Keil C++ is a development environment tailored for C++ programming in embedded systems, allowing developers to efficiently write, compile, and debug their code for various microcontrollers.
Here’s a simple example that demonstrates how to define and use a class in Keil C++:
class LED {
public:
void turnOn() {
// Code to turn on the LED
}
void turnOff() {
// Code to turn off the LED
}
};
int main() {
LED myLED;
myLED.turnOn();
return 0;
}
What is Keil C++?
Understanding Keil
Keil is recognized as a major player in the development of embedded systems, providing a powerful integrated development environment (IDE) designed specifically for microcontroller developers. With a rich history dating back to the early 1980s, Keil has built a solid reputation for reliability and efficiency.
The Keil IDE offers a suite of features tailored for embedded programming, enabling developers to design, debug, and optimize their applications seamlessly. It supports a variety of compilers, but its focus on C and C++ brings it to the forefront of embedded system programming.
The Role of C++ in Embedded Systems
Selecting the appropriate programming language is critical in embedded systems. Many developers prefer C++ over C due to its support for Object-Oriented Programming (OOP). This paradigm enhances code organization and allows for concepts such as:
- Encapsulation: Grouping data and methods that operate on that data within a single unit (class).
- Inheritance: Facilitating code reuse by creating new classes based on existing ones.
- Polymorphism: Allowing methods to perform differently based on the object that is calling them.
These features provide significant advantages such as improved code readability, maintainability, and flexibility, making C++ a compelling choice for many developers in the embedded domain.

Setting Up Keil C++
System Requirements
Before installing Keil C++, it’s important to verify that your system meets the required specifications. The following is a list of needed hardware and software components:
- Minimum Hardware Requirements:
- Intel or AMD processor with at least 1.0 GHz clock speed
- 2 GB RAM (4 GB recommended)
- 1 GB of available disk space
- Screen resolution of 1024x768 pixels or higher
- Software Requirements:
- Windows 10 or later operating system
- .NET Framework version 4.5 or later
Installation Instructions
Once you have ensured your system meets the requirements, follow these steps to install Keil C++:
-
Downloading the Installer: Visit the official Keil website to download the latest installer. Ensure you are selecting the appropriate version for your target microcontroller.
-
Installation Process Breakdown:
- Run the downloaded installer.
- Follow the prompts to complete the installation.
- Select the components you wish to install; by default, all options are typically offered.
-
Activating the Software: After installation, you may need to activate Keil C++ using a license key. Follow the license activation process as directed by the installer.
Configuring the IDE for C++
Once Keil C++ is installed, the next step is configuring the IDE for optimal performance:
-
Main Interface Overview: Familiarize yourself with the Keil IDE layout, including the toolbar, code editor, and debugging windows. Understanding the navigation will streamline your coding sessions.
-
Setting Up Projects: Keil structures its projects in a specific way, so it’s essential to grasp the layout. You will typically create a workspace for your project comprising all related files, source codes, and libraries.
-
Toolchain Configuration: Ensure the toolchain is correctly set for C++. Navigate to the options menu to specify which compiler and linker options you wish to employ for C++ development.

Writing Your First C++ Program in Keil
Project Creation
Creating a new C++ project involves several straightforward steps:
- How to Create a New C++ Project: Use the project wizard feature in Keil to start a new project. Specify the target microcontroller you intend to work with, as this will greatly affect the settings and libraries available to you.
Coding in C++
Once you have set up your project structure, it’s time to write your first C++ program. The following example illustrates the basic structure of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation of the Code Structure and Compilation Process
- `#include <iostream>`: This line includes the standard input-output stream library, required for performing input and output operations.
- `int main() {...}`: This function defines the entry point of the program. Every C++ program must have a `main` function.
- `std::cout << "Hello, World!" << std::endl;`: Here, we are using `std::cout` to print "Hello, World!" to the console, followed by `std::endl` for a new line.
- `return 0;` indicates successful termination of the program.
Compiling and Running Your Program
To compile your C++ code in Keil:
- Locate the compile button in the toolbar and click it to start the compilation process.
- If there are any syntax errors or issues, they will be displayed in the output window. Make sure to resolve these before proceeding.
Once you have successfully compiled your code, you can run your program:
- Use the debugger mode to step through your code and inspect variable values.
- For initial tests, utilize the run button to see output directly.
Advanced Features of Keil C++
Object-Oriented Programming (OOP) in C++
As you advance in your C++ journey, understanding OOP concepts is crucial. Here is an example that encapsulates how to create a simple class:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
In this example:
- `class Dog {...}` defines a new class called `Dog`.
- `void bark() {...}` is a method that belongs to the `Dog` class.
- An instance of `Dog`, `myDog`, is created in the main function, allowing `myDog.bark();` to be invoked.
The use of classes enables efficient management of related data and functions, particularly beneficial in large embedded systems projects.
Utilizing Keil’s Middleware
Keil offers various middleware libraries designed to streamline development. Middleware can include real-time operating systems (RTOS), communication protocols, and file systems. Here, we’ll explore:
- Overview of Middleware Libraries: These libraries streamline processes by providing reusable components that save development time.
- Example: Using RTOS in an Embedded Project: Implementing an RTOS allows for multitasking capabilities, improving the efficiency of time-sensitive applications.
- Integrating Middleware into Your Project Settings: Access the project settings to link necessary middleware libraries, enhancing your project’s functionality and capabilities.

Debugging and Optimization in Keil C++
Debugging Tools in Keil
Keil provides a robust set of debugging tools. Key features include:
- Breakpoints: Set breakpoints to pause program execution at specific lines. This allows for examination of variable states at critical moments.
- Watch Windows: Utilize watch windows to monitor variable values while the program runs, providing insight into how data is manipulated.
- Call Stacks: View call stacks to trace function calls and pinpoint where an issue may have arisen in complex applications.
Optimization Techniques
Code optimization is vital for enhancing performance in embedded systems. Consider the following tips:
- Avoid using excessive global variables, as they can lead to increased memory usage.
- Implement efficient data structures to minimize processing time.
- Analyzing your program’s execution with profiling tools can provide insights into performance bottlenecks.

Common Challenges and Solutions
Performance Issues
During development, you may encounter performance bottlenecks, such as slow execution speed due to inefficient algorithms. To address these issues:
- Identify time-consuming sections of your code using profiling tools.
- Consider simpler algorithms or logic that produce the same results more efficiently.
Code Portability and Compatibility
To ensure that your C++ code is portable across different platforms, follow a set of best practices:
- Adhere to standard C++ conventions to enhance compatibility.
- Avoid platform-specific libraries unless absolutely necessary, as this can hamper portability.
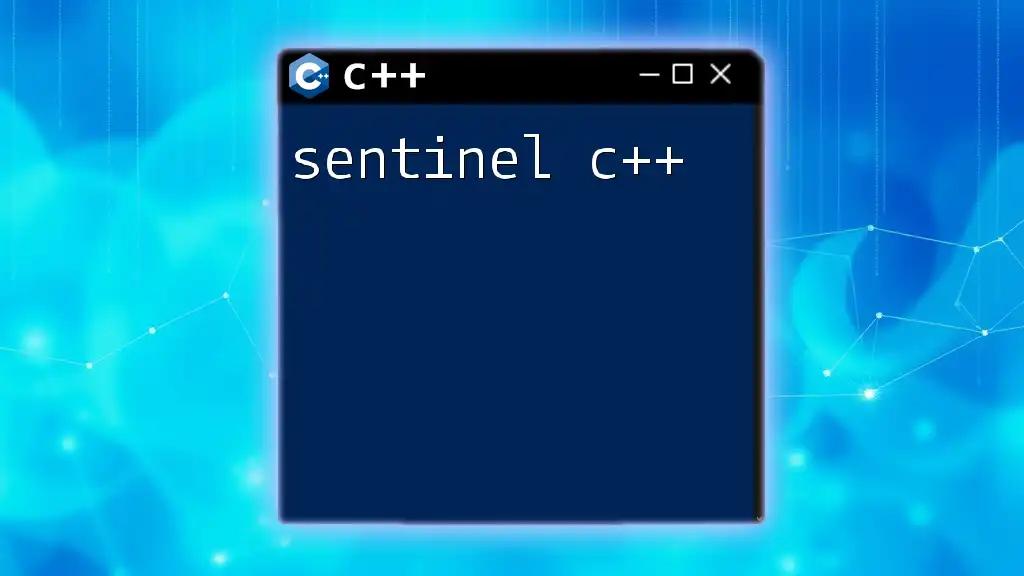
Real-World Applications
Case Studies
C++ is used to develop a wide range of embedded applications. For instance:
- Industrial Automation: Companies often utilize C++ programming in the development of control systems, enabling efficient operation and data exchange among various sensors and actuators.
- Consumer Electronics: Devices like smart appliances or wearables employ embedded C++ to manage complex user interfaces while optimizing battery life and performance.
Discussion of Custom Embedded C++ Projects Developed Using Keil
Developing a custom project with Keil C++ could involve creating an intelligent home automation system. Such a project showcases the integration of various sensors, communication protocols, and user interfaces, highlighting the expansive capabilities of the Keil environment.
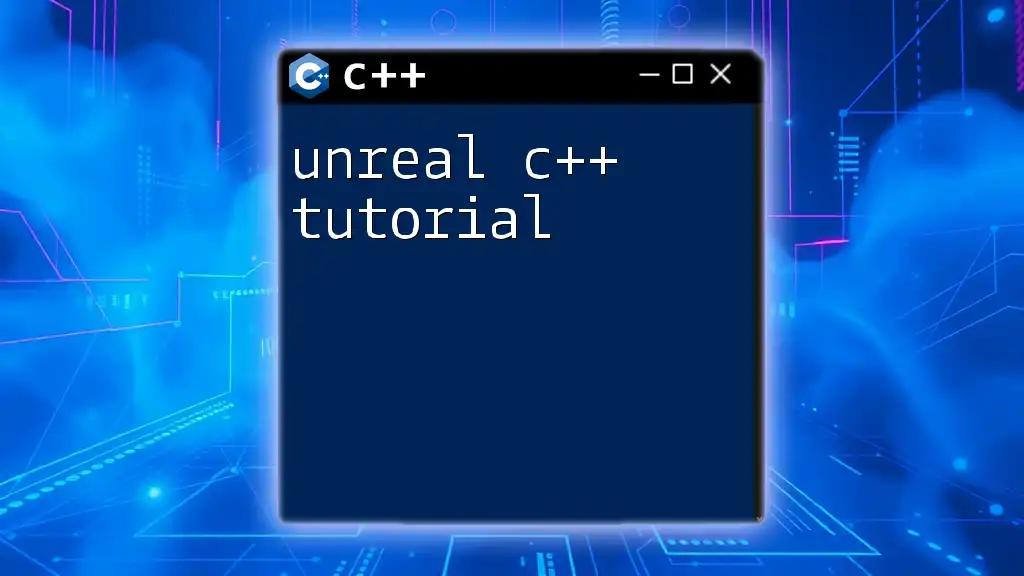
Conclusion
Keil C++ stands at the forefront of embedded systems development, offering advanced tools that leverage the benefits of C++ programming. The IDE’s comprehensive features foster innovation while simplifying the development process. By mastering Keil C++, you equip yourself with the skills needed to tackle a wide array of challenges in the embedded technology domain.
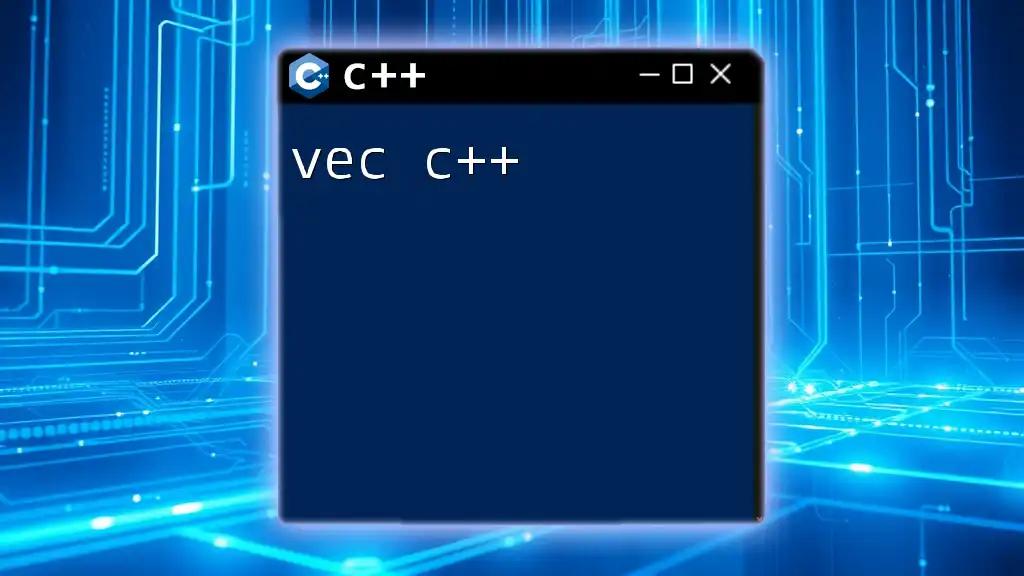
Additional Resources
To further enhance your knowledge of Keil C++, consider exploring recommended books, tutorials, and online courses tailored to embedded programming. Engaging with the Keil community and participating in forums can also provide valuable support and networking opportunities.

FAQs
- What microcontrollers are compatible with Keil C++?
- How can I troubleshoot compilation errors in Keil?
- Can I use Keil C++ for real-time applications?
These resources and support avenues can help you navigate your journey in learning Keil C++ effectively.