Binary sort, commonly implemented as binary search, efficiently finds the position of a target value within a sorted array by repeatedly dividing the search interval in half.
Here’s a simple C++ code snippet demonstrating binary search:
#include <iostream>
using namespace std;
int binarySearch(int arr[], int size, int target) {
int left = 0, right = size - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1; // Target not found
}
int main() {
int arr[] = {1, 2, 3, 4, 5, 6};
int target = 4;
int result = binarySearch(arr, 6, target);
cout << (result != -1 ? "Element found at index: " + to_string(result) : "Element not found") << endl;
return 0;
}
What is Binary Sort?
Binary sort, often referred to in the context of binary search algorithms, is a vital algorithm utilized for organizing data into a specific order, usually ascending or descending. Sorting is a fundamental operation in computer science, enhancing data retrieval and manipulation efficiency. While many sorting algorithms exist, binary sort stands out due to its simplicity and effectiveness for specific applications. The fundamental principle of sorting is to rearrange elements in a list so that they follow a defined sequence, which allows for easier and faster data access.
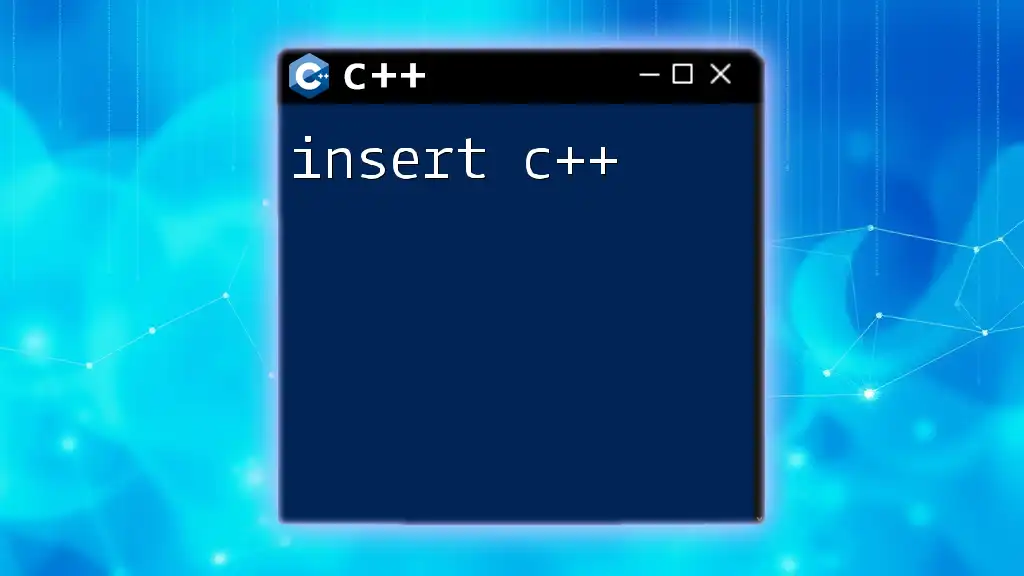
Overview of Sorting Algorithms
Sorting algorithms come in various forms, each with its characteristics and performance outcomes. Commonly known algorithms include:
- Bubble Sort
- Selection Sort
- Insertion Sort
- Merge Sort
- Quick Sort
Binary sort is a term often used interchangeably with binary insertion sort, which is an efficient form of insertion sort utilizing binary search to find the location of elements. This unique approach enhances the sorting process, particularly when dealing with numerous elements.
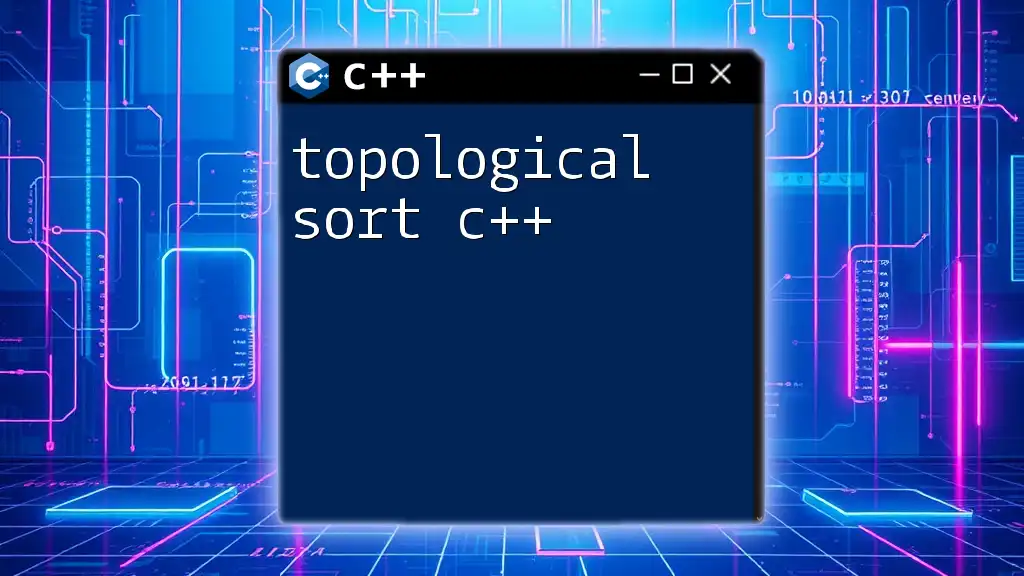
Understanding Binary Search vs. Binary Sort
Defining Binary Search
To grasp the concept of binary sort, it's essential first to understand binary search. Binary search is an algorithm used for finding an element's position within a sorted array. It operates under the principle of divide-and-conquer, repeatedly dividing the search interval in half until the target value is found or the interval is empty.
It is important to note that binary search only functions on sorted data. Thus, creating an ordered list through an efficient sorting algorithm like binary sort is crucial before performing a binary search.
Binary Sort Explained
When contrasting binary search with binary sort, it is evident that binary search identifies the position of an item, whereas binary sort organizes data before searching.
In essence, binary sort assists in preparing data for efficient searching and retrieval—notably in cases where the data is frequently read but infrequently modified. Its implementation can significantly reduce the time complexity of search operations, leading to improved performance in data-intensive applications.
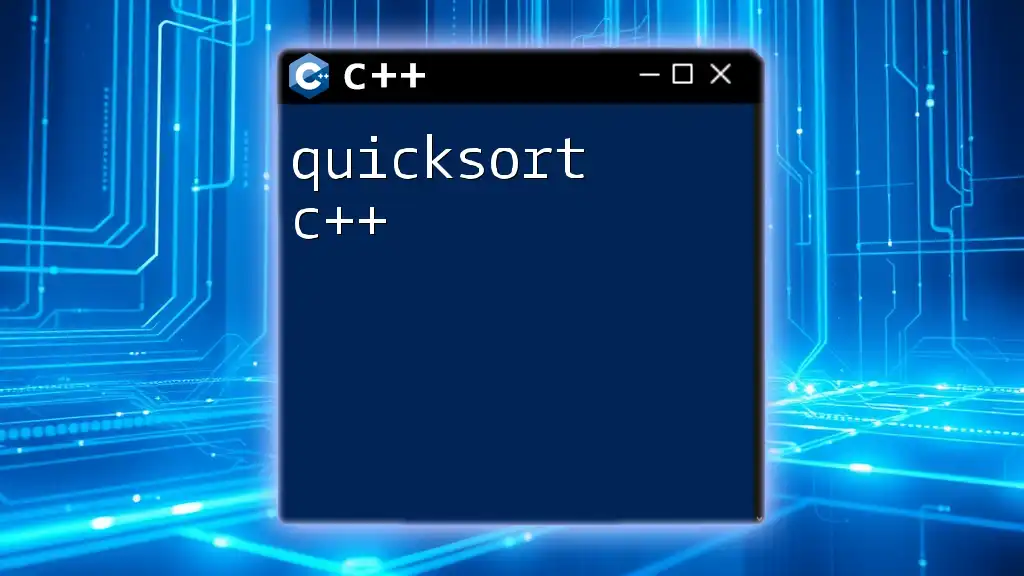
Key Concepts in Binary Sort
Sorting Mechanisms
Binary sort operates based on two main principles: in-place sorting and comparison-based sorting.
-
In-place sorting means the algorithm requires a small, constant amount of additional memory space. This factor is a significant advantage when space conservation is imperative.
-
Comparison-based sorting implies that elements are ordered by comparing them to one another, which is a fundamental behavior of algorithms such as binary sort.
Time Complexity
In discussing the performance of binary sort, it's crucial to break down its time complexity. The average time complexity of binary insertion sort is O(n^2) due to the nested loops typically involved in the insertion process. However, the binary search optimization reduces the number of comparisons needed to find the correct position for each element.
When analyzing the effectiveness of binary sort compared to other algorithms, it's worth noting that while binary sort may not outperform more advanced techniques in terms of time complexity, its simplicity and ease of implementation make it an appealing choice for smaller datasets or where simplicity is prioritized.
Stability in Sorting Algorithms
The concept of stability in sorting algorithms indicates whether or not two equivalent elements retain their original order relative to one another. Binary sort is considered a stable sorting algorithm, meaning that it preserves the relative order of equal elements. This feature is especially useful in situations where data entries contain multiple fields, ensuring coherence in sorted output.
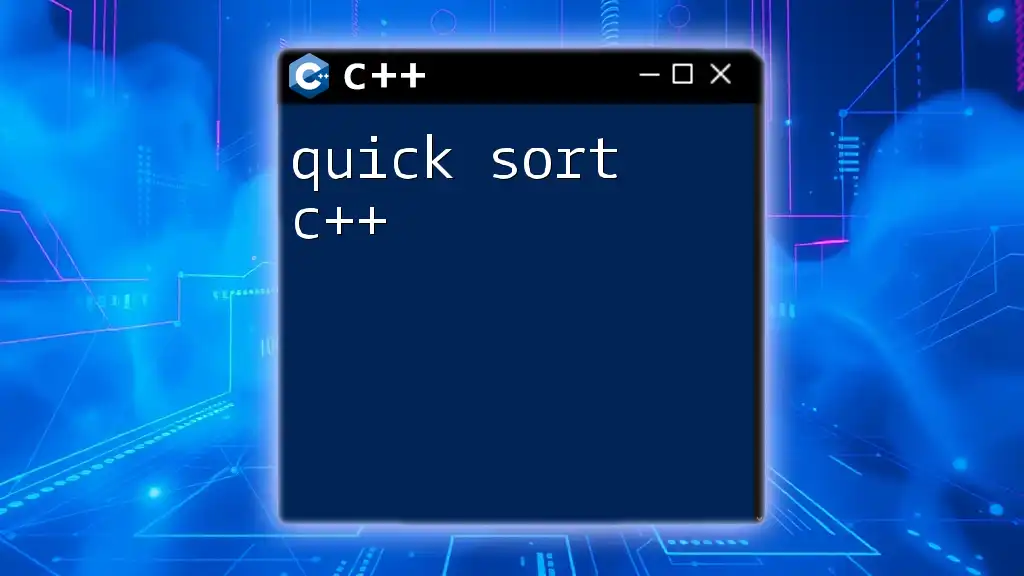
Implementing Binary Sort in C++
Setting Up Your Environment
Before diving into coding binary sort, you’ll need to set up your development environment. A C++ compiler and an integrated development environment (IDE) like Visual Studio, Code::Blocks, or even online compilers can provide the tools necessary for running C++ code effectively.
Writing the Binary Sort Function
The basic implementation of binary sort hinges on a series of comparisons and insertions into a sorted segment of the array. Below is a simple demonstration of what that function may look like.
void binarySort(int arr[], int n) {
// Iterate through elements in the array
for (int i = 1; i < n; i++) {
int key = arr[i]; // Current element to be positioned
int j = i - 1; // Index of the last sorted element
// Find the correct position for the key
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j]; // Shift larger elements to the right
j = j - 1; // Move to the next element
}
arr[j + 1] = key; // Insert the key at its correct position
}
}
Understanding the Code
The implementation begins by defining a function named `binarySort` that accepts an array and its size as parameters. Within the function, a loop iterates through each element. The variable `key` stores the current element being examined, while `j` tracks the last element of the sorted subarray.
The inner while loop shifts larger elements to the right until the correct position for the `key` is found, allowing for a precise insertion into the sorted portion of the array. Once the insertion logic is executed, the `key` is placed at its suitable index.
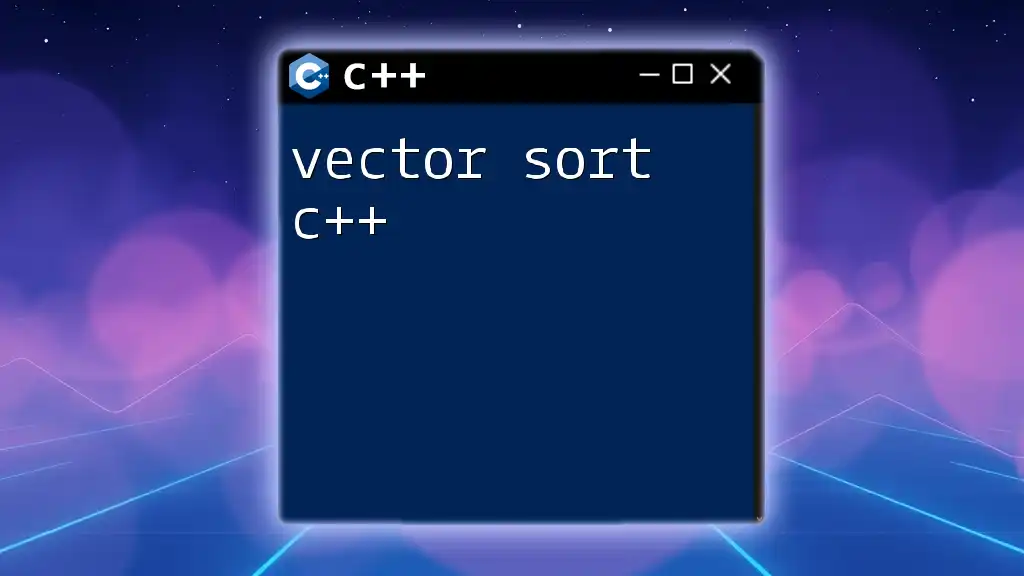
Example of Binary Sort in Action
Sample Data Set
Consider the unsorted array: `{34, 7, 23, 32, 5, 62}`. We will apply binary sort to this set.
Applying Binary Sort
Once we implement the binary sort on the array, we can see how it organizes the elements efficiently.
Here’s the complete implementation, including the `main` function to execute the sort:
#include <iostream>
using namespace std;
void binarySort(int arr[], int n) {
// Implement the binary sort logic
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j = j - 1;
}
arr[j + 1] = key;
}
}
int main() {
int arr[] = {34, 7, 23, 32, 5, 62};
int n = sizeof(arr) / sizeof(arr[0]);
binarySort(arr, n);
cout << "Sorted array: \n";
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
return 0;
}
Output Explained
Upon execution, the program sorts the array and displays the sorted output: `5 7 23 32 34 62`. This process illustrates how binary sort successfully organizes a list of unsorted integers, providing an example of the algorithm in action.
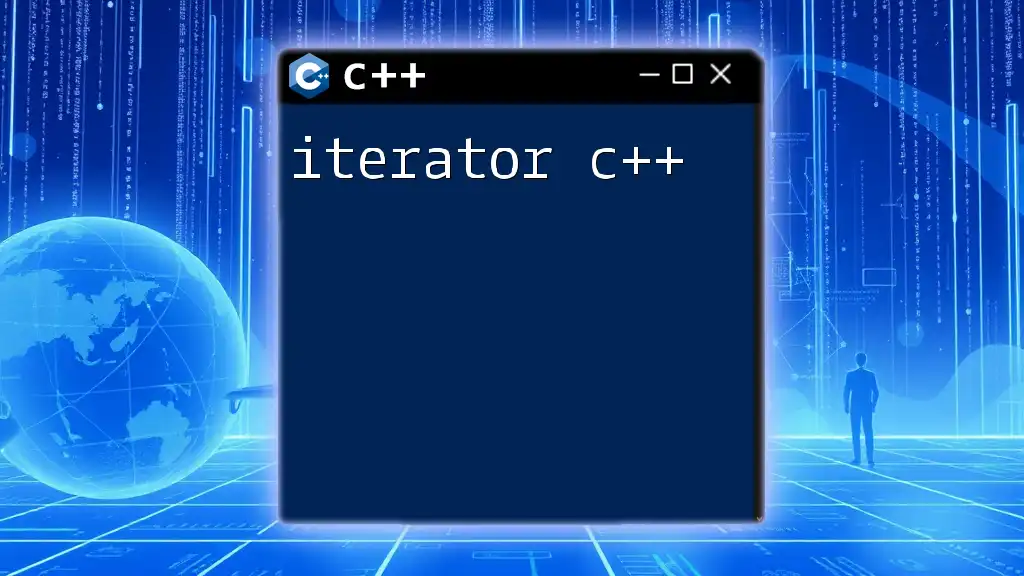
Common Mistakes and Troubleshooting
Common Errors While Implementing Binary Sort
As you implement binary sort, be aware of common pitfalls that may lead to erroneous outputs. Misplacing elements during insertion, misunderstanding array bounds, or neglecting to account for duplicate values can all skew your results.
Performance Optimization Tips
To enhance the performance of binary sort, consider optimizing data storage or utilizing hybrid algorithms such as Timsort for larger datasets. Understanding when to deploy binary sort versus other more sophisticated sorting algorithms is crucial in maintaining optimal efficiency.
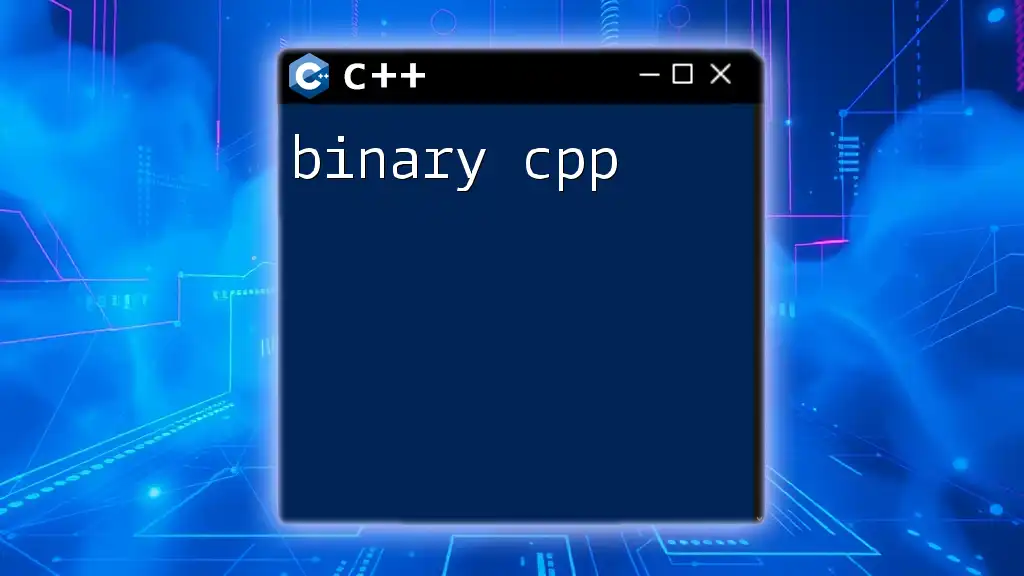
Conclusion
In this guide, we explored the fundamental concepts of binary sort in C++. We delved into implementation details, common challenges, and the underlying principles that inform this sorting algorithm's functionality.
As you continue your journey in C++ programming and algorithm design, practicing with binary sort will enrich your understanding of efficient data organization.
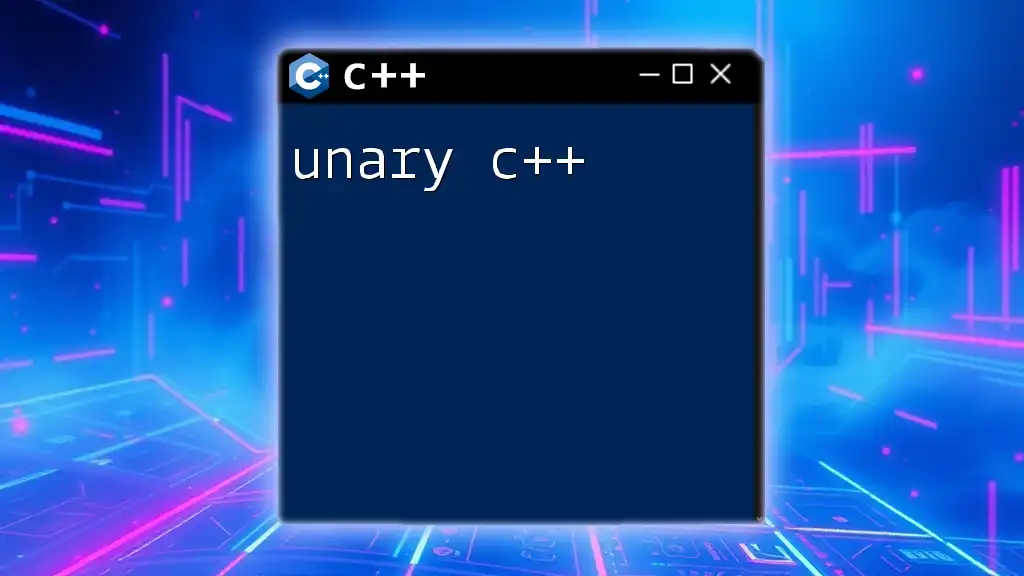
Call to Action
We encourage you to explore binary sort yourself! Implement the code snippets provided, experiment with different datasets, and observe how the sorting process unfolds. Share your experiences or questions in the comments below! Happy coding!