In C++, you can read a binary file by using an `ifstream` object with the `ios::binary` flag, allowing you to extract data in a manner similar to how it is stored in the file.
Here's a simple code snippet demonstrating how to read a binary file:
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("data.bin", std::ios::binary);
if (file) {
int data;
while (file.read(reinterpret_cast<char*>(&data), sizeof(data))) {
std::cout << data << std::endl;
}
file.close();
} else {
std::cerr << "Error opening file." << std::endl;
}
return 0;
}
Understanding Binary Files
What is a Binary File?
A binary file is a type of file that contains data in a format that is not intended to be human-readable. Instead of storing data as sequences of characters like text files, binary files store data in bits and bytes, which can represent numerical values, images, audio, and other complex data types. The primary characteristic of binary files is their efficiency: they can store large amounts of data compactly.
Why Use Binary Files?
Binary files are particularly advantageous for various reasons:
- Efficiency: Binary files can represent data in a more compact format compared to text files, leading to lower storage requirements.
- Speed: Reading and writing operations on binary files are generally faster than on text files, especially for large datasets.
- Data Integrity: Since binary formats maintain their structure without converting to text, there is less risk of data corruption during read/write operations.
Common use cases include saving images, audio files, serialized objects, or configurations that do not need to be human-readable.
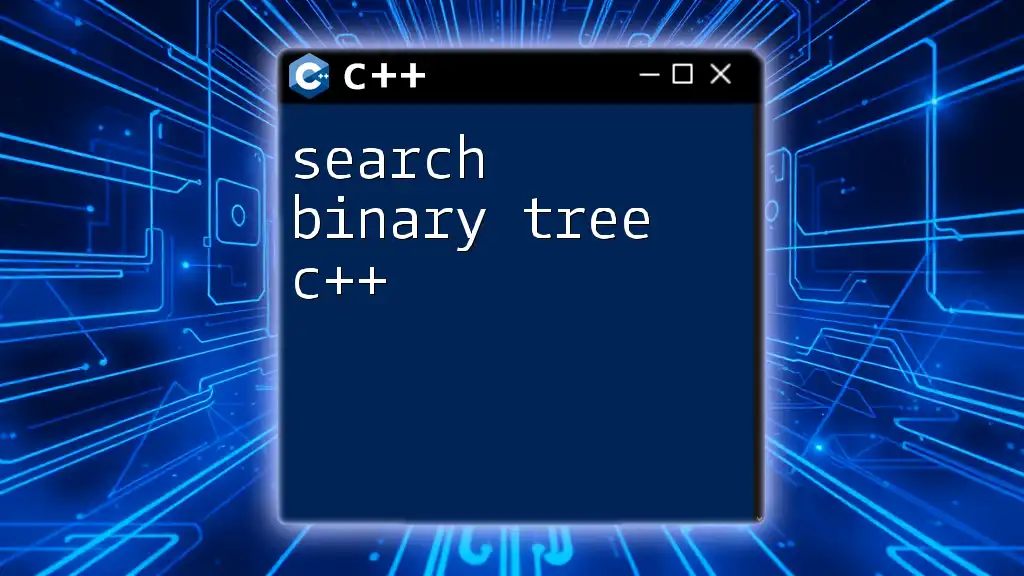
Setting Up the Environment for Reading a Binary File
Required Libraries
In C++, you will typically use the `<fstream>` library to handle file input and output operations. This library provides the necessary functions to work with binary files.
To include the fstream library, you can use the following directive at the top of your program file:
#include <fstream>
File Operations in C++
In C++, file handling is performed using stream classes. The two main classes are `ifstream` for reading from files and `ofstream` for writing to files.
Difference Between Binary and Text Mode
When handling files, it is crucial to open them in the correct mode. Binary files should be opened using the binary mode flag (`std::ios::binary`). This ensures that the data is read and written in its raw format.
To open a file in binary mode, you can use the following syntax:
std::ifstream inputFile("filename.bin", std::ios::binary);
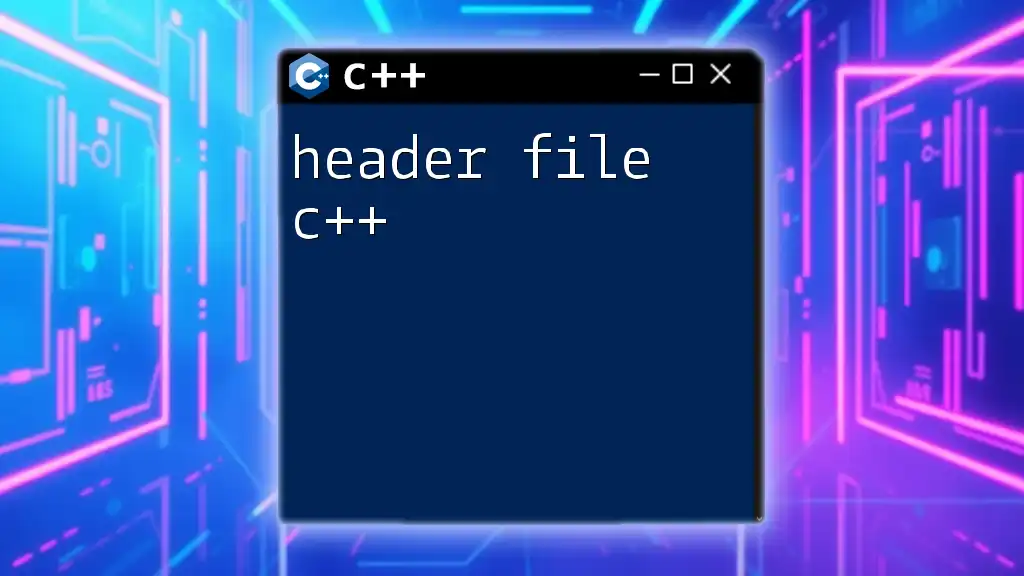
Opening a Binary File in C++
Syntax for Opening Files
The basic syntax for opening a binary file starts with creating an instance of `ifstream`:
std::ifstream inputFile("data.bin", std::ios::binary);
This line of code initializes an input file stream object named `inputFile` and attempts to open a file named "data.bin" in binary mode.
Error Handling When Opening Files
Always check if the file was successfully opened before proceeding with operations. This is vital in preventing runtime errors.
You can do this with the following code:
if (!inputFile.is_open()) {
std::cerr << "Error opening file!" << std::endl;
return 1; // or handle the error as needed
}
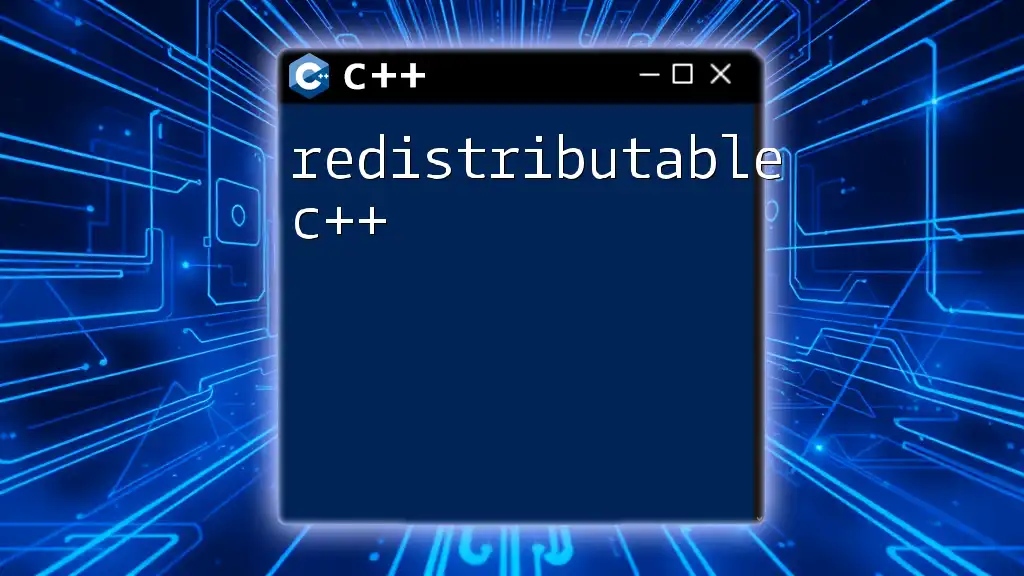
Reading Data from a Binary File
Basic Structure for Reading Binary Files
When you read from a binary file, you typically want to read data types such as integers, floats, or even user-defined structures. The structure of your code will generally follow this format:
- Open the file.
- Read the data into variables.
- Close the file.
Reading Data into Variables
Example: Reading Integers
To read integers from a binary file, the following example can be used:
int number;
inputFile.read(reinterpret_cast<char*>(&number), sizeof(number));
In this snippet, `reinterpret_cast<char*>(&number)` converts the address of the variable to a character pointer, which is required by the `read` method. This allows you to read raw bytes directly into the variable.
Example: Reading Custom Structures
You can also read custom data types using structures. Here’s how to define a struct and read it from a binary file:
struct Data {
int id;
float value;
};
Data data;
inputFile.read(reinterpret_cast<char*>(&data), sizeof(data));
This code reads a struct named `Data` directly from the binary file, filling the `id` and `value` fields with the corresponding data.
Using the `read()` Function
The `read()` function is a crucial method in C++ for reading data from binary files. It allows you to specify the type of data and the number of bytes you wish to read.
Example usage:
char buffer[256];
inputFile.read(buffer, sizeof(buffer));
This snippet reads up to 256 bytes into `buffer`, enabling you to handle data more directly when needed.
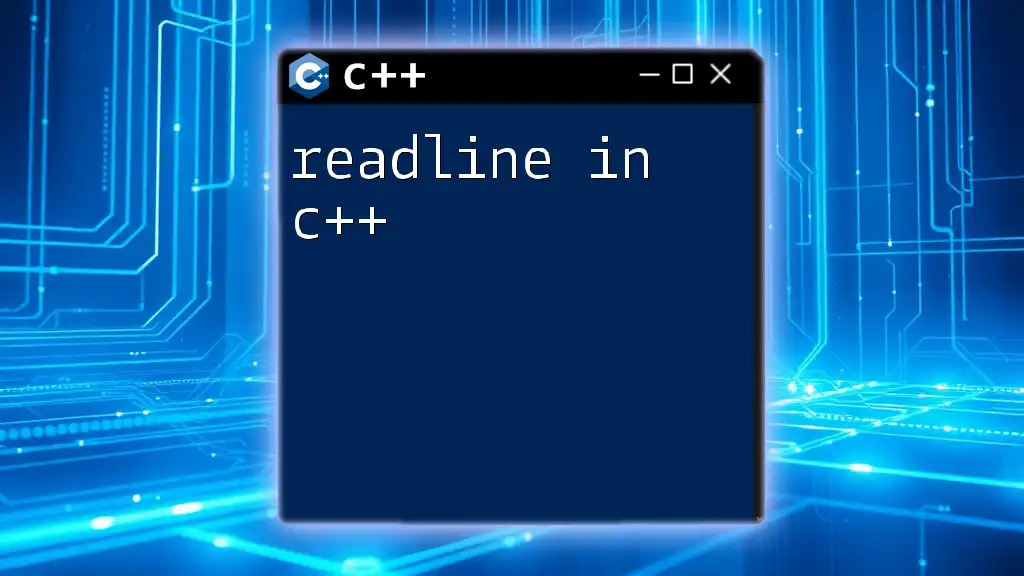
Practical Considerations
Buffers and Efficient Reading
Using buffers when reading large files can enhance performance. By reading a large block of data at once, you minimize the number of I/O operations, which can be slow.
Here’s a simple buffer example:
char buffer[1024];
while (inputFile.read(buffer, sizeof(buffer))) {
// Process the buffer
}
// Handle remaining bytes if any
if (inputFile.gcount() > 0) {
// Process the remaining bytes
}
Endianness in Binary Files
Endianness refers to the byte order used to represent multi-byte values. When reading or writing binary files, it’s essential to consider the target platform's endianness. Some systems use big-endian while others use little-endian representations.
To handle different byte orders, you may need to convert values after reading them, based on the architecture you are working with.
Reading and Validating File Sizes
Before reading data, it’s good practice to validate the file size to prevent reading beyond the available data. You can use the `seekg` and `tellg` methods to achieve this:
inputFile.seekg(0, std::ios::end); // Move to the end
std::streampos fileSize = inputFile.tellg(); // Get size
inputFile.seekg(0, std::ios::beg); // Return to beginning
This ensures you are aware of the total bytes available for reading.
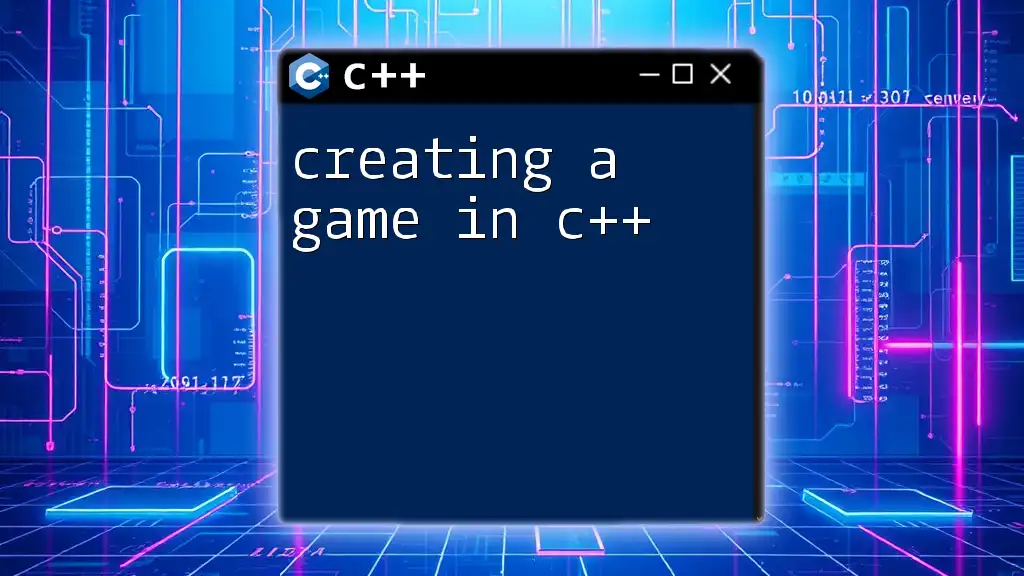
Common Use Cases for Reading Binary Files
Applications in Game Development
Binary files are widely used in game development for storing assets such as textures, models, and game state data. By reading data into memory efficiently, games can deliver smoother experiences and reduced load times.
Reading Configurations and Settings
Many applications utilize binary files to store user settings or configuration options. When reading these files, performance can be enhanced, and the integrity of the stored settings can be maintained.
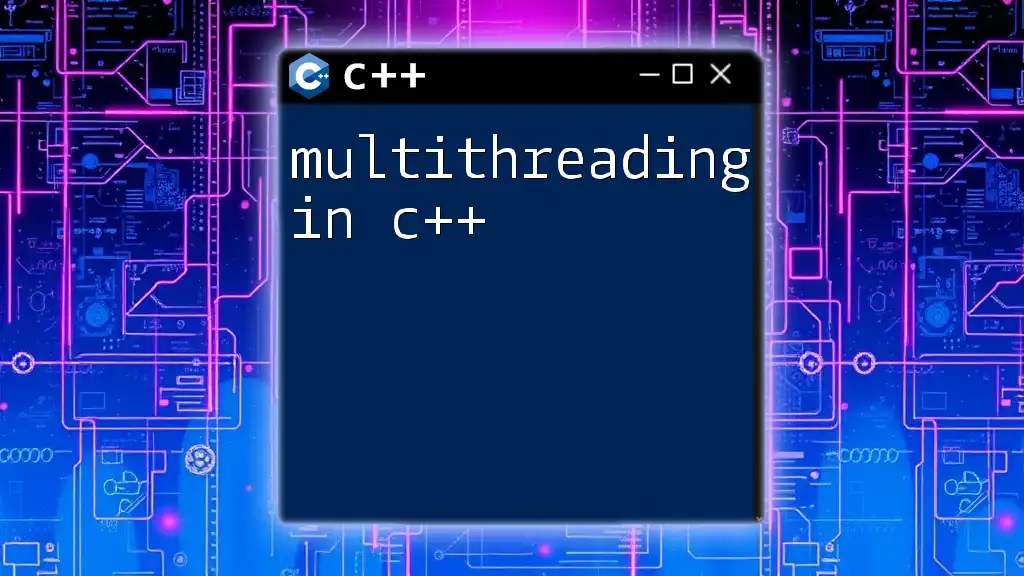
Troubleshooting Common Issues
Debugging File Read Errors
Common errors when reading binary files may include:
- File Not Found: Ensure the file path is correct.
- End-of-File Reached Early: Confirm the expected file size matches what is on disk.
- Data Type Mismatches: Check that the structure or data type size matches that of the written data.
Ensuring Compatibility Across Different Platforms
When dealing with binary files, switching between platforms can lead to issues. It is vital to ensure consistent data formats across different systems. Consider incorporating checks and conversions for data types if your application will run on multiple platforms.
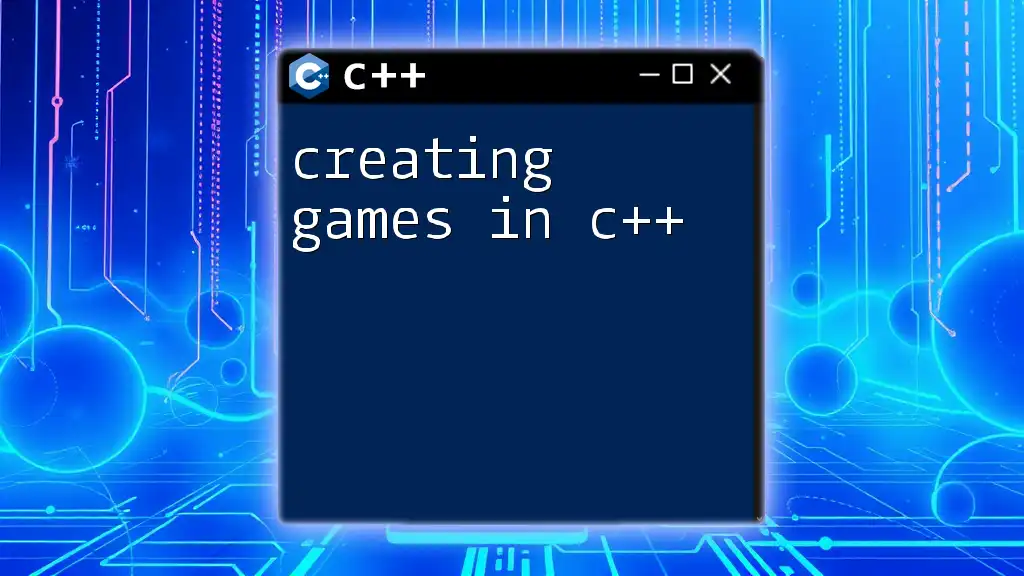
Conclusion
Reading binary files in C++ is an essential skill that involves understanding file operations, data structures, and error handling. With the proper techniques and practices outlined above, you can efficiently manage binary file I/O, leading to better performance and more robust applications. Practice with various examples will further enhance your understanding and capabilities in handling binary data.
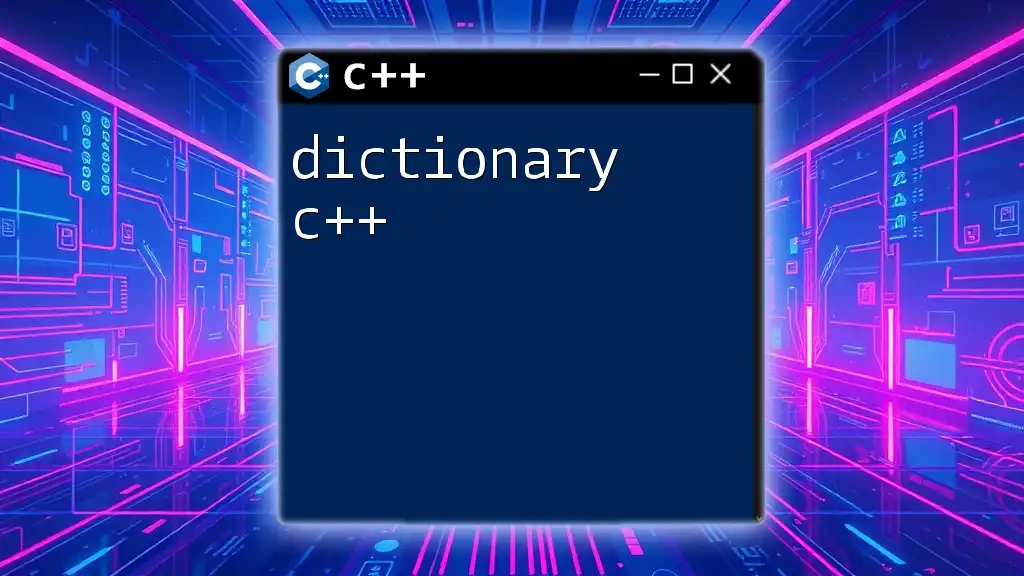
Additional Resources
To deepen your understanding further, consider exploring additional readings and tutorials that discuss advanced topics in file handling and I/O operations specific to C++.
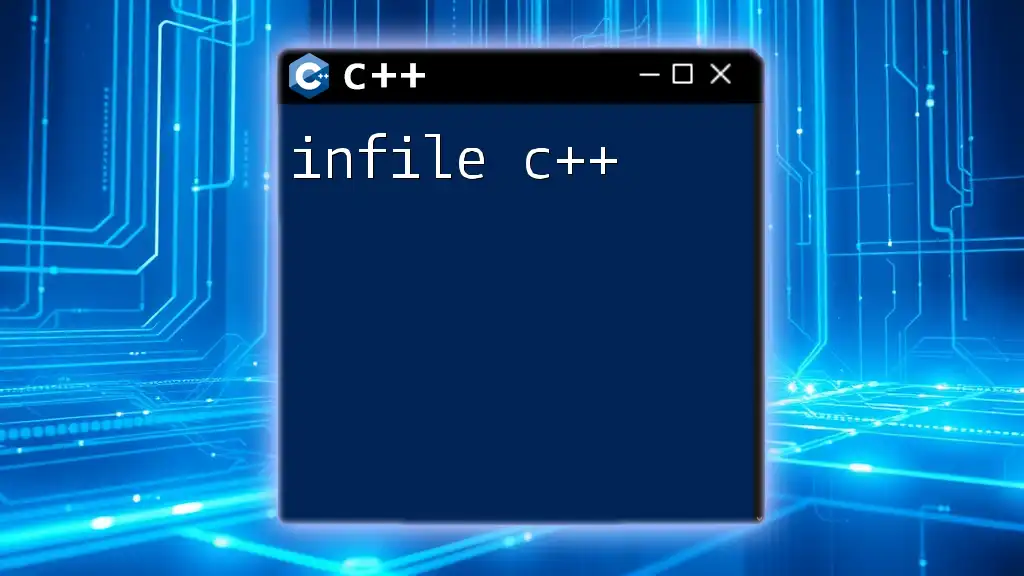
FAQs About Reading Binary Files in C++
If you have additional questions regarding binary file operations in C++, do not hesitate to seek out further clarification—understanding the nuances of binary file handling can greatly enhance your programming expertise. For those venturing into C++, becoming proficient in reading binary files is a valuable and rewarding endeavor.