The `<cassert>` header in C++ provides a macro to facilitate diagnostics by allowing you to test assumptions made by the program and trigger an assertion failure if a specified condition evaluates to false.
Here’s a code snippet demonstrating the use of `assert` from `<cassert>`:
#include <cassert>
int main() {
int x = 5;
assert(x > 0); // This assertion will pass
assert(x < 0); // This assertion will fail and terminate the program
return 0;
}
Understanding the cassert Library
What is cassert?
The `cassert` header file is part of the C++ Standard Library, playing a crucial role in managing assertions. It provides a straightforward way to enforce conditions that must hold true during program execution. Assertions are vital for debugging as they help detect and diagnose problems early in the development process, thereby contributing to robust code.
How to Include cassert
To use the functionality offered by `cassert`, you simply need to include it in your C++ files. This addition allows you to access the assertion macro that helps validate assumptions in your code.
Here’s how to include `cassert` in your program:
#include <cassert>
Once included, you can leverage the functionality of assertions throughout your code.
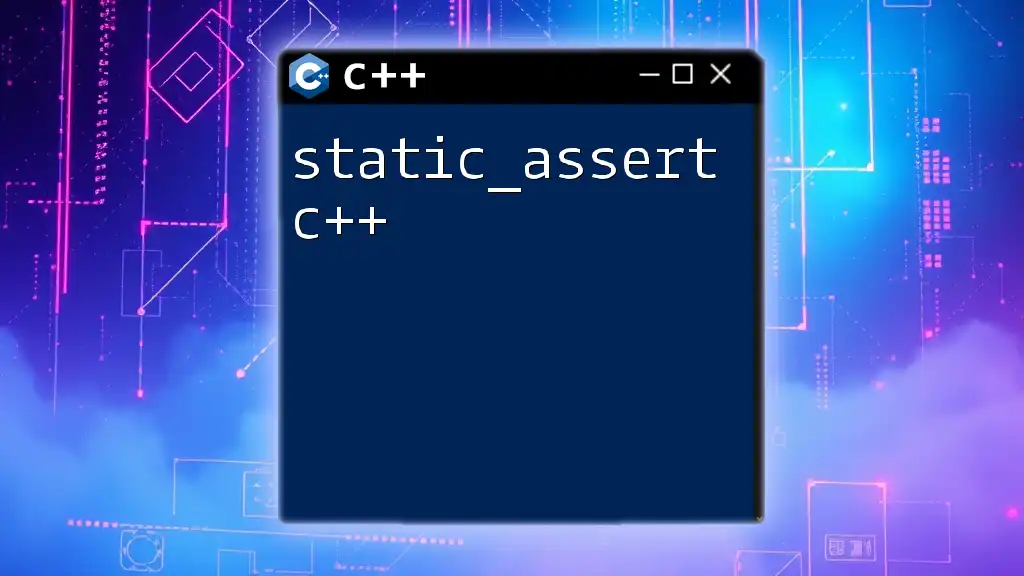
The assert Macro
Syntax and Usage
The core feature provided by `cassert` is the `assert` macro. Its general syntax is as follows:
assert(expression);
Here, the `expression` is a condition you want to test. If the condition evaluates to false, the program will terminate, and an error message will be displayed. This behavior is particularly useful for identifying logical errors in your code.
Behavior of assert
When you run a program built in debug mode, the `assert` macro will activate and check the validity of the specified expression. If the expression evaluates to true, nothing happens. However, if it evaluates to false, the program outputs error information regarding the assertion failure and stops execution.
It’s important to note that in release builds (typically the versions of a program sent to users), assertions are usually disabled. This means `assert` statements are not evaluated, thus protecting against performance overhead in a fully-optimized release.
Example of the assert Macro
Consider this simple program that demonstrates how to use the assert macro:
#include <cassert>
#include <iostream>
int main() {
int x = 10;
assert(x == 10); // This assertion passes, program continues
assert(x == 5); // This assertion fails
std::cout << "This line will not execute if the assert fails." << std::endl;
return 0;
}
In this example, when the code reaches the assertion checking whether `x == 5`, the program will stop execution and display an assertion failure message. Understanding this behavior is crucial for using assertions effectively for debugging.
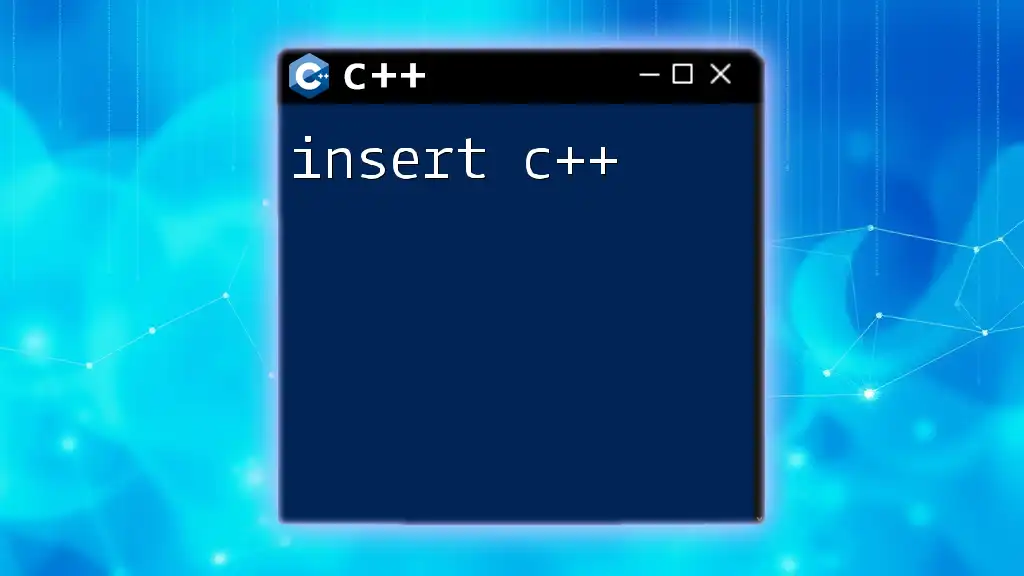
Important Concepts
Assertions vs. Exceptions
While both assertions and exceptions serve as safeguards in your code, they are used in different contexts. Assertions are intended to catch programming errors during development or debugging. They verify that certain conditions remain valid throughout the execution of the code. Conversely, exceptions are a mechanism for handling runtime errors gracefully, allowing a program to continue running or provide user feedback instead of terminating immediately.
Typically, you would use assertions to validate conditions that "should never happen," like checking the validity of assumptions about inputs or states within your code.
Debugging with Assertions
Assertions empower developers to check the consistency of their assumptions during runtime. For instance, if you assume a value will never be negative, you can validate that assumption with an assertion. If the assumption proves false, you can address the issue immediately, preventing harder-to-diagnose issues later on.
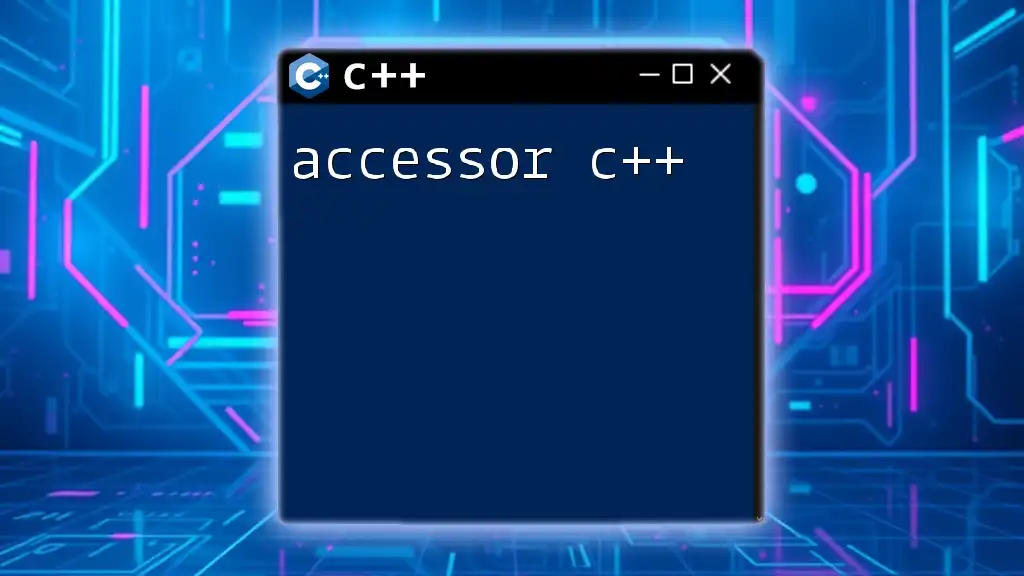
Best Practices
When to Use Assertions
Assertions are particularly effective in a few specific situations:
- Invariants: To verify conditions that must always hold true at a certain point in your program.
- Preconditions: To validate the requirements that must be satisfied before executing a function.
- Postconditions: To check the results returned from functions.
For example, you might assert that a function parameter’s value is within an expected range before proceeding with computations.
Avoiding the Use of Assertions in Production
One essential rule of thumb is to refrain from using assertions in a production environment. The logic behind this is that assertions are primarily diagnostic tools and should serve strictly during the debugging process. When a user runs a release version of your application, it should handle cases like invalid input gracefully through exceptions or error codes instead of simply terminating with an assertion failure message.
Understanding the Impact of Assertions on Performance
While assertions can help catch bugs, they can introduce runtime overhead, especially when many assertions are evaluated. However, this impact is generally minimal when using assertions during the debugging phase. Still, it’s essential to keep in mind that removing assertions in release builds can lead to improved performance.
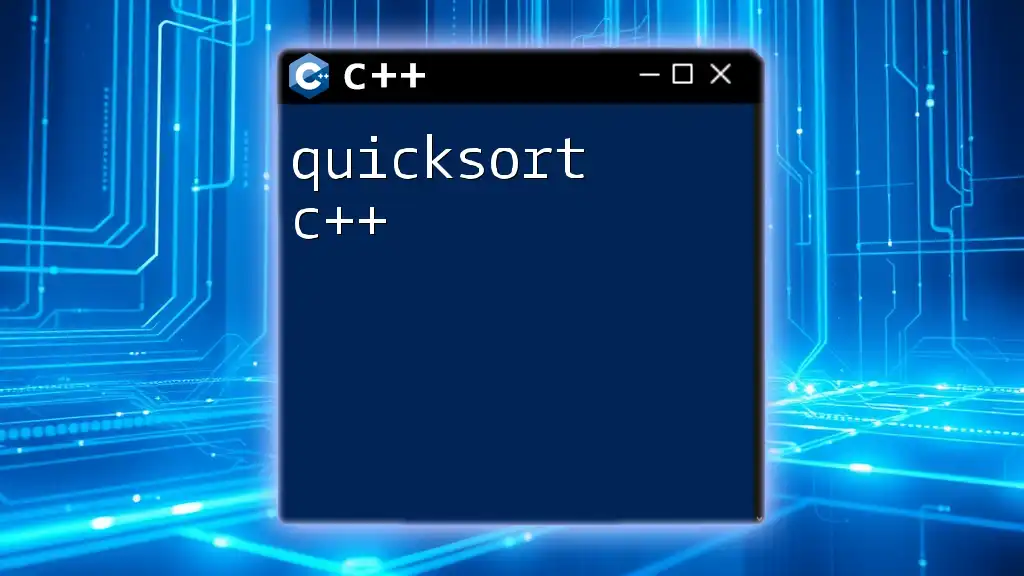
Advanced Usage
Custom Assertions
As C++ developers, you may find the need to define custom assertions tailored to your specific use case. This enables more contextual error messages and behavior. For illustration, see how to define a custom assertion macro:
#define MY_ASSERT(expr) assert((expr) && #expr)
Now, each time you use `MY_ASSERT`, it will display the expression that failed along with the assertion message, making it easier to debug specific cases.
Assertions in Multi-threaded Environments
Using assertions in multi-threaded environments can present unique challenges. For instance, if multiple threads write to shared resources simultaneously without proper synchronization, you might end up with race conditions that could violate the conditions you're checking with assertions. It's crucial to ensure that assertions do not lead to undefined behavior in such scenarios.
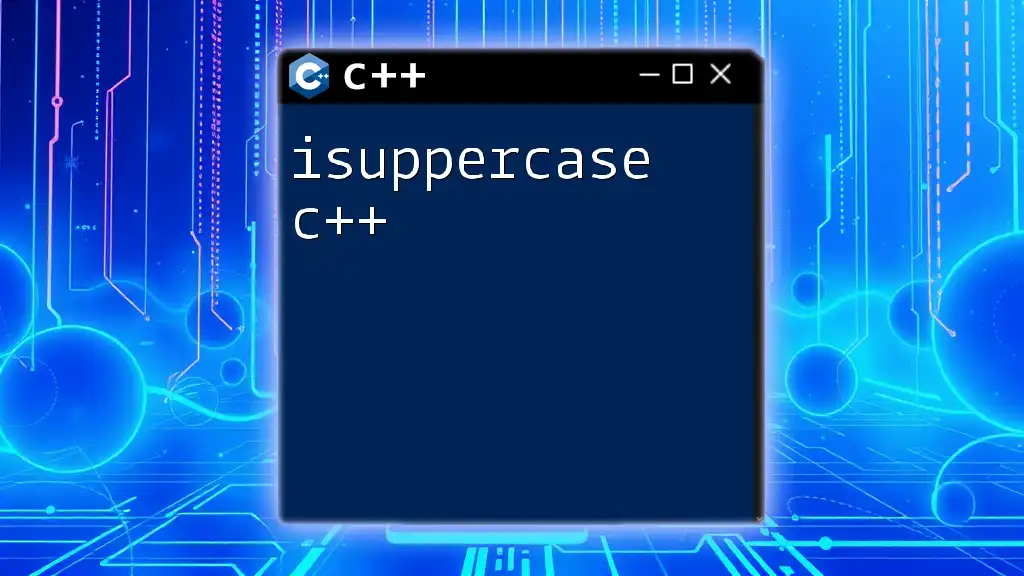
Common Mistakes
Misunderstanding Assertion Failure
When an assertion fails, it can lead to program termination. However, interpreting the failure message correctly is crucial. It typically contains the failed expression and the file/line number where the failure occurred, which are invaluable for debugging.
Overusing Assertions
Lastly, one common mistake is overusing assertions. Assertions are not intended to be a replacement for proper error handling. They should highlight logical errors rather than be used for checking user input or runtime data. Over-reliance on assertions can make code less readable and maintainable, leading developers to miss critical error handling pathways.
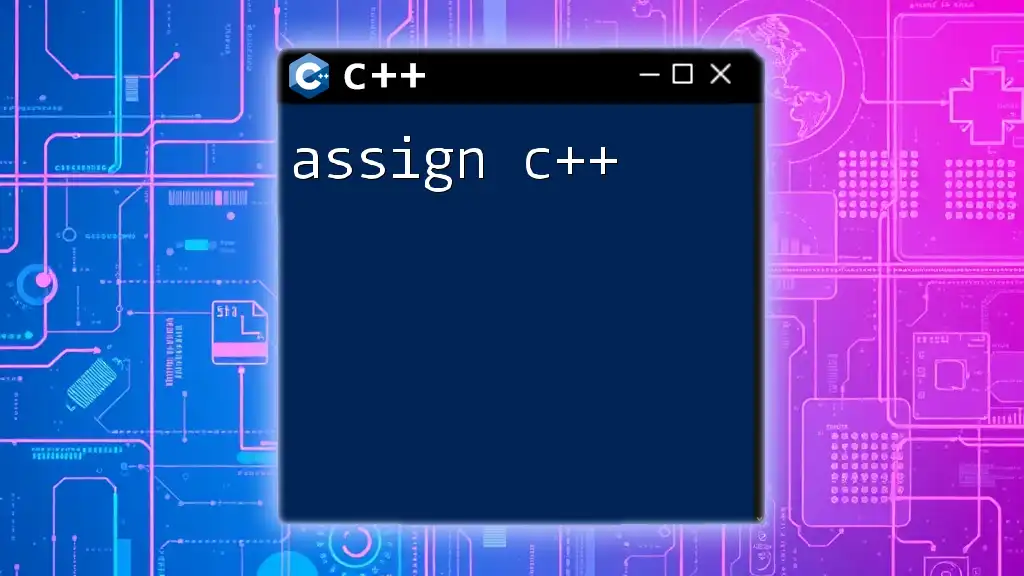
Conclusion
Assertions, implemented through `cassert` in C++, provide a powerful mechanism for validating assumptions and enhancing code safety during development. Understanding how and when to use assertions can significantly aid in debugging and lead to cleaner, more robust code.
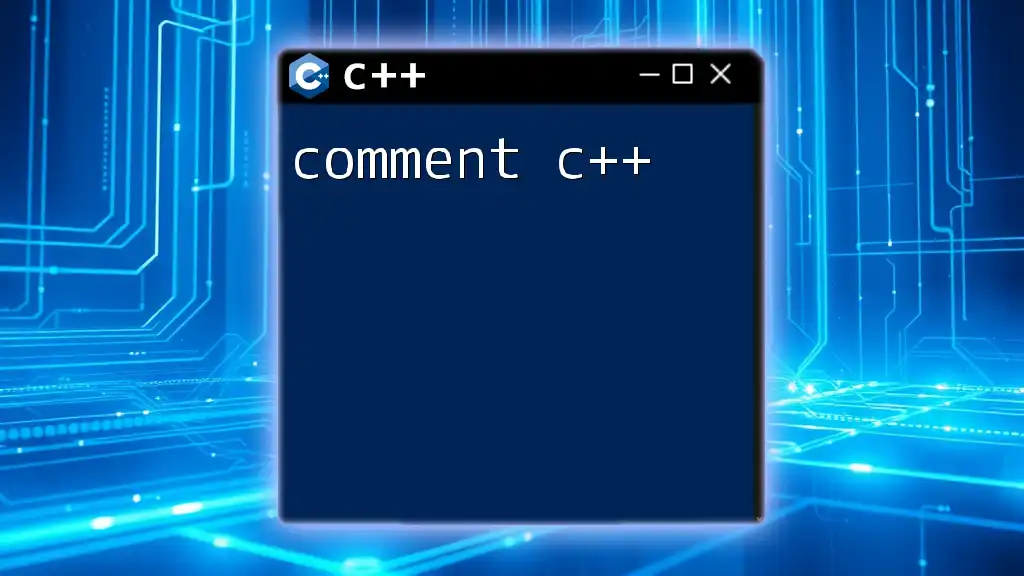
Additional Resources
To deepen your knowledge of assertions and debugging in C++, several resources are available. Consider reading C++ reference materials, bonus books on debugging techniques, and participating in forums or online classes focused on advanced C++ practices.
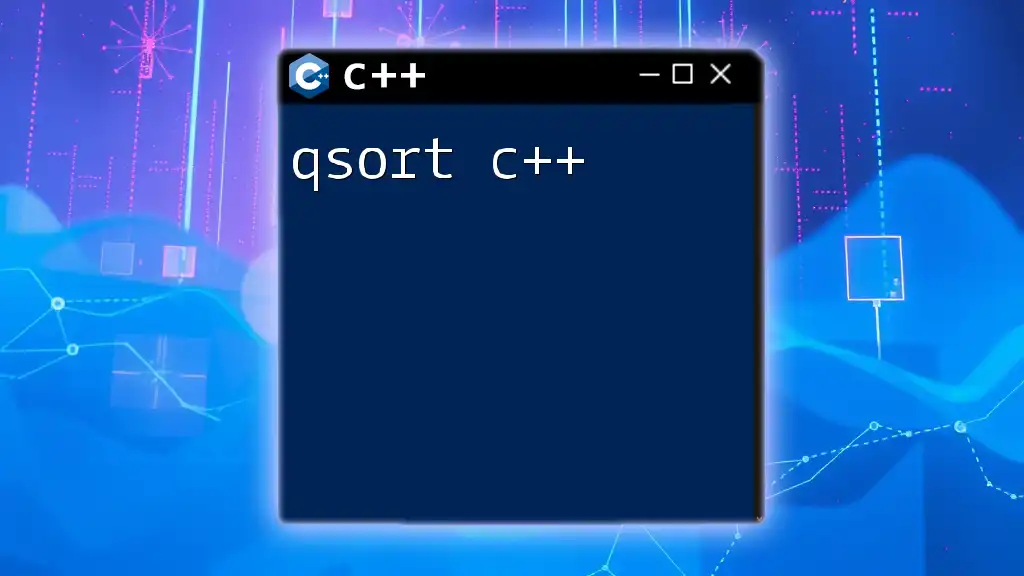
Call to Action
Try incorporating assertions in your own projects and reflect on their impact. Share your experiences, insights, or questions in the comments section below!