In C++, binary literals allow you to express integer values directly in binary format by prefixing them with `0b` or `0B`.
Here's an example code snippet:
#include <iostream>
int main() {
int binaryValue = 0b101010; // Binary literal for decimal 42
std::cout << "The decimal value of binary 101010 is: " << binaryValue << std::endl;
return 0;
}
Understanding Binary Representation
Why Use Binary Literals?
Binary literals in C++ offer several advantages, particularly in scenarios involving bit manipulation. When programmers need to represent numbers in a form that is closely aligned with how data is structured at the machine level, binary literals become invaluable. Using them makes the code clearer and more maintainable, especially when dealing with specific bits within a byte.
Binary literals also provide a more straightforward representation compared to decimal or hexadecimal systems, particularly for those who are accustomed to thinking in binary. For instance, the number 10 can be represented as `0b1010` in binary, while its hexadecimal equivalent is `0xA`. This often leads to reduced errors during programming, particularly in low-level or performance-critical applications.
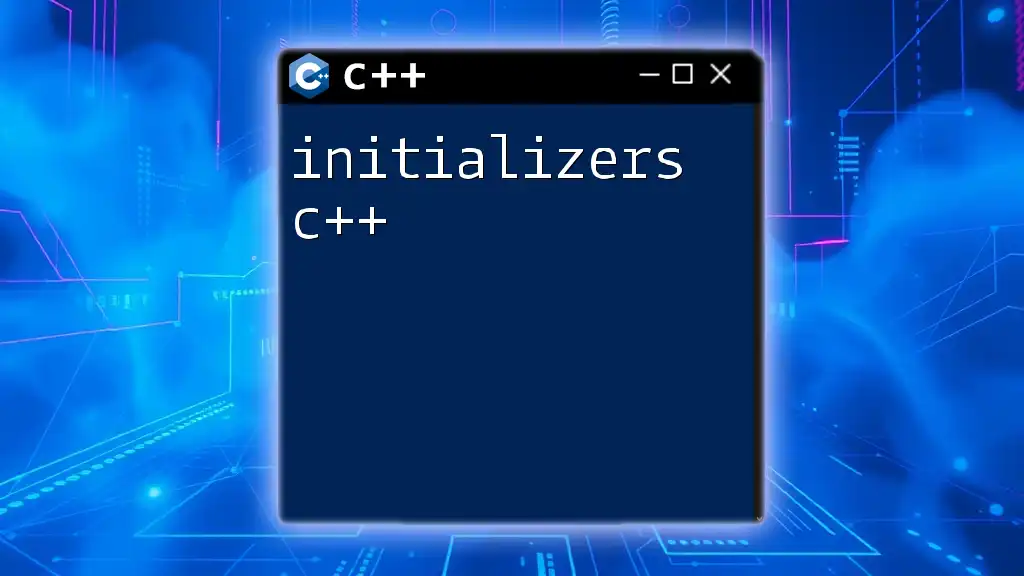
Syntax and Notation of Binary Literals in C++
The Binary Literal Prefix
In C++, binary literals are introduced using the prefixes `0b` or `0B`. This notation indicates to the compiler that the subsequent digits represent a binary value.
For example, you can declare binary literals as follows:
int binaryNumber1 = 0b1010; // Equals 10 in decimal
int binaryNumber2 = 0B1101; // Equals 13 in decimal
These declarations allow you to work with binary numbers directly, which can be especially useful for specific programming tasks, such as defining bit masks or configuring flags.
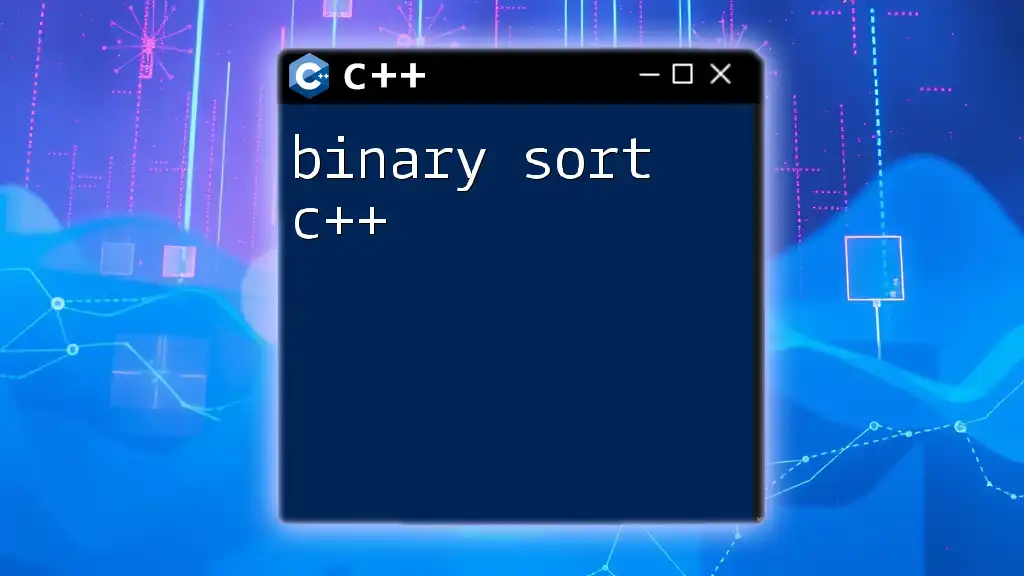
Using Binary Literals in Your Code
Binary Literals in Arithmetic Operations
Binary literals can be seamlessly integrated into arithmetic expressions. They behave like any other numeric type in C++, which allows for straightforward calculations.
For instance:
int a = 0b1010; // 10
int b = 0b0101; // 5
int sum = a + b; // 15 (in decimal)
This example illustrates how you can use binary literals in arithmetic operations, making the code both concise and intuitive.
Bitwise Operations with Binary Literals
Binary literals shine when it comes to bitwise operations, which manipulate specific bits within integers. C++ supports a range of bitwise operators, including AND (`&`), OR (`|`), NOT (`~`), and XOR (`^`).
Consider this example demonstrating a bitwise AND operation:
int x = 0b1100; // 12 in decimal
int y = 0b1010; // 10 in decimal
int result = x & y; // Result is 0b1000 (8 in decimal)
In this example, `x` and `y` are binary literals, and the result demonstrates how using binary values can simplify operations at the bit level.
Representing Data with Binary Literals
Setting and Clearing Bits
By leveraging binary literals, programmers can easily manipulate specific bits within a number. Setting and clearing bits is a common task in embedded programming and hardware interfacing.
Here's how you can set and clear bits using binary literals:
int flags = 0b0000; // All flags off
flags |= 0b0001; // Set first bit to 1
flags &= ~0b0001; // Clear first bit back to 0
These operations allow developers to manage flags efficiently without having to convert between decimal and binary, enhancing readability and reducing complexity.
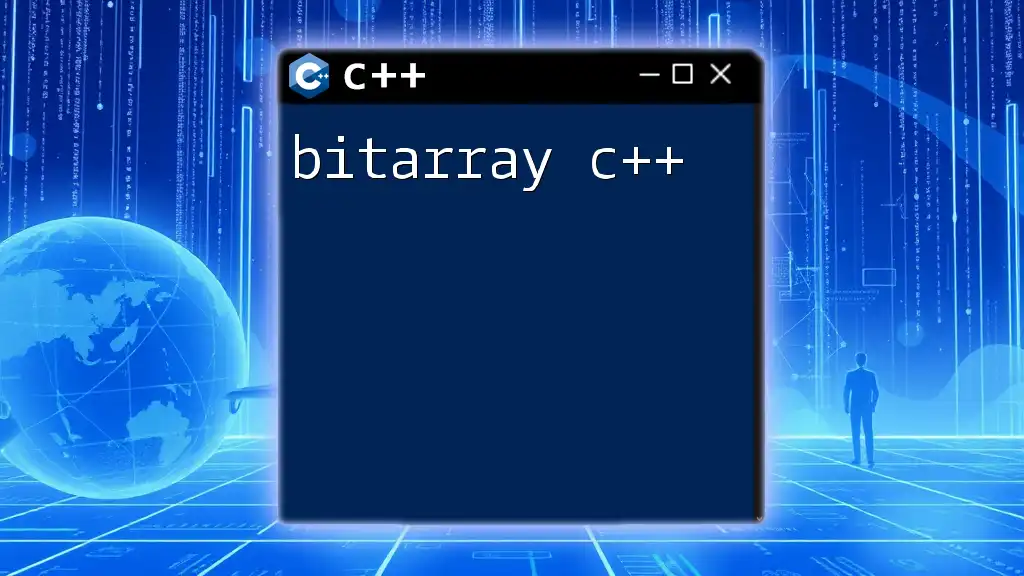
Limitations and Considerations
Compatibility with Older Versions of C++
Binary literals were introduced in C++14. Therefore, if you are working with compilers that do not support this version or later, you will need to rely on hexadecimal or decimal representations instead. This effectively limits the use of binary literals to environments where modern C++ standards are in play.
Performance Implications
While using binary literals can make code more readable and easier to manage, it's important to consider whether this clarity impacts performance. Generally, the performance of operations involving binary literals is closely comparable to operations performed with other numeric types. However, overusing direct binary representations might lead to readability concerns, making it essential to strike the right balance between performance and clarity.
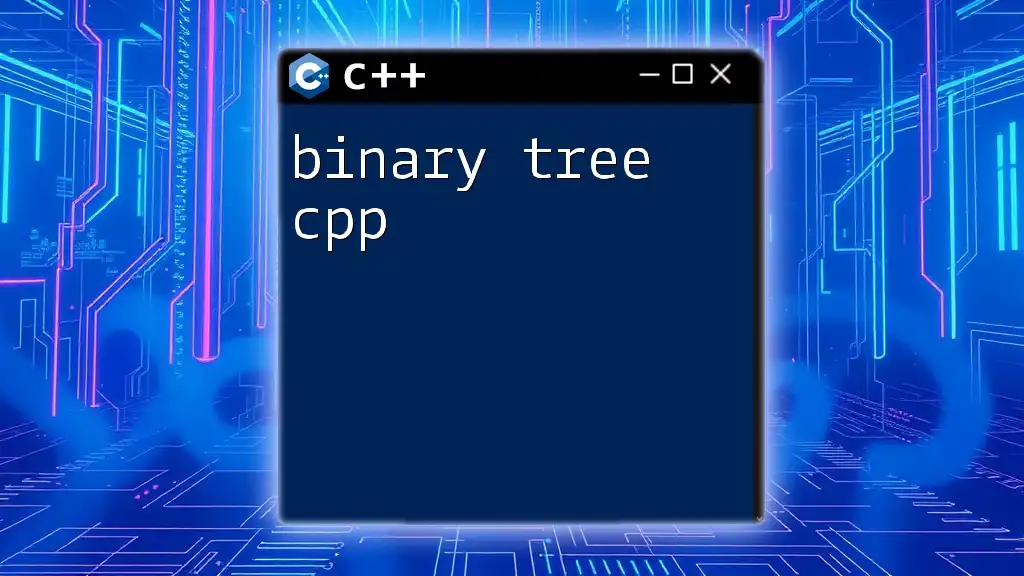
Common Use Cases for Binary Literals
Digital Logic and Bit Manipulation
Binary literals are commonly employed in digital logic designs and low-level programming tasks. When configuring registers or interacting directly with hardware, using binary literals allows for a clear representation of the required settings.
For example, setting specific bits in a GPIO register or configuring communication protocols can be achieved with just a few lines of code, ensuring that key bits are accurately represented.
Configuration Flags
Using binary literals for feature flags in applications is another classic use case. In a configuration settings context, developers can easily turn on and off specific features by manipulating individual bits without having to think about more verbose numeric systems.
Network Protocols
Binary literals are also prominent in low-level network programming. When defining headers or flags in communication protocols, binary representation permits easier manipulation of bits that are critical for communication.
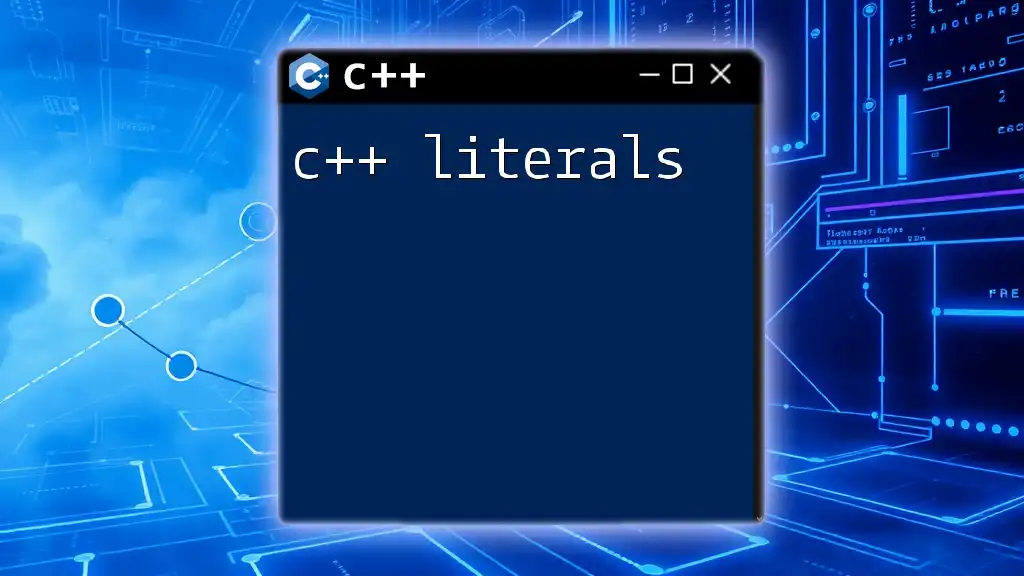
Best Practices for Using Binary Literals
When using binary literals, it’s crucial to understand when their use is most appropriate compared to other numeral systems. Clarity should take precedence over brevity; if using binary literals leads to confusion, alternative representations might be preferable.
For example, operations performed on masks or configurations are excellent candidates for binary literals, while purely arithmetic operations might be better served with decimal or hexadecimal representations.
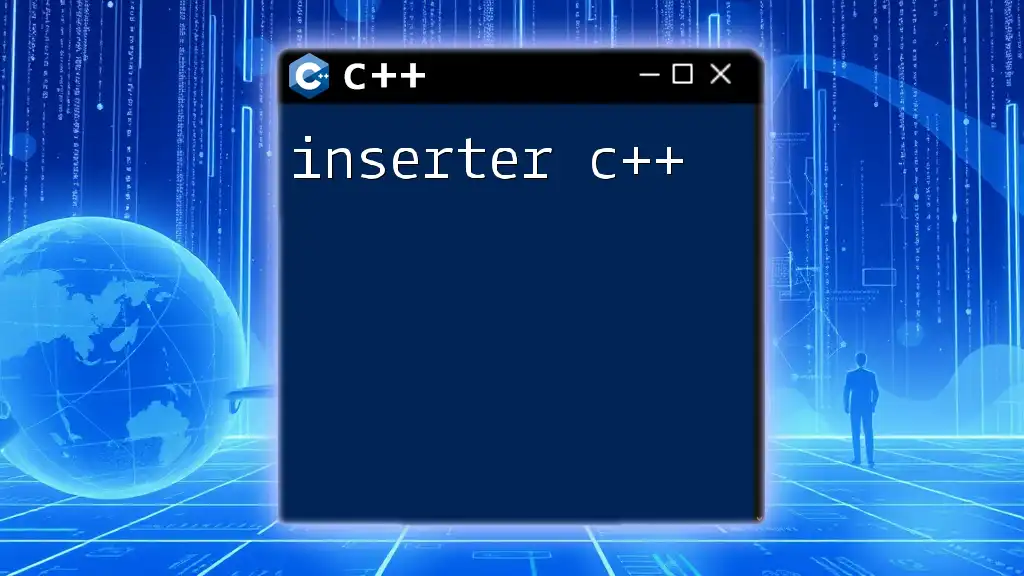
Conclusion
Binary literals in C++ provide a powerful and clear way to represent binary numbers directly in your code. By adopting binary literals, you can improve readability and enhance maintainability, especially in applications where bit-level manipulation is common. Embrace binary literals not only for their utility but also for the clarity they bring to your code, ensuring that your programming practices remain concise and effective.