cout << (search(root, 3) ? "Found" : "Not Found") << endl;
return 0;
}
What is a Binary Tree?
A binary tree is a hierarchical structure that consists of nodes, where each node has at most two children referred to as the left child and the right child. This structure provides a foundation for various data structures and algorithms, facilitating efficient data organization and retrieval.
In a binary tree:
- Each element is called a node.
- There’s a distinction between the root (the top node) and the leaves (nodes with no children).
- The height of a tree signifies the longest path from the root to a leaf.
Binary trees are instrumental in numerous applications, including serving as organizational structures for databases and process management in operating systems.

Types of Binary Trees
Full Binary Tree
A full binary tree is characterized by every node having either zero or two children. This structure ensures that none of the nodes has a single child.
Complete Binary Tree
In a complete binary tree, all levels, except possibly the last one, are fully filled, and all nodes are as far left as possible. This characteristic makes complete binary trees effective for certain algorithms and data structures, including heaps.
Balanced Binary Tree
A balanced binary tree maintains a height difference between the left and right subtrees of any node no greater than one. This balance guarantees efficient operations for insertions, deletions, and lookups, which can otherwise degrade in performance for unbalanced trees.
Binary Search Tree (BST)
A Binary Search Tree is defined by the property that for any given node:
- All values in its left subtree are less than the node’s value.
- All values in its right subtree are greater than the node’s value.
This property enables efficient searching, insertion, and deletion operations.

Understanding Binary Search Trees (BST)
What is a Binary Search Tree?
A Binary Search Tree not only provides a structured way to store data but also ensures that operations involving data retrieval are optimized. The underlying property of a BST allows it to maintain a sorted order, making it a vital choice for various applications in programming.
Advantages of Using a Binary Search Tree
Binary Search Trees offer significant benefits:
- Efficiency in Data Storage: Due to the organized structure, searching, inserting, and deleting elements can be performed quickly compared to other data structures like arrays and linked lists.
- Dynamic Data Handling: Rather than requiring resizing as arrays do, BSTs can grow or shrink in size based on the data inserted or deleted.

Searching in Binary Search Trees
The Search Operation
Definition of Search Operation
Searching a binary tree involves traversing its structure to locate a specific value. Efficient searching is essential, particularly with large datasets, as it can significantly affect performance.
How the Search Algorithm Works
The search process within a Binary Search Tree operates through a simple decision-making approach:
- Start from the root node.
- Compare the target value to the root’s value:
- If the target matches the root’s value, the search is successful.
- If the target is less than the root’s value, proceed to the left child.
- If the target is greater, move to the right child.
- Repeat the process recursively until the node is found or a leaf is reached—indicating the value is not present.
Time Complexity of Search Operation
The average time complexity for searching in a balanced Binary Search Tree is O(log n), where n is the number of nodes. However, in the worst case, such as in an unbalanced tree resembling a linked list, the time complexity can degrade to O(n).
Code Snippet: Basic Search Function
The following code illustrates a fundamental search function in C++ for a Binary Search Tree:
struct Node {
int data;
Node* left;
Node* right;
};
Node* searchBST(Node* root, int key) {
if (root == nullptr || root->data == key)
return root;
if (key < root->data)
return searchBST(root->left, key);
return searchBST(root->right, key);
}
Explanation of the code:
- The `Node` structure defines a binary tree node containing data and pointers to the left and right children.
- The `searchBST` function performs a recursive search: it returns the node if the key matches or navigates left or right based on the value comparison.
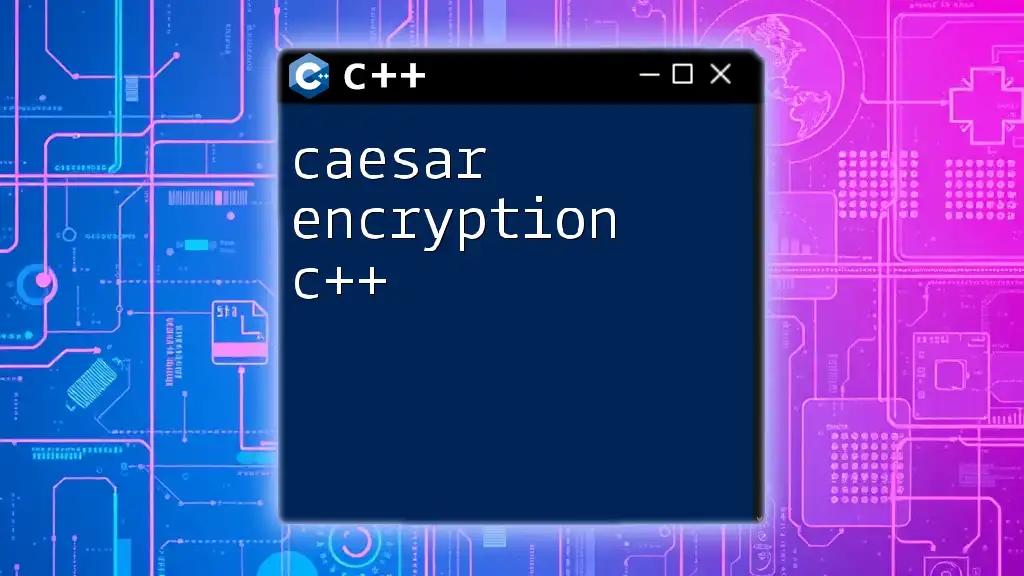
Example: Searching in a Binary Search Tree
Setting Up the Binary Search Tree
Before performing a search operation, we need to create a Binary Search Tree. The following code demonstrates how to insert nodes:
Node* insertNode(Node* root, int data) {
if (root == nullptr) {
return new Node{data, nullptr, nullptr};
}
if (data < root->data) {
root->left = insertNode(root->left, data);
} else {
root->right = insertNode(root->right, data);
}
return root;
}
Node* root = nullptr;
root = insertNode(root, 10);
root = insertNode(root, 5);
root = insertNode(root, 15);
// Add more nodes for a larger tree...
This structure begins with a `nullptr` root, then inserts elements to build the tree, ensuring the proper placement of each node according to the Binary Search Tree property.
Running the Search
The following example showcases how to use the search function defined earlier:
int main() {
// Previous code to create BST...
Node* result = searchBST(root, 15);
if (result) {
std::cout << "Found: " << result->data << std::endl;
} else {
std::cout << "Not Found!" << std::endl;
}
return 0;
}
By employing the searchBST function, the program searches for the value 15. If found, it returns the corresponding node; otherwise, it indicates that the value is not present.
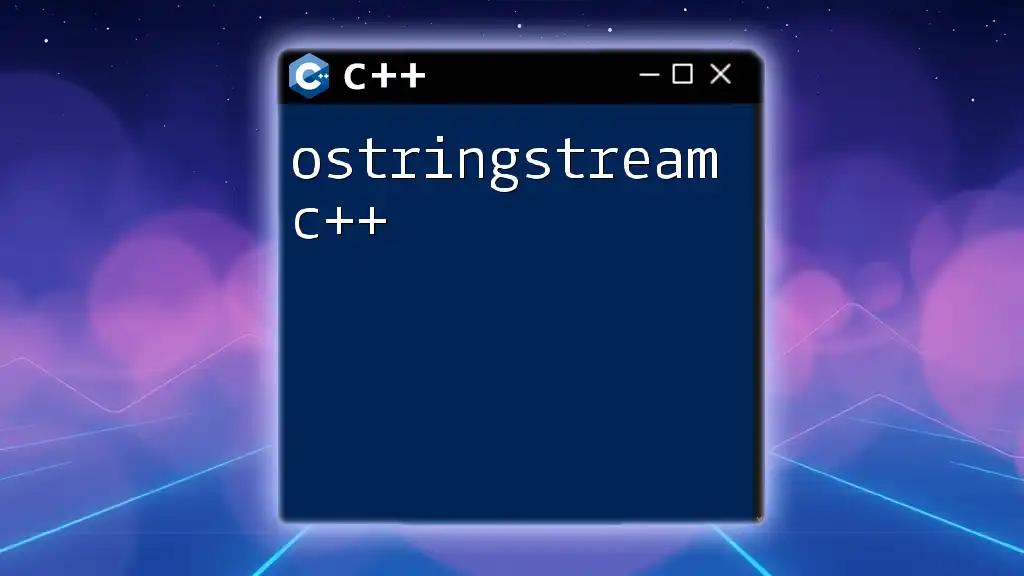
Common Issues and Troubleshooting
Search Failures
Failures during search operations can occur for various reasons, including:
- Incorrect implementation of the Binary Search Tree properties.
- Insertion of duplicate values which may lead to ambiguity in retrieval.
Debugging and Testing Your Search Algorithm
To troubleshoot the search functionality:
- Validate the structural integrity of the tree after each insertion.
- Use unit testing to ensure that the algorithm works as expected, both when searching for existing and non-existing values.
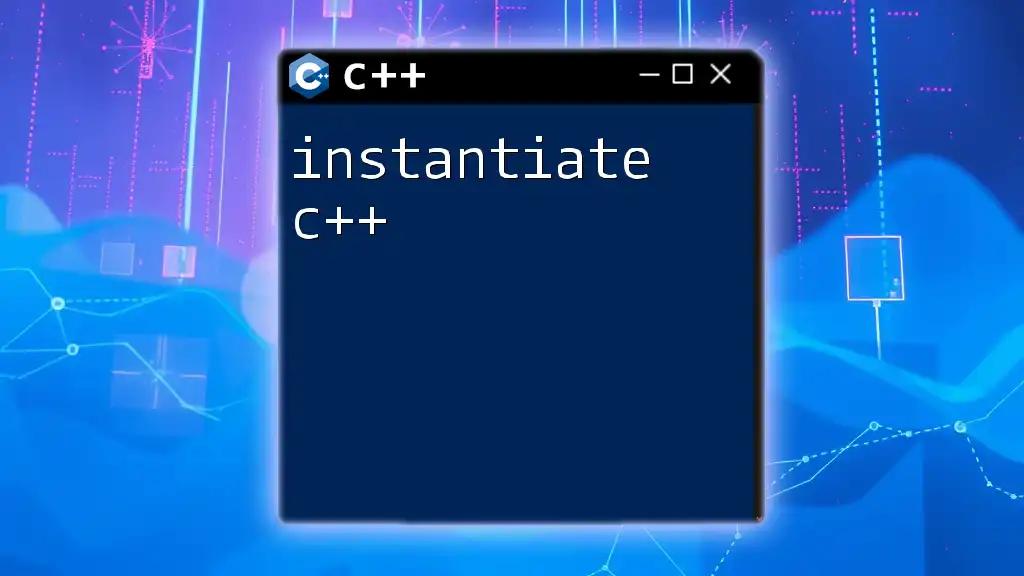
Conclusion
Recap of Key Points
Binary trees, particularly Binary Search Trees, are fundamental in programming. Their properties allow for efficient searching, making them invaluable in various applications. Mastering the search operation within a BST will significantly enhance your ability to manage data effectively.
Key Takeaways
Understanding and implementing the search operation in a binary tree using C++ is essential for anyone looking to deepen their knowledge in data structures. Practical examples, along with rigorous testing, will aid in solidifying these concepts.

Additional Resources
Recommended Books and Online Courses
Explore literature and courses focusing on data structures to deepen your understanding of binary trees and other essential concepts in C++ programming.
Relevant C++ Libraries
Consider utilizing libraries like STL (Standard Template Library) to explore higher-level implementations of binary trees and their search functionalities. This can provide a broader perspective and enrich your coding practices.