Turbo C++ is an integrated development environment and compiler for the C++ programming language, widely known for its fast compilation speed and ease of use in learning to code.
Here's a simple code snippet to demonstrate a basic "Hello, World!" program in Turbo C++:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
What is Turbo C++?
Turbo C++ is an integrated development environment (IDE) and compiler designed for C and C++ programming languages. Developed by Borland in the early 1990s, it played a pivotal role in making C++ programming accessible to beginners by offering a user-friendly graphical interface. Its significance lies in its legacy, as it popularized the rapid application development approach, allowing programmers to write, compile, and run their code swiftly.
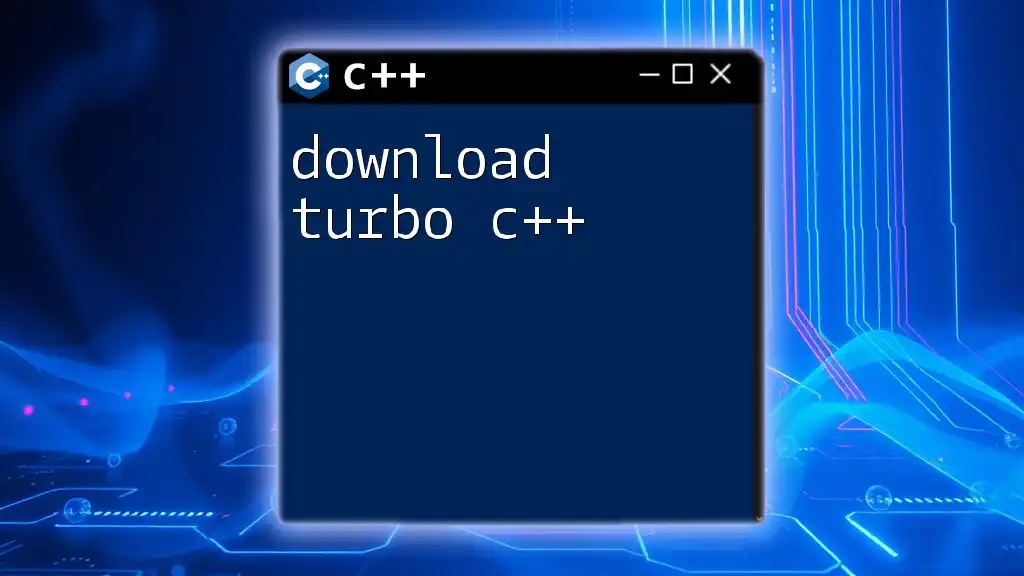
Why Learn Turbo C++?
Learning Turbo C++ provides several benefits for both beginners and advanced users:
- Foundation for Programming Concepts: It's an excellent starting point for grasping C++ fundamentals without the complexities of modern environments.
- Simplicity: Its simple interface provides a focused learning experience without distractions.
- Historical Relevance: Understanding Turbo C++ offers insights into the evolution of C++ programming and its core concepts.
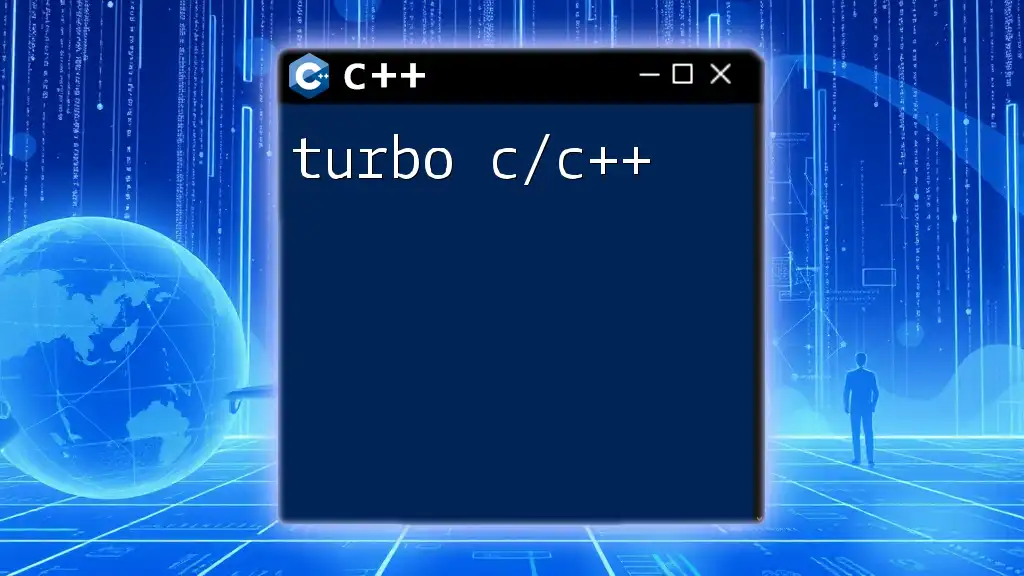
Getting Started with Turbo C++
Installation of Turbo C++
To begin using Turbo C++, follow these steps for installation:
System Requirements
Before installation, ensure your system meets the minimum requirements:
- Operating System: Windows 95/98/XP (with compatibility mode for newer systems)
- RAM: Minimum 256 MB
- Storage: At least 100 MB of free space
Step-by-Step Installation Guide
- Downloading Turbo C++: Start by downloading Turbo C++ from a reliable source. Ensure you obtain an official or trusted version.
- Installation Process on Windows:
- Run the downloaded setup file.
- Follow the prompts to complete the installation, selecting your preferred directory.
Setting Up Turbo C++ for First Use
Launch Turbo C++ after installation. To ensure your environment is ready:
- Configure the Initial Settings: Set up paths for required libraries and configure your environment as needed.
- Creating Your First Project: Start by selecting “File” > “New” to create a new project file.
Overview of Turbo C++ Interface
Understanding the Turbo C++ interface is vital for navigating its features efficiently:
- Menu Bar: Access various options such as File, Edit, Compile, Debug, and Execute from the menu.
- Toolbars: Utilize toolbars for quick access to commonly used commands.
- Programming Window: This is where you write your code. Familiarize yourself with syntax highlighting and code formatting options.
Customizing the Interface
Turbo C++ allows you to tailor the interface to enhance your coding experience:
- Changing Compiler Settings: Navigate to Options > Compiler to modify compiler options according to your preferences.
- Setting Up Custom Shortkeys: Improve efficiency by defining shortcut keys for frequently used commands. Access this feature under Options > Editor.
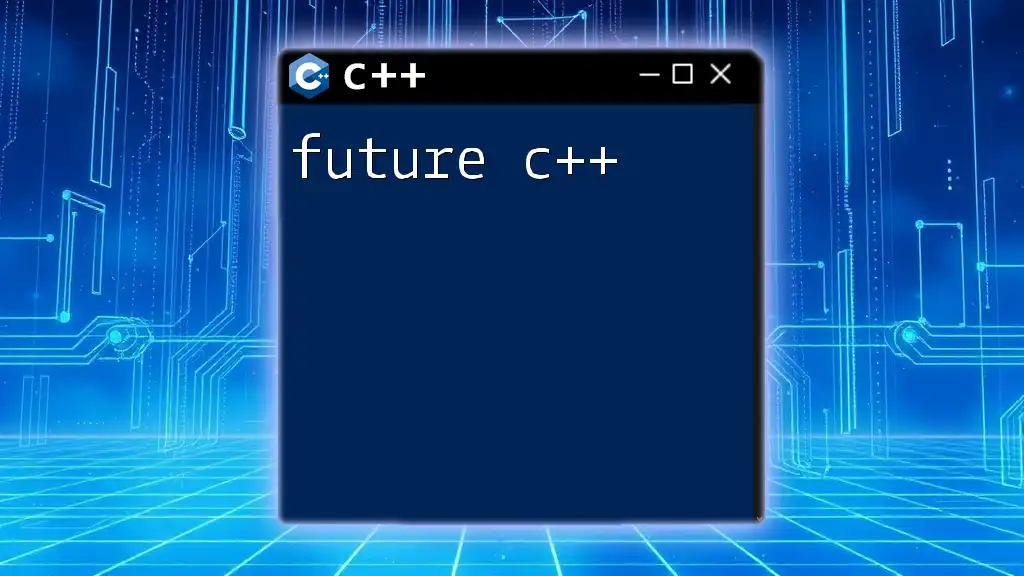
Basic Features of Turbo C++
Writing a Simple C++ Program
Creating your first program is crucial for understanding the basics of Turbo C++. Let’s write a simple "Hello World" program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this snippet:
- `#include <iostream>`: Includes the Input/Output stream library necessary for `cout`.
- `using namespace std;`: Allows the use of standard C++ functions without prefixing with `std::`.
- `int main()`: The main function where the execution begins.
- `cout << "Hello, World!" << endl;`: Outputs "Hello, World!" to the console.
Compiling and Running Programs
Compiling and running your program is led by straightforward processes:
- How to Compile Code in Turbo C++: This is done by navigating to Compile > Compile (or simply pressing `Alt + F9`). The compiler will check your code for errors.
- Running Your Program: After compilation, execute your code by selecting Run > Run (or pressing `Ctrl + F5`). You’ll see the output in the console window.
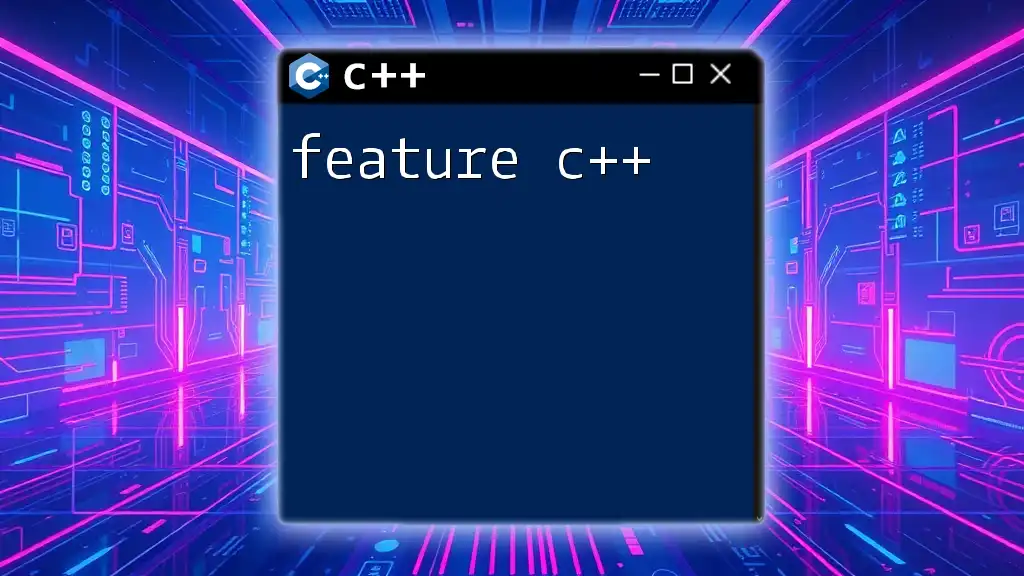
Advanced Features of Turbo C++
Debugging in Turbo C++
Debugging is an essential skill for any programmer. Turbo C++ offers several tools for effective debugging:
- Introduction to Debugging: Debugging allows you to identify and fix errors in your code, enhancing its reliability.
- Tools Available for Debugging: Use breakpoints to pause execution at specific points, inspect variables while stepping through code, and analyze the flow of your program using the debugging options under the Debug menu.
Using Turbo C++ Libraries
Libraries streamline coding by providing pre-written functions. Here’s a closer look:
- Understanding Libraries: Libraries in C++ hold collections of functions and classes that simplify programming tasks.
- Commonly Used Libraries:
- `<iostream>`: For input and output operations.
- `<conio.h>`: Contains functions for console input/output operations.
- `<math.h>`: Provides mathematical functions like sin, cos, sqrt, etc.
Graphics Programming
Turbo C++ allows you to create simple graphics applications. Here's an introduction:
- Introduction to Graphics in Turbo C++: Graphics programming enables the representation of data visually.
- Basic Graphics Example:
#include <graphics.h>
#include <conio.h>
int main() {
int gd = DETECT, gm;
initgraph(&gd, &gm, "");
circle(200, 200, 100);
getch();
closegraph();
return 0;
}
In this snippet:
- `initgraph(&gd, &gm, "");`: Initializes the graphics mode.
- `circle(200, 200, 100);`: Draws a circle at the coordinates (200, 200) with a radius of 100.
- The program waits for a key press before closing the graphics window.
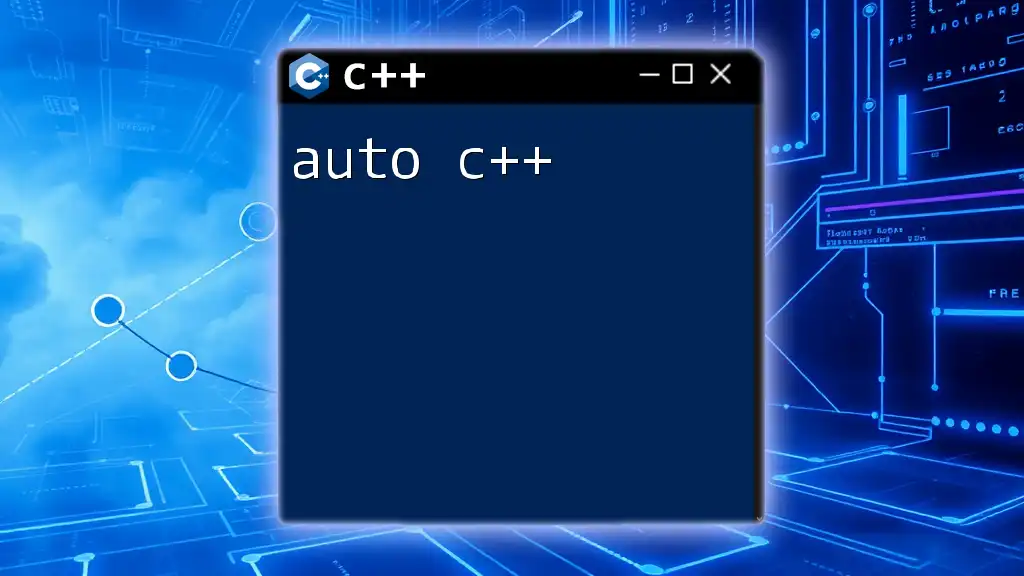
Turbo C++ vs. Modern C++
Comparing Turbo C++ with Modern C++ IDEs
Understanding the differences between Turbo C++ and modern IDEs can contextualize its relevance:
- Compatibility and Limitations: Turbo C++ does not support many of the features introduced in newer C++ standards (C++11, C++14, etc.).
- Community and Support: While Turbo C++ has a dedicated user base, modern C++ IDEs like Visual Studio and Code::Blocks have larger support communities offering updated resources.
Transitioning from Turbo C++ to Modern C++
Transitioning requires adapting to newer methodologies:
- What to Expect: Modern C++ emphasizes object-oriented programming, advanced libraries, and a more extensive standard library.
- Recommended Resources for Learning Modern C++: Books like "Effective Modern C++" by Scott Meyers and online courses from platforms like Coursera and Udacity are excellent for advancing your Java skills.
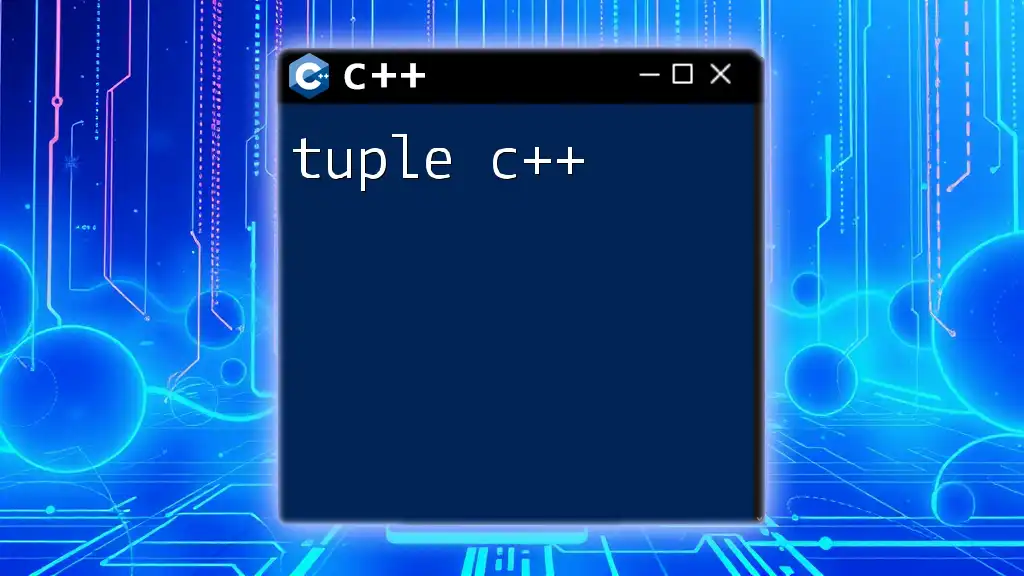
Conclusion
Turbo C++ remains a significant tool for learners aiming to grasp foundational programming concepts in C++. Its simplicity and ease of use make it an ideal starting point for newcomers. While more advanced environments are essential for exploring modern C++, understanding Turbo C++ can deepen your grasp of C++ fundamentals.
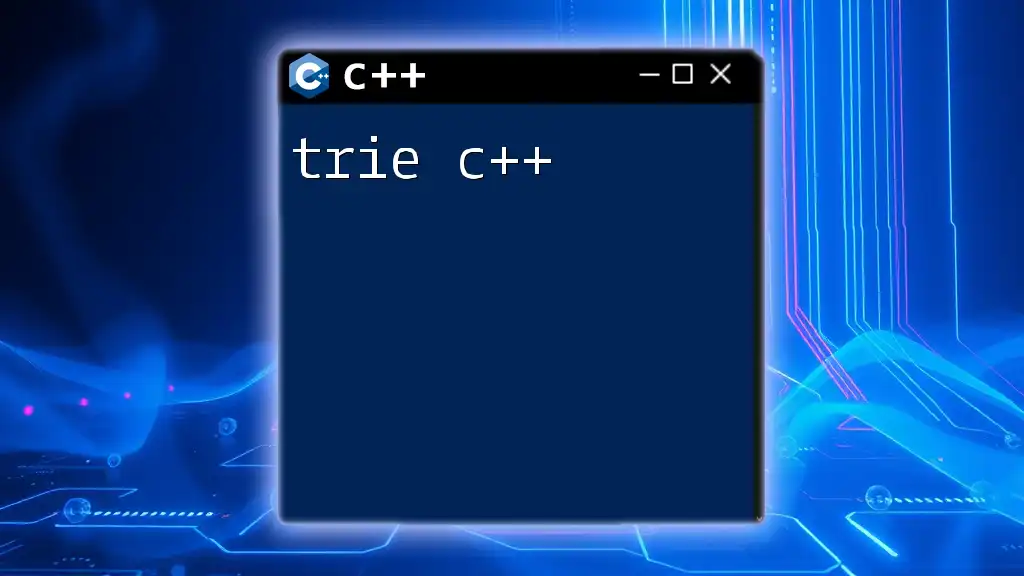
Additional Resources
To continue your learning journey, refer to:
- Online Tutorials: Websites dedicated to C++ tutorials offer hands-on exercises.
- Books and Literature on Turbo C++ and C++: Look for titles that focus on practical programming insights.
- Community Forums and Support Groups: Engage with fellow programmers to share knowledge and solve challenges together.
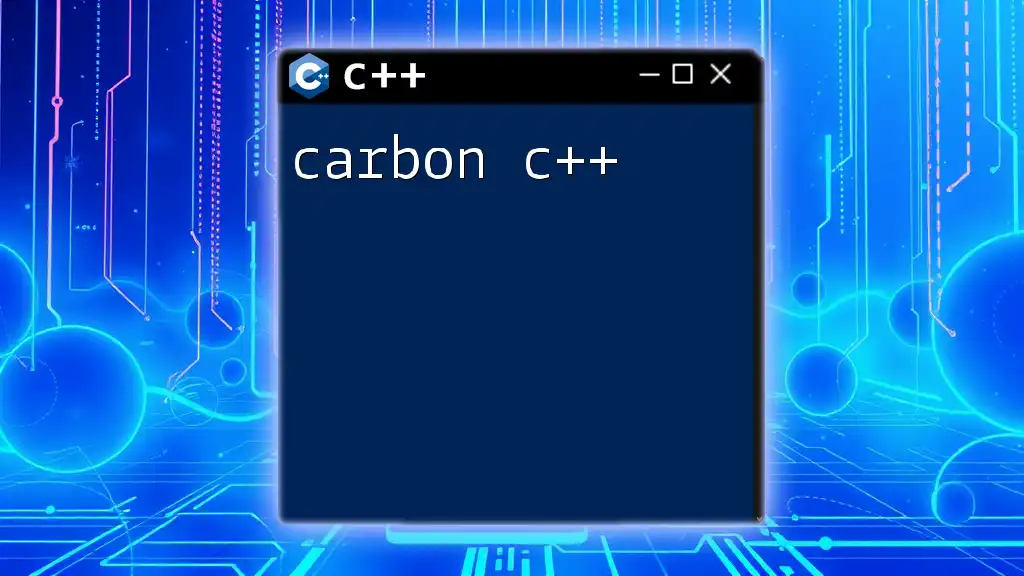
Frequently Asked Questions (FAQs)
What are the pros and cons of using Turbo C++?
- Pros include a simple learning curve, a straightforward interface, and historical significance. However, its compatibility with newer standards is a significant drawback.
Is Turbo C++ still relevant today?
- While it serves as a useful educational tool, modern standards and IDEs have largely overshadowed Turbo C++ in professional settings.
How do I troubleshoot common installation issues?
- Refer to community forums for solutions, and ensure your system meets the requirements and configurations are correctly set.
This comprehensive guide equips you with the essential knowledge needed to navigate Turbo C++, making your programming experience both effective and enjoyable.