Turbo C/C++ is an integrated development environment and compiler for the C and C++ programming languages that simplifies the process of writing and executing code with a user-friendly interface.
Here's a basic example of a C++ program in Turbo C++:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, Turbo C/C++!" << endl;
return 0;
}
Understanding Turbo C/C++
What is Turbo C/C++?
Turbo C/C++ is an Integrated Development Environment (IDE) and compiler designed for the C and C++ programming languages. Developed by Borland in the late 1980s, it quickly gained popularity among developers for its fast compilation speeds and user-friendly interface. For many, it served as an introduction to programming, making it a historical staple in the field of software development.
Features of Turbo C/C++
One of the key strengths of Turbo C/C++ lies in its rich set of features:
-
Speed and Efficiency: It is renowned for its extremely fast compilation and execution speeds, enabling developers to see the results of their code in real-time.
-
Integrated Debugging Tools: The IDE comes with built-in debugging features, making it easier to find and fix errors within your code compared to more manual processes used in other environments.
-
Structured Programming Support: Turbo C/C++ encourages best practices through its support for structured and object-oriented programming paradigms. This allows developers to write organized and maintainable code.
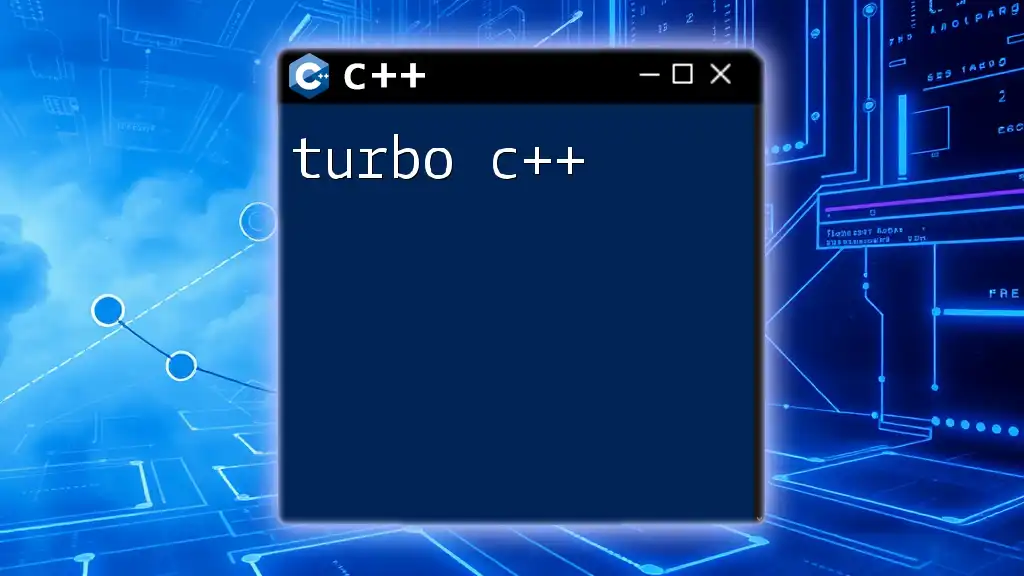
Setting Up Turbo C/C++
System Requirements
Before diving into coding, you need to ensure that your system meets the necessary requirements to run Turbo C/C++. These include:
-
Operating System: It is compatible with older versions of Windows (specifically Windows 95/98/XP) or DOS.
-
Memory: A minimum of 1 MB RAM is required, but having at least 4 MB is recommended for smoother operation.
-
Disk Space: You will need around 10 MB of free disk space to accommodate the installation.
Installation Steps
To properly install Turbo C/C++, follow these steps:
-
Download Turbo C/C++: Find a trusted website that offers the Turbo C++ executable file for download.
-
Install Turbo C/C++: Once downloaded, run the executable file and follow the installation prompts to install the software onto your computer.
-
Setting Up Environment Variables: After installation, you will need to set the PATH variable to include the directory where Turbo C/C++ is installed. This allows your system to recognize Turbo C/C++ commands from the command line.
Configuring Turbo C/C++
After successful installation, it is essential to configure Turbo C/C++ to fit your development needs. Open the IDE and navigate to the configuration settings. Important areas to adjust include:
-
Directories: Ensure that the directories for source files, include files, and libraries are correctly set to point to the appropriate folders.
-
Compiler Options: Depending on your project requirements, you may need to adjust compiler options for optimal performance.
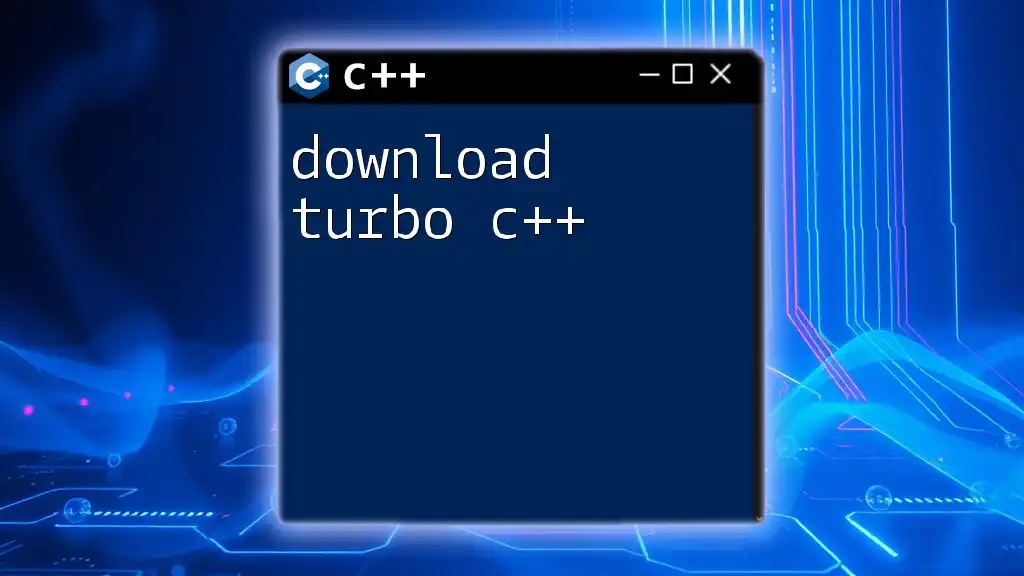
Basic Commands and Features in Turbo C/C++
Navigating the Turbo C/C++ IDE
The Turbo C/C++ IDE is organized around a menu system that simplifies coding tasks. Key components of the menu bar include:
-
File: For opening, saving, and managing source code files.
-
Edit: Provides functionalities like cutting, copying, and pasting code.
-
Search: Allows users to find specific lines or functions within their code.
-
Compile: A crucial feature for compiling your code to check for errors.
-
Execute: Let’s you run the compiled program.
-
Debug: Essential for troubleshooting and debugging your code.
-
Help: Provides access to documentation and guides for using Turbo C/C++.
Writing Your First Turbo C++ Program
To get started, let’s write a simple "Hello, World!" program that showcases the basic structure of a C++ program:
#include <iostream.h>
void main() {
cout << "Hello, World!" << endl;
}
Explanation:
-
`#include <iostream.h>` is necessary to include the input-output stream library, allowing you to use standard I/O functions.
-
`void main()` defines the main function, which is the entry point for the program's execution.
-
`cout << "Hello, World!" << endl;` uses `cout` to display output to the console.
Compiling and Running Programs
To compile and run your code in Turbo C/C++, follow these simple steps:
-
Compiling a Program: Press `Ctrl + F9` to compile your code. If there are no errors, it will generate an executable file.
-
Running a Program: After a successful compilation, you can run the program by pressing `Ctrl + F5`. This will execute the compiled code and display the output in a console window.
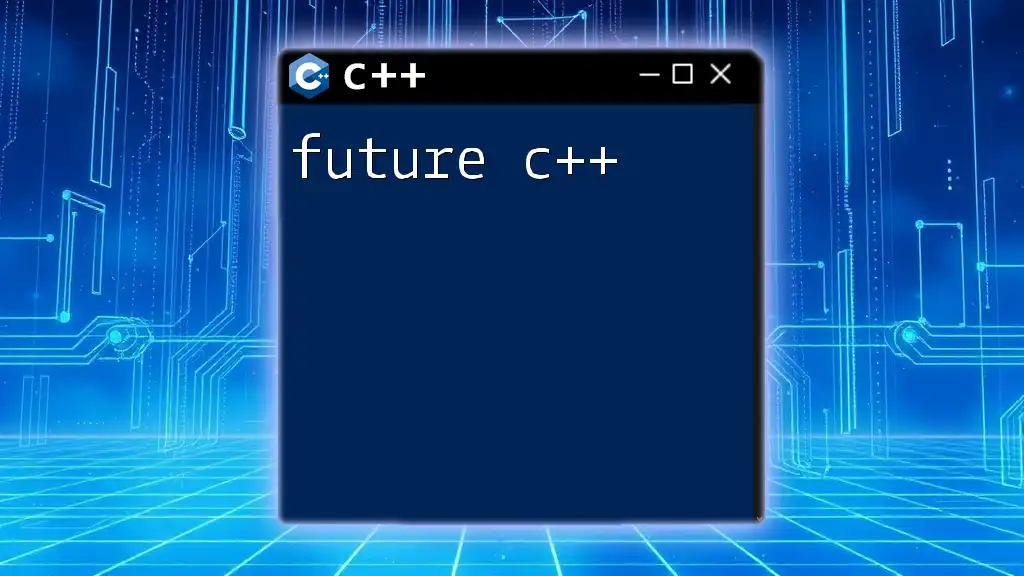
Developing with Turbo C/C++
Writing Functions and Structs
Functions and structs are fundamental building blocks in C/C++. Here’s how to define each:
Functions Example:
int add(int a, int b) {
return a + b;
}
In the example above, `add` is a function that takes two integer parameters and returns their sum.
Structures Example:
struct Student {
char name[50];
int roll_number;
};
The `Student` struct above encapsulates a student’s name and roll number, showcasing how to define a data type that groups related data.
Understanding Pointers
Pointers are a powerful feature in C/C++, providing direct access to memory. Here’s a simple example of using pointers:
int main() {
int var = 10;
int *ptr = &var; // Pointer to var
cout << *ptr; // Dereferencing pointer to get the value
}
In this snippet, `ptr` is a pointer that holds the address of `var`. By dereferencing `ptr` using the `*` operator, you can retrieve the value stored at that memory location.
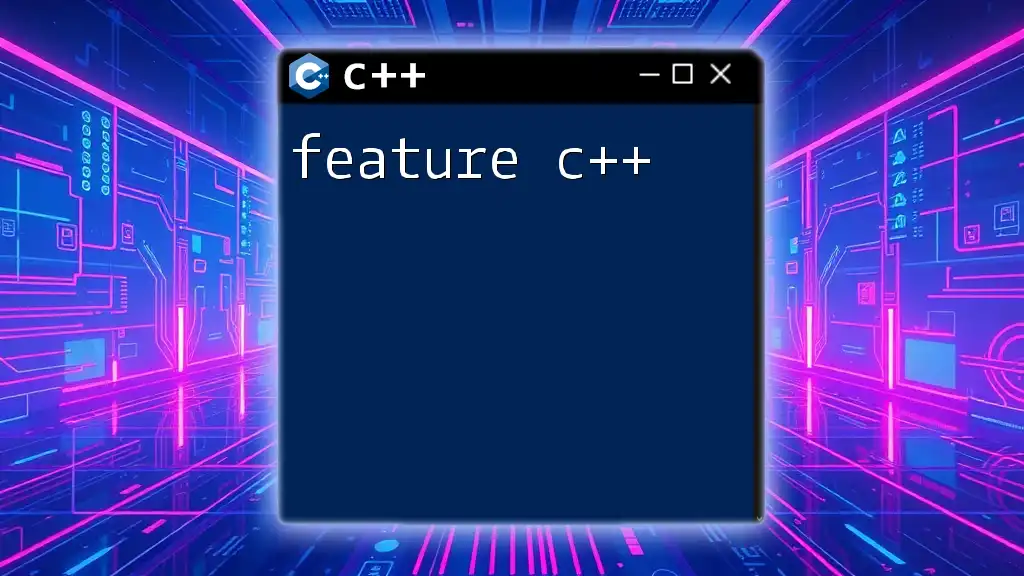
Turbo C/C++ vs. Modern Compilers
Limitations of Turbo C/C++
While Turbo C/C++ served as an excellent learning tool, it has several limitations in today’s programming landscape:
-
Outdated Language Standards: It largely supports C and C++ standards that predate significant updates such as C++11, which introduced numerous enhancements to the language.
-
Compatibility Issues: Turbo C/C++ struggles to run on modern operating systems without compatibility layers, making it less practical for current software development needs.
Recommendations for Modern Usage
For developers looking to expand their skills beyond Turbo C/C++, consider transitioning to updated compilers such as:
-
GCC (GNU Compiler Collection): Widely used in various environments and supports the most recent C/C++ standards.
-
Microsoft Visual Studio: Offers a robust IDE experience for Windows users, complete with extensive libraries and frameworks.
-
Clang: Known for its fast compilation and effective diagnostics, Clang is a modern alternative that is gaining popularity among developers.
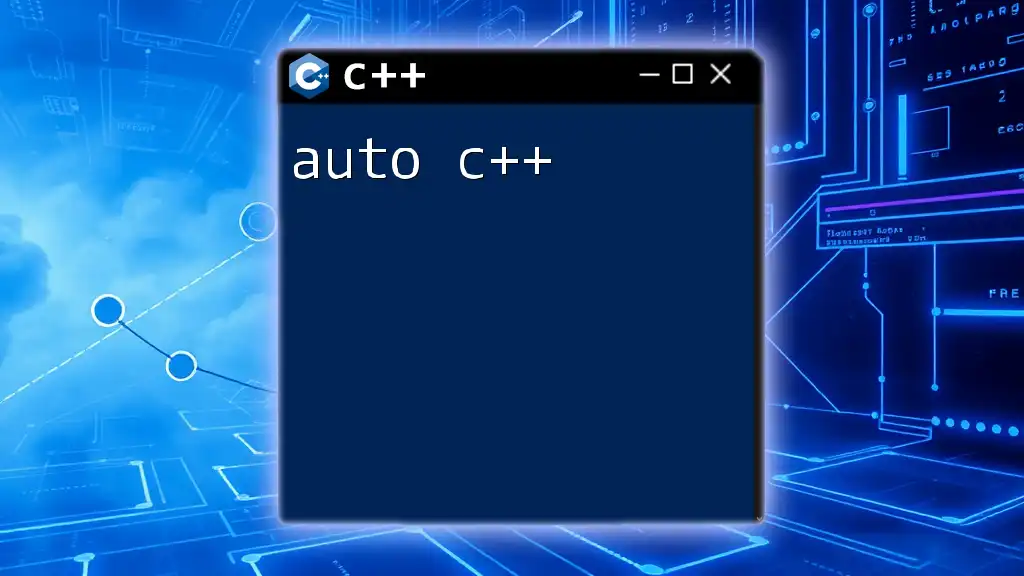
Conclusion
Why Learn Turbo C/C++?
Despite its limitations, Turbo C/C++ remains an excellent foundational tool for beginners to grasp programming fundamentals due to its concise command structure and simplicity.
Final Thoughts
Learning Turbo C/C++ provides a strong base in programming concepts. By embracing both Turbo C/C++ for foundational skills and modern compilers for real-world applications, you can become a well-rounded programmer equipped to tackle various software development challenges.