Converting C++ to Java involves translating C++ syntax and constructs into Java equivalents, with special attention to memory management and object-oriented features.
Here’s a simple code snippet illustrating the conversion of a C++ class to Java:
// C++ code
class Animal {
public:
void speak() {
cout << "Animal speaks!" << endl;
}
};
// Java code
class Animal {
void speak() {
System.out.println("Animal speaks!");
}
}
Understanding the Differences Between C++ and Java
Syntax Differences
When you begin to convert C++ to Java, one of the first things you'll encounter is the basic syntax structures that both languages share. While they have similar constructs, key differences do exist.
For instance, the way you declare variables is straightforward:
int a = 10; // C++
int a = 10; // Java
In this example, the syntax is identical, but as you dive deeper, you'll find distinctions in how variables are initialized, especially with regard to object types and certain primitive types.
Memory Management
C++ allows for manual memory management, while Java employs automatic garbage collection. This fundamental difference requires you to recognize how memory is allocated and deallocated in both languages.
In C++, if you allocate memory on the heap using `new`, you must remember to release it using `delete`. Here's an example:
int* ptr = new int; // C++
delete ptr; // C++
In contrast, Java manages memory automatically. When an object is no longer referenced, the garbage collector takes care of deallocation, reducing the risk of memory leaks.
Object-Oriented Paradigms
Both C++ and Java are object-oriented, yet they implement inheritance differently. C++ supports multiple inheritance, allowing a class to inherit features from more than one parent class, as shown below:
class Base1 {};
class Base2 {};
class Derived : public Base1, public Base2 {}; // C++
Java, however, only allows single inheritance, which means a class can only extend one superclass, but can implement multiple interfaces. An example in Java would appear as:
class Base {}
class Derived extends Base {} // Java
Compilation vs. Interpretation
C++ is compiled directly to machine code, making it platform-dependent. This results in highly optimized code but may not run on different architectures without recompilation. Java, on the other hand, is compiled into an intermediate bytecode that runs on the Java Virtual Machine (JVM), enabling cross-platform capability. Understanding this distinction is crucial as you convert C++ to Java, impacting how you think about code compatibility and deployment.
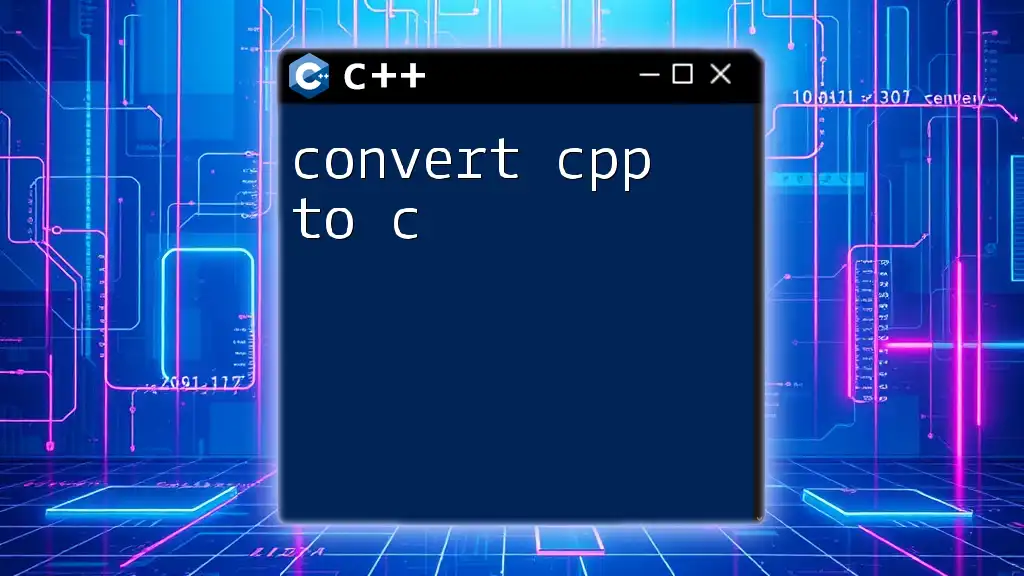
Step-by-Step Approach to C++ to Java Conversion
Step 1: Analyzing the C++ Code
Before you convert C++ code to Java, take the time to thoroughly analyze the existing C++ code. Understanding its structure, what libraries it utilizes, and how it accomplishes its tasks will provide a solid foundation for your conversion efforts. Focus on key areas such as functionality and performance-critical sections.
Step 2: Translating Data Types
Data type conversions are essential. Below is a comparison of some common data types:
- C++ `int` maps to Java `int`
- C++ `float` maps to Java `float`
- C++ `double` maps to Java `double`
- C++ `std::string` maps to Java `String`
Here's an illustration of how you might translate a C++ string to Java:
std::string str = "Hello"; // C++
String str = "Hello"; // Java
Familiarizing yourself with these translations will streamline your overall conversion process.
Step 3: Converting Control Structures
Control structures in C++ and Java are largely parallel; the syntax generally remains unchanged. For instance, an `if` statement looks identical:
if (a > b) { /* code */ } // C++
if (a > b) { /* code */ } // Java
However, ensure that logical operators and conditions are properly ported. Minor details can impact functionality.
Step 4: Handling Functions
When converting functions, note that both languages define functions similarly. However, you'll need to adapt the visibility and accessibility. Here’s an example:
void func() { /* code */ } // C++
void func() { /* code */ } // Java
Recognize that Java has stricter access control, so you may need to adjust visibility modifiers according to the object's lifecycle.
Step 5: Object and Class Conversion
When dealing with classes, Java lacks certain C++ features, such as destructors. In C++, you'd write:
class MyClass {
public:
~MyClass() { /* cleanup code */ } // Destructor in C++
};
In Java, memory is handled automatically, so you wouldn’t have a destructor explicitly:
class MyClass {
// No Destructor in Java
}
Keep in mind that constructors in both languages are similar but should always be defined with the same name as the class.
Step 6: Translating Libraries and APIs
Identifying libraries in C++ and locating the appropriate Java counterparts is crucial. Both languages have extensive libraries, but their APIs can differ significantly. For instance, C++ uses `iostream` for input/output, whereas in Java, you might use `java.io.*`.
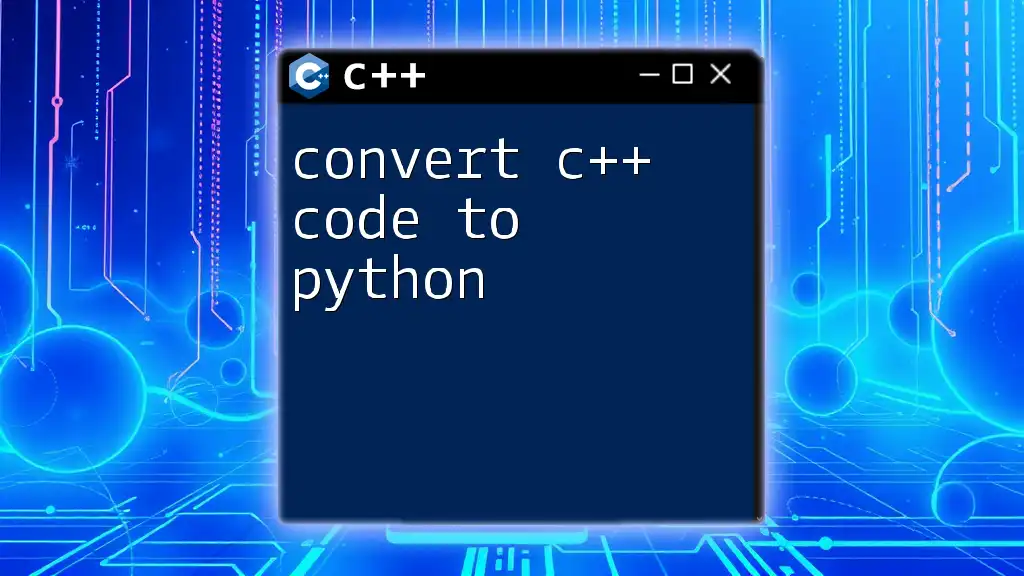
Common Pitfalls and How to Avoid Them
Memory Management Issues
One of the most common pitfalls is not recognizing the implications of manual memory management versus garbage collection. Ensure that any C++ destructors or manual memory management practices are either replicated correctly in Java or omitted, considering Java's garbage collector.
The Use of Pointers
In C++, pointers are widely used, but they do not exist in Java. Always think about the logic behind pointer usage and refactor the code to utilize references instead. This can initially complicate the convert C++ to Java process but is crucial for maintaining functionality.
Exception Handling Differences
Exception handling in C++ and Java diverges significantly. For instance, in C++, you might write:
try {
// code that may throw
} catch (const std::exception& e) {
// handle exception
} // C++
In Java, you would structure it as follows:
try {
// code that may throw
} catch (Exception e) {
// handle exception
} // Java
Best Practices for Converting Code
Maintain Readability: Ensure that your converted code remains clean and maintainable. Following naming conventions and keeping the code structure organized will ease understanding.
Testing Converted Code: After conversion, implementing thorough unit tests is vital to confirm that the code you’ve migrated operates as expected in Java. This ensures that logic and functionality are preserved.
Performance Considerations: Be mindful of performance implications. While Java's garbage collection simplifies memory management, it may introduce latency, particularly in large applications. Profiling your Java code post-conversion can reveal performance bottlenecks.
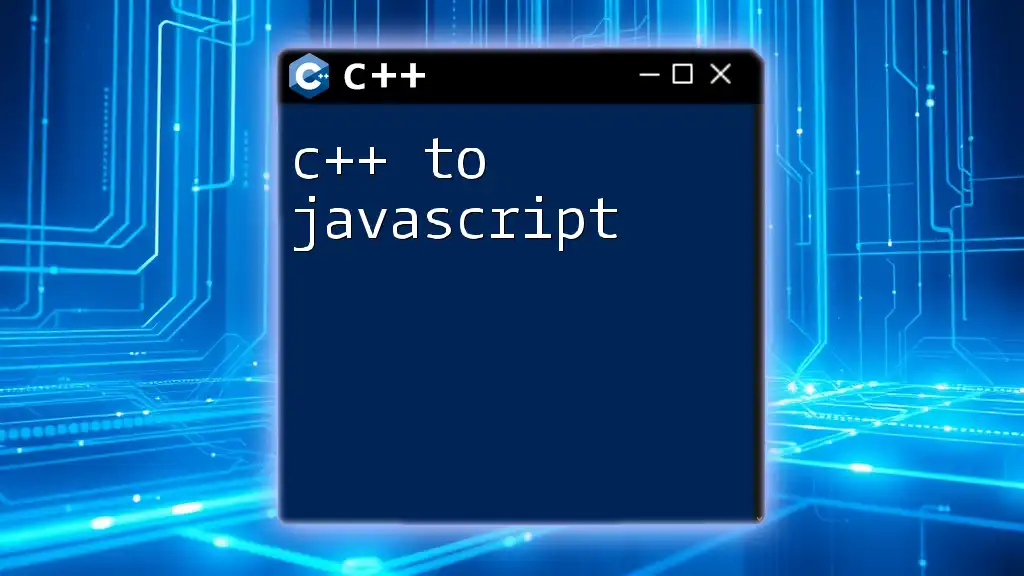
Conclusion
Successfully converting C++ to Java requires patience and attention to detail. By following the outlined steps and being aware of the fundamental differences between the languages, you can effectively translate code while preserving its original functionality. Remember that practice is the key—experiment with sample projects to hone your skills further.
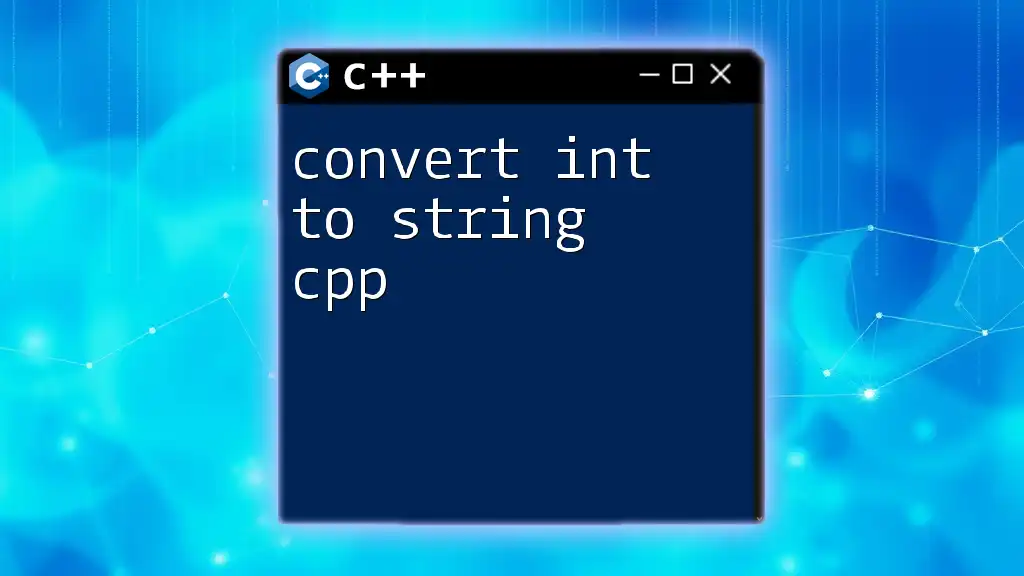
Resources
As you embark on your journey to convert C++ to Java, consider exploring recommended books, online tutorials, and community forums where you can seek help, share experiences, and learn best practices from fellow developers.