The `typeid` operator in C++ is used to retrieve type information at runtime, enabling you to check the type of an object or variable.
#include <iostream>
#include <typeinfo>
int main() {
int num = 5;
std::cout << "The type of num is: " << typeid(num).name() << std::endl;
return 0;
}
What is typeid in C++?
The `typeid` operator in C++ is a powerful tool for type identification within a strongly-typed language. It plays a significant role in maintaining type safety, allowing developers to query the type of expressions at runtime. Understanding `typeid` is essential as it relates closely to the concepts of Run-Time Type Information (RTTI), which is crucial for implementing polymorphism effectively.
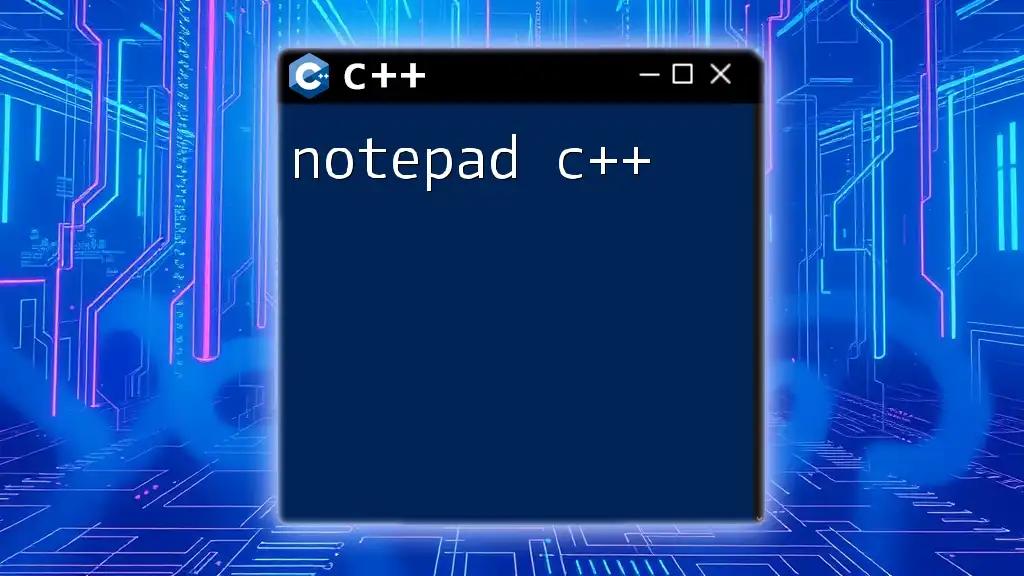
Syntax of typeid
Basic Syntax
The syntax for using `typeid` is straightforward. It can be used to obtain the type information of a given expression. Simply put, you would use it in the format:
typeid(expression)
Example of using typeid with built-in types:
#include <iostream>
#include <typeinfo>
int main() {
int a;
std::cout << "Type of a: " << typeid(a).name() << std::endl;
return 0;
}
In this example, the `typeid(a)` expression retrieves the type information of the variable `a`, which is an integer.
Using typeid with Classes and Structs
In addition to built-in types, `typeid` can also be utilized with user-defined types such as classes and structs. This makes it especially useful for polymorphic hierarchies where understanding the actual object type at runtime is essential.
Code example showcasing typeid with user-defined types:
#include <iostream>
#include <typeinfo>
class Sample {
public:
virtual void display() {}
};
int main() {
Sample obj;
std::cout << "Type of obj: " << typeid(obj).name() << std::endl;
return 0;
}
In this case, `typeid(obj)` outputs the type information of the `Sample` object.

Understanding the Output of typeid
What Does typeid Return?
When you use `typeid`, it returns a reference to a `std::type_info` object that contains information about the requested type. This object provides various member functions to retrieve details like the name of the type.
Common functions include:
- `name()`: Returns the name of the type as a string.
Examples of typeid Output
It’s important to remember that the output of `typeid` can vary based on the type being queried.
Comparing output for different types:
#include <iostream>
#include <typeinfo>
int main() {
double d;
int i;
std::cout << "Type of d: " << typeid(d).name() << std::endl;
std::cout << "Type of i: " << typeid(i).name() << std::endl;
return 0;
}
The example above shows how `typeid` effectively communicates the type information of variable `d` (double) and variable `i` (int), helping developers verify the expected types.

Using typeid with Pointers and References
Pointers and Polymorphism
When dealing with polymorphism, `typeid` becomes crucial for identifying the actual type of objects pointed to by base class pointers. The correct type can be determined only when the object is dereferenced.
Code example demonstrating polymorphism and typeid:
#include <iostream>
#include <typeinfo>
class Base {
public:
virtual void func() {}
};
class Derived : public Base {
public:
void func() override {}
};
int main() {
Base* b = new Derived();
std::cout << "Type of b: " << typeid(*b).name() << std::endl; // Dereferencing for correct type
delete b;
return 0;
}
In this example, dereferencing the pointer `b` using `*b` allows `typeid` to return the actual type of the object it points to, which is `Derived`.
References and typeid
Using `typeid` with references behaves similarly to pointers but directly references the typed object. Given the strong typing, this yields the actual type of the referenced object.
Code example showcasing typeid with references:
#include <iostream>
#include <typeinfo>
class Base {
public:
virtual void func() {}
};
class Derived : public Base {
public:
void func() override {}
};
int main() {
Derived d;
Base& b = d;
std::cout << "Type of b: " << typeid(b).name() << std::endl; // Right type due to reference
return 0;
}
Here, `typeid(b)` outputs the type of `Derived` because `b` is a reference to an object of that type.

Typeid with Templates
Understanding Type Identification in Templates
In C++, templates allow for type-safe programming with generic types. When `typeid` is used within template functions, it can help identify the template type deduced by the compiler.
Code examples to illustrate:
#include <iostream>
#include <typeinfo>
template<typename T>
void showType(T t) {
std::cout << "Type of T: " << typeid(T).name() << std::endl;
}
int main() {
showType(5);
showType(3.14);
return 0;
}
In this example, the output displays the type of `T` based on its instantiation in each call, helping developers understand how templates deduce types under different circumstances.

Limitations and Considerations of typeid
Limitations of typeid
While powerful, `typeid` has its limitations. For example, if you try to use `typeid` on a non-polymorphic type, it only returns the static type of the pointer/reference.
Considerations for Using typeid in C++
It's crucial to be aware of performance implications when using RTTI through `typeid`. Frequent usage in performance-critical sections may lead to overhead. It is also advisable to avoid overreliance on `typeid` for type checking, as polymorphic design often can be achieved through better patterns like visitor classes or type-safe unions.
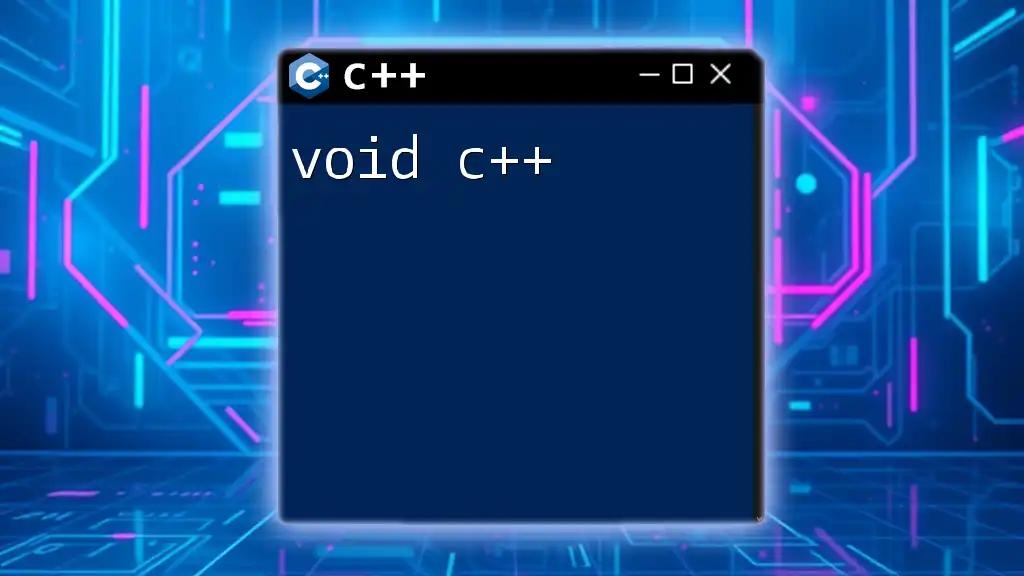
Conclusion
In conclusion, the `typeid` operator in C++ offers invaluable functionality for understanding and interacting with types at runtime. By harnessing `typeid`, developers can implement robust and type-safe designs while effectively utilizing polymorphism.

Additional Resources
For further exploration of the `typeid` operator, consider reading through C++ documentation or exploring resources on Run-Time Type Information (RTTI) for a deeper understanding of type identification in C++. Such resources can help solidify knowledge and application of `typeid` in real-world programming scenarios.