A UML diagram for C++ visually represents the structure of a class, including its attributes, methods, and relationships with other classes, which aids in understanding and designing object-oriented software.
class Car {
public:
void start();
void stop();
private:
int speed;
std::string color;
};
What is UML?
The Unified Modeling Language (UML) is a standardized modeling language used to visualize the design of a system. UML provides a set of graphic notation techniques to create visual models of software systems, making it an essential tool in software development.
Importance of UML in Software Development
UML helps bridge the gap between conceptual design and actual implementation. It allows developers, stakeholders, and designers to communicate effectively about how the system should be built and how it will function, ensuring that everyone involved has a clear understanding of the system’s architecture.
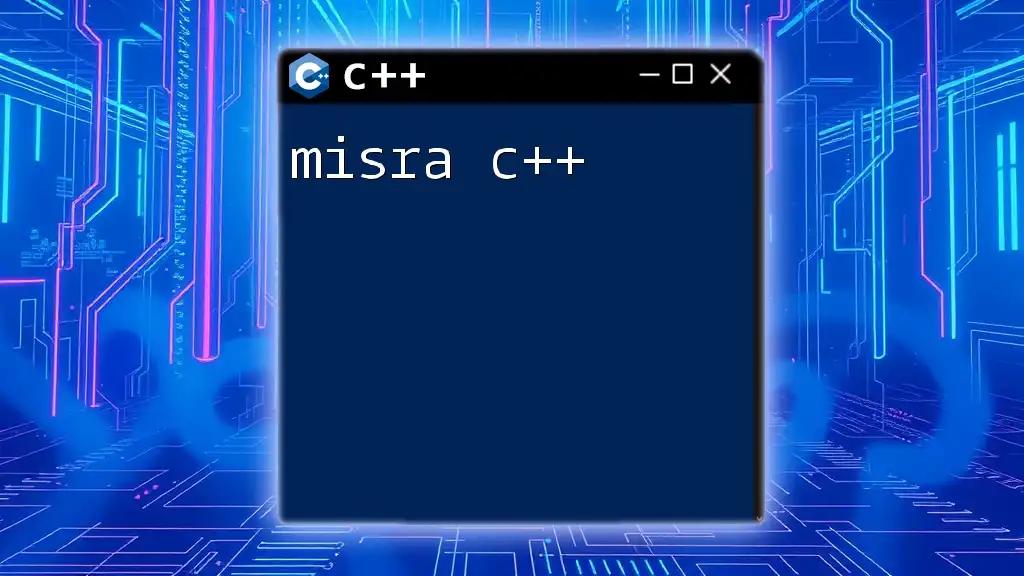
Why Use UML Diagrams in C++?
Integrating UML diagrams into C++ development offers significant advantages:
- Enhanced Readability: UML provides a visual representation of the system, making complex interactions easier to comprehend compared to reading traditional code alone.
- Improved Maintainability: Diagrams serve as documented blueprints for the code, easing future modifications, updates, and debugging processes.
- Promotion of Best Practices: UML encourages developers to think about object-oriented design principles, thereby promoting clean and organized code.
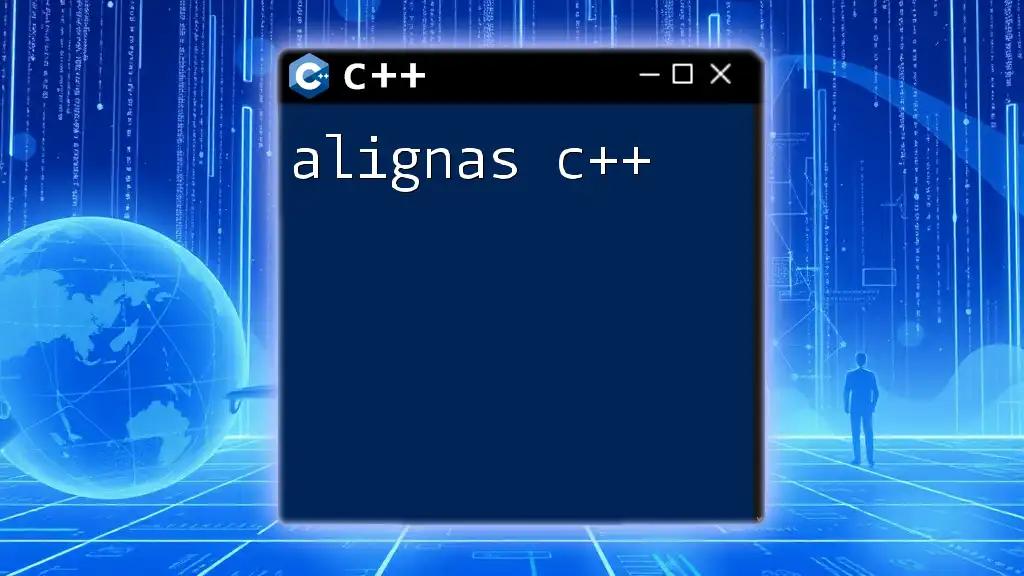
Types of UML Diagrams
Class Diagrams
Class diagrams are the backbone of object-oriented design and describe the classes within a system and their relationships.
Components of Class Diagrams:
- Class: Represents a blue print for objects.
- Attributes: Data members of the class.
- Methods: Functions that define the behavior of the class.
- Relationships: The connections and interactions between classes such as inheritance and associations.
In a simple C++ class diagram, you might depict a `Car` class like this:
+----------------+
| Car |
+----------------+
| - model: string|
| - year: int |
+----------------+
| + start() |
| + stop() |
+----------------+
Sequence Diagrams
Sequence diagrams illustrate how objects interact over time, showcasing the order of messages exchanged.
Purpose: They help visualize the process and identify the flow of control among different parts of the program.
For example, a sequence of operations in a typical C++ application might look like this:
Request -> Server: Process Request
Server -> Database: Fetch Data
Database -> Server: Return Data
Server -> Request: Send Response
Use Case Diagrams
Use case diagrams demonstrate the various functionalities of a system from an end-user perspective. They showcase the interaction between actors (users or other systems) and the system itself.
Components:
- Actors: Represent entities that use the system.
- Use Cases: The functionalities or services the system provides.
- System Boundary: Defines the scope of the system.
For example, the use cases for a C++ application might include user logins and dashboard views.
Activity Diagrams
Activity diagrams model the flow of activities and actions within a system, showing how different parts of a system interact to achieve a goal.
Components:
- Actions: Specific tasks or operations executed within the system.
- Transitions: The movement from one action to another.
- Decisions: Points where branching occurs based on conditions.
Creating UML Diagrams for C++ Applications
Step-by-Step Guide to Drawing Class Diagrams:
- Identify Classes: Start by determining the primary classes that your system will have.
- Define Relationships: Clarify how these classes will interact with one another.
- Add Attributes and Methods: List the necessary data members and functions, defining class operations.
Tools: Several online tools such as Lucidchart, draw.io, and PlantUML can facilitate the creation of UML diagrams, offering templates and easier editing interfaces.
Tips for Creating Effective UML Diagrams
- Keep it Simple: The primary goal of UML is clarity. Avoid creating overly complex diagrams that can confuse rather than enlighten.
- Use Consistent Notation: Adhere to UML standards to maintain uniformity across diagrams, making it easier for team members to interpret.
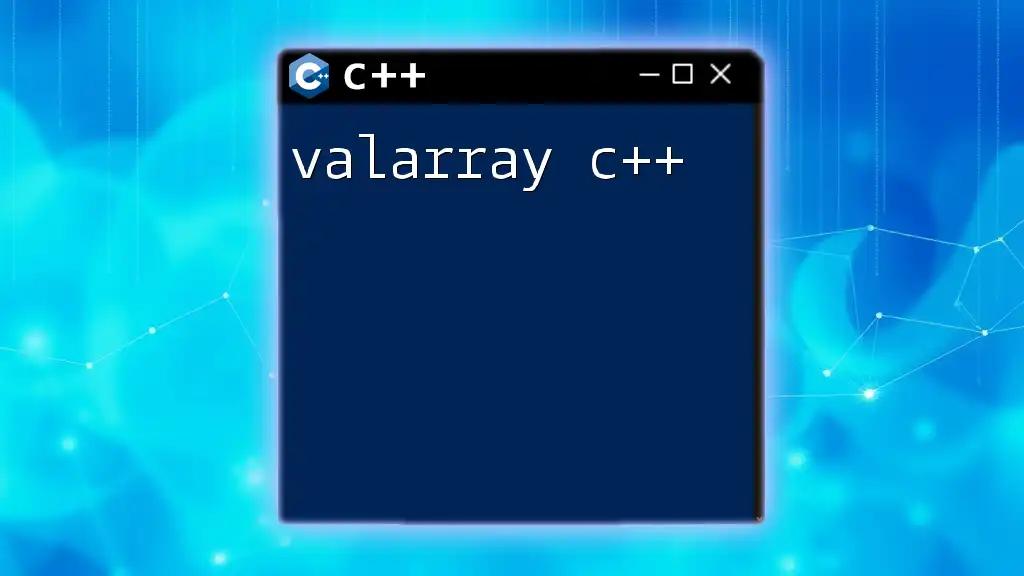
Integrating UML Diagrams with C++ Code
Mapping UML Elements to C++ Constructs
To successfully integrate UML with C++:
- Classes in UML map directly to C++ classes.
- Attributes translate to member variables in C++.
- Methods become functions defined within the class.
Example: Class Diagram to C++ Code
Consider the following UML diagram representing an `Animal` class:
+----------------+
| Animal |
+----------------+
| - name: string |
+----------------+
| + makeSound() |
+----------------+
The C++ implementation of the `Animal` class may look like this:
class Animal {
private:
std::string name;
public:
Animal(const std::string& name) : name(name) {}
void makeSound();
};
This implementation captures the essence of the UML class diagram, providing a clear and cohesive representation of the `Animal` class in C++.

Best Practices for UML in C++
Consistency Across Diagrams
Maintaining consistency is crucial. When drawing UML diagrams, ensure that your symbols and notations align with UML standards. This consistency aids comprehension among team members and stakeholders who interact with your diagrams.
Documentation and Collaboration
UML diagrams significantly enhance documentation efforts. They serve as a common language for technical and non-technical stakeholders, facilitating collaboration and minimizing misunderstandings. By using UML, teams can have clearer discussions about system design and requirements.
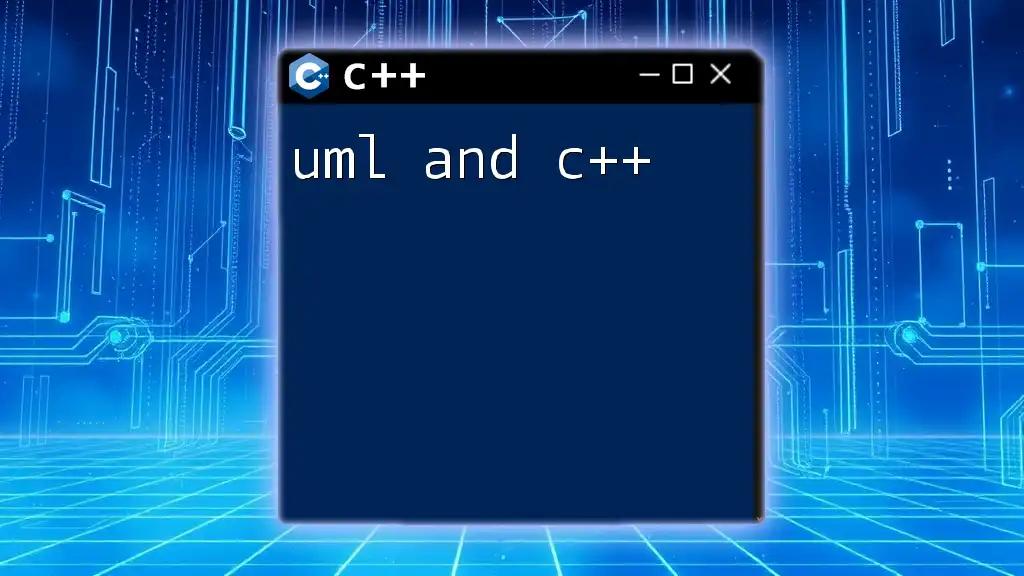
Conclusion
UML diagrams serve as a valuable asset in C++ development, promoting better communication, design clarity, and project organization. By integrating UML into your workflow, you not only enhance your understanding of the application architecture but also improve your coding practices.
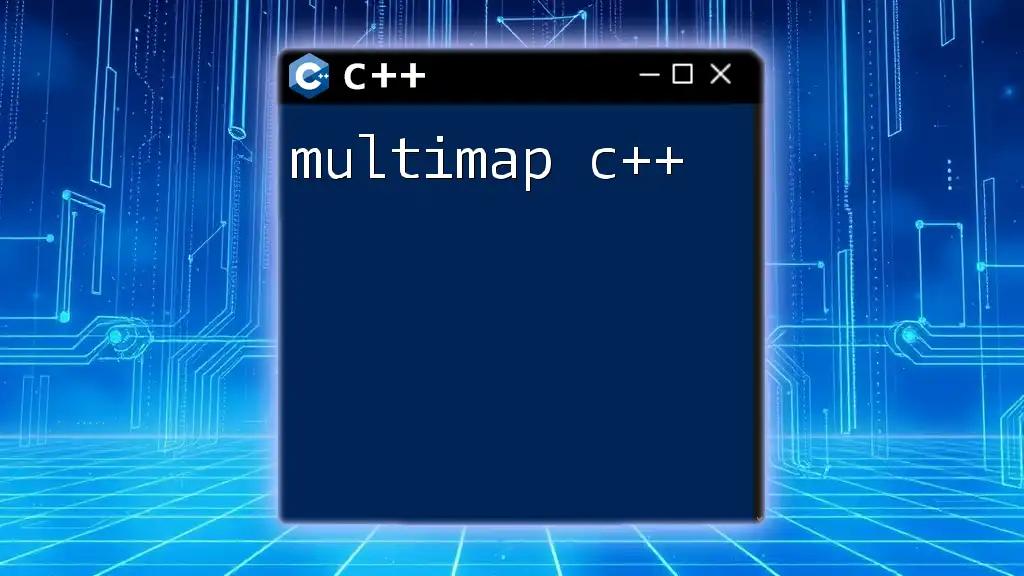
Call to Action
Now that you've grasped the importance of UML diagrams in C++, consider diving deeper into your programming journey with our expert-led tutorials on C++ commands and UML integration—designed to elevate your coding skills to the next level.

Frequently Asked Questions
What software can I use to create UML diagrams?
There are various software tools available for creating UML diagrams, including commercial options like Microsoft Visio, online tools like Lucidchart and draw.io, and open-source software like StarUML.
How do UML diagrams help in debugging?
UML diagrams provide a high-level overview of system architecture, enabling developers to identify errors in design or interactions, and thus make debugging processes more efficient by pinpointing potential flaw areas in the structural design.
Can I use UML diagrams in agile development?
Absolutely! UML is flexible enough to be adopted in agile methodologies. While agile emphasizes iterative development, UML diagrams can still be used to quickly capture designs that evolve throughout the project lifecycle, ensuring that documentation keeps pace with development changes.