Converting a C program to C++ typically involves adding C++ features like classes and namespaces, while maintaining the core C logic; here's a simple example of converting a C-style function to a C++ class method:
#include <iostream>
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
};
int main() {
Calculator calc;
std::cout << "Sum: " << calc.add(5, 3) << std::endl;
return 0;
}
Understanding the Basics
C Language Fundamentals
C is a powerful procedural programming language primarily used for system programming and developing applications requiring high performance. The syntax is straightforward, focusing on functions and structured data types. C is known for its efficiency and control over system resources, making it ideal for low-level programming tasks.
C++ Language Fundamentals
C++, on the other hand, builds upon the foundation of C by introducing object-oriented programming (OOP) principles. This allows for code organization and reusability through concepts like classes and inheritance. C++ retains all of C’s features while offering more advanced capabilities, such as templates and exception handling. Understanding these differences is crucial when deciding to convert C programs to C++.

Why Convert C Code to C++?
Converting C code into C++ can yield several benefits:
-
Enhanced Code Reusability: C++ enables the use of classes and objects, which promote encapsulation and modular design.
-
Improved Memory Management: The introduction of constructors and destructors allows for automatic resource management, reducing memory leaks.
-
Exception Handling: C++'s features simplify error management with structured exception handling, providing a cleaner approach than traditional error codes.
-
Standard Template Library (STL): C++ offers powerful predefined classes and functions for handling collections of data efficiently.
These benefits make the conversion worthwhile, especially for larger projects aiming for long-term maintainability.
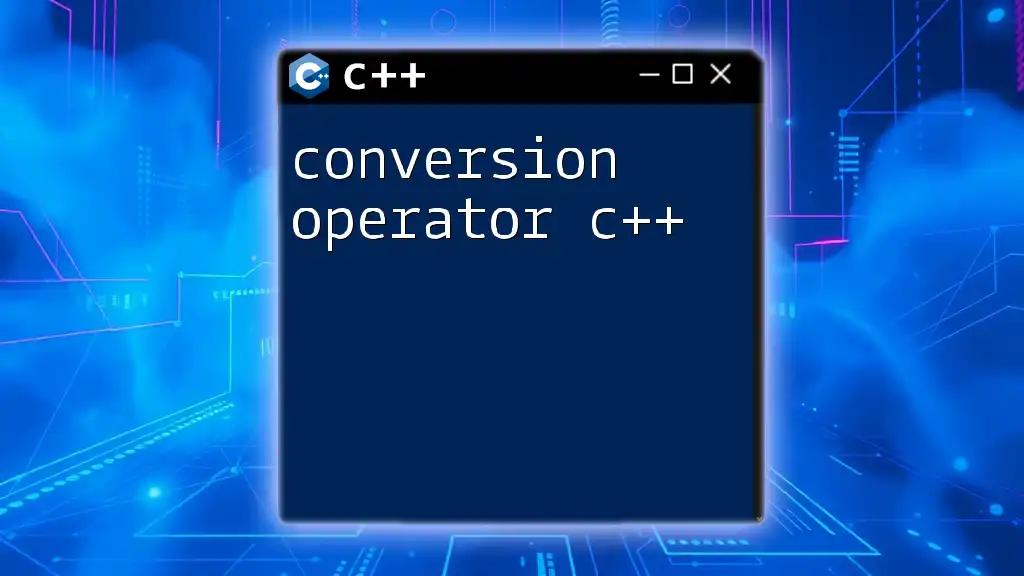
Preparing for the Conversion
Assessing Your C Code
Before undertaking the conversion, evaluate your existing C code. Identify sections that can be directly translated and those requiring modifications. This step ensures a smoother transition while minimizing potential issues.
Setting Up Your Development Environment
Choose a robust IDE that supports both C and C++, such as Visual Studio, Code::Blocks, or CLion. Ensure that your compiler can handle both languages, allowing you to test your converted code thoroughly.
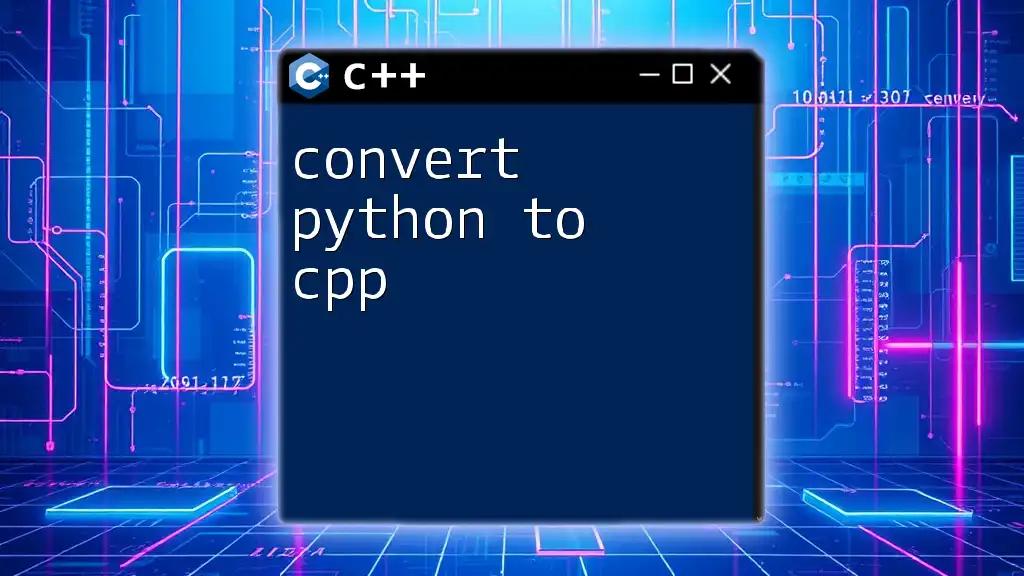
Step-by-Step Process to Convert C Code to C++
Converting Basic Data Types
C and C++ share many fundamental data types; however, C++ adds support for stronger type checking. When converting:
// C Code
int a = 10;
float b = 5.5;
// Converted C++ Code
int a = 10;
float b = 5.5f; // Specifying 'f' for float explicitly
Transitioning Functions and Libraries
In C, you typically use header files like `stdio.h` for input and output. In C++, you should transition to using `iostream`:
// C Code
#include <stdio.h>
void printHello() {
printf("Hello, World!\n");
}
// Converted C++ Code
#include <iostream>
void printHello() {
std::cout << "Hello, World!" << std::endl;
}
This change not only modernizes your code but also leverages C++'s features.
Transforming Structs to Classes
While both C and C++ use structs, C++ allows these to have methods and access modifiers, turning them into full-fledged classes. Here’s how to convert:
// C Code
struct Rectangle {
int width;
int height;
};
int area(struct Rectangle rec) {
return rec.width * rec.height;
}
// Converted C++ Code
class Rectangle {
public:
int width;
int height;
int area() {
return width * height;
}
};
Handling Memory Management
In C, dynamic memory is allocated using `malloc` and deallocated with `free`. C++ simplifies this with `new` and `delete`:
// C Code
int* arr = (int*)malloc(5 * sizeof(int));
// Use arr...
free(arr);
// Converted C++ Code
int* arr = new int[5];
// Use arr...
delete[] arr; // Notice usage of delete[]
Error Handling Adjustments
While C traditionally uses error codes, C++ introduces try-catch blocks for exception handling. Consider the following examples:
// C Code
if (file == NULL) {
printf("Error opening file.\n");
}
// Converted C++ Code
try {
throw std::runtime_error("Error opening file.");
} catch (const std::runtime_error& e) {
std::cerr << e.what() << std::endl;
}
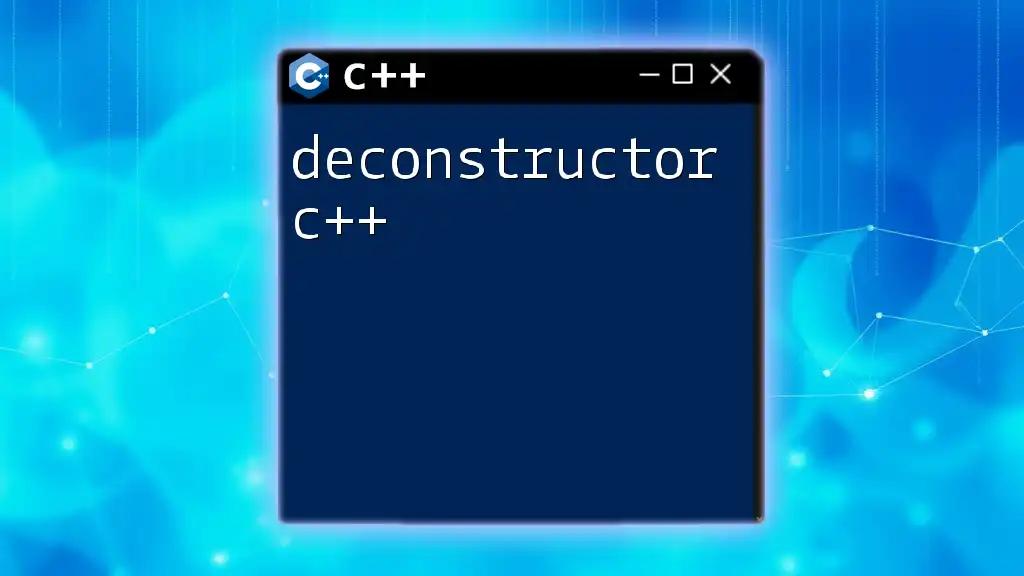
Common Challenges During Conversion
Syntax Differences
During the conversion process, syntactical inconsistencies may arise. For instance, C requires main to return an integer explicitly, while C++ also allows constructors to replace the main function. Here’s a typical mistake and its correction:
// C Code
int main() {
return 0;
}
// Converted C++ Code
int main() {
return 0; // Still valid in C++
}
Dependencies and Compatibility Issues
When integrating existing C libraries, ensure that you use `extern "C"` to prevent name mangling in C++. This can prevent potential linking errors when compiling:
extern "C" {
#include "some_c_library.h"
}

Best Practices for C to C++ Translation
Write Clean and Maintainable Code
As you convert, prioritize code readability. Use meaningful variable names and apply proper indentation. Well-structured C++ code might look like this:
class Circle {
public:
Circle(double r) : radius(r) {}
double area() const {
return 3.14159 * radius * radius;
}
private:
double radius;
};
Utilizing C++ Features
Encourage leveraging powerful C++ features that promote efficiency and performance. For example, consider using STL containers like `std::vector` to manage dynamic arrays systematically:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
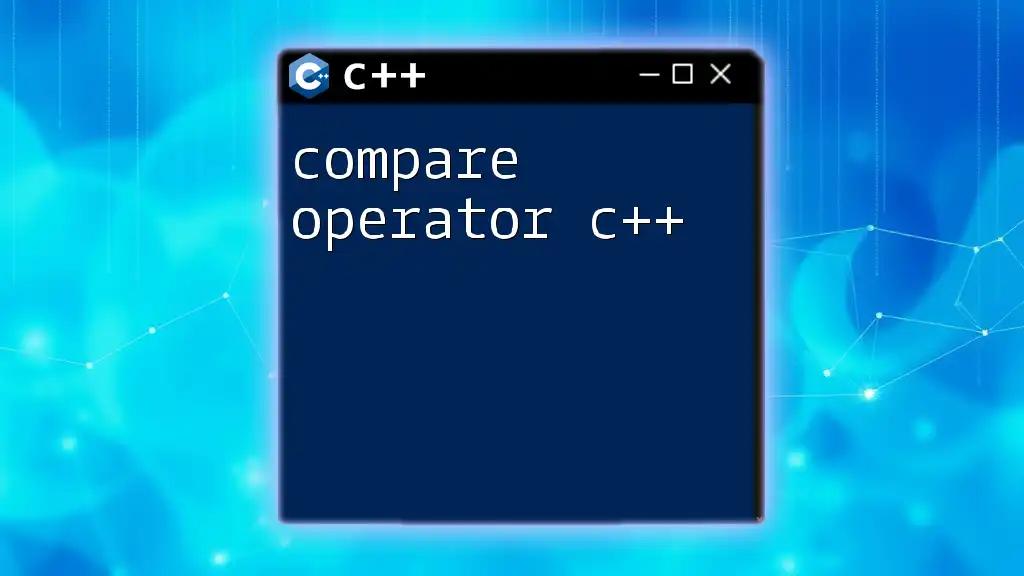
Conclusion
Converting a C program to C++ involves understanding the foundational differences between the languages, assessing your code, and systematically applying various translation techniques. By taking advantage of C++'s advanced features, you can write more efficient, maintainable, and reusable code. With this guide, you are now equipped to embark on your conversion journey and explore the rich possibilities C++ has to offer.