PyTorch C++ is a library that provides a seamless and efficient way to implement deep learning models using C++ syntax, similar to its Python counterpart.
Here's a simple example of using PyTorch C++ to create a tensor and perform basic operations:
#include <torch/torch.h>
#include <iostream>
int main() {
// Create a tensor
torch::Tensor tensor = torch::rand({2, 3});
std::cout << "Random Tensor:\n" << tensor << std::endl;
// Perform a simple operation
torch::Tensor result = tensor + 2;
std::cout << "Result after adding 2:\n" << result << std::endl;
return 0;
}
What is PyTorch?
PyTorch is an open-source machine learning library that primarily developed by Facebook's AI Research lab. It provides two high-level features: tensor computation (like NumPy) and automatic differentiation for building complex neural networks. PyTorch is appreciated for its flexibility and ease of use, allowing developers to construct dynamic computational graphs that enable real-time changes during model training. This dynamic nature makes it particularly appealing for research and experimentation.
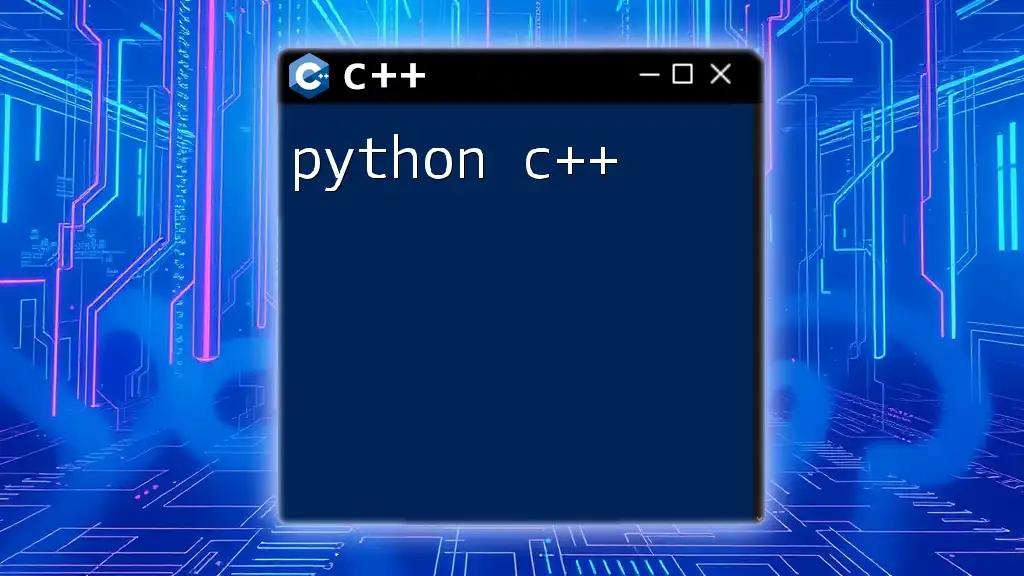
Why Use C++ with PyTorch?
C++ brings a pedigree of performance and control that is essential for high-performance machine learning applications. There are several reasons why one might choose to use PyTorch with C++:
-
Performance Benefits: C++ is known for its performance efficiency, particularly in resource-intensive computing tasks, which is crucial when training large models or processing massive datasets.
-
Integration with Existing Codebases: Many businesses already have established C++ systems and libraries. Using C++ with PyTorch allows easy integration of advanced machine learning models into these existing frameworks without the need to switch languages.
-
Production Suitability: When deploying machine learning models in production, C++ often outperforms Python in terms of speed and resource usage, making it the language of choice for scaling applications.
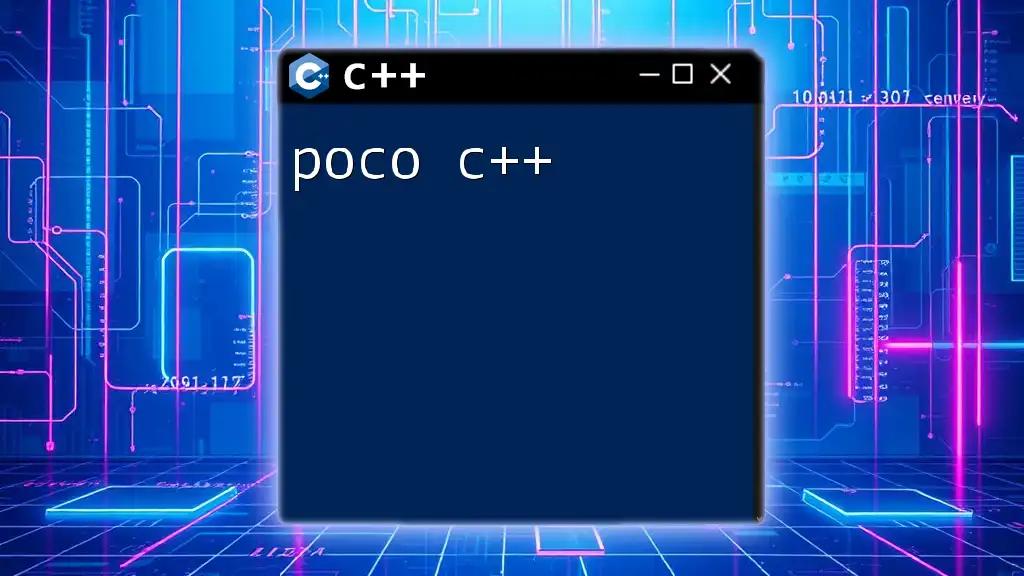
Setting Up Your Environment
Installing PyTorch C++ (LibTorch)
To start working with PyTorch in C++, you need to install LibTorch, the C++ distribution of PyTorch. Here’s how you can do it:
-
Step 1: Download LibTorch Visit the [official PyTorch website](https://pytorch.org/get-started/locally/#start-locally) and select the appropriate version of LibTorch based on your operating system (Linux, Windows, or macOS).
-
Step 2: Setting Up Environment After downloading, extract the files and set the environment variables, particularly the paths to the include and lib directories, so your compiler can find LibTorch.
-
Step 3: Create a CMake project CMake is essential for managing the build configuration. Create a `CMakeLists.txt` file and include the following lines to link against LibTorch:
cmake_minimum_required(VERSION 3.0 FATAL_ERROR) project(my_project) find_package(Torch REQUIRED) add_executable(my_project main.cpp) target_link_libraries(my_project "${TORCH_LIBRARIES}") set_property(TARGET my_project PROPERTY CXX_STANDARD 14)
-
Troubleshooting: If you encounter issues, ensure that your compiler supports C++14 and that all paths are correctly set.
Required Tools and Dependencies
To utilize PyTorch C++ efficiently, you will need:
- A C++ compiler. GCC and Clang are widely used options.
- CMake for managing the build process.
- A text editor or IDE that supports C++ with features like syntax highlighting and debugging, such as Visual Studio, CLion, or VS Code.
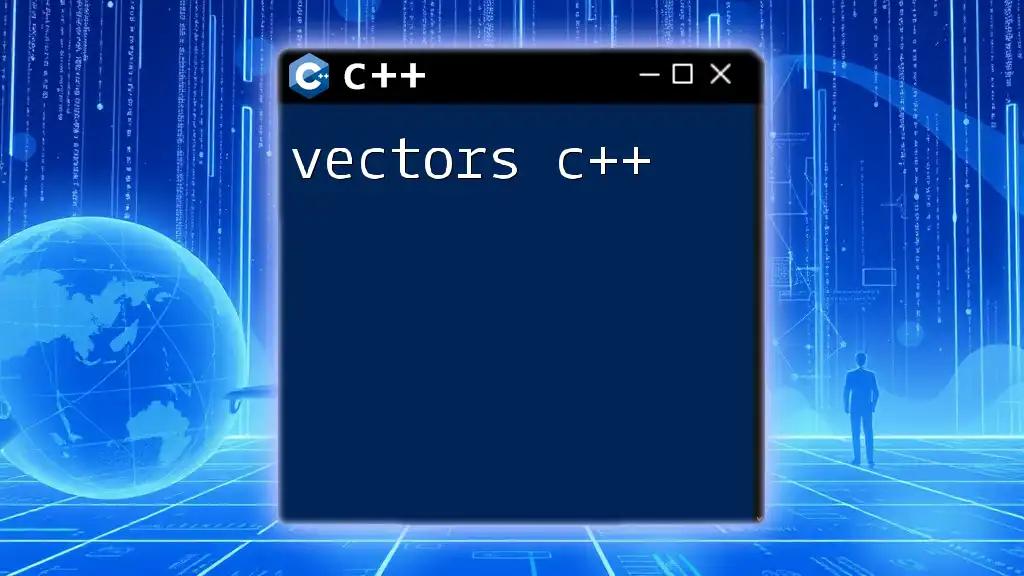
Understanding PyTorch C++ Basics
Core Concepts of Tensor
At its core, PyTorch uses tensors, which are multi-dimensional arrays like NumPy but can run on GPUs, making them essential for high-performance computing.
-
Creating Tensors in C++ You can easily create tensors in C++ using LibTorch. For example, to create a random tensor:
#include <torch/torch.h> // Creating a tensor auto tensor = torch::rand({2, 3}); std::cout << tensor << std::endl; // Outputs a 2x3 tensor filled with random numbers
Basic Operations on Tensors
Arithmetic Operations
PyTorch supports a variety of arithmetic operations on tensors. For example:
auto tensor1 = torch::tensor({1, 2, 3});
auto tensor2 = torch::tensor({4, 5, 6});
auto result = tensor1 + tensor2;
std::cout << result << std::endl; // Outputs: [5, 7, 9]
You can also perform element-wise multiplication or other arithmetic operations similarly.
Indexing and Slicing
Indexing and slicing in PyTorch C++ is reminiscent of slicing in Python. Access elements or sub-tensors easily:
auto my_tensor = torch::tensor({1, 2, 3, 4});
auto sliced = my_tensor.index({"...", torch::indexing::Slice(0, 2)});
std::cout << sliced << std::endl; // Outputs: [1, 2]
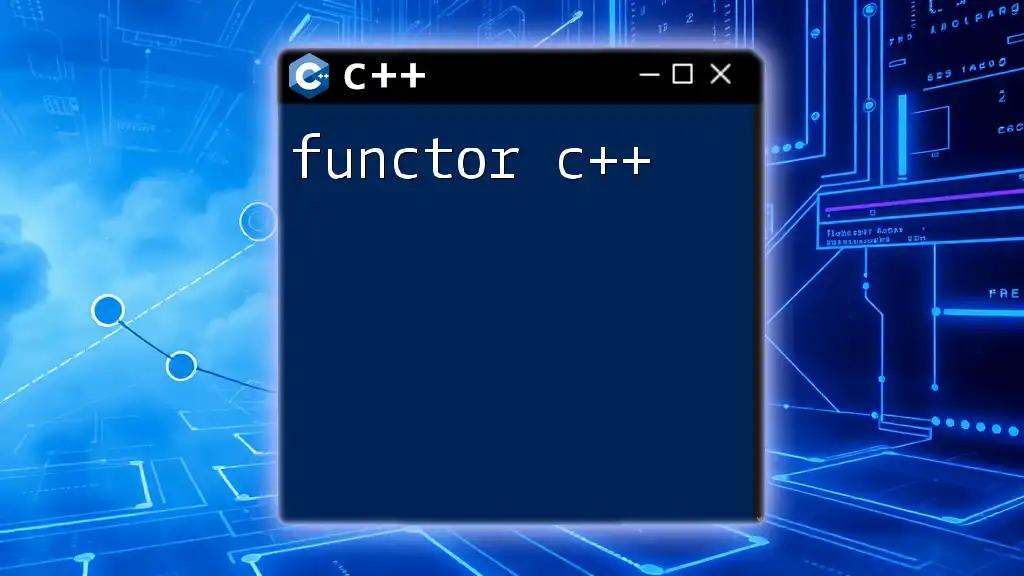
Building Neural Networks with PyTorch C++
Defining a Simple Neural Network
PyTorch C++ allows you to construct neural networks using custom module classes. Here's an example:
struct Net : torch::nn::Module {
Net() {
fc1 = register_module("fc1", torch::nn::Linear(784, 256));
fc2 = register_module("fc2", torch::nn::Linear(256, 10));
}
torch::Tensor forward(torch::Tensor x) {
x = torch::relu(fc1->forward(x.view({-1, 784})));
return fc2->forward(x);
}
torch::nn::Linear fc1{nullptr}, fc2{nullptr};
};
This code constructs a simple feedforward neural network with an input layer, one hidden layer, and an output layer.
Training the Network
To train your network, you need to define a training loop and set up a loss function and optimizer:
-
Loss Functions and Optimizers You can utilize `torch::nn::CrossEntropyLoss` for classification tasks and `torch::optim::SGD` for stochastic gradient descent optimization.
-
Example Training Loop
for (size_t epoch = 1; epoch <= num_epochs; ++epoch) {
for (auto& batch : *data_loader) {
optimizer.zero_grad(); // Reset gradients
auto output = model->forward(batch.data); // Forward pass
auto loss = criterion(output, batch.target); // Calculate loss
loss.backward(); // Backpropagation
optimizer.step(); // Update weights
}
}
This is a skeleton of a basic training loop, integrating the forward pass, loss calculation, and backpropagation steps.
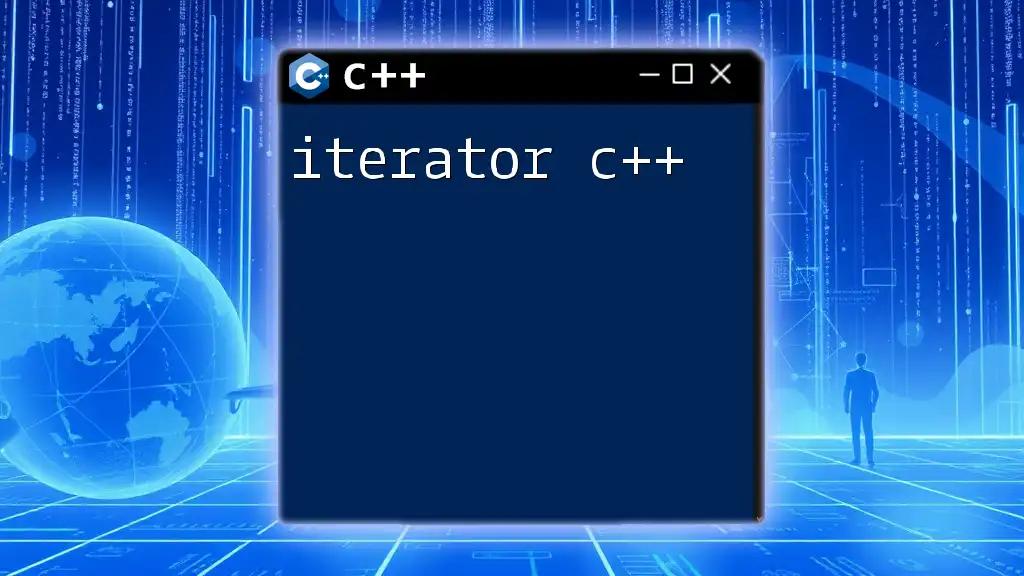
Advanced Features of PyTorch C++
Data Loading and Preprocessing
Efficient data handling is critical for successful training. PyTorch C++ offers the `torch::data` API, facilitating the creation of custom datasets and data loaders.
Saving and Loading Models
When training is complete, saving and loading your models is straightforward using `torch::save` and `torch::load`:
// Save model
torch::save(model, "model.pt");
// Load model
torch::load(model, "model.pt");
This functionality ensures that models can be persisted and shared conveniently, making deployments much easier.
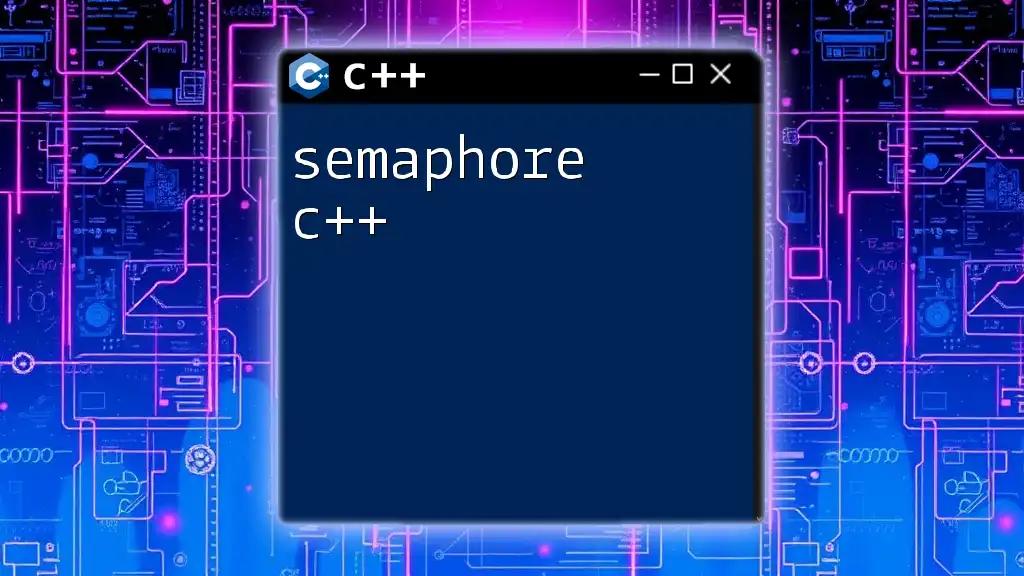
Troubleshooting Common Issues
Debugging Tips for PyTorch C++
Debugging in C++ can be challenging. Here are a few tips to navigate common pitfalls:
- Check syntax and data types diligently; mismatches can lead to compilation errors.
- Use logging to monitor tensor shapes and values during the training process, making it easier to trace issues.
Performance Optimization Techniques
In C++, performance tuning is crucial. Utilize the following techniques:
- Optimize memory usage by selecting appropriate tensor types (e.g., float vs. double).
- Employ multi-threading for data loading to avoid bottlenecks during training.
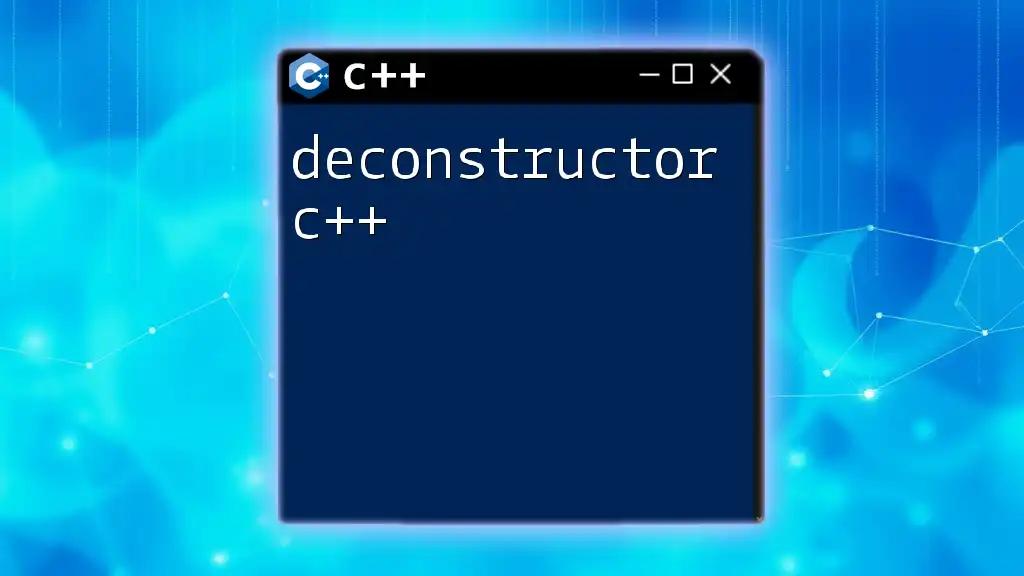
Conclusion
In summary, PyTorch C++ provides a robust framework for developing high-performance machine learning models. With its seamless integration of efficient computation, dynamic graphs, and easy deployment options, it empowers developers to leverage the power of C++ in deep learning applications. Whether you're interested in engaging in research or deploying production models, mastering PyTorch C++ will be invaluable.
Resources for Further Learning
For more in-depth knowledge, the official PyTorch documentation and community forums offer an immense resource pool to dive deeper into PyTorch C++ usage and best practices.
Call to Action
We encourage you to explore the nuances of PyTorch in C++, experiment with your models, and share your experiences with the community. The world of machine learning awaits your innovative insights!