Creating a simple game in C++ can be accomplished by utilizing basic structures and functions to handle user input and game logic, as demonstrated in the following snippet that illustrates a basic console-based guessing game.
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(std::time(0)); // Seed random number generator
int number = std::rand() % 100 + 1; // Generate random number between 1 and 100
int guess;
std::cout << "Guess a number between 1 and 100: ";
while (true) {
std::cin >> guess;
if (guess < number) {
std::cout << "Too low! Try again: ";
} else if (guess > number) {
std::cout << "Too high! Try again: ";
} else {
std::cout << "Congratulations! You guessed the number." << std::endl;
break;
}
}
return 0;
}
Setting Up Your Development Environment
Choosing an IDE
When embarking on the journey of creating a game in C++, the first step is to select a suitable Integrated Development Environment (IDE). Popular options include:
- Visual Studio: Known for its robust features and superb debugging tools. Ideal for Windows developers.
- Code::Blocks: A lightweight and flexible IDE that is cross-platform compatible.
- CLion: A powerful IDE from JetBrains with support for CMake, great for larger projects.
Each IDE has its own advantages and disadvantages. Choose based on factors like project size, collaboration needs, and personal preference.
Installing Required Libraries
To enhance your game's capabilities, you’ll need to use game libraries. Two highly recommended libraries are SFML (Simple and Fast Multimedia Library) and SDL (Simple DirectMedia Layer).
Step-by-step guide to installing SFML
-
On Windows:
- Download the SFML package from its official website.
- Unzip it and link the folders in your project settings.
- Make sure to adjust the Visual Studio properties to include the SFML directories.
-
On Linux:
- You can install SFML directly using a package manager. For instance:
sudo apt-get install libsfml-dev
This sets the stage for efficient multimedia handling in your game. Remember, you can also explore alternatives like SDL or Allegro, depending on your game's specific needs.
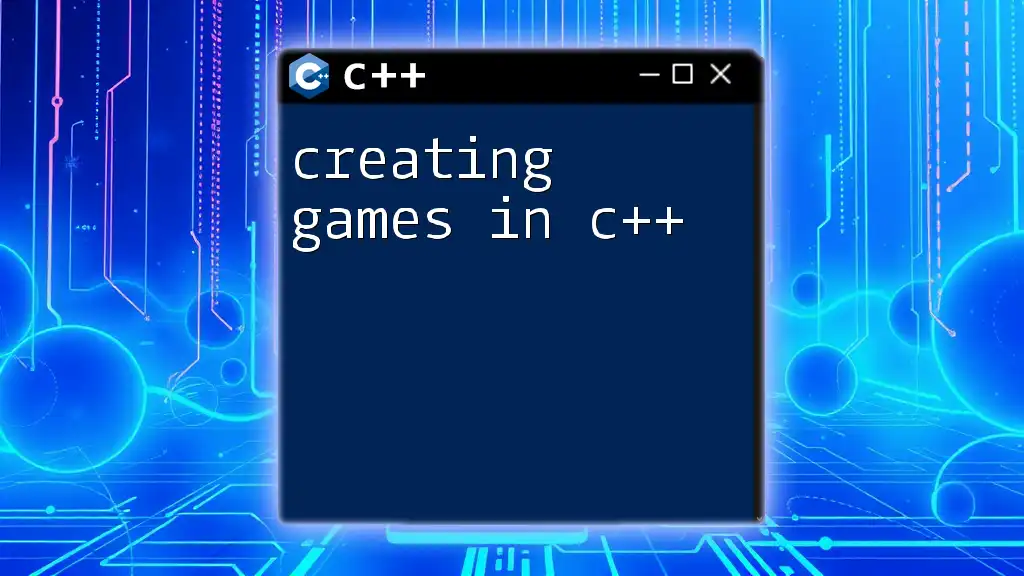
Core Concepts of Game Development
Game Loops
Every game has a core routine known as the game loop. This loop continuously runs while your game is active, processing inputs, updating game states, and rendering graphics. A basic structure of a game loop can look like this:
while (window.isOpen()) {
processEvents();
update();
render();
}
This structure ensures that your game remains responsive, smooth, and interactive.
Game State Management
Understanding how to manage different game states is crucial. Common states include Menu, Play, Pause, and Game Over. This can be handled using enums for clarity:
enum class GameState { Menu, Play, Pause, GameOver };
GameState currentState = GameState::Menu;
This allows for easier transitions and checks throughout the game cycle.
Rendering Graphics
When it comes to rendering graphics, one must understand the difference between 2D and 3D graphics. We will focus on 2D graphics for our initial game development.
Using SFML, you can draw basic shapes to represent game elements. Here's how to draw a rectangle:
sf::RectangleShape rectangle(sf::Vector2f(100, 50));
rectangle.setFillColor(sf::Color::Green);
window.draw(rectangle);
This code snippet demonstrates how easy it is to create and display shapes on the screen.
Handling User Input
Capturing user input is essential for gameplay. You need to read the keyboard or mouse state to respond to player actions. Here’s an example using SFML to capture keyboard input for movement:
if (event.type == sf::Event::KeyPressed) {
if (event.key.code == sf::Keyboard::W) {
// Move Up
}
}
Understanding user input will greatly enhance the interactivity of your game.
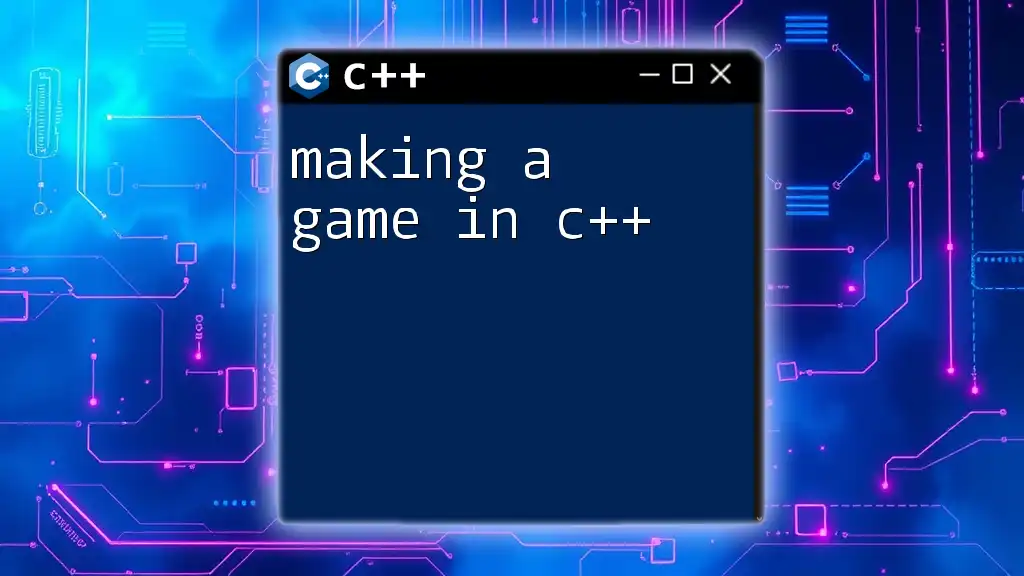
Developing a Simple Game
Concept Development
Before diving into coding, spend some time on concept development. Choose a game genre that excites you—be it a platformer, shooter, or puzzle game. Brainstorm game mechanics, design elements, and plot ideas. Consider creating designs and wireframes to visualize the final product.
Building the Game Structure
Setting Up the Project
A well-structured project is crucial for successful game development. Organize your files with clear naming conventions. Create a `src` folder for source files, a `res` folder for resources like images and sounds, and a `docs` folder for documentation.
Main Class Structure
Your game’s code should revolve around classes. A basic `Game` class might encompass the following structure:
class Game {
public:
Game();
void processEvents();
void update();
void render();
private:
sf::RenderWindow window;
};
This encapsulation makes it easier to manage different functionalities.
Implementing Game Features
Graphics and Sprites
To incorporate graphics, you will need to load images. Here's how you can load a sprite using SFML:
sf::Texture texture;
if (!texture.loadFromFile("character.png")) {
// handle error
}
sf::Sprite sprite(texture);
window.draw(sprite);
This example shows how to load and render a sprite, which adds visual appeal to your game.
Adding Sound
Sounds can significantly enhance gameplay. SFML makes playing sound a breeze. Here's how to add sound effects:
sf::SoundBuffer buffer;
if (!buffer.loadFromFile("effect.wav")) {
// handle error
}
sf::Sound sound;
sound.setBuffer(buffer);
sound.play();
Incorporating sound will elevate the gaming experience, pulling players deeper into your world.
Collision Detection
Implementing collision detection ensures that interactions between game entities are processed correctly. A simple bounding box collision can be managed as follows:
if (player.getGlobalBounds().intersects(enemy.getGlobalBounds())) {
// handle collision
}
This logic checks if there is overlap between the player and enemy objects.
Scoring and Game Logic
The game's rules dictate how players interact with the game’s mechanics. Implementing a basic scoring system can be as simple as:
int score = 0;
score += 100; // Increment score on some game event
This approach allows for easy tracking of player performance.
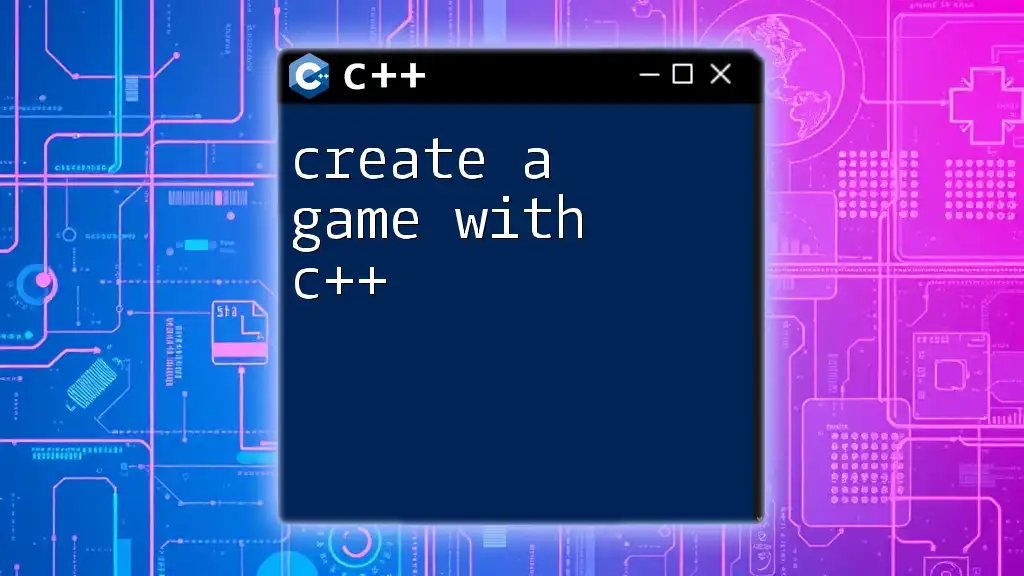
Testing and Debugging
Importance of Testing
Testing is vital before releasing your game. Regular playtests can uncover bugs and provide feedback from users on gameplay mechanics and controls.
Debugging Tips
As you refine your game, debugging becomes a crucial skill. Use tools like the debugger provided in your IDE to trace errors. Keep an eye out for common pitfalls such as pointer errors, memory leaks, and infinite loops.
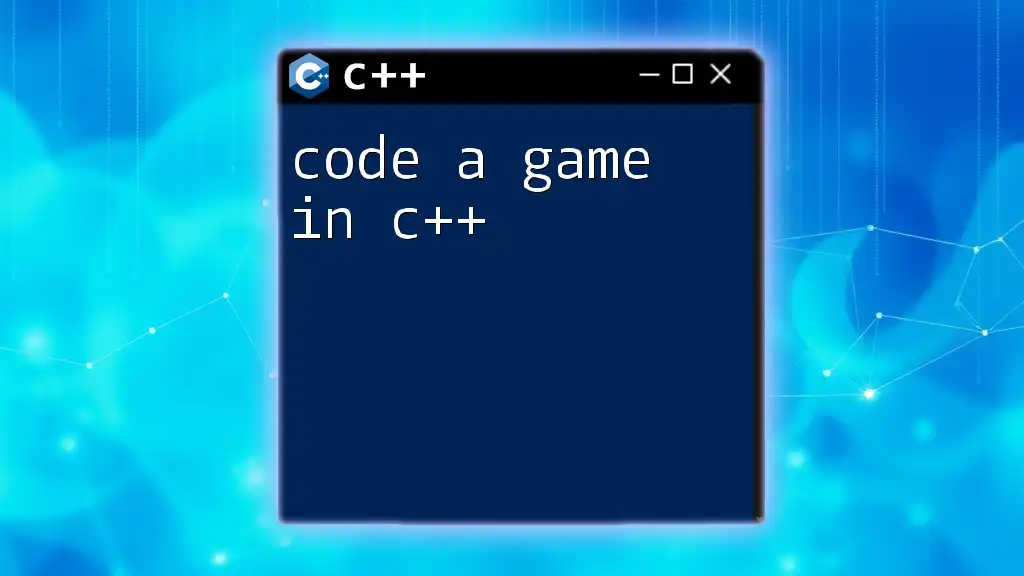
Final Touches
Optimizing Your Game
Once your game is functional, it’s time to consider performance optimization. Techniques to enhance your game might include reducing draw calls, optimizing algorithms, and utilizing data structures appropriately. Here’s a simple optimization example:
// Instead of drawing every object independently, use a vertex array to batch draw.
sf::VertexArray triangles(sf::Triangles, 3);
Polishing Your Game
Finally, add finishing touches like polished menus, settings screens, and help sections. Playtesting for feedback can greatly improve the gameplay experience.
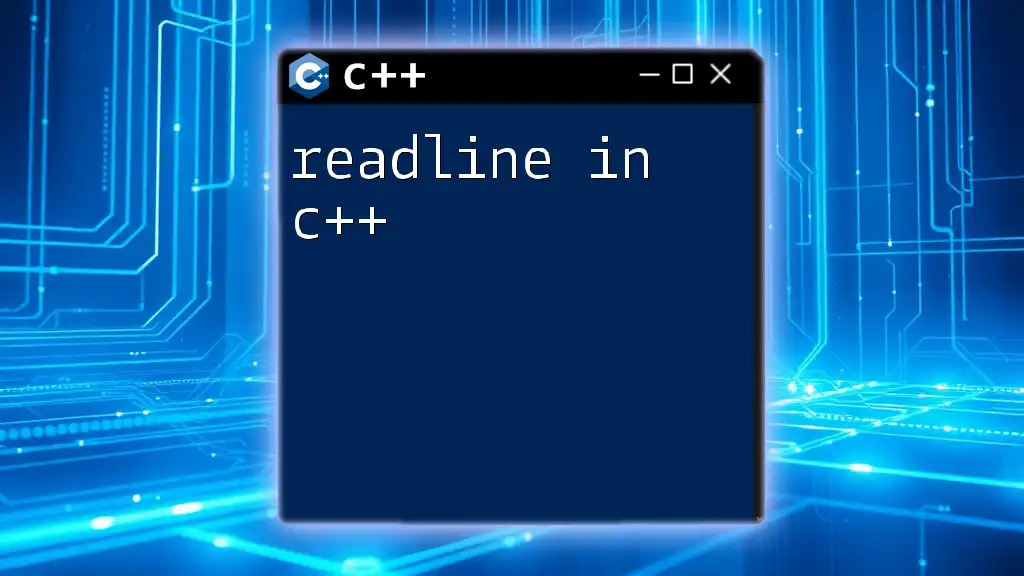
Conclusion
In this article, we explored the essential components and techniques used in creating a game in C++. Remember that game development is an iterative process. Don’t hesitate to experiment and explore new ideas. Use the resources available to deepen your knowledge and skills. With practice and perseverance, you can create engaging games that captivate players.
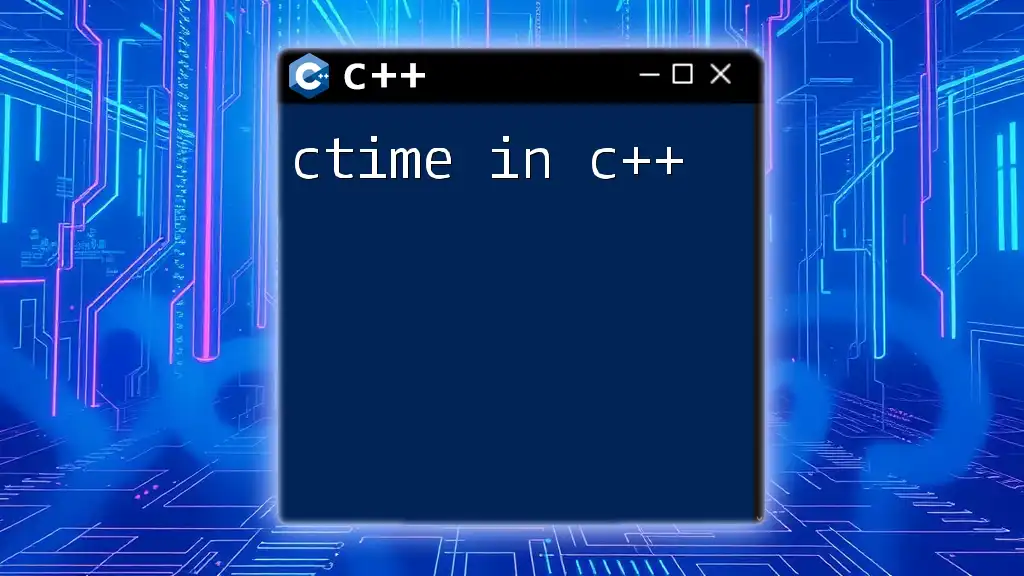
Additional Resources
For further learning, explore tutorials, books, and online communities focused on game development in C++. Official documentation for SFML and SDL is also invaluable for understanding the intricacies of these libraries. Happy coding!