The `atoi` function in C++ is used to convert a string to an integer, effectively parsing the integer value from a sequence of characters.
Here's a simple example:
#include <iostream>
#include <cstdlib> // for atoi
int main() {
const char* str = "1234";
int num = atoi(str);
std::cout << "The integer value is: " << num << std::endl; // Output: The integer value is: 1234
return 0;
}
Understanding `atoi`
`atoi`, which stands for ASCII to Integer, is a function provided in C++ that facilitates the conversion of a string (character array) representing a numerical value into its equivalent integer type. This function plays a crucial role in applications where user input is often given in a string format, such as command-line arguments or string data from files, requiring conversion into integers for processing.
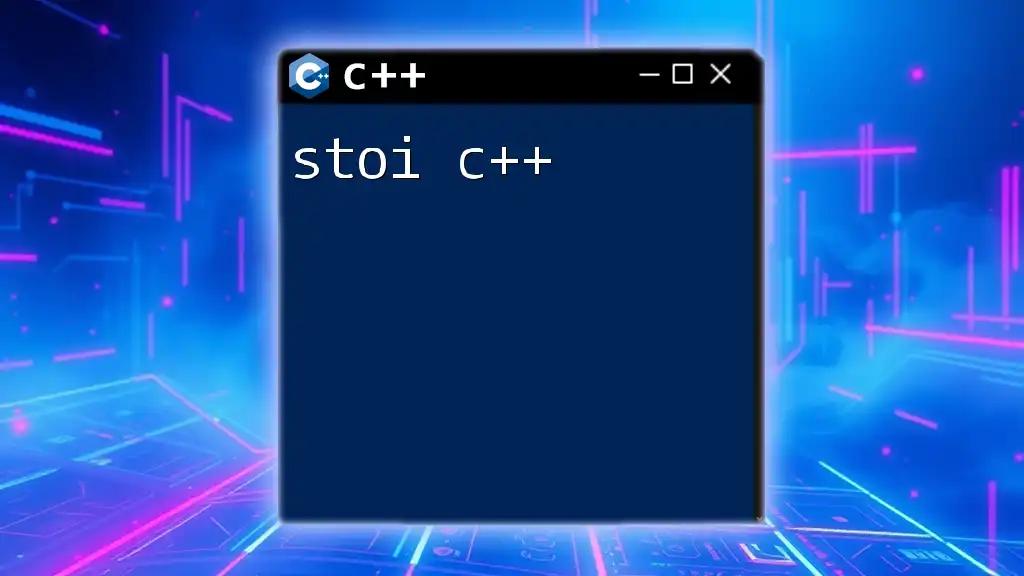
Importance of `atoi` in C++ Programming
In programming, especially when handling user input or parsing structured text, there comes a time when a developer needs to take a string that represents a number and convert it for mathematical operations. `atoi` serves this purpose efficiently. Its simplicity allows for quick conversions, which is particularly advantageous in scenarios where performance matters.
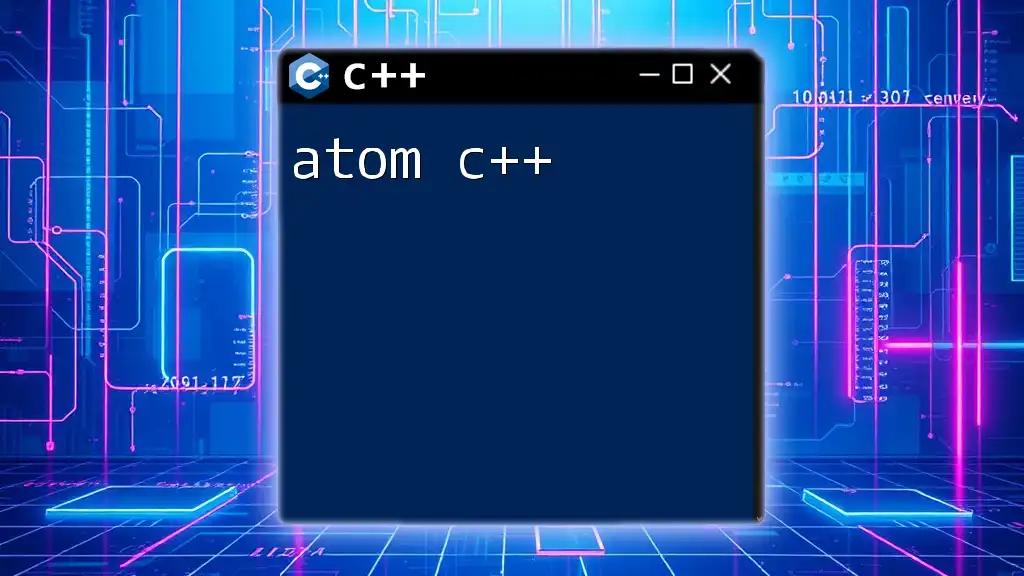
Syntax of `atoi`
Basic Syntax
To utilize the `atoi` function, it follows a straightforward syntax:
int atoi(const char *str);
- The parameter `str` is a pointer to a character array (C-string) representing the numerical value.
- The function returns an `int`, which is the converted numerical value derived from the string.
Header File Requirement
To use `atoi`, you must include the header file `<cstdlib>` at the beginning of your program:
#include <cstdlib>
Failure to include this header results in a compilation error, as the compiler won't recognize the function, highlighting the importance of including necessary libraries in your C++ programs.
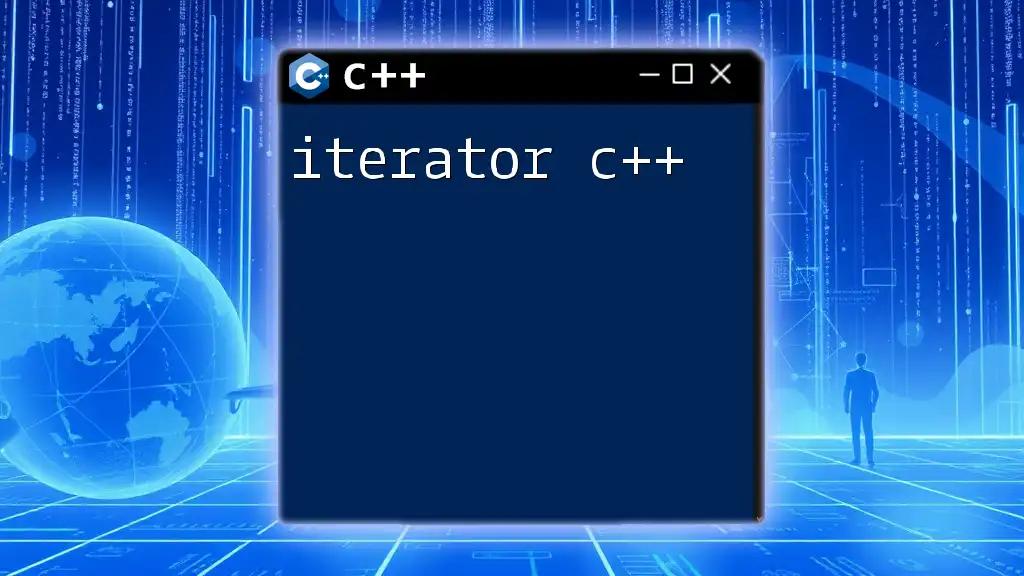
How `atoi` Works
Conversion Process
The functionality of `atoi` requires a thorough understanding of how it processes input strings. When a string is passed to `atoi`, the function reads each character from left to right, identifying numeric characters (0-9), and it stops processing as soon as it encounters a non-numeric character. If the string contains valid characters before any non-numeric character, those characters are converted into their integer representation.
Handling Whitespace
`atoi` gracefully handles leading whitespace in the input string. For example, the following string:
const char* str = " 456";
int number = atoi(str); // number would be 456
This showcases how `atoi` ignores leading spaces before processing the digits, demonstrating its robustness in dealing with various input formats.
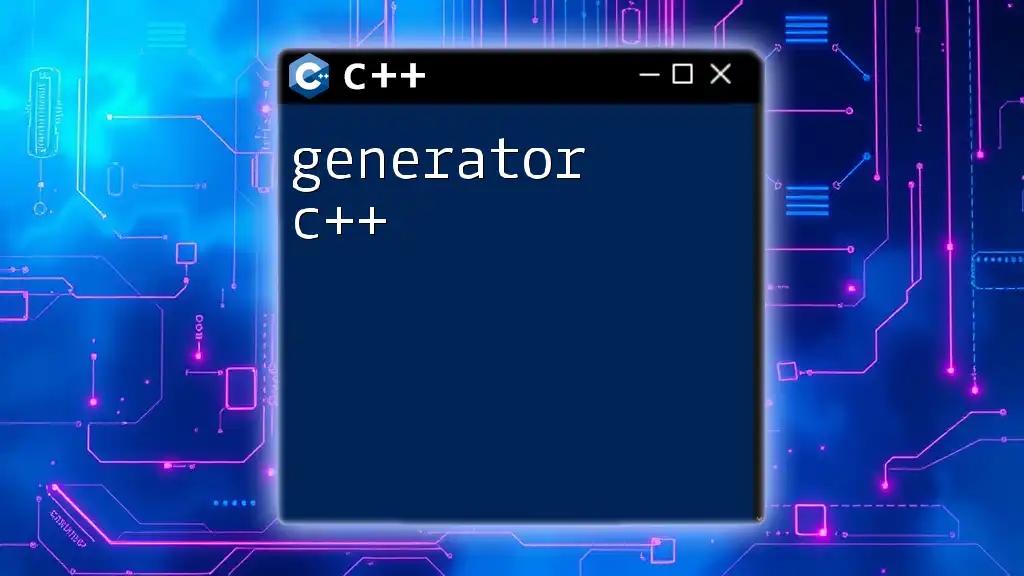
Error Handling with `atoi`
Understanding the Limitations of `atoi`
One significant downside of `atoi` is its lack of error checking. If it encounters a string that cannot be wholly converted into an integer, it will return 0, which could easily lead to misleading results. For instance, if the input string is `"abc"`, the output will be 0, but this could be confused with a valid conversion from the string `"0"`.
Common Mistakes
Developers often run into pitfalls when using `atoi`, primarily due to its leniency with input:
- Providing a string with non-numeric characters, which can yield incorrect conversions.
- Not accounting for potential integer overflow or underflow. For instance, if the string represents a number too large to fit in an integer type, the behavior of `atoi` can lead to undefined results.
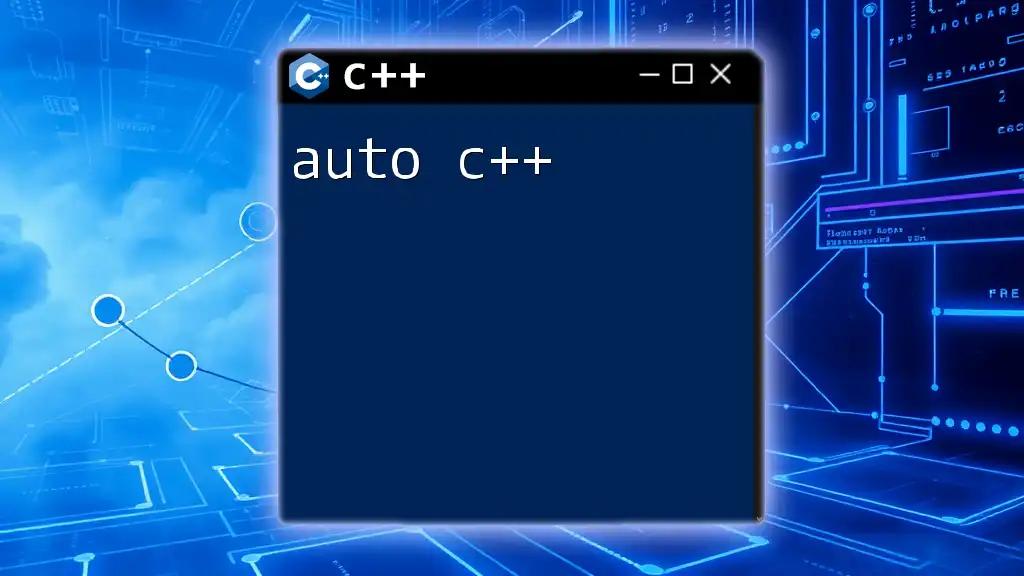
Best Practices for Using `atoi`
Using `atoi` with Valid Inputs
When using `atoi`, ensure the input string is validated before calling the function. Here’s an example of properly handling expected inputs:
const char* validNumString = "1234";
int validNumber = atoi(validNumString); // validNumber would be 1234
Alternatives to `atoi`
Due to the shortcomings of `atoi`, many developers opt for alternatives. One notable replacement is `std::stoi`, introduced in C++11, which provides error handling capabilities.
- Comparison between `atoi` and `std::stoi`: while `atoi` provides no error feedback and can lead to confusion, `std::stoi` throws exceptions (like `std::invalid_argument` or `std::out_of_range`) if conversion is impossible.
Using `std::stoi` looks like this:
#include <string>
std::string str = "1234";
int number = std::stoi(str); // number would be 1234
This snippet illustrates how `std::stoi` can offer a safer and more reliable alternative for string-to-integer conversion.
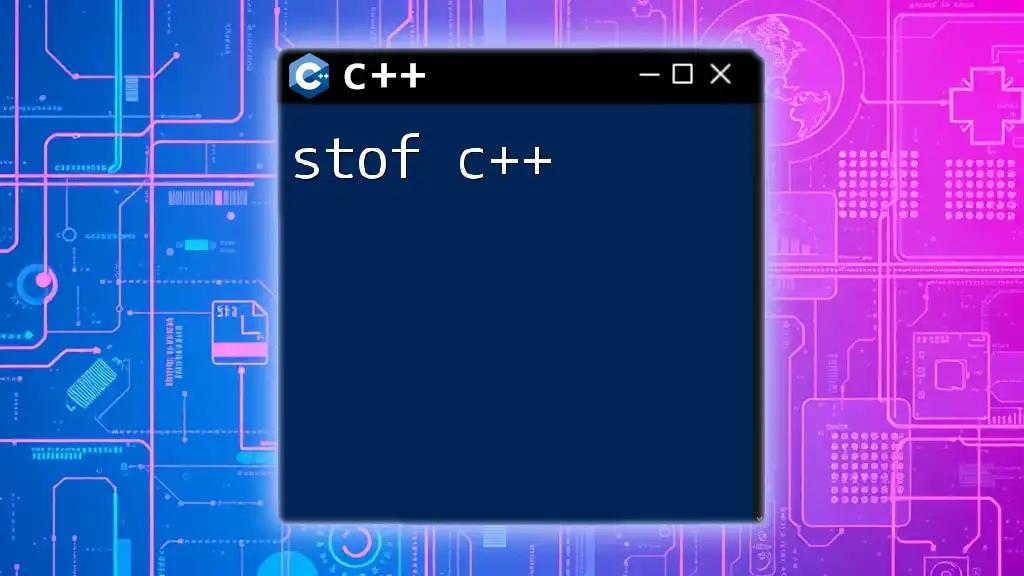
Use Cases for `atoi`
Real-world Examples
One prevalent use case for `atoi` arises when dealing with command-line arguments. Here’s an example that illustrates this usage:
#include <iostream>
#include <cstdlib>
int main(int argc, char *argv[]) {
if (argc > 1) {
int num = atoi(argv[1]);
std::cout << "You entered: " << num << std::endl;
}
return 0;
}
In this scenario, the program reads an integer passed from the command line and converts it from a string representation to an actual integer using `atoi`.
Performance Considerations
While `atoi` is generally efficient, performance can take a hit in scenarios involving large-scale data conversions or frequent calls. Profiling different methods is crucial; if error handling is paramount, switching to `std::stoi` may benefit performance better under conditional checks.
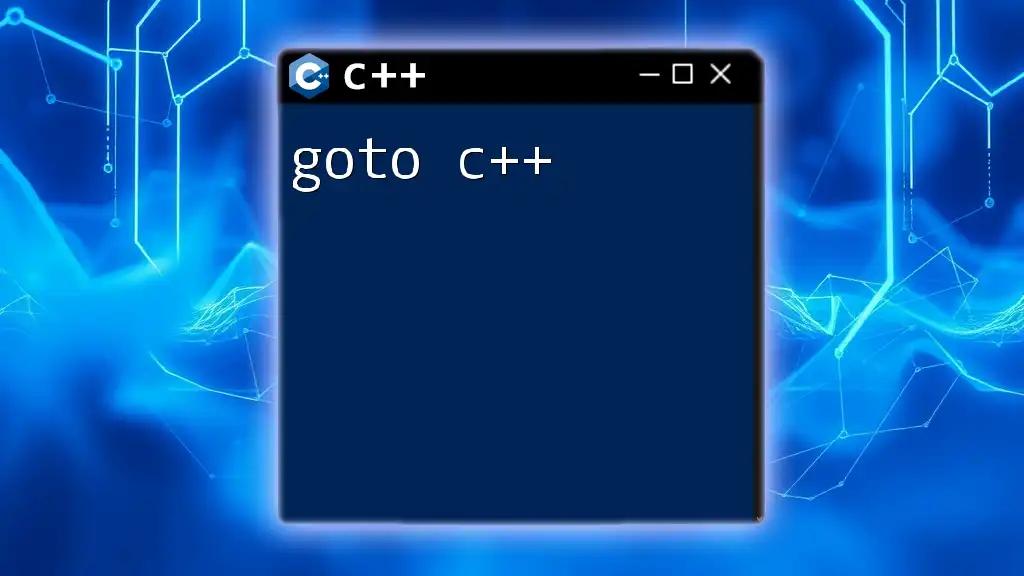
Conclusion
In summary, understanding and leveraging `atoi` is essential for effective C++ programming, particularly when handling string conversions. Although it provides a quick solution for converting strings into integers, developers should be aware of its inherent limitations and consider alternatives for enhanced error handling. Emphasizing the importance of input validation and error checking will lead to more robust and reliable applications in C++. By mastering the use of `atoi` and its alternatives, developers can enhance their applications' functionality and resilience.
Further Reading
For those looking to deepen their understanding of string manipulation and integer conversion in C++, numerous resources such as official documentation, programming tutorials, and community forums provide valuable insights and advanced techniques.