The `tellg` function in C++ is used to obtain the current position of the get pointer in a file stream, allowing you to track how many bytes have been read from the beginning of the file.
Here’s a simple code snippet demonstrating its usage:
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt");
if (file.is_open()) {
char ch;
while (file.get(ch)) {
// Read the file character by character
}
std::streampos pos = file.tellg(); // Get the current position
std::cout << "Current position: " << pos << std::endl;
file.close();
}
return 0;
}
What is tellg?
The `tellg` function in C++ is used to determine the current position of the file pointer, also known as the "get" pointer, in a file stream. It provides a convenient way to ascertain where we are in the file, which is particularly useful in file handling and manipulation.
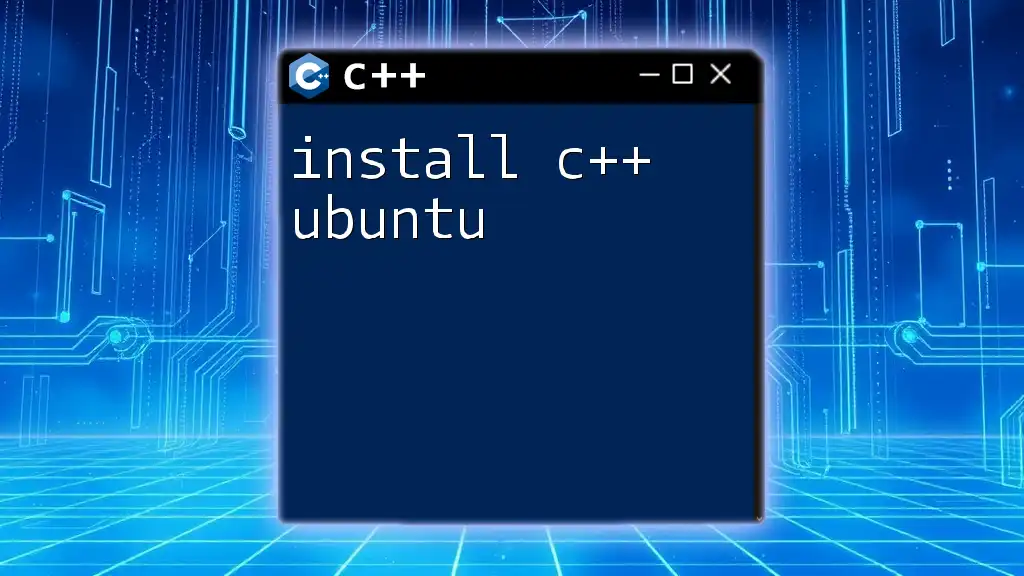
Why is tellg important in C++?
Knowing the position of the file pointer is crucial for several reasons:
- File Navigation: It allows programmers to navigate through a file effectively, understanding the precise location from where data can be read or where new data can be written.
- Debugging: Understanding where the pointer is can help identify issues in file handling or processing logic.
- File Size Calculation: `tellg` can be employed to determine the size of the file by moving the pointer to the end and obtaining its position.
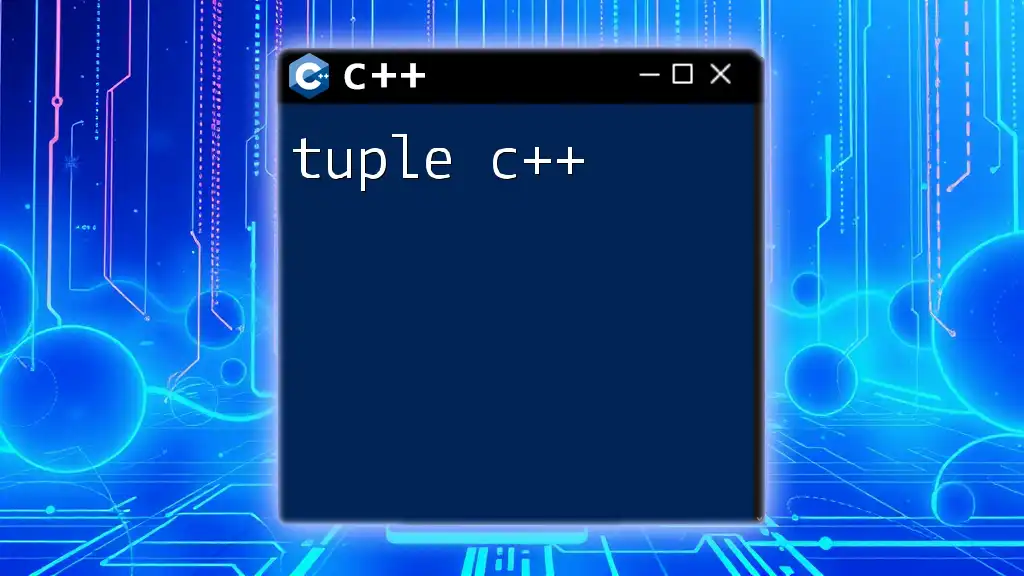
Understanding File Streams in C++
What are file streams?
File streams in C++ are abstractions that allow data to be read from or written to files in a structured manner. There are primarily two types of streams that handle file I/O:
- Input Stream (`istream`): Used for reading data from files.
- Output Stream (`ostream`): Used for writing data to files.
Creating and opening file streams
To utilize file streams, you first need to declare and open them. The basic syntax for file stream creation in C++ is as follows:
#include <fstream>
using namespace std;
ifstream inputFile("example.txt");
ofstream outputFile("output.txt");
In this example, `ifstream` is used for opening a file in read mode, while `ofstream` is for write mode.
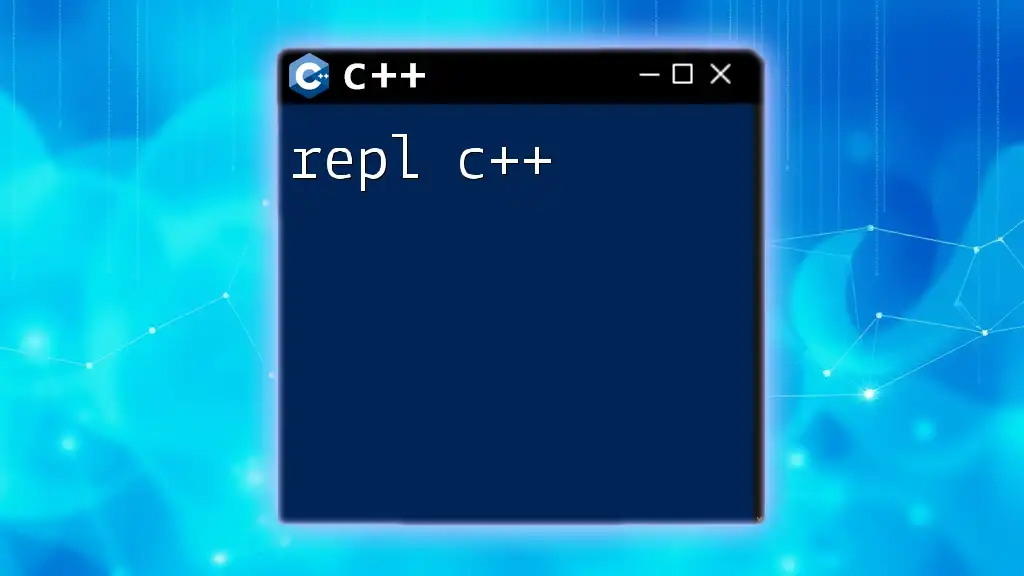
The tellg Function Explained
Syntax of tellg
The syntax for using `tellg` is straightforward:
streampos tellg();
- Return Type: The function returns a value of type `streampos`, which represents the position in the file stream.
- Parameters: `tellg` does not take any parameters.
How tellg works
When invoked on an input stream, `tellg` returns the current position of the "get" pointer. If the file is successfully opened and positioned, it will return the number of bytes from the beginning of the file. If an error occurs or the stream is not open, it may return `-1`, indicating the failure.
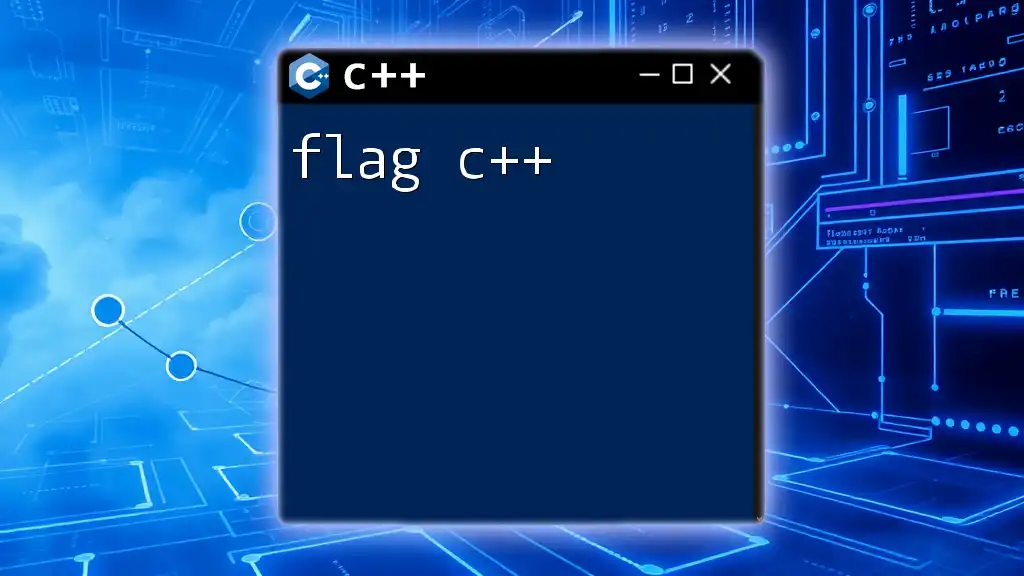
Practical Applications of tellg
Using tellg to determine the current position
A common use of `tellg` is to check the current position of the file pointer. This can be helpful for debugging or logging purposes. Here’s a simple example:
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ifstream inputFile("example.txt");
if (!inputFile) {
cerr << "File could not be opened!" << endl;
return 1;
}
streampos pos = inputFile.tellg();
cout << "Current position: " << pos << endl;
inputFile.close();
return 0;
}
This code snippet checks the position in an opened file and outputs it to the console.
Combining tellg with seekg
The `tellg` function can be paired with `seekg` to manipulate the file pointer. Using `seekg`, you can move the pointer to a specific location and subsequently check that position using `tellg`. Here's an example:
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ifstream inputFile("example.txt");
if (!inputFile) {
cerr << "File could not be opened!" << endl;
return 1;
}
inputFile.seekg(0, ios::end);
streampos endPos = inputFile.tellg();
cout << "End position: " << endPos << endl;
inputFile.close();
return 0;
}
In this snippet, the pointer is moved to the end of the file, and `tellg` is used to retrieve its position, indicating the total size of the file.
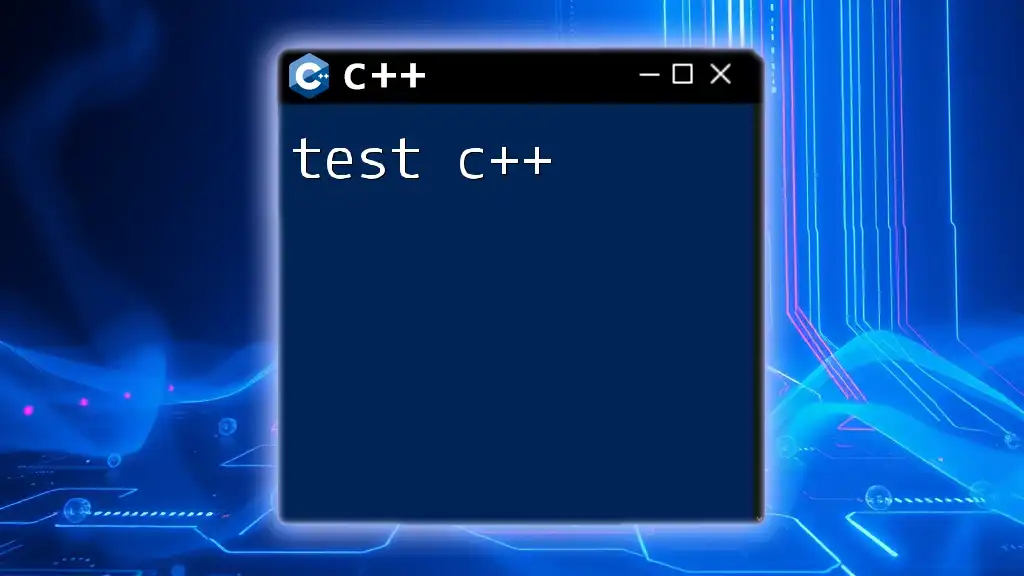
Common Use Cases for tellg
Reading and writing binary files
In binary file handling, the `tellg` function plays a pivotal role in understanding the structure and size of the data being processed. Here’s a sample code demonstrating its application in a binary file context:
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ifstream binaryFile("data.bin", ios::binary);
if (!binaryFile) {
cerr << "Binary file could not be opened!" << endl;
return 1;
}
binaryFile.seekg(0, ios::end);
cout << "Binary file size: " << binaryFile.tellg() << " bytes" << endl;
binaryFile.close();
return 0;
}
This code opens a binary file, moves the pointer to the end, and outputs the file size, which is critical when dealing with fixed-length records.
Processing text files
Another effective use of `tellg` is in processing text files, where you may need to know the position for parsing or extraction of data. Below is an example where we read each line of a text file and track the position:
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
ifstream inputFile("example.txt");
if (!inputFile) {
cerr << "File could not be opened!" << endl;
return 1;
}
string line;
while (getline(inputFile, line)) {
streampos currentPos = inputFile.tellg();
// Process line...
cout << "Current line position: " << currentPos << endl;
}
inputFile.close();
return 0;
}
In this example, we iterate through each line while capturing the position, which can be useful for tasks like tracking where specific data points are located.
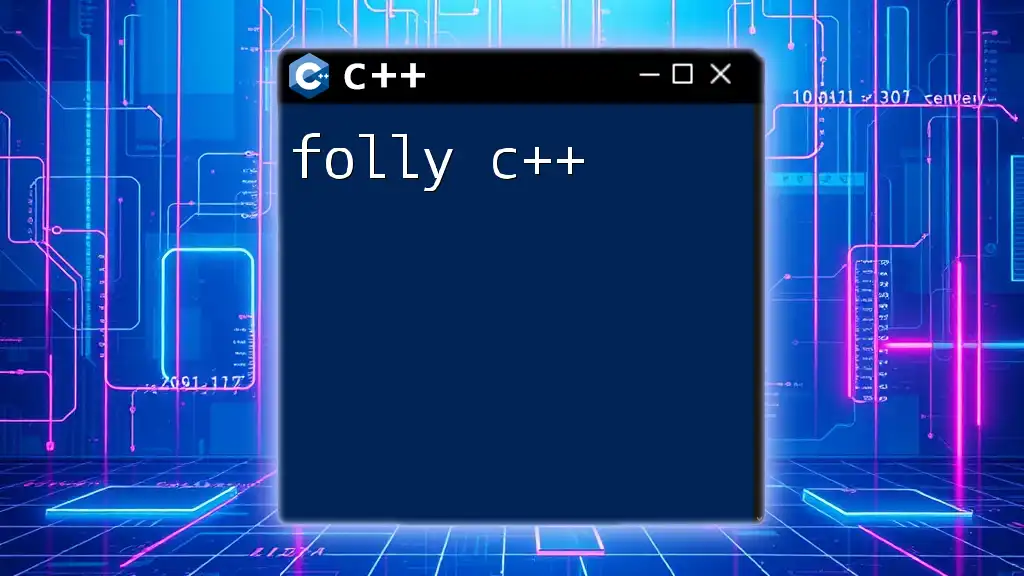
Limitations of tellg
While `tellg` is a powerful function, there are some limitations to be aware of:
- Not Always Accurate: If the stream has encountered any errors or if it is not open, `tellg` may return unexpected results, such as `-1`.
- Seekability: Not all streams are seekable, particularly when dealing with certain types of external inputs or special file formats. Using `tellg` on non-seekable streams can lead to inaccurate or undefined behavior.
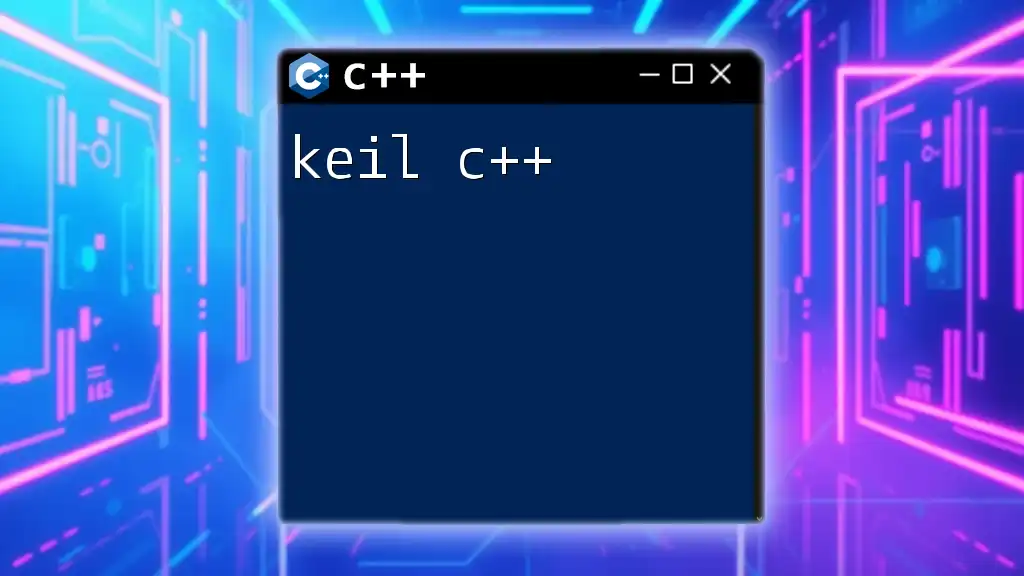
Conclusion
In summary, the `tellg` function in C++ is essential for file handling as it provides the current position of the file pointer within a stream. Understanding its utilization alongside file streams can enhance your ability to manage data effectively within your applications. Mastery of `tellg` and its companion functions allows for precise control in reading and writing files, making it a crucial element of robust file I/O practices.
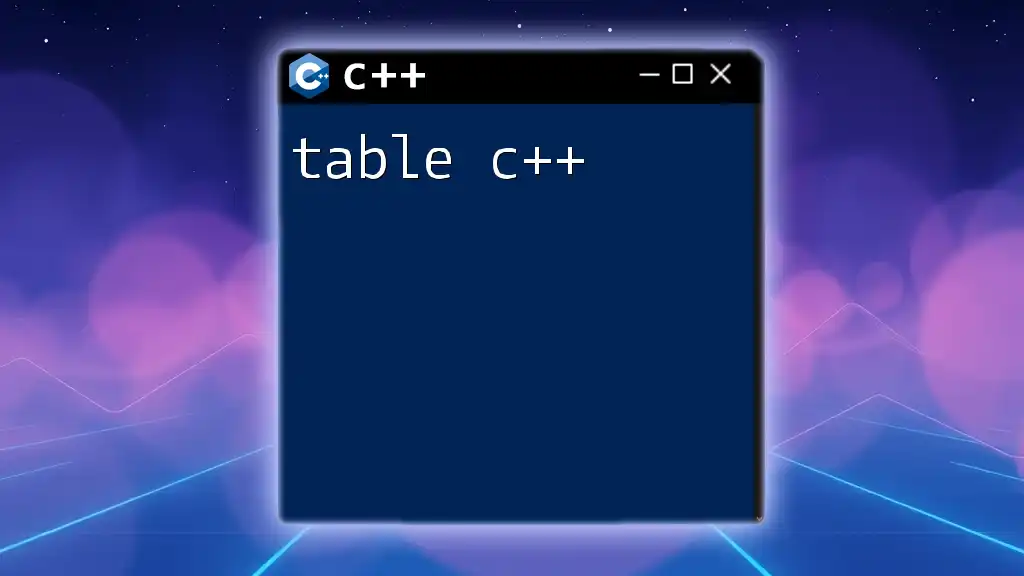
Additional Resources
To further enhance your knowledge of file I/O in C++, consider exploring recommended books, articles, and online tutorials available within the C++ community. Engaging with forums can also provide you with valuable insights and support as you continue your learning journey in mastering file handling in C++.