`ctime` in C++ is a header file that provides functions to manipulate date and time, allowing you to obtain current time and format it easily.
Here's a simple code snippet using `ctime`:
#include <iostream>
#include <ctime>
int main() {
std::time_t now = std::time(0); // get the current time
std::cout << "Current time: " << std::ctime(&now); // convert to string and print
return 0;
}
Understanding Time Representation
What is time representation in C++?
Time representation in C++ is fundamental for managing date and time information in various applications. Accurate handling of time-related data is crucial, especially in databases, event scheduling, and time-based operations. Understanding how C++ stores and manipulates time allows developers to write more efficient and robust code.
The `time_t` Data Type
`time_t` is a built-in type in C++ used to represent calendar time. It is primarily used to store the number of seconds since the epoch (00:00:00 UTC on 1 January 1970). To declare and use `time_t`, you can do the following:
#include <ctime>
#include <iostream>
int main() {
time_t currentTime = time(0);
std::cout << "Current time in seconds since epoch: " << currentTime << std::endl;
return 0;
}
This simple example outputs the current time in seconds since the epoch, showcasing how `time_t` serves as a universal time representation.
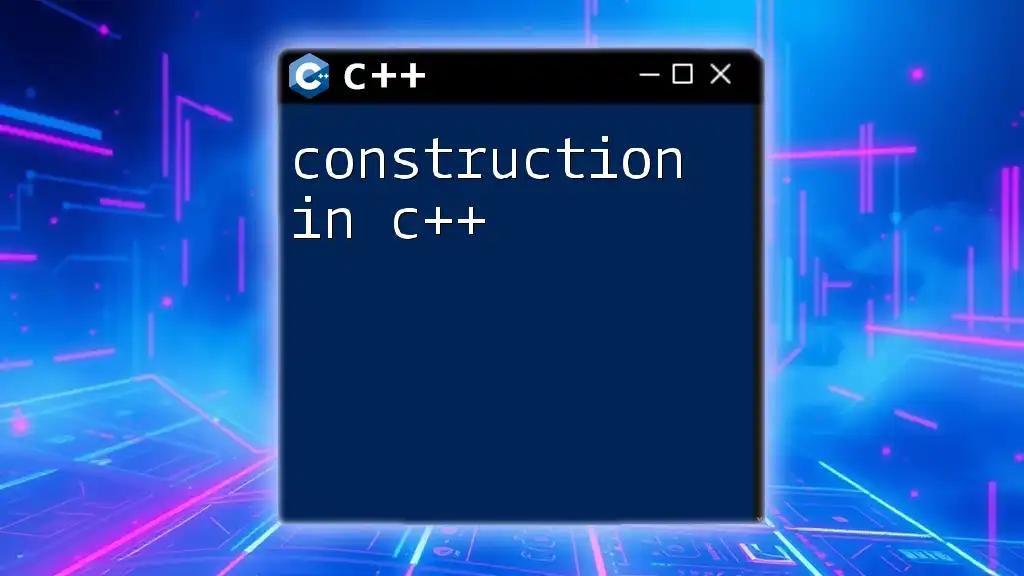
Key Functions of the `ctime` Library
`time()`
The `time()` function retrieves the current calendar time. When called without an argument, it returns the current time as a `time_t` object. Here's an example:
#include <ctime>
#include <iostream>
int main() {
time_t now = time(0);
std::cout << "Current time: " << now << std::endl;
return 0;
}
This outputs the number of seconds since the epoch, providing a baseline for further time manipulations.
`localtime()`
The `localtime()` function converts the `time_t` value into a `struct tm` that contains local time representation, including year, month, day, hours, minutes, and seconds. An example is as follows:
#include <ctime>
#include <iostream>
int main() {
time_t now = time(0);
struct tm *localTime = localtime(&now);
std::cout << "Local time: " << localTime->tm_hour << ":"
<< localTime->tm_min << ":" << localTime->tm_sec << std::endl;
return 0;
}
This snippet converts the current time into local time and prints the hour, minute, and second components.
`gmtime()`
In contrast to `localtime()`, the `gmtime()` function provides the Coordinated Universal Time (UTC). Using both functions illustrates varying time representations:
#include <ctime>
#include <iostream>
int main() {
time_t now = time(0);
struct tm *localTime = localtime(&now);
struct tm *utcTime = gmtime(&now);
std::cout << "Local Time: " << localTime->tm_hour << ":"
<< localTime->tm_min << ":" << localTime->tm_sec << std::endl;
std::cout << "UTC Time: " << utcTime->tm_hour << ":"
<< utcTime->tm_min << ":" << utcTime->tm_sec << std::endl;
return 0;
}
This example highlights the differences in output based on local timezone settings versus UTC.
`asctime()`
The `asctime()` function converts the `struct tm` to a human-readable string format. Here’s an example:
#include <ctime>
#include <iostream>
int main() {
time_t now = time(0);
struct tm *localTime = localtime(&now);
std::cout << "Current Time: " << asctime(localTime);
return 0;
}
The output will look something like this: `Tue Feb 14 17:03:10 2023`, demonstrating the human-friendly format generated by `asctime()`.
`ctime()`
The `ctime()` function also converts `time_t` to a string format, but it returns a pointer to statically allocated memory. For example:
#include <ctime>
#include <iostream>
int main() {
time_t now = time(0);
std::cout << "Current time as string: " << ctime(&now);
return 0;
}
This gives a similar output, making `ctime()` an easy alternative for getting the current time in string format.
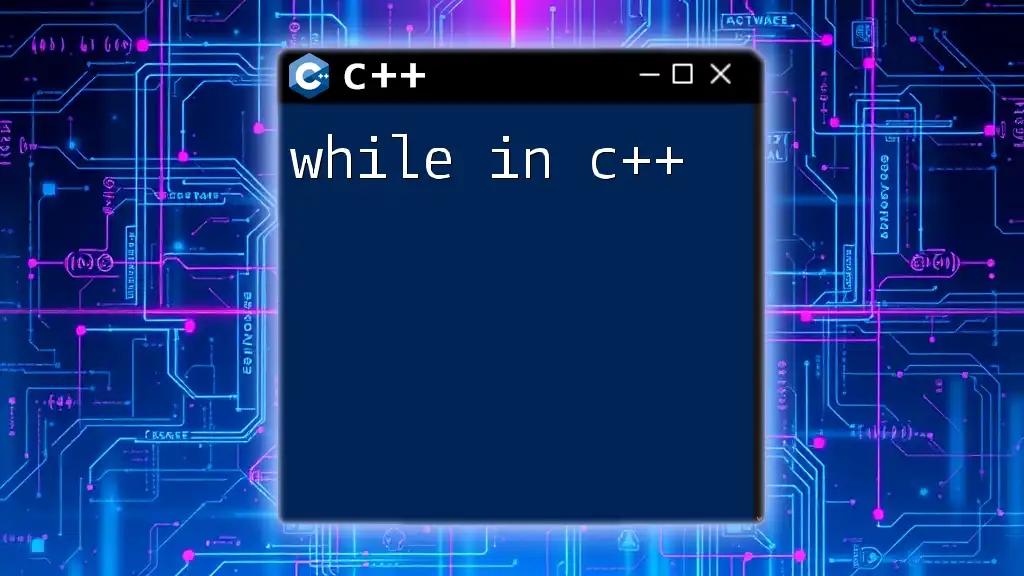
Formatting Time and Date
Custom Formatting with `strftime()`
The `strftime()` function allows developers to create custom formats for time representation. Here's how you can use it:
#include <ctime>
#include <iostream>
int main() {
time_t now = time(0);
struct tm *localTime = localtime(&now);
char buffer[80];
strftime(buffer, 80, "Today is %Y-%m-%d %H:%M:%S.", localTime);
std::cout << buffer << std::endl;
return 0;
}
In this example, `strftime()` formats the local time into a readable string format, demonstrating the flexibility of time formatting in C++.
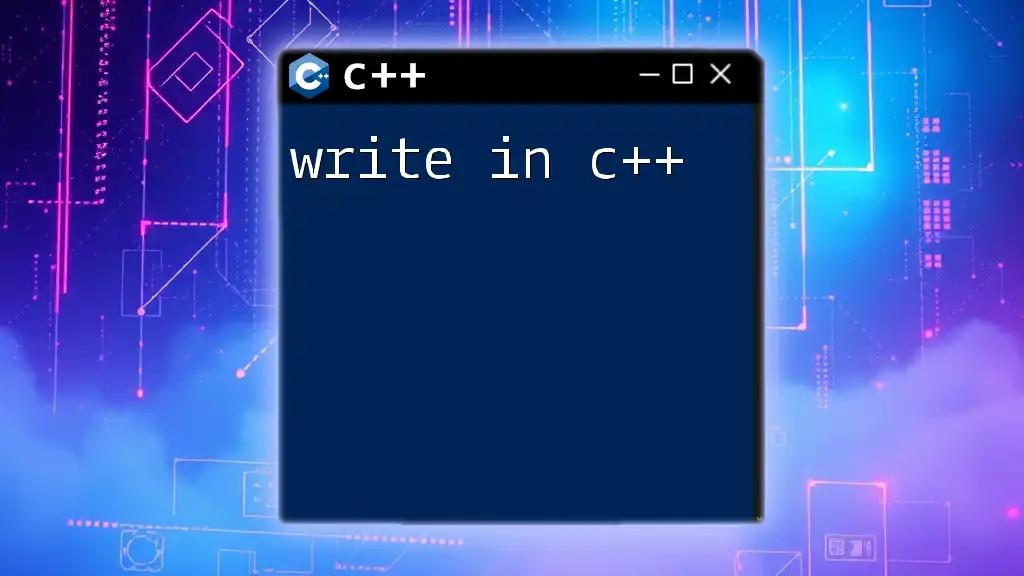
Real-World Applications of `ctime`
Simple Timer Application
Creating a countdown timer is an excellent application of the `ctime` library. Here’s a basic example:
#include <ctime>
#include <iostream>
#include <thread>
void countdown(int seconds) {
while(seconds >= 0) {
std::cout << seconds << " seconds remaining." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
seconds--;
}
std::cout << "Time's up!" << std::endl;
}
int main() {
countdown(10);
return 0;
}
This simple countdown function utilizes `ctime` to track remaining seconds, providing real-time feedback.
Date and Time Logging
Accurate logging is essential for tracking application performance and events. Here is a simple logging function using `ctime`:
#include <ctime>
#include <iostream>
#include <fstream>
void logEvent(const std::string& event) {
std::ofstream logFile("events.log", std::ios::app);
time_t now = time(0);
logFile << asctime(localtime(&now)) << ": " << event << std::endl;
logFile.close();
}
int main() {
logEvent("Application started.");
return 0;
}
This code appends a log entry with a timestamp to a file, showcasing how `ctime` can enhance event tracking.
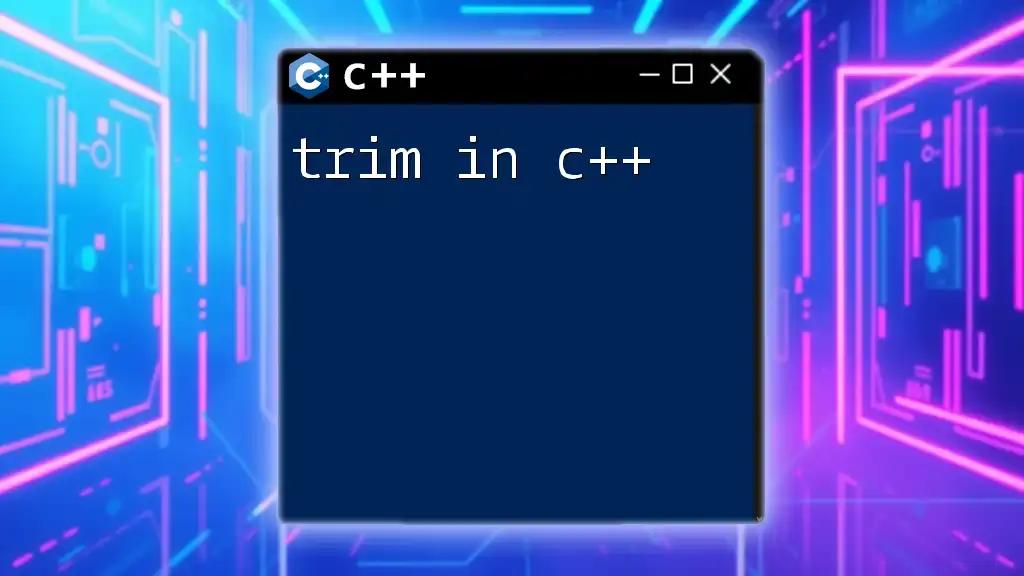
Best Practices When Using `ctime`
Handling Timezones
Timezone awareness is vital when managing dates and times, primarily when your application operates across multiple regions. It's recommended to always consider the user’s local time and provide options to specify time zones when necessary.
Managing Daylight Saving Time
Daylight Saving Time (DST) can introduce complexity in time calculations. Always be mindful of the implications of DST, especially when logging or scheduling events. Implementing libraries that handle such nuances or maintaining alternative strategies to track local time adjustments can be beneficial.

Troubleshooting Common Issues
Misunderstanding Time Zones
Developers often miscalibrate time settings due to local versus UTC time confusion. To avoid such pitfalls, always ensure clarity on whether a function is returning local time or UTC, and document your time handling logic explicitly.
Incorrect Time Calculations
Time arithmetic can produce unexpected results if not carefully managed. Always validate your time calculations, particularly when adding or subtracting time values. Using structured functions like `mktime()` may help in conversions and sanity checks.

Conclusion
The `ctime` library in C++ offers versatile tools for managing time representation and manipulations. From retrieving the current time to formatting and logging timestamps, it proves critical for various applications. Embrace these functions and best practices to enhance your coding skillset and understanding of time in C++. Keep exploring this integral aspect of C++ programming for deeper insights and functionality.