In C++, you can terminate a program using the `return` statement within the `main` function or by using the `exit()` function from the `<cstdlib>` library.
Here’s an example using `return`:
#include <iostream>
int main() {
std::cout << "Ending program...\n";
return 0; // Exits the program
}
And an example using `exit()`:
#include <iostream>
#include <cstdlib>
int main() {
std::cout << "Ending program...\n";
exit(0); // Exits the program
}
Understanding C++ Program Termination
What Does It Mean to End a C++ Program?
Ending a C++ program refers to the process of halting its execution and returning control to the operating system. Proper termination ensures that resources are released, and all the necessary clean-up processes are carried out. Understanding the nuances of how a program is ended is crucial for writing efficient, error-free software.
Common Scenarios for Ending a Program
There are several scenarios when a program might need to be ended:
- User-initiated termination, such as pressing Ctrl+C in the console.
- Error handling scenarios where an unexpected condition arises.
- Natural conclusion, which occurs when the code execution reaches the end of the `main()` function.
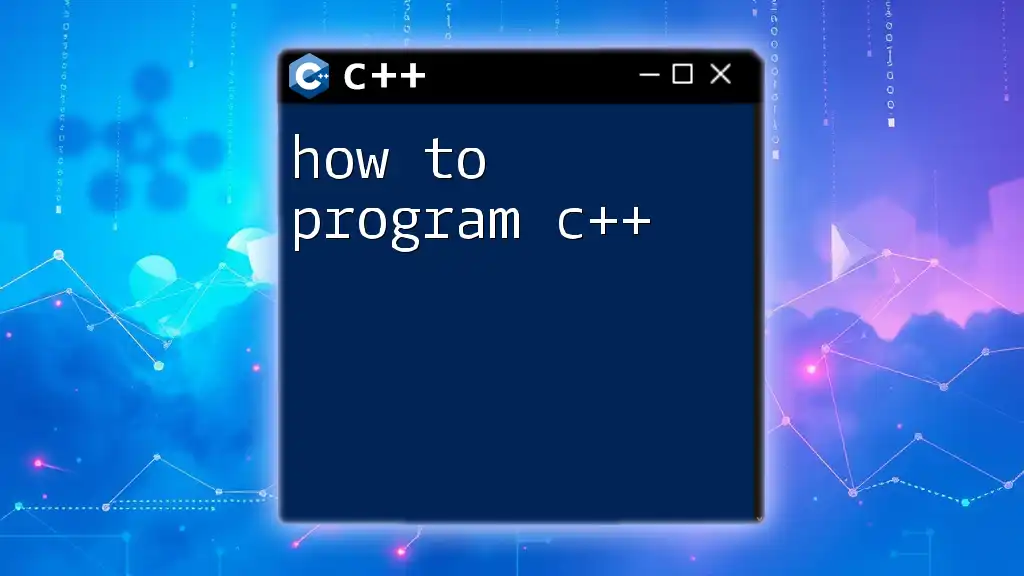
C++ Methods for Ending a Program
Using `return` Statement
The simplest and most common method to end a C++ program is through the `return` statement. This statement is used within the `main()` function, and it conveys the exit status of the program back to the operating system. A return value of `0` typically indicates a successful termination, while any non-zero value signifies an error.
Example:
int main() {
// your code here
return 0; // Ends the program successfully
}
In this example, the program will terminate with an exit status of `0`, indicating everything went smoothly.
Using `exit()` Function
The `exit()` function provides another way to end a program immediately from anywhere within a code block and is defined in the `<cstdlib>` header. Unlike `return`, which only terminates the `main()` function, `exit()` will terminate a program no matter where it is called.
Differences between `return` and `exit()`:
- `return` can only be used within the `main()` function, while `exit()` can be called from anywhere.
- `exit()` requires you to specify an exit code, which informs the operating system about the reason for termination.
Example:
#include <cstdlib> // Required for exit()
int main() {
// your code here
exit(1); // Ends the program with an error code
}
In this example, the program calls `exit(1)`, indicating an error occurred.
Using `abort()` Function
The `abort()` function is another option, which immediately terminates the program without performing any cleanup. It is defined in the `<cstdlib>` header as well. This function is best used in situations that require immediate termination due to critical errors.
Example:
#include <cstdlib>
int main() {
// An unrecoverable error occurs
abort(); // Terminates the program immediately
}
Using `abort()` can be risky, as it does not allow for resource cleanup and may leave behind locks or unsaved data.
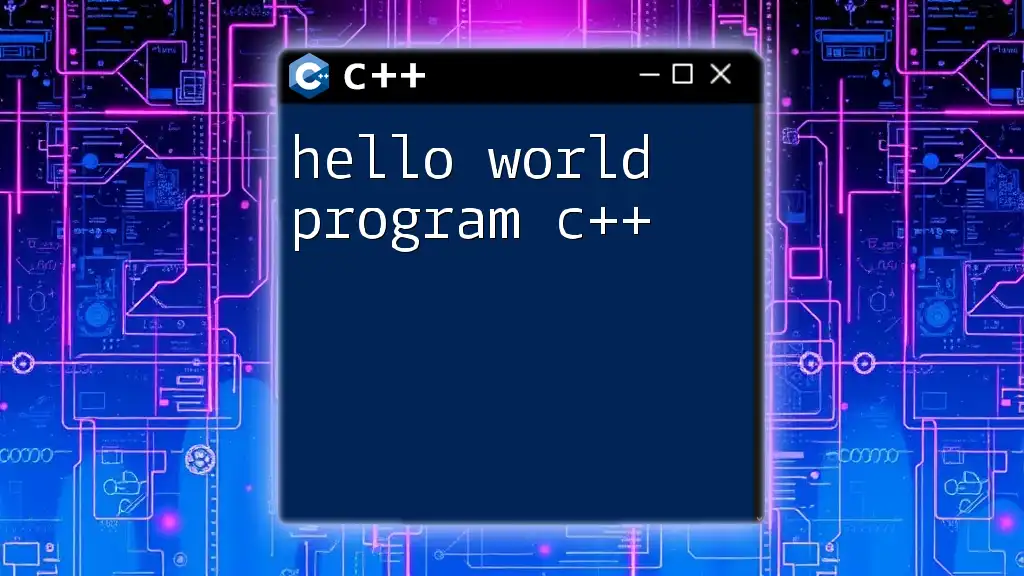
Graceful vs. Abrupt Program Termination
What is Graceful Termination?
Graceful termination refers to the process of ending a program in an orderly manner. It allows for the release of resources, such as memory and file handles. It is essential for maintaining system stability and avoiding resource leaks - conditions that can lead to a slowdown or crashes in long-running applications.
What is Abrupt Termination?
Abrupt termination occurs when a program is stopped immediately without following through with cleanup procedures. This can happen due to unhandled exceptions, calling `abort()`, or external interrupts (such as user commands). Abrupt terminations can lead to issues such as corrupted data, memory leaks, or inconsistent application states.
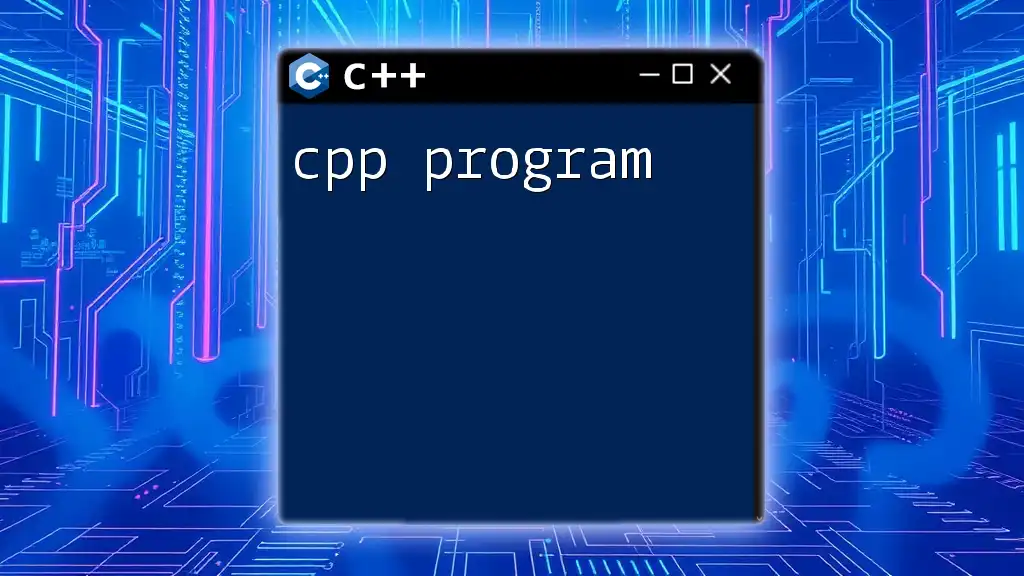
How to Handle Program Termination in C++
Using Exception Handling
In C++, exception handling helps manage errors that may require abrupt program termination. By utilizing `try-catch` blocks, developers can catch exceptions and handle them gracefully, allowing for resource management and clean-up.
Example:
#include <iostream>
#include <exception>
int main() {
try {
// Code that may throw exceptions
throw std::runtime_error("An error occurred");
} catch (std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
exit(1); // Terminate the program after handling exception
}
}
In this case, if an exception occurs, an error message is printed and the program will terminate gracefully with `exit(1)`.
Cleaning Up Resources Before Termination
Cleaning up resources is an important aspect of program termination. This ensures that memory is deallocated and files are closed properly, preventing memory leaks and ensuring data integrity. In C++, this can be effectively managed using destructors and the RAII (Resource Acquisition Is Initialization) principle.
Example:
class Resource {
public:
~Resource() {
// Cleanup code here
std::cout << "Resource released" << std::endl;
}
};
int main() {
Resource res;
// your code here
return 0; // Destructor is called automatically
}
In this program, when `main()` returns, the destructor for the `Resource` class triggers automatically, ensuring proper clean-up.
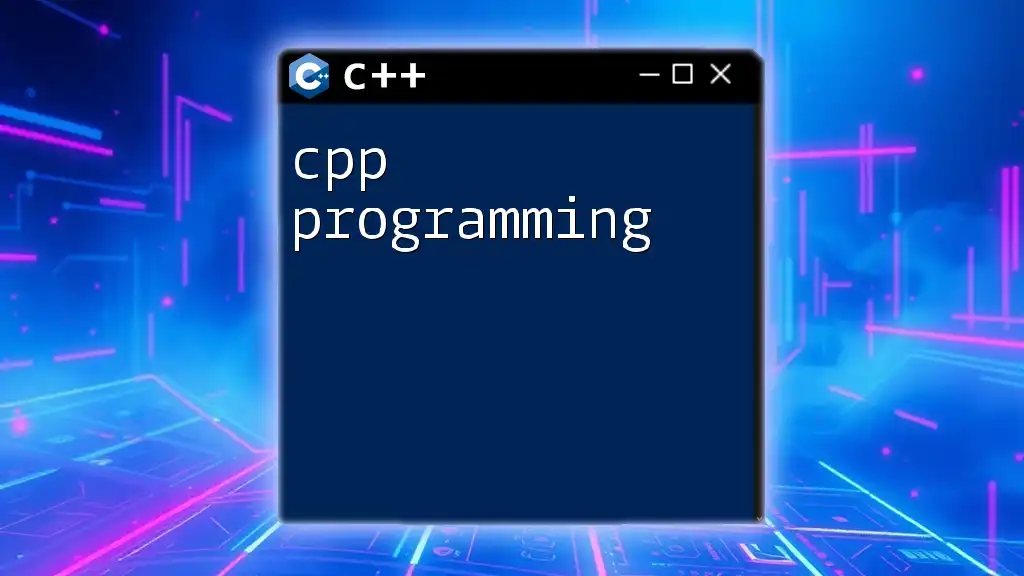
Debugging and Termination
Debugging Techniques Related to Program Ending
When a program does not terminate as expected, you may need to debug it to understand the root cause. Common tools for debugging C++ programs include gdb (GNU Debugger), which allows you to step through your code and inspect variables at runtime.
Common issues that might arise during termination include:
- Infinite loops preventing program completion.
- Unhandled exceptions causing suboptimal exits.
- Resource locks leading to deadlock situations.
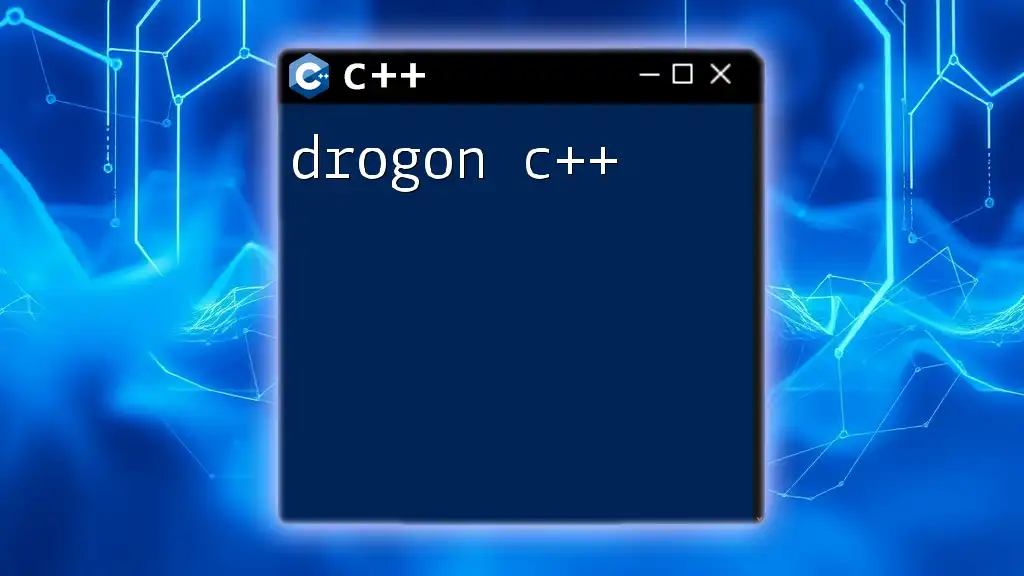
Conclusion
In conclusion, understanding how to end a program in C++ is an essential skill for any developer. Proper program termination not only ensures that resources are managed correctly but also reduces the chances of encountering errors that can compromise application integrity. By using the various methods discussed, developers can choose the most appropriate approach based on their specific needs and scenarios.
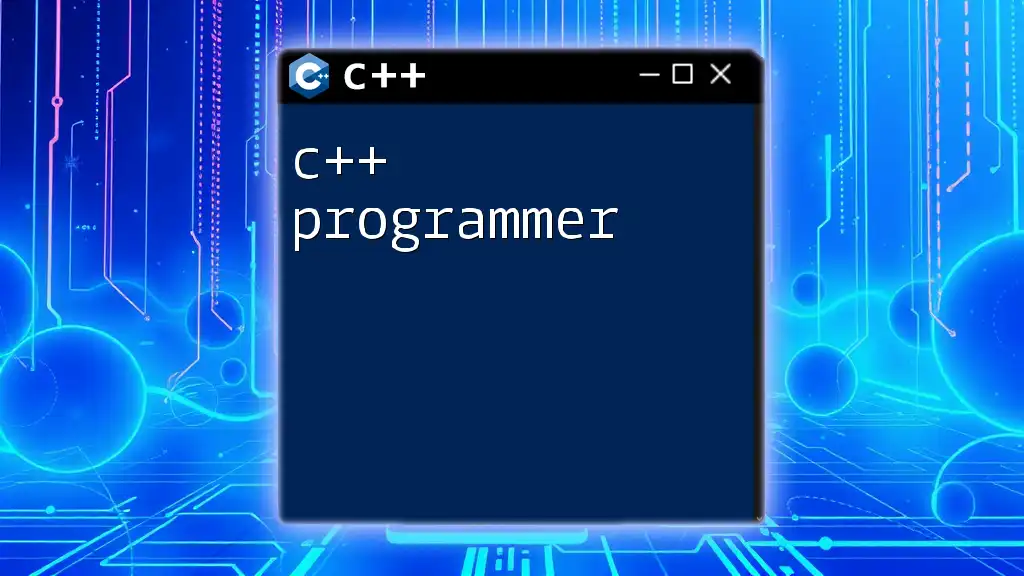
FAQs
What should I do if my program doesn't end properly?
If your program doesn't terminate correctly, check for infinite loops, unhandled exceptions, and proper resource management. Utilizing debugging tools like gdb can help you track down where the program is stuck.
How can I ensure my data is saved before terminating?
To ensure data is saved, incorporate checkpoints in your program where data is regularly written to disk or persisted in some form. Implementing cleanup functions that run during the termination process can also help ensure data integrity.
Can external factors affect program termination?
Yes, external factors such as interruptions (user input, operating system signals) can affect how a program terminates. Make sure your program captures and handles these signals appropriately to manage graceful exits.