A virtual class in C++ is a class declared with the `virtual` keyword to facilitate polymorphism, allowing derived classes to override its methods for dynamic method resolution.
Here’s a simple code snippet demonstrating the use of a virtual class:
#include <iostream>
using namespace std;
class Base {
public:
virtual void show() {
cout << "Base class show function called." << endl;
}
virtual ~Base() {}
};
class Derived : public Base {
public:
void show() override {
cout << "Derived class show function called." << endl;
}
};
int main() {
Base* b = new Derived();
b->show(); // Calls the Derived class show function
delete b;
return 0;
}
Understanding Virtual Classes
What is a Virtual Class?
A virtual class in C++ serves as a mechanism that allows a derived class to override methods from a base class. The primary purpose of marking a function as virtual is to achieve runtime polymorphism, which enables the program to determine the correct function to invoke at runtime based on the type of the object being pointed to or referenced, rather than the type of reference or pointer itself.
Unlike abstract classes, which can contain pure virtual functions and cannot be instantiated, a virtual class can have both virtual and non-virtual members. An abstract method is declared by placing `= 0` after its declaration. Understanding these distinctions is crucial when designing systems that require dynamic behavior.
Why Use Virtual Classes?
Virtual classes are beneficial for several reasons:
- Achieving Polymorphism: They facilitate polymorphic behavior, allowing objects of different derived classes to be treated as objects of the base class.
- Extending Functionality: You can derive new classes from existing ones and add or modify functionalities in derived classes without altering the base classes.
- Ease of Maintenance: Virtual classes simplify code maintenance. With polymorphism, you can change or extend functionality without needing to completely rework existing code.
Real-world applications of virtual classes often include software simulations, graphics applications, and any system that requires dynamic behavior based on the object type.
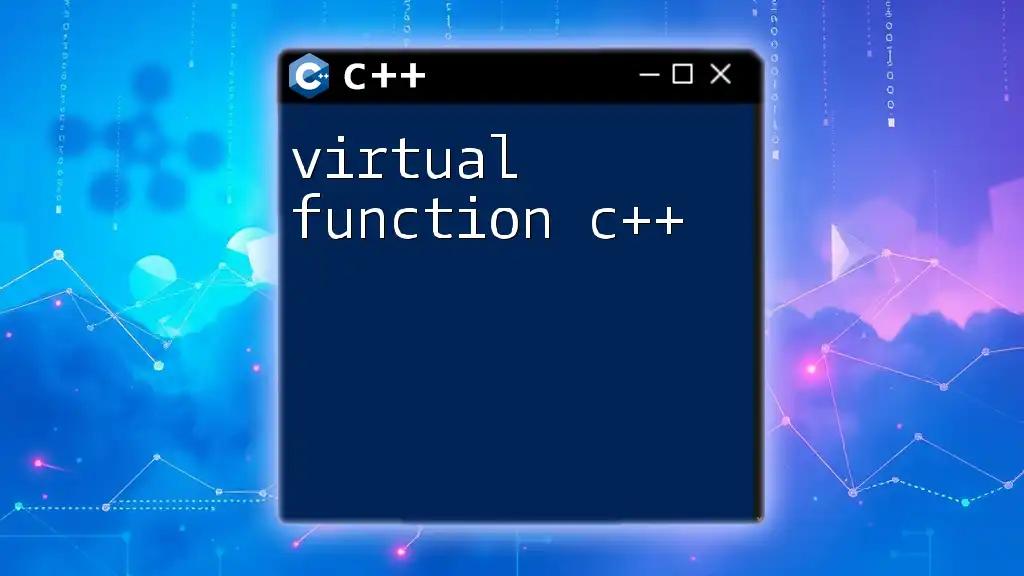
The Syntax of Virtual Classes
Declaring a Virtual Class
To declare a virtual function, the `virtual` keyword is used in the base class's function declaration. This signals to the compiler that this function may have a different implementation in a derived class.
Here's a simple example demonstrating this:
class Base {
public:
virtual void show() {
cout << "Base Class" << endl;
}
};
Implementing Virtual Methods
When implementing virtual methods in derived classes, you can use the `override` keyword to ensure that you are correctly overriding a base class method.
Consider the following implementation:
class Derived : public Base {
public:
void show() override {
cout << "Derived Class" << endl;
}
};
In this example, when a `Derived` object is treated as a `Base` object, calling `show()` will invoke the `Derived` class's implementation, thanks to the virtual keyword.

Polymorphism and Virtual Classes
Concept of Polymorphism
Polymorphism allows methods to do different things based on the object it is acting upon. In C++, polymorphism is primarily achieved through virtual functions. With virtual functions, the program can decide at runtime which function to invoke based on the actual object type rather than the pointer type.
For instance, if you have a pointer pointing to the base class, but it refers to a derived class object, calling a virtual function will invoke the derived class's version.
Run-time vs Compile-time Polymorphism
There are two types of polymorphism in C++: compile-time and run-time. Compile-time polymorphism is achieved through function overloading and templates, while run-time polymorphism is accomplished via virtual functions and inheritance.
Virtual classes specifically help in realizing run-time polymorphism. They allow the decision of which function to call to be made at runtime, thus enabling more flexible and reusable code.
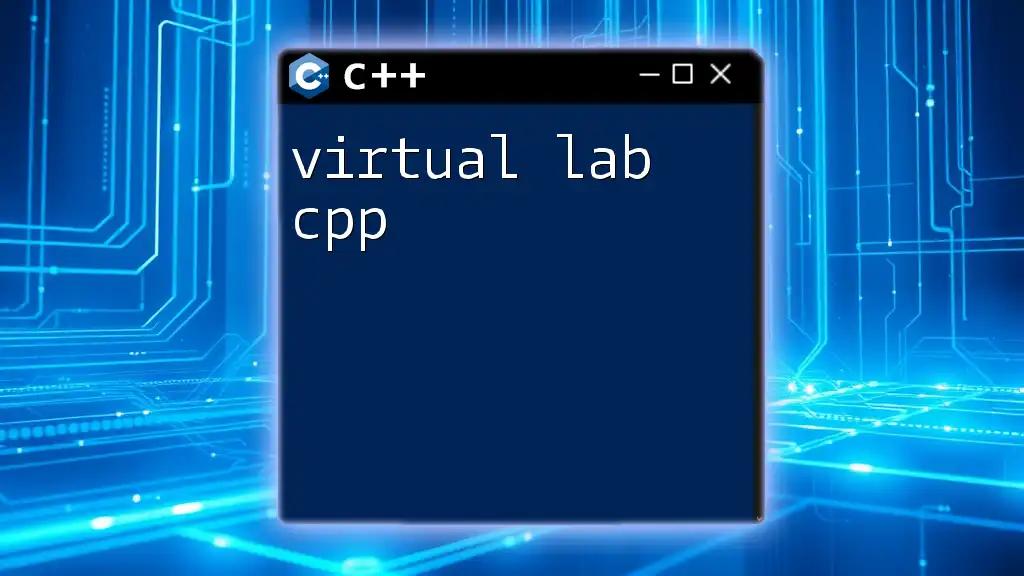
Practical Examples of Virtual Classes
Example 1: Vehicle Hierarchy
Let's consider a hierarchy of `Vehicle` classes with both a base class and several derived classes. This setup can help illustrate the utility of virtual functions.
class Vehicle {
public:
virtual void start() {
cout << "Vehicle starting" << endl;
}
};
class Car : public Vehicle {
public:
void start() override {
cout << "Car starting" << endl;
}
};
class Motorcycle : public Vehicle {
public:
void start() override {
cout << "Motorcycle starting" << endl;
}
};
In this example, each vehicle type can override the `start()` method to provide a specific implementation. When a pointer or reference of type `Vehicle` points to a `Car` or `Motorcycle`, the correct `start()` method will be called based on the actual object type.
Example 2: Shape Area Calculation
Another commonly used scenario for virtual classes is in geometric shapes where you might need to calculate the area of different shapes.
Here's a simple implementation:
class Shape {
public:
virtual double area() = 0; // Pure virtual function
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() override {
return 3.14 * radius * radius;
}
};
class Rectangle : public Shape {
private:
double width, height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double area() override {
return width * height;
}
};
In this example, the `Shape` class contains a pure virtual function called `area()`. It forces all derived classes (like `Circle` and `Rectangle`) to provide their own implementations of this function, thus achieving a common interface for calculating areas.
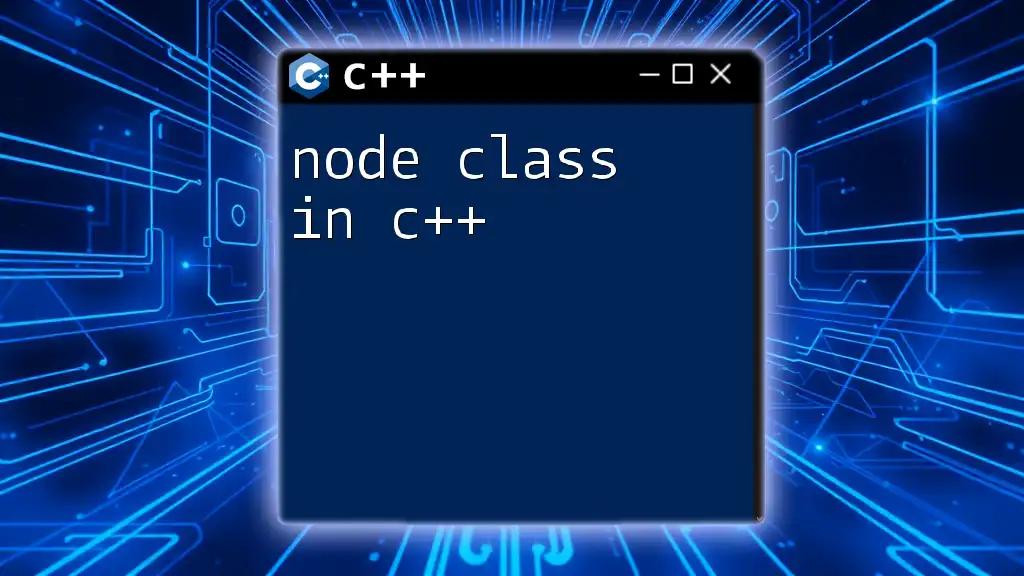
Best Practices for Using Virtual Classes
Tips for Implementing Virtual Classes
- Use Virtual Functions Judiciously: Implement virtual functions only when necessary, as they introduce a small runtime overhead.
- Declare Destructors Virtual: Ensure that if any class has virtual functions, its destructor should also be virtual. This allows derived classes to clean up their resources properly.
- Utilize `override` and `final` Specifiers: Use the `override` specifier for clarity and error checking. You can also use `final` to prevent further overriding in derived classes.
Common Mistakes to Avoid
-
Forgetting to Mark Destructors as Virtual: If a base class has virtual functions, it must have a virtual destructor to avoid memory leaks when deleting derived objects through base class pointers.
-
Ignoring the Benefits of `override`: Not using `override` can lead to subtle bugs, as there may be cases where you intend to override a function, but the function signature doesn’t match the base class.

Conclusion
Virtual classes in C++ are instrumental in implementing polymorphism, which enhances flexibility and maintainability in software design. By understanding how to declare and implement virtual classes and methods, developers can create robust systems that cater to diverse requirements and behaviors.
As you gain experience with C++, practicing the concepts introduced in this guide will solidify your grasp of virtual classes. Consider exploring C++ further through other complex scenarios and challenges.

Additional Resources
To expand your knowledge, consider reading the following:
- Recommended books for advanced C++ programming.
- Online platforms that provide C++ tutorials and courses.
- Forums and communities where seasoned C++ developers can share insights and expert advice.

Call to Action
Share your experiences with virtual classes in C++! What challenges have you faced, and how did you overcome them? Let’s explore this fascinating programming paradigm together, and don’t hesitate to leverage the resources available through our platform for further learning.