In C++, you can create an array of objects from a class to manage multiple instances in a structured way, as shown in the following example:
class MyClass {
public:
MyClass(int val) : value(val) {}
int getValue() { return value; }
private:
int value;
};
int main() {
MyClass myArray[3] = { MyClass(1), MyClass(2), MyClass(3) };
for (int i = 0; i < 3; i++) {
std::cout << myArray[i].getValue() << std::endl; // Outputs: 1, 2, 3
}
return 0;
}
Understanding Classes in C++
What is a Class?
A class in C++ is a blueprint for creating objects. It encapsulates data for the object and methods to operate on that data.
Example: Here's a simple class definition:
class Car {
public:
string brand;
int year;
void displayInfo() {
cout << "Brand: " << brand << ", Year: " << year << endl;
}
};
Class Features
Classes typically have attributes (data members) and methods (functions that operate on the data).
Code Snippet: A more detailed representation of a class with attributes and methods can be seen in the following example:
class Rectangle {
private:
double length;
double width;
public:
Rectangle(double l, double w) : length(l), width(w) {}
double area() {
return length * width;
}
};
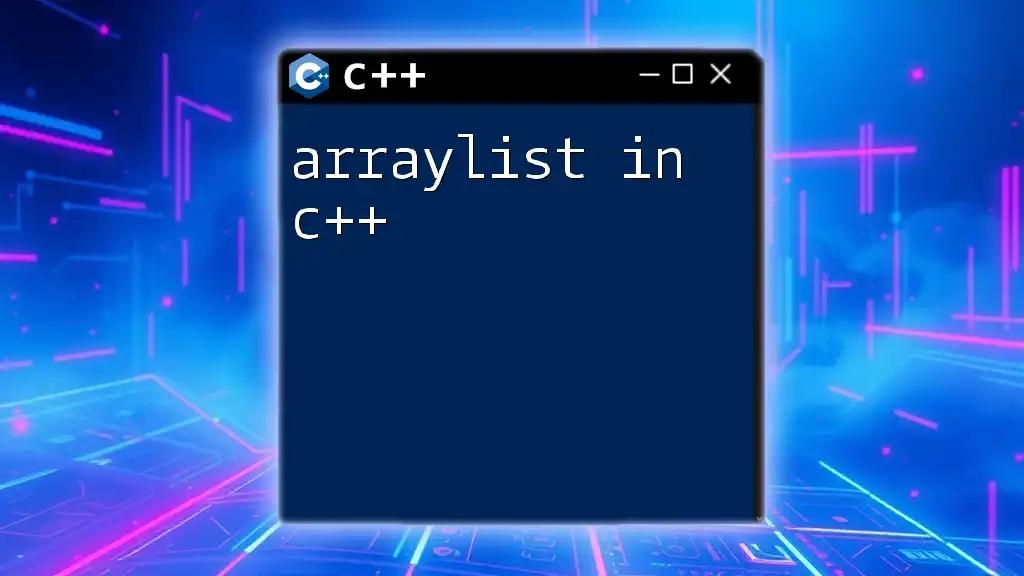
What are Arrays in C++?
Definition of Arrays
An array is a collection of items stored at contiguous memory locations. The most common usage of arrays is to store data of the same type.
Declaring and Initializing Arrays
To declare an array, use the following syntax:
int numbers[5]; // Declares an array of 5 integers
You can also initialize an array upon declaration:
int numbers[5] = {1, 2, 3, 4, 5};
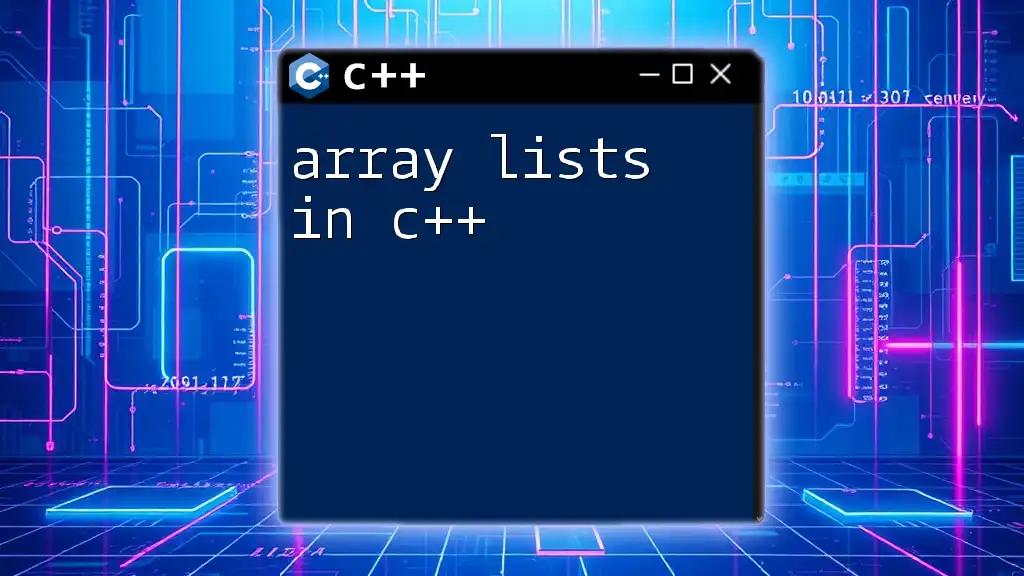
Array of Classes in C++
What is an Array of Classes?
An array of classes allows you to store multiple objects of the same class type in contiguous memory locations. This approach is beneficial when you want to manage collections of similar objects.
Declaring an Array of a Class
Declare an array of class objects by specifying the class name, followed by the array size:
Car cars[3]; // Declares an array to hold three Car objects
Accessing Class Objects within an Array
Accessing Attributes
To access and modify the attributes of class objects stored in an array, use the indexing operator:
cars[0].brand = "Toyota";
cars[0].year = 2022;
Calling Methods
You can also call methods on objects in the array:
cars[0].displayInfo(); // This will output: Brand: Toyota, Year: 2022

Class with Array in C++
Defining a Class that Contains an Array
A class can encapsulate an array as its member. For instance:
class Classroom {
public:
string students[30]; // An array to hold student names
int count;
Classroom() : count(0) {}
void addStudent(const string &name) {
if (count < 30) {
students[count++] = name;
}
}
void displayStudents() {
for (int i = 0; i < count; i++) {
cout << students[i] << endl;
}
}
};
Common Operations on a Class Array
Adding Elements to the Array
In the above example, the `addStudent` method allows you to add a new student's name to the array while keeping track of the count.
Iterating Through the Array
To iterate through elements within a class array, use a loop. In this example, the `displayStudents` method performs this task:
for (int i = 0; i < count; i++) {
cout << students[i] << endl;
}
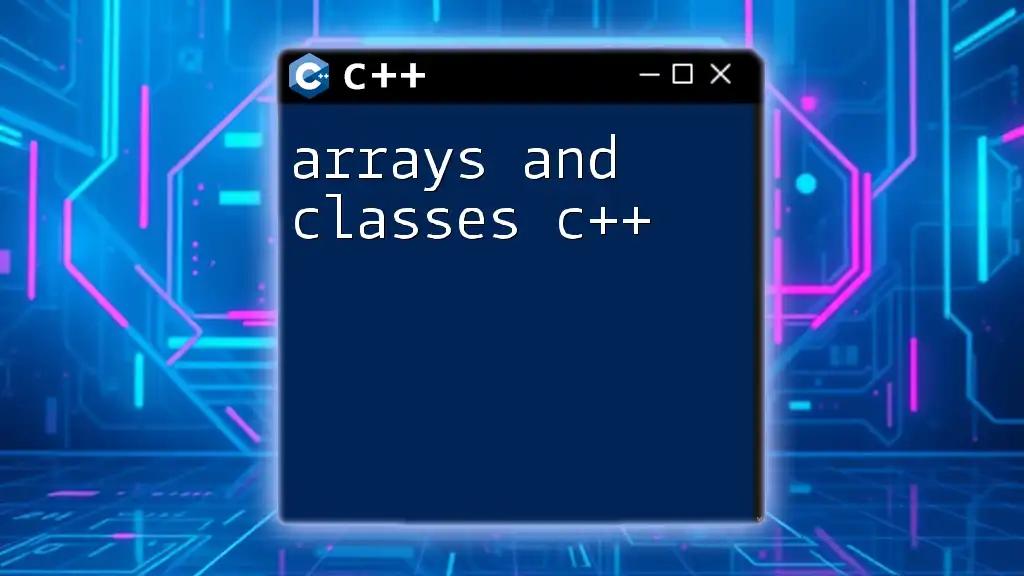
Best Practices for Using Arrays with Classes
Memory Management
When using arrays in classes, be conscious about memory management. Dynamic arrays using pointers can be more flexible than static arrays. Use dynamic memory allocation with `new` and `delete` to manage memory properly.
Encapsulation
Encapsulating array data within classes ensures that the data is shielded from outside interference. This is an essential principle of OOP (Object-Oriented Programming). For instance, instead of allowing direct access to the array, provide member functions that serve as an interface.
Example: As demonstrated in the `Classroom` class, operations are performed through methods rather than directly accessing the internal array.
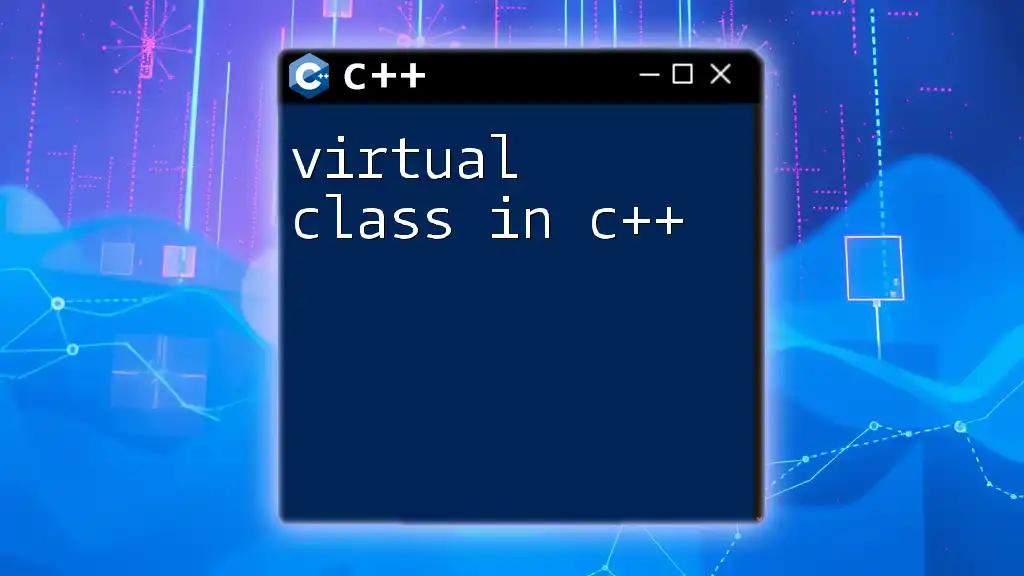
Real-world Applications
Use Cases of Class Arrays
- Gaming: Use arrays of class objects such as `Player`, `Enemy`, etc., to manage multiple characters in a game.
- Data Management: Arrays of `Record` class objects can help manage entries in databases.
- Simulations: In simulations, you may need to create multiple instances of a class that represents objects in a simulated environment.

Conclusion
In summary, combining arrays with classes in C++ provides powerful ways to manage related data efficiently. Whether you are storing multiple objects or encapsulating an array within a class, leveraging the concept of relationship between arrays and classes can significantly enhance your programming techniques.
Call to Action
As you explore the world of C++, don’t hesitate to experiment with these concepts. Try building your own classes and arrays to deepen your understanding and mastery of the language.

Additional Resources
For further learning about C++ arrays and classes, consider exploring online courses, tutorials, or C++ programming books that delve into object-oriented programming.

FAQ Section
Common questions regarding arrays and classes in C++ may include:
- What happens if I exceed the bounds of an array?
- How do I implement a dynamic array in C++?
- Can I store different types of classes in the same array?
Providing concise and informative answers can clarify these queries for beginners and help solidify their understanding.