Learn to code a simple game in C++ by creating a basic number guessing game that prompts the player to guess a randomly generated number between 1 and 100.
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned int>(time(0)));
int number = rand() % 100 + 1;
int guess;
std::cout << "Guess a number between 1 and 100: ";
while (true) {
std::cin >> guess;
if (guess < number) {
std::cout << "Too low! Try again: ";
} else if (guess > number) {
std::cout << "Too high! Try again: ";
} else {
std::cout << "Congratulations! You guessed the number." << std::endl;
break;
}
}
return 0;
}
Setting Up Your C++ Development Environment
Choosing a C++ IDE
To code a game in C++, the first step is setting up your development environment. Selecting the right Integrated Development Environment (IDE) can significantly enhance your coding experience. Here are some popular options:
- Visual Studio: Offers a robust set of tools and features specifically for C++. It's user-friendly and comes with powerful debugging capabilities.
- Code::Blocks: A lightweight, open-source IDE that's great for beginners. It supports multiple compilers.
- CLion: A commercial IDE with excellent support for CMake projects and a strong focus on smart code assistance.
Once you've chosen an IDE, download and configure it following the provided installation guidelines.
Installing Necessary Libraries
For game development, you'll often need to utilize additional libraries that help manage graphics and sound. Two of the most popular libraries are SFML (Simple and Fast Multimedia Library) and SDL (Simple DirectMedia Layer).
To install SFML or SDL:
- Visit their official websites.
- Download the version compatible with your platform.
- Follow the instructions to set them up within your IDE.
This setup allows your C++ projects to harness the power of graphics and sound manipulation effortlessly.
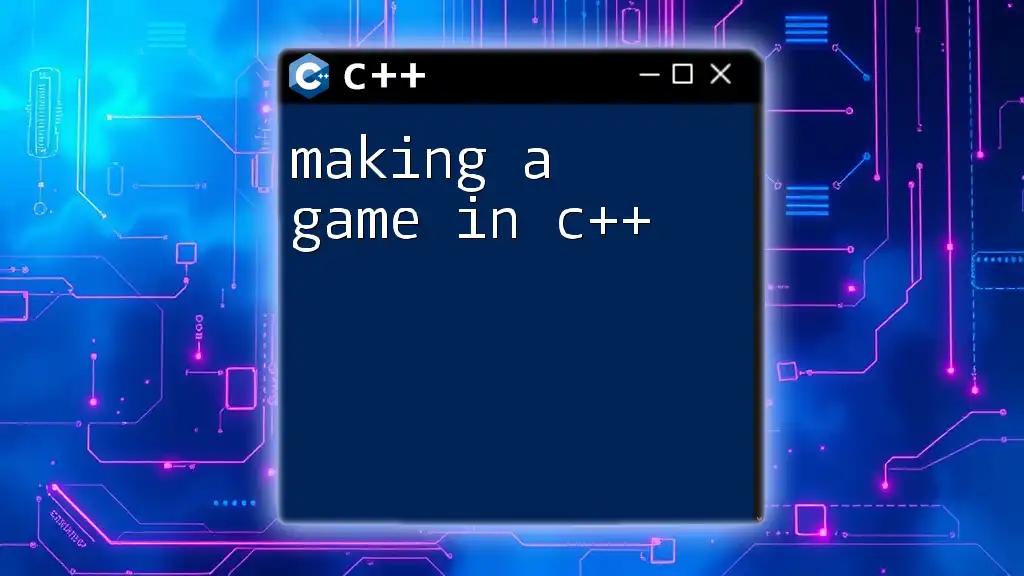
Fundamentals of Game Development in C++
Understanding Game Loop Architecture
The game loop is at the heart of any game application. It continuously cycles through processing input, updating game state, and rendering graphics. Understanding this structure is crucial for coding a game in C++.
A basic structure of a game loop in C++ looks like:
while (gameRunning) {
ProcessInput();
Update();
Render();
}
This loop runs until the game is no longer active. Each function within this loop performs critical tasks:
- ProcessInput() captures user inputs.
- Update() modifies game state based on inputs.
- Render() draws the updated state on the screen.
Game States Management
Managing different game states is essential; it enables smooth transitions between menu, gameplay, and pause interactions. An enum class can efficiently handle the various states:
enum class GameState { MENU, PLAYING, PAUSED };
GameState currentState;
if (currentState == GameState::MENU) {
// Show menu
} else if (currentState == GameState::PLAYING) {
// Execute game logic
} else if (currentState == GameState::PAUSED) {
// Show pause menu
}
This organization makes your code cleaner and easier to maintain.
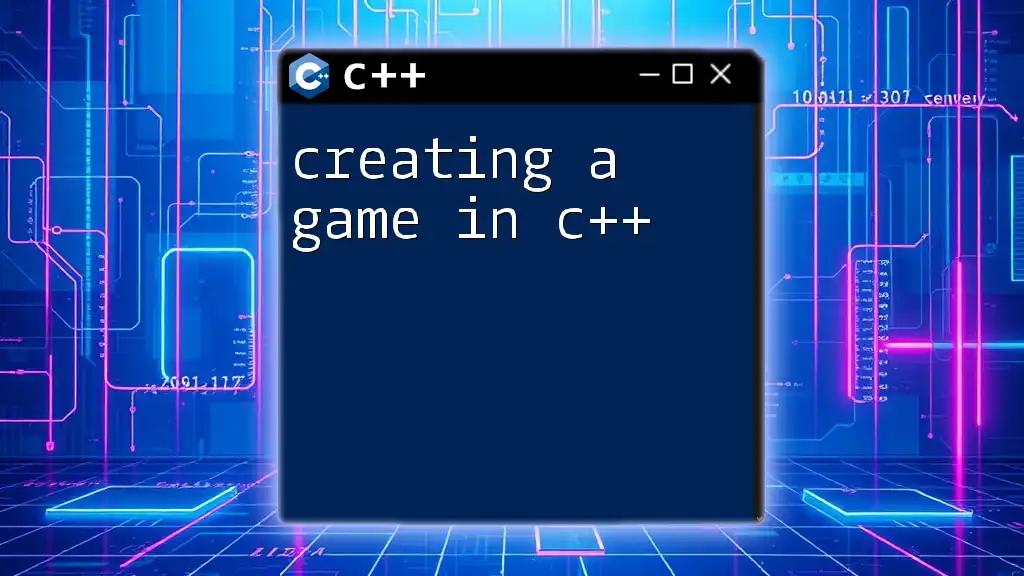
Designing Your First Simple Game
Choosing a Game Concept
When you begin your game development journey, it's advisable to pick a simple idea. Some suggestions include:
- Tic-Tac-Toe: A turn-based game that introduces players to logic and conditionals.
- Snake: A classic that demonstrates basic movement and collision detection.
- A simple platformer: A lightweight game that can refine your skills in managing physics and character control.
Creating Game Assets
Visual assets and sound play an integral role in making your game engaging. Use software like GIMP for sprites or Audacity for sound effects. These tools allow you to create custom assets that can bring your game to life.
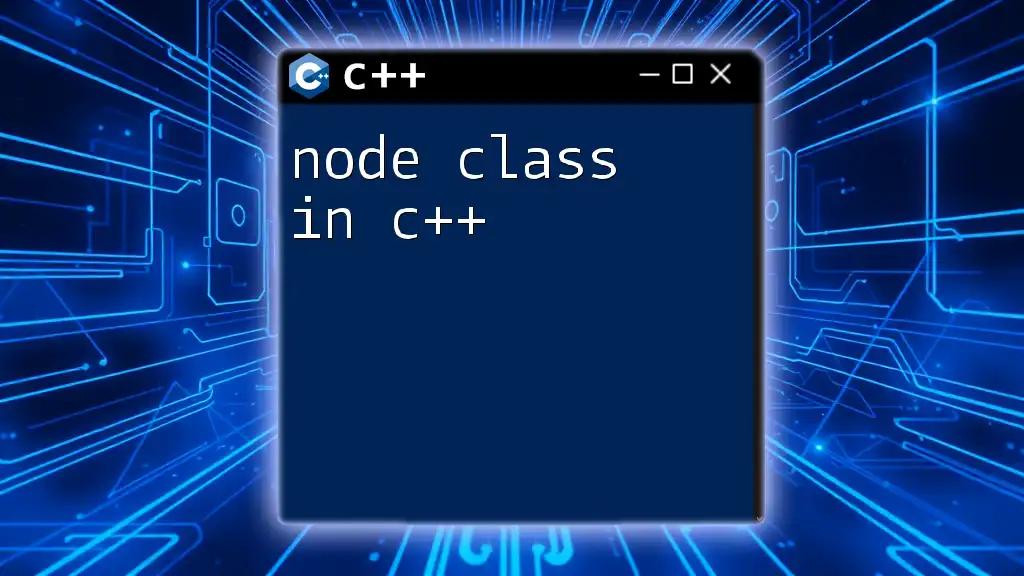
Core Programming Concepts in Game Development
Using Classes and Objects
C++ is an object-oriented language, making it useful for game development. By using classes, you can create modular and reusable code components. For instance, a `Player` class could look like this:
class Player {
public:
void Move(int x, int y);
private:
int positionX, positionY;
};
This abstraction lets you manage different players and their behaviors efficiently.
Handling User Input
Capturing user input is vital for interactive gameplay. Libraries like SFML allow you to detect keyboard and mouse interactions effortlessly. Here's a simple example using SFML:
if (sf::Keyboard::isKeyPressed(sf::Keyboard::W)) {
player.Move(0, -1);
}
This snippet allows your player to move up when the "W" key is pressed.
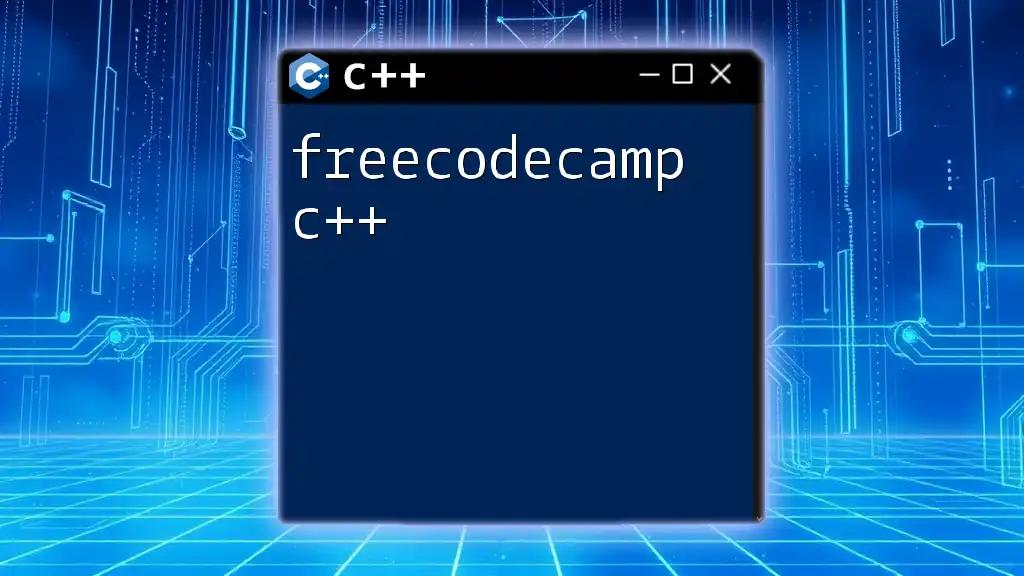
Implementing Basic Game Mechanics
Collision Detection
Collision detection is crucial for making the game world interactively rich. You can implement it by checking for intersections between objects. Here’s a basic example:
if (player.getBounds().intersects(enemy.getBounds())) {
// Handle collision
}
This code checks if the player intersects with an enemy object, and you can define what happens during the collision, whether it's losing a life or triggering a specific event.
Game Scoring System
In most games, a scoring system is an integral feature. It not only motivates players but also provides feedback on their performance. You can maintain scores easily:
int score = 0;
void UpdateScore(int points) {
score += points;
}
This way, your score can be updated based on player actions, creating a rewarding experience.
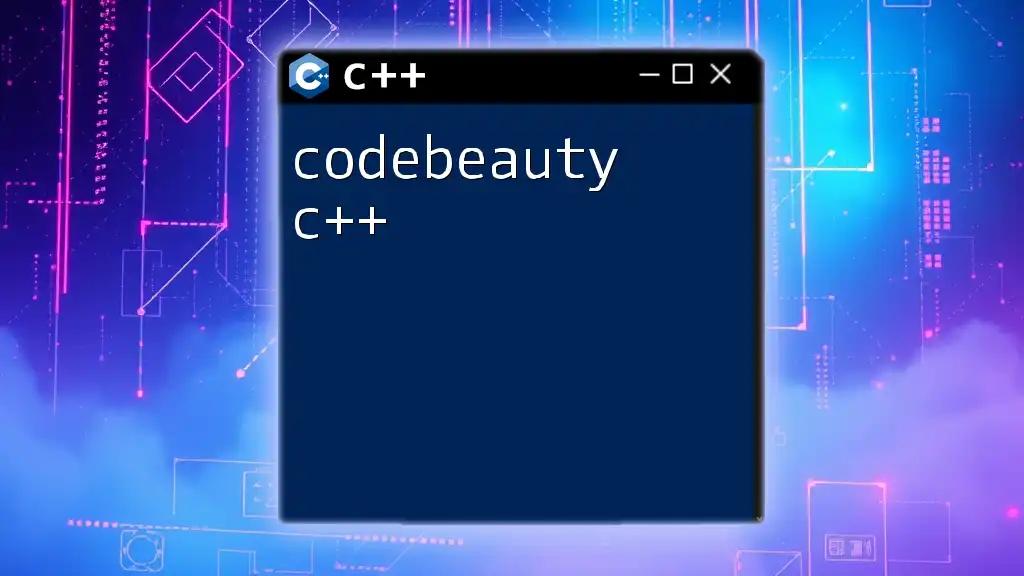
Adding Graphics and Sound
Rendering Sprites on Screen
Visual elements are pivotal when coding a game in C++. Using SFML or SDL, you can render sprites quickly. A simple code snippet to render a player sprite looks like:
window.draw(playerSprite);
This draws the `playerSprite` onto the game window, making it visible during each frame of the game loop.
Incorporating Sound Effects and Music
Sound enhances the gaming experience. With SFML, integrating sound is straightforward. Here’s how you can play a sound effect:
sf::Sound sound;
sound.setBuffer(soundBuffer);
sound.play();
This enables you to give feedback to players, enhancing immersion.

Enhancing Your Game
Implementing Levels and Difficulty
As you expand your game, consider levels and difficulty progression. This keeps players engaged and challenges them. You might structure your game to load different levels like this:
void LoadLevel(int level) {
// Load level-specific assets and settings
}
Building a User Interface (UI)
An effective user interface enhances usability, allowing players to navigate through menus and view scores effortlessly. Consider implementing a simple menu using buttons and overlays, ensuring the UI enhances the overall experience.

Testing and Debugging Your Game
Common Debugging Techniques
Debugging is crucial in game development. Through careful debugging practices, like printing variable states or using breakpoints, you can identify and fix issues promptly.
Testing Game Performance
Performance testing is vital, especially for larger projects. Use profiling tools to identify bottlenecks and ensure your game runs smoothly across devices, enhancing the player experience.

Final Project: Coding a Simple Game
Putting It All Together
Now that you have learned the essential concepts, it’s time to create a complete simple game from scratch. Start by implementing the discussed features, and utilize existing code snippets to ease the development process.
Code Snippet and Explanation
For your final project, consider coding a simple snake game. Here’s a foundational code snippet to get started:
// main.cpp
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Simple Snake Game");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
// Draw game objects here
window.display();
}
return 0;
}
Tips for Future Improvements
Once you've created your basic game, think about what features you would like to add next. Implementing enhancements like power-ups, multiple levels, or a high score leaderboard can delight players and keep them engaged.
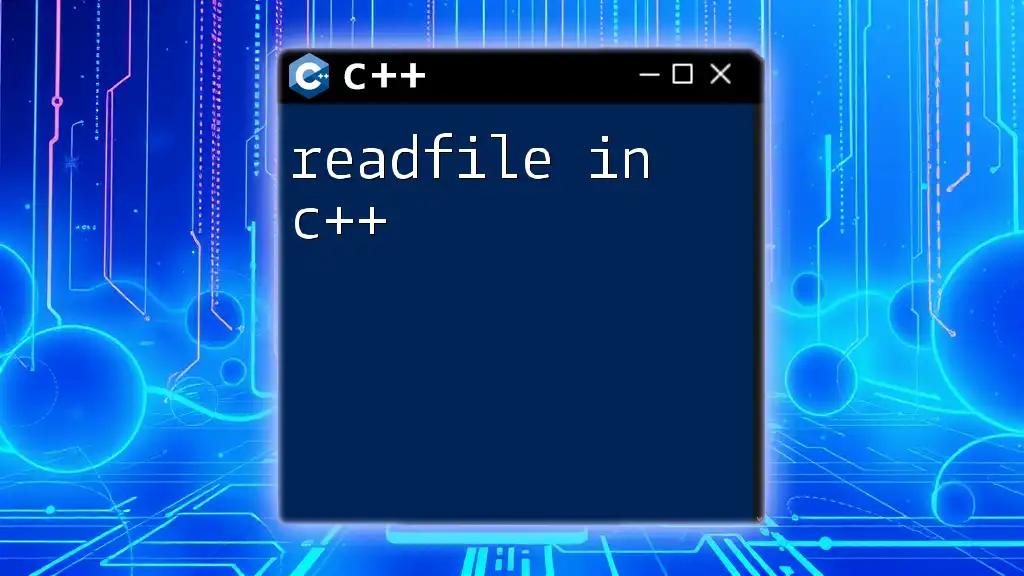
Conclusion
Coding a game in C++ opens a pathway to vibrant creativity and technical skill growth. As you dive deeper into game development, don't hesitate to explore new resources and learn from the community. Your journey is just beginning, so stay curious and keep experimenting!
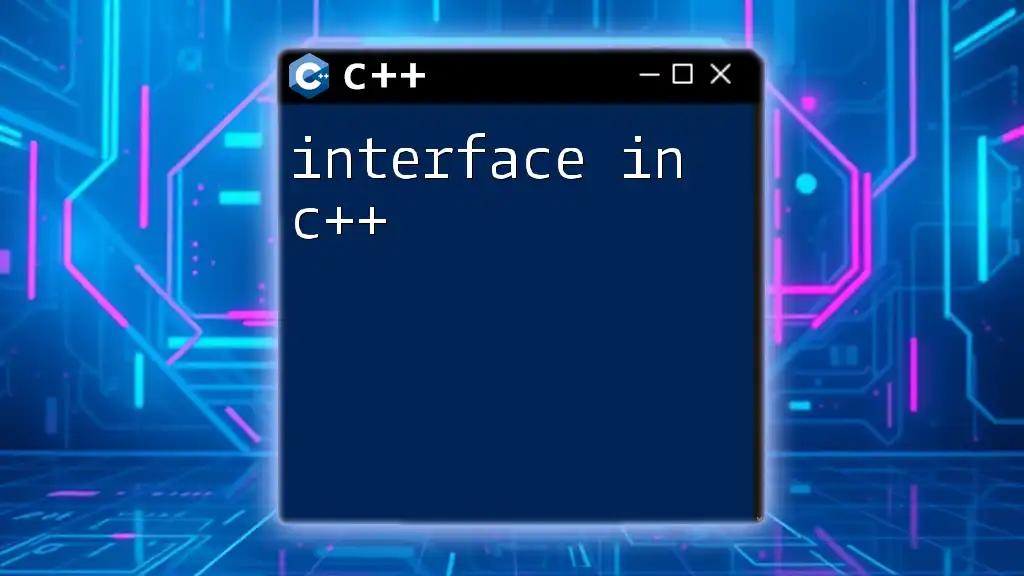
Call to Action
Are you ready to dive deeper into C++ and game development? Check out our courses and resources designed specifically for aspiring game developers. We welcome your feedback and questions as you embark on your coding journey!