In C++, a "flag" generally refers to a command-line argument that influences the behavior of a program, often used to enable or disable specific features.
Here's a sample code snippet demonstrating how to read a flag from the command line in C++:
#include <iostream>
int main(int argc, char *argv[]) {
bool myFlag = false;
for (int i = 1; i < argc; ++i) {
if (std::string(argv[i]) == "--enable-feature") {
myFlag = true;
}
}
if (myFlag) {
std::cout << "Feature is enabled!" << std::endl;
} else {
std::cout << "Feature is disabled." << std::endl;
}
return 0;
}
What is a C++ Flag?
Definition of a Flag
In programming, a flag is a variable or value that is used to signify a specific condition or state within a program. Flags are quintessential in controlling the flow and behavior of applications. In the context of C++, flags can be categorized mainly into two types: compiler flags and command-line flags.
Types of Flags in C++
Flags can take various forms in C++. Broadly speaking, they can be identified as:
- Compiler Flags: These are options provided to the compiler that control the compilation process. They can optimize code, enable warnings, or affect debugging information.
- Command-Line Flags: These flags change how an application operates when it is executed. They allow users to pass commands directly into the application, tweaking its behavior without altering the codebase.
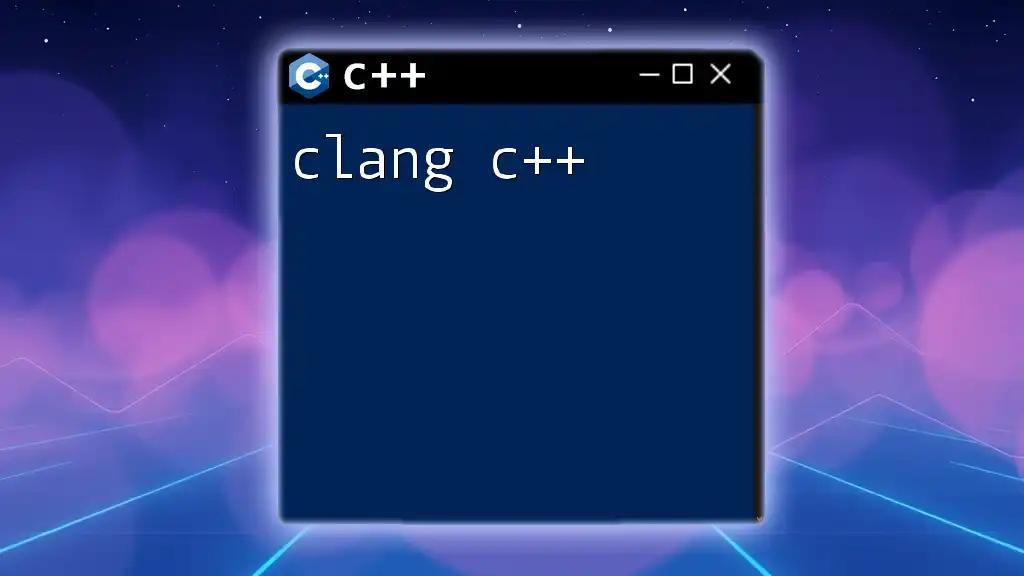
Compiler Flags in C++
Overview of Compiler Flags
Compiler flags play a pivotal role in how C++ code is compiled and structured. By utilizing these flags, developers can enhance the performance of their code, set specific behaviors during compilation, and improve overall code quality.
Commonly Used Compiler Flags
Optimization Flags
Optimization flags are essential for refining the performance of C++ applications. They instruct the compiler on how to improve code efficiency and execution speed.
- Flag: `-O` (optimization level)
For instance, when running the following command:
g++ -O2 main.cpp -o main
This command compiles `main.cpp` with level 2 optimization, balancing performance and compilation time. Understand that different levels exist:
- `-O1` for minimal optimization.
- `-O2` for more robust optimizations while still maintaining reasonable compilation times.
- `-O3` for aggressive optimizations that may increase compile time and size without guaranteeing a corresponding increase in runtime performance.
Warning Flags
Warnings during compilation are crucial in spotting potential issues early on. These flags inform developers about questionable code practices that could lead to errors or bugs.
- Flag: `-Wall` (enable all warnings)
To compile with warnings, use:
g++ -Wall main.cpp -o main
This command sets all pertinent compiler warnings into motion, encouraging developers to pay attention to subtle issues that might evade immediate detection.
Debugging Flags
Debugging flags are invaluable when troubleshooting code. They allow developers to run their applications step-by-step, making it easier to pinpoint issues.
- Flag: `-g` (generate debug information)
For debugging, use:
g++ -g main.cpp -o main
This command generates debug information in the compiled file, making it easier to utilize debugging tools such as gdb.

Command-Line Flags in C++
Overview of Command-Line Flags
Command-line flags provide users the flexibility to modify application behavior at runtime. By implementing these flags, developers empower the end-user to customize the functionality without diving into the source code.
Implementing Command-Line Flags in C++
To effectively use command-line flags in C++, you will work primarily with the `argc` and `argv` parameters of the `main` function.
Using `argc` and `argv`
- argc: Refers to the count of command-line arguments, including the program name.
- argv: An array of C-strings (character arrays) that contains the command-line arguments.
Example Implementation
Here's a simple implementation that prints out all provided command-line arguments:
#include <iostream>
int main(int argc, char* argv[]) {
for (int i = 0; i < argc; ++i) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
In the above example, when the program is executed with arguments, it will enumerate each command-line input, helping you understand how arguments are received.
Parsing Flags
For more intricate flag parsing, libraries such as `getopt` can simplify the process, allowing for easier command-line argument handling.
Example with `getopt`
The following example demonstrates how to use `getopt` to manage command-line flags:
#include <iostream>
#include <unistd.h>
int main(int argc, char* argv[]) {
int opt;
while ((opt = getopt(argc, argv, "ab:")) != -1) {
switch (opt) {
case 'a':
std::cout << "Option A selected\n";
break;
case 'b':
std::cout << "Option B with value: " << optarg << std::endl;
break;
default:
std::cerr << "Usage: " << argv[0] << " [-a] [-b value]\n";
return EXIT_FAILURE;
}
}
return EXIT_SUCCESS;
}
In this code, we parse two options: `-a` and `-b`. The flag `-b` requires an additional argument, indicated by the colon `:`. When executed, the program assesses the flags and prints corresponding messages, illustrating how flags can dynamically alter output.

Best Practices for Using Flags in C++
General Guidelines
To ensure that flags integrate seamlessly into your C++ projects, follow these general guidelines:
- Keep flags intuitive and easily understandable to facilitate user experience.
- Offer clear documentation specifying flag functionalities and expected behaviors.
Debugging Flags
Utilizing debugging flags during the development phase is critical. They enable developers to identify the root cause of issues promptly, ensuring code quality.
Performance Considerations
When employing optimization flags, be mindful of the associated trade-offs. While optimization can significantly boost performance, it can also increase compile time and generate larger binary files. Always evaluate the implications of each optimization flag on your project.
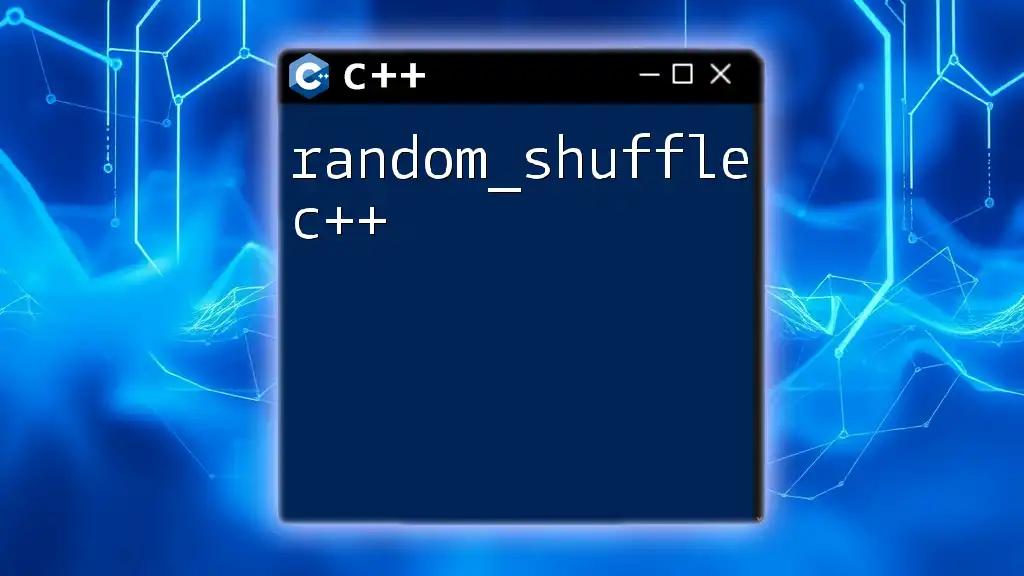
Conclusion
Understanding and effectively using C++ flags empower developers and enhance overall programming proficiency. By mastering both compiler and command-line flags, you unlock the ability to control and optimize both the compilation and execution of your C++ applications. Explore, experiment, and embrace the power these flags offer in your programming journey!

Additional Resources
Consider delving into further educational materials, such as books and online tutorials, to deepen your understanding of C++ programming and the strategic implementation of flags.
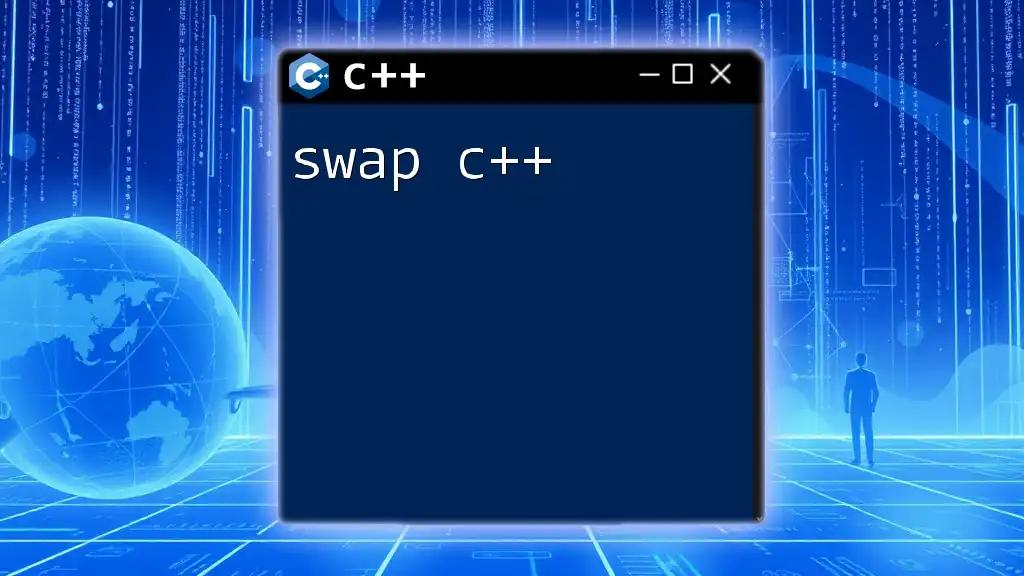
Call to Action
Now that you're equipped with knowledge on how to use flags in your C++ applications, I encourage you to experiment with these concepts in your projects. Share your experiences and insights on how you’ve leveraged flags to enhance your programs!