A node class in C++ is typically used in data structures, such as linked lists and trees, to encapsulate the data and a pointer to the next node or child, facilitating traversal and manipulation of these structures.
Here’s a simple example of a node class for a singly linked list:
class Node {
public:
int data; // Data to store
Node* next; // Pointer to the next node
Node(int value) : data(value), next(nullptr) {} // Constructor to initialize the node
};
What is a Node Class?
A node class in C++ serves as a fundamental building block in various data structures, such as linked lists, trees, and graphs. Each node typically encapsulates data and a pointer (or multiple pointers) to other nodes, enabling the dynamic resizing of these structures.
Components of a Node Class
The primary components of a node class generally include:
- Data Storage: This holds the value carried by the node.
- Pointer(s): These are essential for linking nodes in data structures.
A constructor is crucial for initializing these components. It establishes the initial state of the node upon creation.
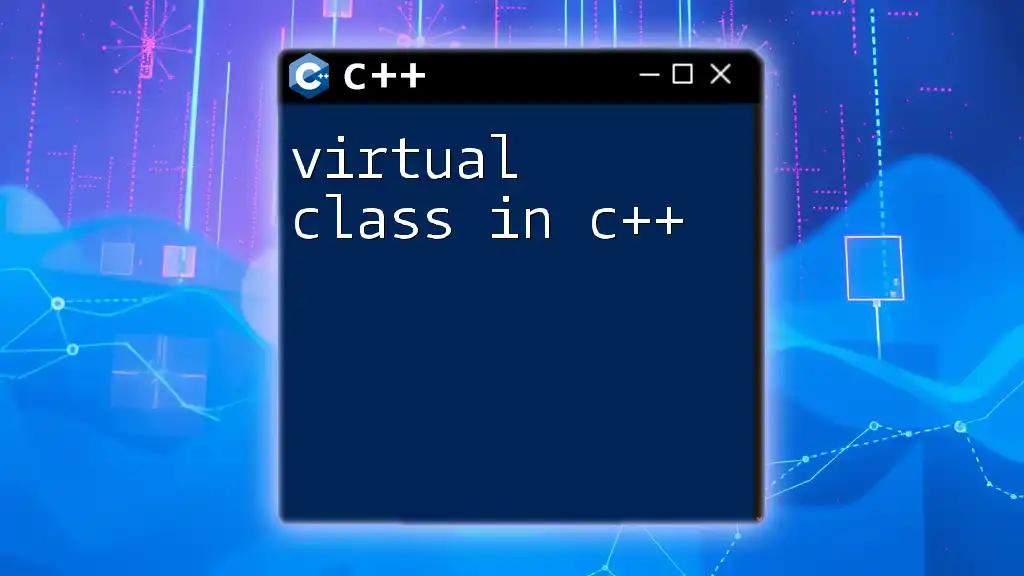
Basic Structure of a Node Class in C++
Creating a node class in C++ is straightforward. Below is an example of a simple node class definition:
class Node {
public:
int data; // Data held by the node
Node* next; // Pointer to the next node
// Constructor to initialize the node
Node(int value) : data(value), next(nullptr) {}
};
In this code:
- We define a class named Node.
- data is an integer representing the node's value.
- next is a pointer to the next node in the sequence.
- The constructor initializes the node's data with a value and sets next to `nullptr`, indicating it initially points to nothing.

Implementing a Singly Linked List using Node Class
What is a Singly Linked List?
A singly linked list is a linear data structure consisting of nodes, where each node points to the next node. Unlike arrays, linked lists allow dynamic memory allocation, making them more flexible in size and structure as data grows or shrinks.
Creating the Linked List Class
In implementing a singly linked list, we can define a class that uses our node class:
class SinglyLinkedList {
private:
Node* head; // Pointer to the head of the list
public:
SinglyLinkedList() : head(nullptr) {} // Constructor initializes the head to nullptr
// Method to insert a new node at the end
void insert(int value) {
Node* newNode = new Node(value);
if (!head) {
head = newNode; // If the list is empty, assign newNode as head
return;
}
Node* temp = head; // Start at the head to find the last node
while (temp->next) {
temp = temp->next; // Traverse the list
}
temp->next = newNode; // Link the new node
}
// Method to display the linked list
void display() {
Node* temp = head; // Start at the head
while (temp) {
std::cout << temp->data << " -> ";
temp = temp->next; // Move to the next node
}
std::cout << "nullptr" << std::endl; // Indicate end of the list
}
};
In this implementation:
- head is a pointer to the first node of the list.
- The insert method creates a new node and appends it to the end of the list.
- The display method traverses the linked list and prints each node's data.

Advanced Node Class Features
Creating a Doubly Linked List
Doubly linked lists are an extension of singly linked lists, where each node contains pointers to both the next and previous nodes. This bi-directional capability allows for better traversal in both directions.
Example Code for Doubly Node Class
Here’s an example of a doubly node class:
class DoublyNode {
public:
int data; // Data encapsulated in the node
DoublyNode* next; // Pointer to the next node
DoublyNode* prev; // Pointer to the previous node
// Constructor
DoublyNode(int value) : data(value), next(nullptr), prev(nullptr) {}
};
This code introduces an additional pointer, prev, which references the previous node in the list. This structure enhances navigability.
Implementing the Doubly Linked List
Building on the DoublyNode, we can create a doubly linked list:
class DoublyLinkedList {
private:
DoublyNode* head; // Pointer to the head of the list
public:
DoublyLinkedList() : head(nullptr) {} // Constructor initializes head to nullptr
void insert(int value) {
DoublyNode* newNode = new DoublyNode(value);
if (!head) {
head = newNode; // If list is empty, newNode becomes head
return;
}
DoublyNode* temp = head; // Start from head
while (temp->next) {
temp = temp->next; // Traverse to the last node
}
temp->next = newNode; // Link newNode to the last node
newNode->prev = temp; // Link back to the previous node
}
void display() {
DoublyNode* temp = head; // Start from head
while (temp) {
std::cout << temp->data << " <-> ";
temp = temp->next; // Move to the next node
}
std::cout << "nullptr" << std::endl; // Indicate end of the list
}
};
Here, insert links the new node both forward and backward, helping maintain the doubly linked structure.

Handling Memory Management in Node Classes
Importance of Memory Management in C++
When using pointers in C++, memory management becomes crucial. Incorrect handling can lead to memory leaks and undefined behavior, especially with dynamic allocations.
Using Smart Pointers
To mitigate risks associated with raw pointers, C++ provides smart pointers such as `std::shared_ptr` and `std::unique_ptr`. These automate memory management and help ensure no memory is leaked.
An example using `std::shared_ptr` in a node class looks like this:
#include <memory>
class Node {
public:
int data;
std::shared_ptr<Node> next; // Smart pointer to the next node
Node(int value) : data(value), next(nullptr) {}
};
Using smart pointers:
- Automatically deallocates memory when no longer needed.
- Prevents memory leaks and dangling pointers, enhancing code safety and reliability.

Common Errors When Working with Node Classes
Common Mistakes in Node Class Implementation
When working with node classes, several pitfalls can occur:
- Circular References: Misconfigured pointers can create loops, making list traversal impossible.
- Memory Leaks: Failing to release memory can exhaust resources in long-running applications.
Debugging Tips and Best Practices
To avoid these issues:
- Always Initialize Pointers: Never leave pointers uninitialized to prevent undefined behavior.
- Use Destructors: Implement destructors in your classes to ensure memory is released properly, especially when using raw pointers.
- Use Valgrind or Similar Tools: Tools can help track memory usage and detect leaks or issues.

Conclusion
The node class in C++ is a versatile but essential construct in various data structures. Understanding its design and implementation opens doors to manipulating more complex data structures efficiently. Experimenting with node classes through projects will deepen your comprehension and solidify your knowledge in C++.
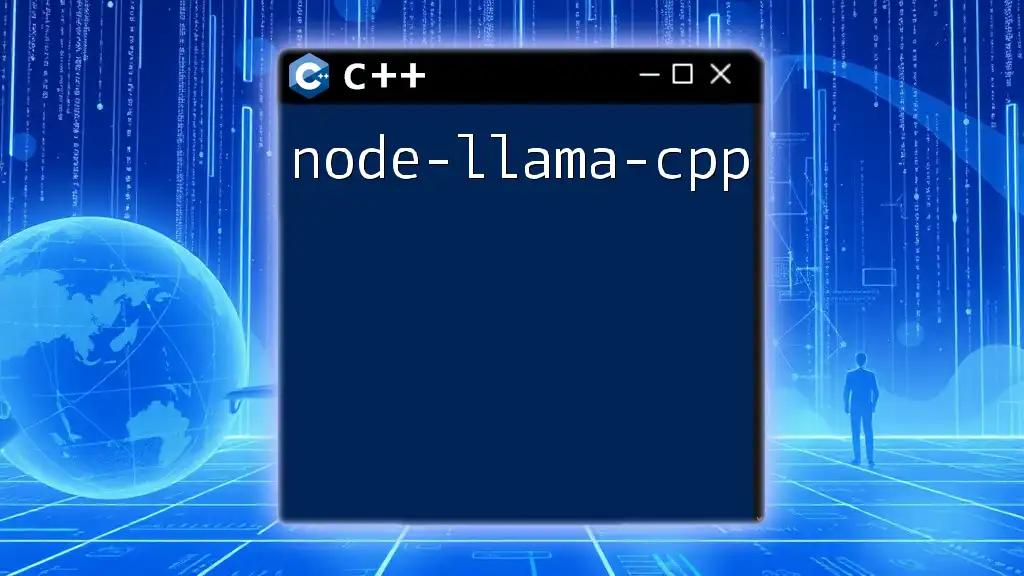
Additional Resources
To further enhance your understanding of node classes and data structures in C++, consider exploring:
- Books on data structures and algorithms in C++.
- Online tutorials and lectures, such as those available on educational platforms.
- Forums like Stack Overflow and Reddit, where you can engage with fellow learners and professionals.
By delving into these resources, you can solidify your grasp on the node class and expand your programming expertise.