In C++, a class is defined using the `class` keyword followed by the class name and a block of code containing its members and methods.
Here's an example:
class MyClass {
public:
int myNumber; // Attribute
void myFunction() { // Method
// Function code
}
};
What is a Class in C++?
A class in C++ is a blueprint for creating objects. It encapsulates data for the object and methods to manipulate that data. Classes form the foundation of Object-Oriented Programming (OOP) in C++, enabling developers to model real-world entities and behaviors.
Classes help foster key OOP principles like encapsulation, inheritance, and polymorphism. Imagine a class as a template for a house: it defines the layout, dimensions, and features, but actual houses (objects) will vary according to what you build from that template.
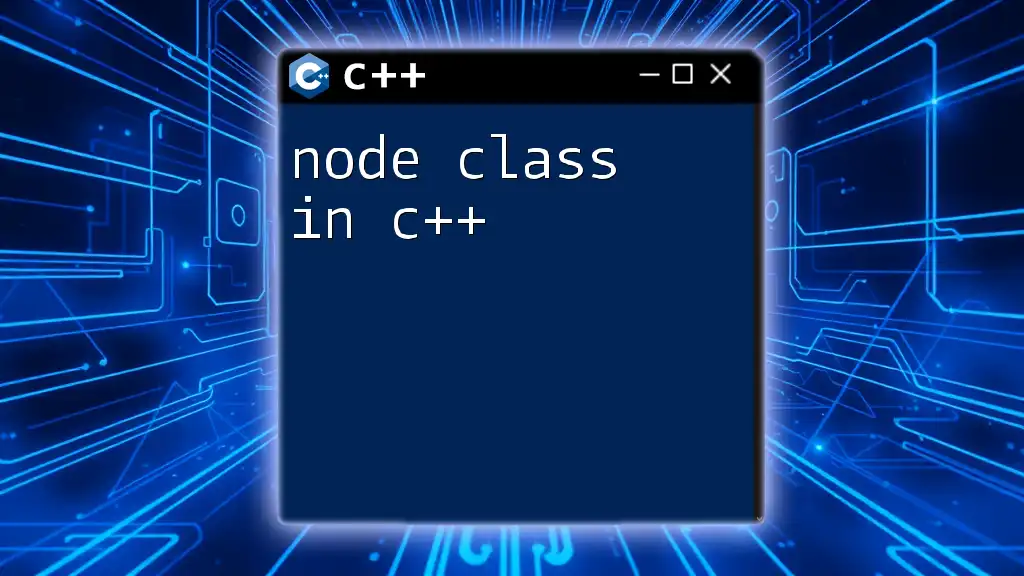
How to Declare a Class in C++
The Basic Syntax of a Class Declaration
To define a class in C++, you use the `class` keyword, followed by the class name, and a pair of curly braces `{}` encompassing its members. After closing the braces, you end the declaration with a semicolon `;`.
Here’s the basic syntax:
class ClassName {
// Member variables and functions
};
Example: A Simple Class Declaration
Let’s look at a straightforward example of a class declaration:
class Car {
public:
string make;
string model;
int year;
};
In this example, we define a class named `Car` with three public member variables: `make`, `model`, and `year`. The `public` access modifier allows these variables to be accessed from outside the class.
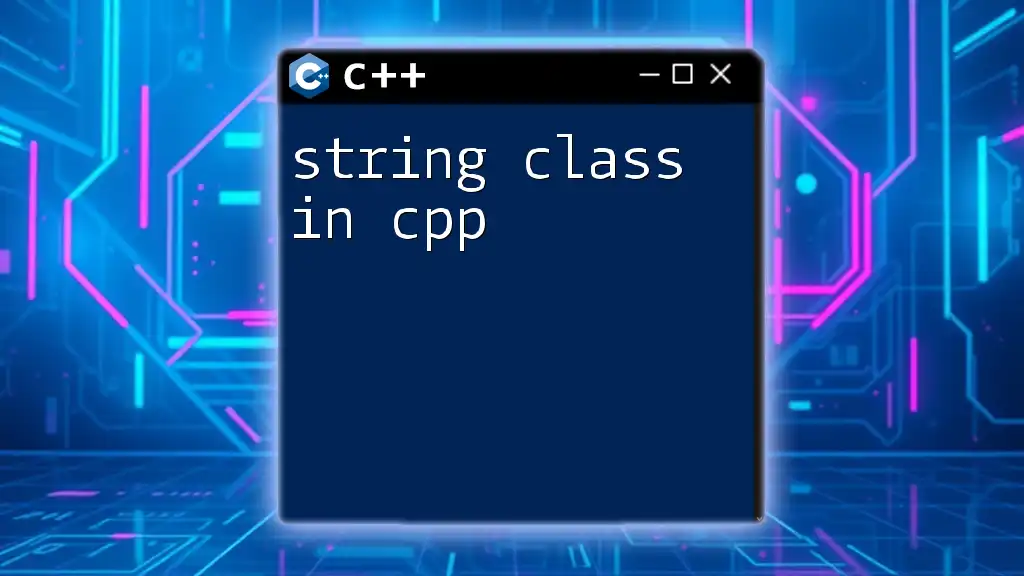
Class Components
Member Variables
Member variables, also known as attributes or properties, hold the state of the object. They represent the data associated with the class instance.
For example, using the `Car` class defined above, we might create an instance like this:
Car myCar;
myCar.make = "Toyota";
myCar.model = "Corolla";
myCar.year = 2022;
Member Functions
Member functions (methods) are functions defined inside a class that operate on its member variables. They define the behavior of the objects created from the class.
Here is an example of a member function:
class Car {
public:
void honk() {
cout << "Honk! Honk!" << endl;
}
};
Creating an instance of `Car` and calling the honk method:
Car myCar;
myCar.honk(); // Outputs: Honk! Honk!
Constructors and Destructors
Constructors are special member functions that are called when an object of the class is created, typically used for initializing member variables.
Destructors, in contrast, are called when an object goes out of scope and are used to release resources.
Defining Constructors in C++
Constructors can be explicitly defined to accept parameters:
class Car {
public:
string make;
string model;
int year;
Car(string m, string mod, int y) {
make = m;
model = mod;
year = y;
}
};
In this case, we create a `Car` object with a specific `make`, `model`, and `year` like so:
Car myCar("Honda", "Civic", 2023);
Defining Destructors in C++
Destructors are declared with a tilde (`~`) before the class name:
class Car {
public:
~Car() {
cout << "Car object is being deleted" << endl;
}
};
When the `Car` object goes out of scope, the destructor’s message will be printed, aiding in resource management during program execution.
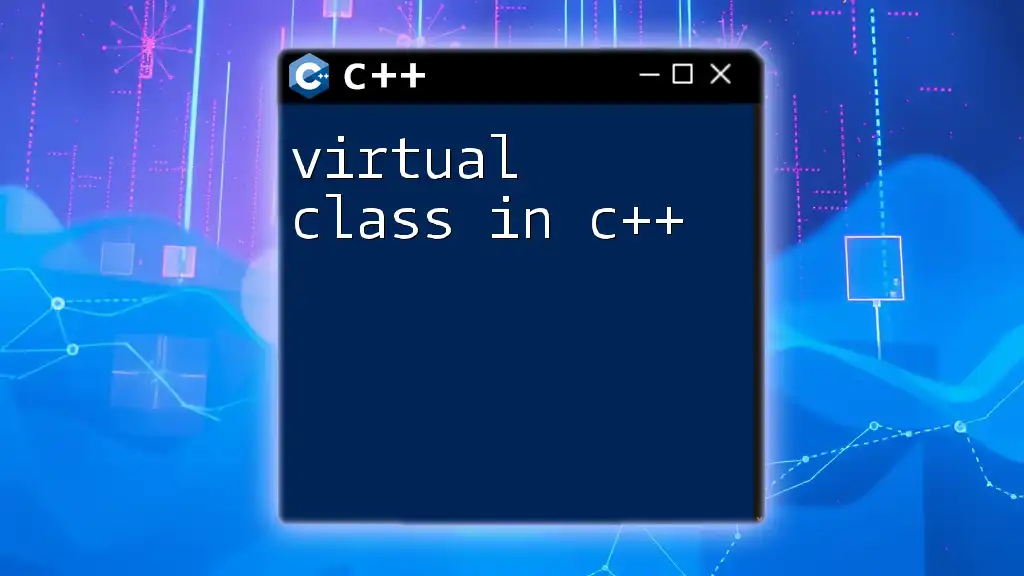
Access Modifiers in C++
Access Modifiers control the accessibility of class members. The three primary access modifiers are:
- Public: Members can be accessed from outside the class.
- Private: Members are only accessible within the class, ensuring encapsulation.
- Protected: Similar to private, but accessible within derived classes.
Example of Access Modifiers
Here’s an example demonstrating the use of access modifiers:
class Car {
private:
string make;
public:
void setMake(string m) {
make = m;
}
string getMake() {
return make;
}
};
In this example, `make` is a private member variable, and we provide public member functions to set and get its value, enforcing encapsulation.
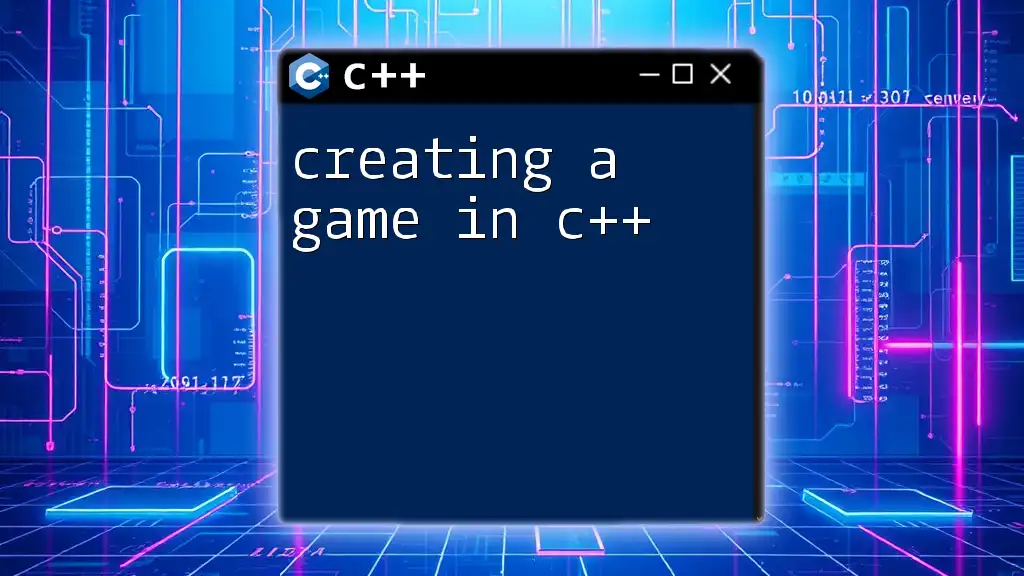
Inheritance in Classes
Inheritance enables one class to inherit characteristics and behaviors from another. This helps avoid redundancy, promoting code reusability.
What is Inheritance?
When one class (the derived class) inherits from another (the base class), it can access the base class's methods and attributes, offering a way to build upon existing classes.
Example of Inheritance
Consider a base class `Vehicle` and a derived class `Car`:
class Vehicle {
public:
void start() {
cout << "Vehicle starting..." << endl;
}
};
class Car : public Vehicle {
public:
void honk() {
cout << "Honk! Honk!" << endl;
}
};
In this case, `Car` inherits the `start` method from `Vehicle` while also having its own `honk` method. You could instantiate `Car` and call both methods:
Car myCar;
myCar.start(); // Outputs: Vehicle starting...
myCar.honk(); // Outputs: Honk! Honk!
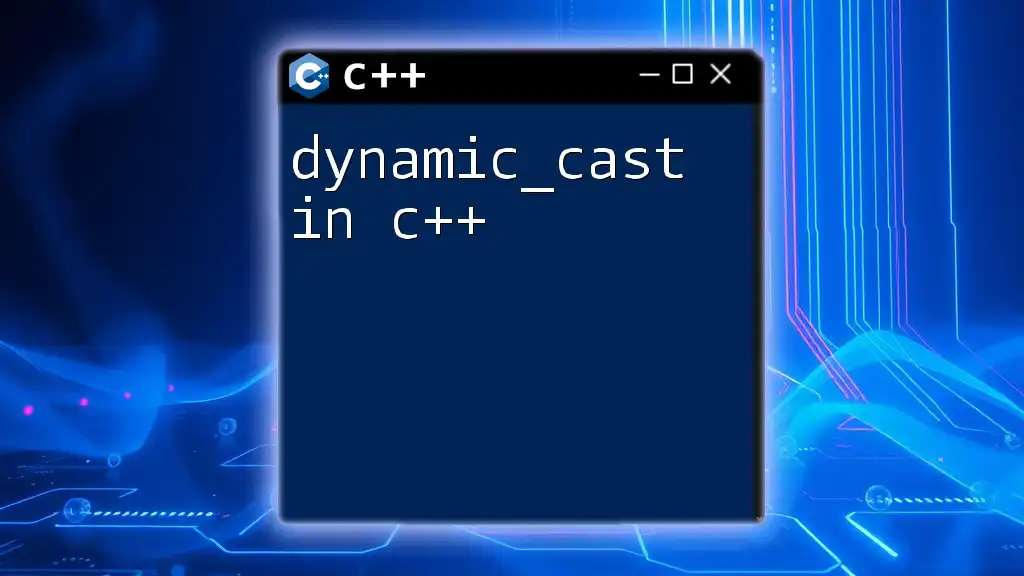
Polymorphism in Classes
Polymorphism allows methods to do different things based on the object doing the operation. It can be categorized into compile-time (overloading) and run-time (overriding) polymorphism.
What is Polymorphism?
In C++, polymorphism is often implemented using virtual functions for run-time polymorphism, where a base class reference can refer to a derived class object.
Example of Run-Time Polymorphism Using Virtual Functions
Here’s an illustration using a virtual function:
class Vehicle {
public:
virtual void sound() {
cout << "Some generic vehicle sound" << endl;
}
};
class Car : public Vehicle {
public:
void sound() override {
cout << "Vroom vroom" << endl;
}
};
When calling the `sound` method, the output varies according to the actual object type:
Vehicle* myVehicle = new Car();
myVehicle->sound(); // Outputs: Vroom vroom
Here, the `Car` class overrides the `sound` method, demonstrating polymorphism effectively.
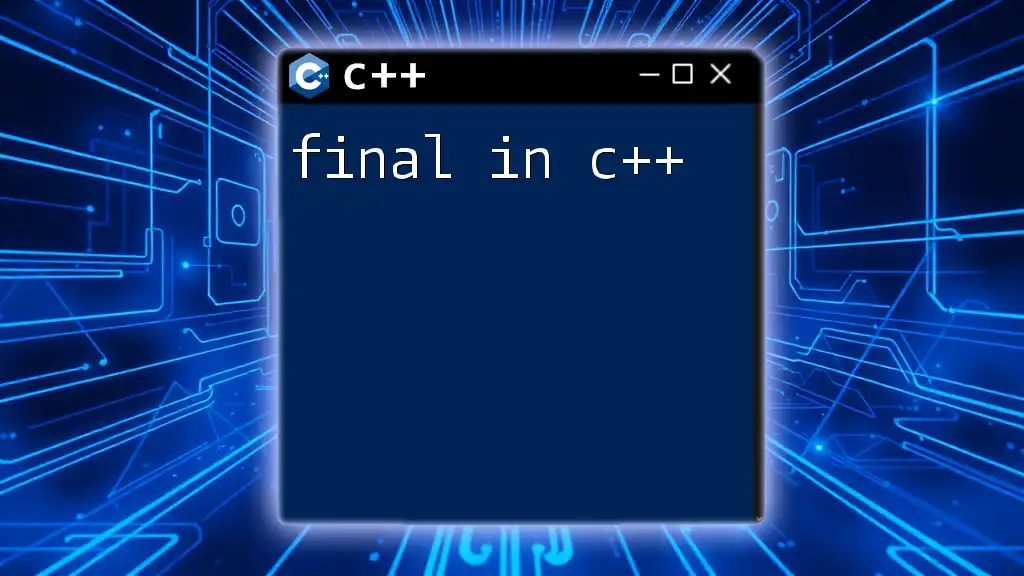
Conclusion
In summary, defining a class in C++ allows for a robust way to model complex data and behavior. From class declarations and member functions to the nuances of constructors, destructors, access modifiers, and OOP principles like inheritance and polymorphism, mastering these concepts is fundamental for effective C++ programming.
As you delve deeper into C++, consider exploring additional topics such as operator overloading and template classes to further enrich your understanding and capabilities in this versatile programming language.
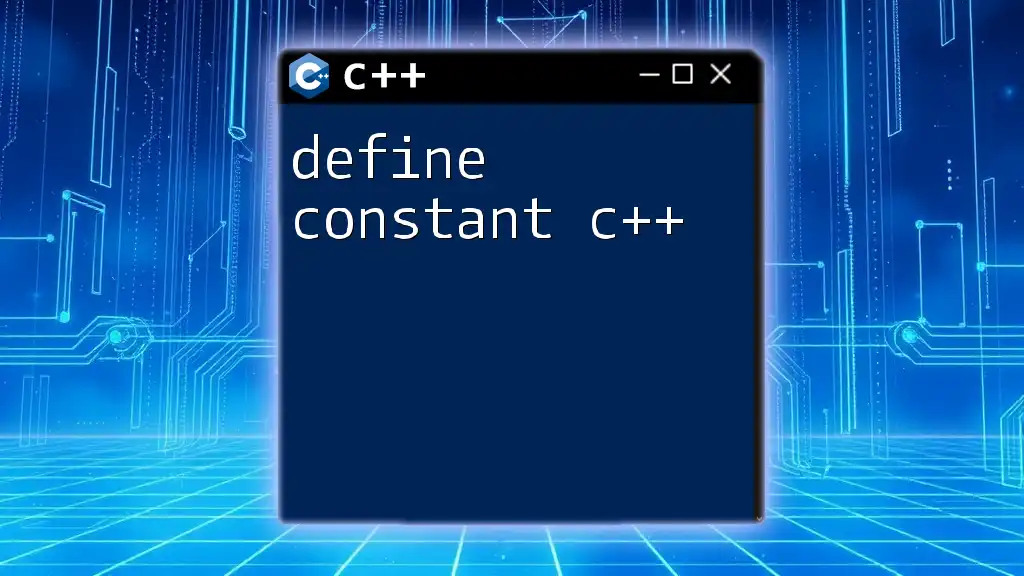
Frequently Asked Questions
What is the difference between a class and a structure in C++? A class is defined with the `class` keyword and has default private access, while a structure is defined with the `struct` keyword and has default public access.
Can a class have multiple constructors? Yes, a class can have multiple constructors, known as constructor overloading, allowing for different ways to initialize an object.
What happens if a destructor is not defined? If a destructor is not explicitly defined, C++ will provide a default destructor that automatically cleans up member variables for the object when it goes out of scope. However, if your class manages resources like dynamic memory, defining a destructor is crucial for proper resource management.