Ncurses is a programming library that provides an API for building text-based user interfaces in a terminal with C++, allowing developers to create visually appealing and interactive console applications.
Here's a simple example of using ncurses in C++ to display "Hello, World!" on the terminal:
#include <ncurses.h>
int main() {
initscr(); // Start ncurses mode
printw("Hello, World!"); // Print "Hello, World!"
refresh(); // Refresh to show the output
getch(); // Wait for user input
endwin(); // End ncurses mode
return 0;
}
What is ncurses?
The ncurses library is a powerful tool for building text-based user interfaces (TUIs) in C and C++. It allows developers to create complex, interactive programs that can display text in a structured manner, handle keyboard input, and manage multiple windows within the terminal. Originally developed in the late 1980s, ncurses has become a fundamental library for anyone looking to build terminal applications.
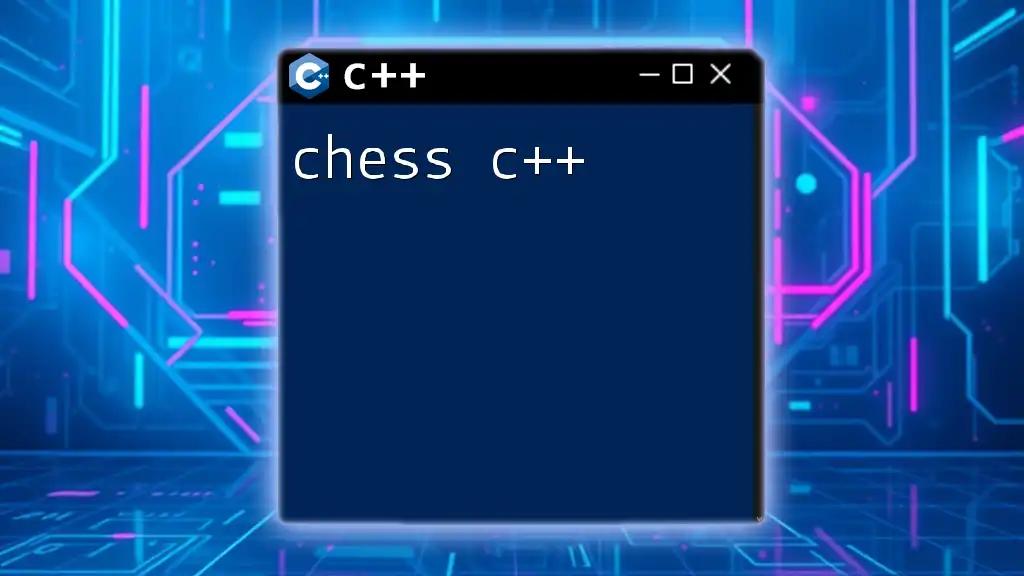
Why Use ncurses in C++?
When developing applications that require user interaction in a command-line environment, ncurses shines due to its ability to manage screen updates efficiently and provide a variety of user interface components. It is particularly beneficial when developing console applications that demand a rich visual representation while maintaining a lightweight footprint. Common use cases include:
- Text editors
- Games
- System monitoring tools
- Configuration interfaces
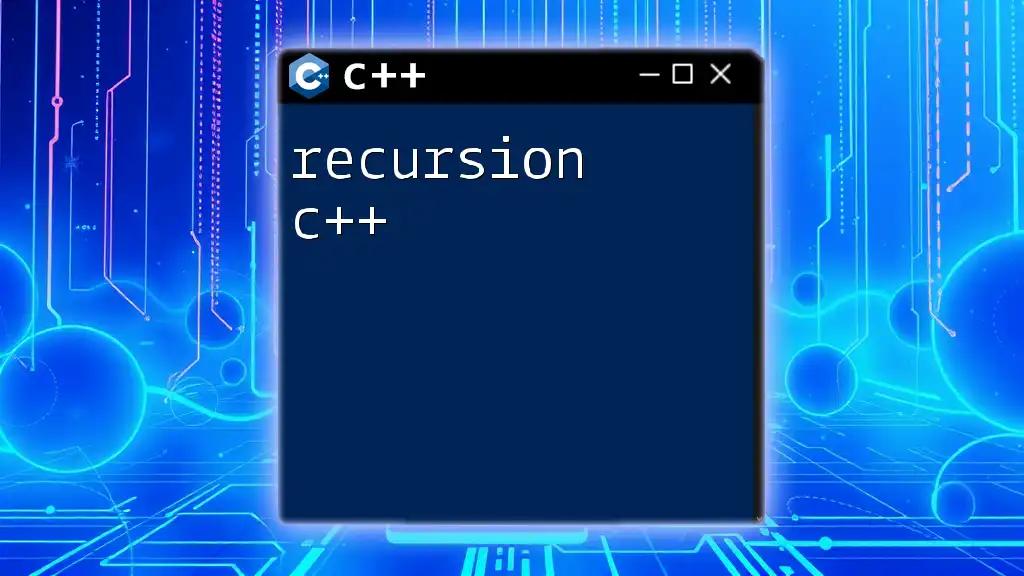
Installing ncurses
To leverage ncurses in your C++ projects, you'll first need to install the library. Below are instructions for various operating systems:
For Linux
Most Linux distributions come with ncurses pre-installed. If it's not available, you can install it using a package manager:
sudo apt-get install libncurses5-dev libncursesw5-dev
For macOS
On macOS, ncurses can be installed via Homebrew:
brew install ncurses
For Windows
On Windows, you can use Cygwin or MinGW to access ncurses. To install via Cygwin, select the ncurses package during installation.
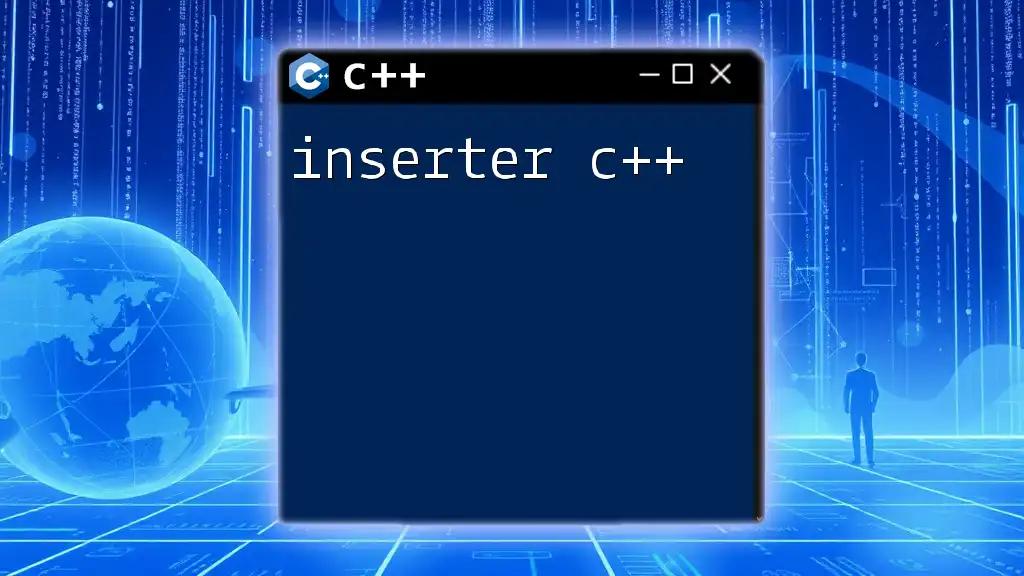
Configuring Your C++ Project
Once ncurses is installed, integrating it into your C++ project is straightforward. You need to include the header file in your source code:
#include <ncurses.h>
To compile your program, ensure to link against the ncurses library. Here’s a sample Makefile snippet:
CXX = g++
CXXFLAGS = -Wall
LIBS = -lncurses
app: app.o
$(CXX) -o app app.o $(LIBS)
app.o: app.cpp
$(CXX) $(CXXFLAGS) -c app.cpp
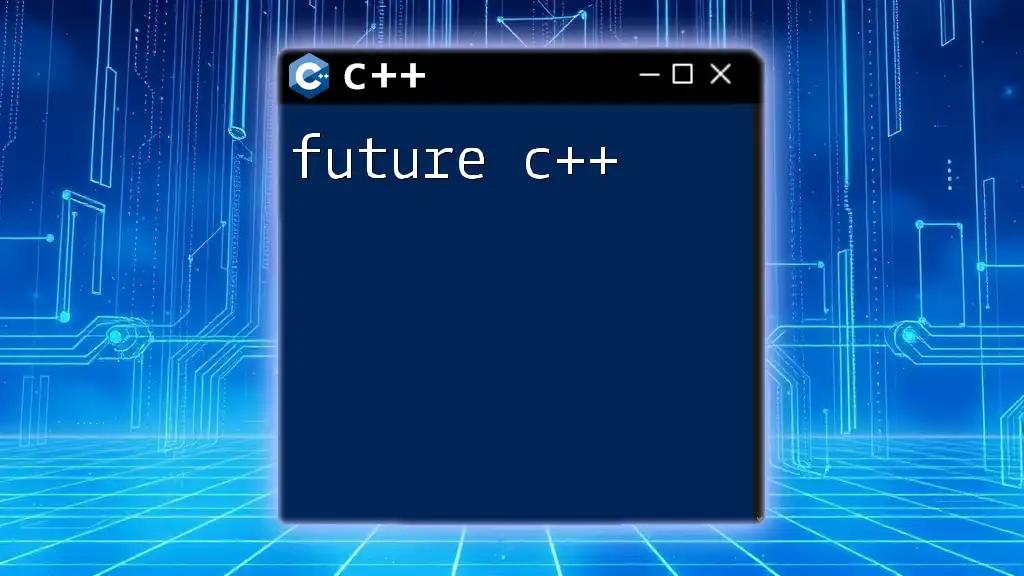
Initializing ncurses
Before using any ncurses functions, you must initialize the library. This is done through the `initscr()` function. Here’s an example:
initscr(); // Initialize the ncurses mode
This function sets up the necessary data structures and clears the screen, preparing your application for interaction.

Creating and Using Windows
ncurses allows you to create multiple windows for displaying information. You can use the `newwin()` function to create a new window, specifying its dimensions and position on the screen.
Here’s an example:
WINDOW *win;
win = newwin(10, 40, 5, 5); // height, width, start_y, start_x
box(win, 0, 0); // Draw a box around the window
wrefresh(win); // Refresh to show the box
In this snippet, we create a window with a height of 10 and a width of 40, positioned at (5,5) on the terminal. The `box()` function creates a border around the newly created window.

Key Input Handling
One of the essential features of ncurses is its ability to handle keyboard input. The `getch()` function waits for user input and returns the corresponding character. Here's a basic example:
int ch;
while((ch = getch()) != 'q') { // Exit loop if 'q' is pressed
printw("You pressed: %c\n", ch);
refresh(); // Update the screen
}
In this code snippet, the program displays the character pressed by the user until they press 'q', at which point it exits.

Color Management
ncurses supports color outputs which enhance the appearance of your application. To use colors, you must first initialize color support with `start_color()` and define color pairs using `init_pair()`. Here's a quick example:
start_color();
init_pair(1, COLOR_RED, COLOR_BLACK);
attron(COLOR_PAIR(1)); // Activate the color pair
printw("This text is red on black background!");
attroff(COLOR_PAIR(1)); // Deactivate the color pair
In this snippet, we initialize the color system and set a color pair (red text on a black background). We then activate this color for the text printed to the screen.

Text Attributes
ncurses allows you to manipulate text attributes, such as making text bold or underlined. This is done with functions like `attron()`, `attroff()`, and `A_BOLD`. Here's an example:
attron(A_BOLD);
printw("This text is bold!\n");
attroff(A_BOLD);
printw("This text is normal.\n");
This will print the first line in bold and the second line in a regular style.

Building a Main Menu
Creating a text-based menu with ncurses is simple. The following outlines how to build an interactive menu:
const char* choices[] = {"Option 1", "Option 2", "Option 3", "Quit"};
int highlight = 0;
int choice = 0;
int c;
while(1) {
clear();
for(int i = 0; i < 4; ++i) {
if(i == highlight) {
attron(A_REVERSE); // Highlight current choice
printw("%s\n", choices[i]);
attroff(A_REVERSE);
} else {
printw("%s\n", choices[i]);
}
}
c = getch();
switch(c) {
case KEY_UP:
highlight = (highlight == 0) ? 3 : (highlight - 1);
break;
case KEY_DOWN:
highlight = (highlight + 1) % 4;
break;
case 10: // Enter key
choice = highlight;
break;
}
if(choice == 3) break; // Exit if "Quit" is chosen
}
This code initializes a simple menu that allows users to navigate through options. Highlighting options enhances user experience, and the menu exits when “Quit” is selected.

Scrolling and Padding
When working with large datasets or text, the ability to scroll through content is crucial. ncurses provides built-in support for scrolling windows. Here's an example of how you can implement scrolling:
WINDOW *scroll_win = newwin(10, 30, 0, 0);
scrollok(scroll_win, TRUE); // Enable scrolling for this window
for(int i = 0; i < 20; ++i) {
wprintw(scroll_win, "Line %d\n", i);
}
wrefresh(scroll_win);
In this snippet, we create a scrollable window and print more lines than its height. The window will scroll up as new content is added.

Forms and Dialog Boxes
To create forms with ncurses, the `form` library can be used. Below is a simple form example:
FIELD *fields[3];
forms_init(); // Initialize form system
fields[0] = new_field(1, 20, 2, 10, 0, 0);
fields[1] = new_field(1, 20, 4, 10, 0, 0);
fields[2] = NULL; // End field array
form_post(my_form);
This code initializes fields for user input with specific sizes and positions, allowing users to enter data interactively.
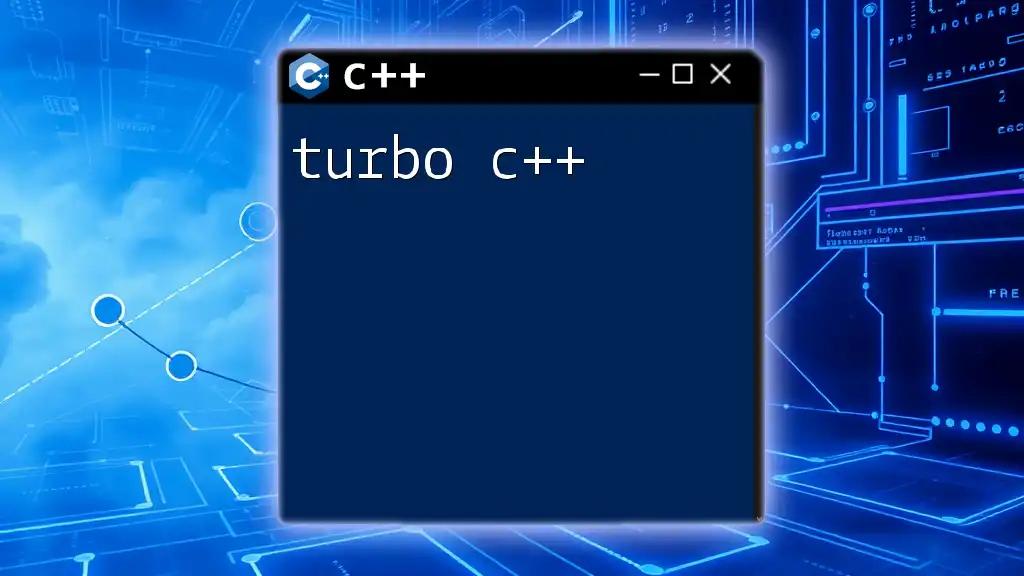
Adding Mouse Support
ncurses can also handle mouse events, allowing for richer user interactions. To enable mouse support, call `mousemask()`:
mousemask(ALL_MOUSE_EVENTS, NULL);
MEVENT event; // Event structure to hold mouse events
while((c = getch()) != 'q') {
if(c == KEY_MOUSE) {
if(getmouse(&event) == OK) {
printw("Mouse clicked at: (%d, %d)", event.x, event.y);
}
}
refresh();
}
In this example, the program detects mouse clicks and prints the coordinates of the click within the terminal.

Debugging ncurses Applications
Debugging ncurses applications is essential to ensure they work correctly in the terminal environment. Common issues include improper window initialization and screen refresh problems.
Common Issues and Solutions
- Screen not refreshing: Ensure you call `refresh()` after any changes to the window or screen.
- Input not capturing: Verify that you are waiting for input with `getch()` and using the correct terminal mode.
Using Logs for Debugging
When debugging complex ncurses applications, logging can provide insights into your program's state. For example, you may want to log inputs or window states to a file:
#include <fstream>
std::ofstream log("debug.log");
log << "User pressed: " << ch << std::endl;
This logs the pressed keys to a file for later review.
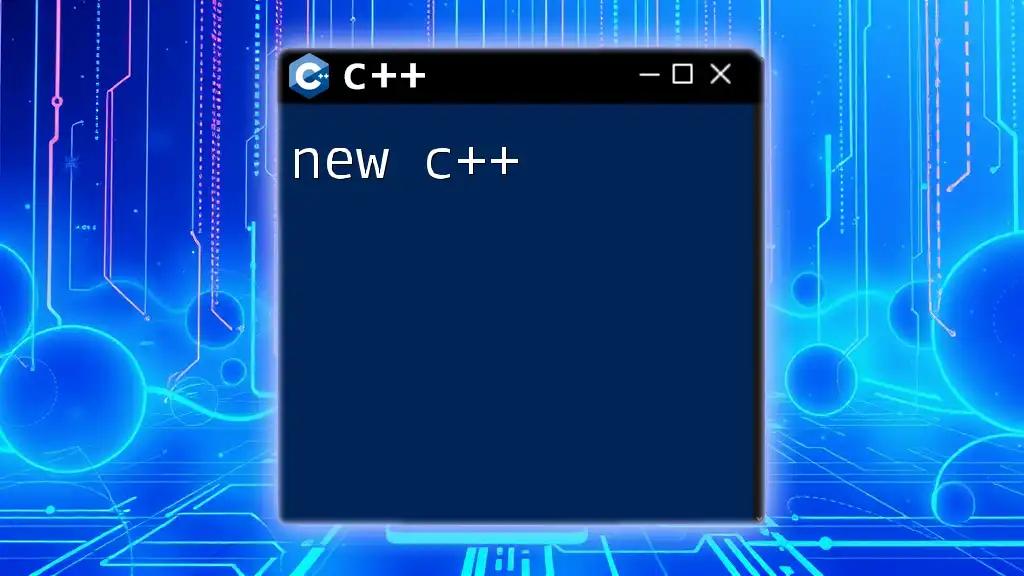
Best Practices
Organizing Your Code
Maintain a clean separation between your logic and the user interface. Organizing your code into functions responsible for various tasks (like displaying a menu or processing input) enhances readability and maintainability.
Performance Considerations
When using ncurses, avoid excessive screen refreshes, as they can slow down your application. Instead, batch updates together whenever possible. For example, combine print statements where feasible before calling `refresh()`.
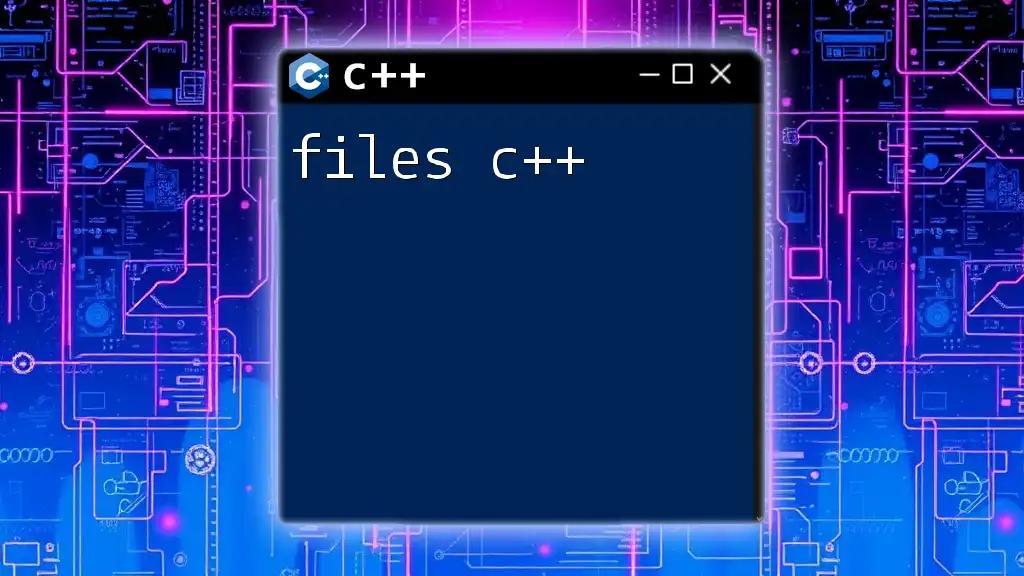
Conclusion
In summary, ncurses offers robust capabilities for developing text-based user interfaces in C++. From its ability to handle multiple windows to support keyboard inputs and colors, it provides everything needed for attractive and interactive terminal applications. The features discussed in this guide empower you to construct powerful command-line tools and enhance user interaction within your programs.

Additional Resources
For further learning, consider exploring resources like:
- The ncurses official documentation
- Books on C++ programming that cover terminal applications
- Online forums and communities dedicated to C and C++ development, where you can seek help and share insights on your ncurses projects.
With this knowledge, you're well on your way to mastering ncurses in your C++ applications!