In C++, the `long` type is used to declare integer variables that can hold larger values than the standard `int` type can accommodate, typically 4 bytes or more depending on the system architecture.
Here's a code snippet demonstrating the use of the `long` type in C++:
#include <iostream>
int main() {
long bigNumber = 1234567890;
std::cout << "The long number is: " << bigNumber << std::endl;
return 0;
}
Understanding Data Types in C++
In programming, data types serve as a crucial foundational element, defining the types of values a variable can hold. C++ offers various data types that can be classified primarily into primitive and user-defined types.
What is a Data Type?
A data type defines a collection of values and the operations that can be performed on those values. In C++, choosing the right data type is vital as it influences the performance and memory usage of your application. Among the common primitive data types in C++ are `int`, `float`, `double`, and `char`.
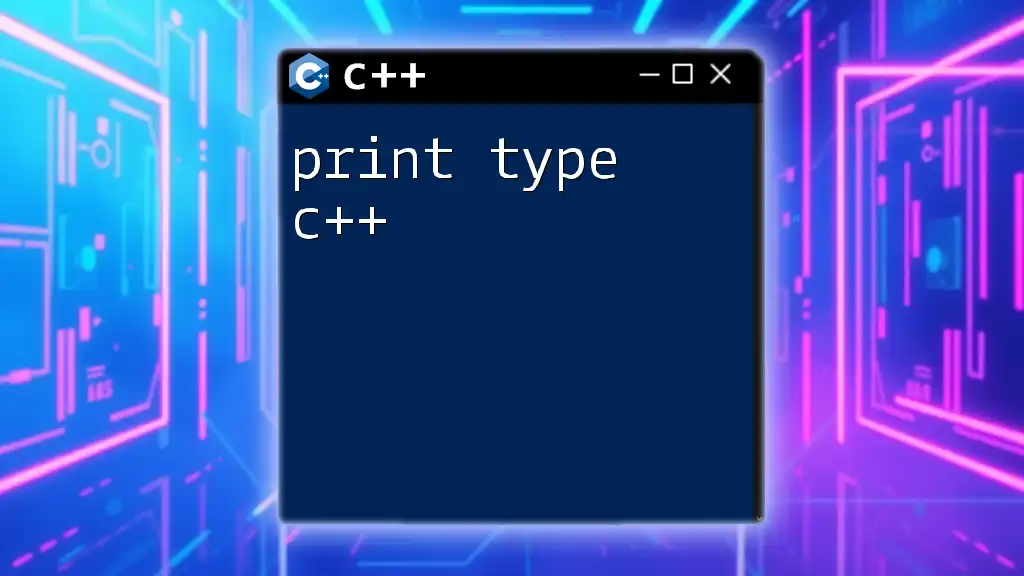
The Long Data Type in C++
The long data type plays a significant role in scenarios requiring storage of larger integer values. It is particularly advantageous when the expected range of data exceeds what an `int` can accommodate.
Characteristics of Long in C++
The size of the `long` data type is platform-dependent, typically taking up 4 bytes on 32-bit systems and 8 bytes on 64-bit systems. This difference has significant implications when you're dealing with large numbers.
- Maximum Values: On 32-bit systems, `long` can typically represent numbers up to 2,147,483,647, while 64-bit systems extend this limit to 9,223,372,036,854,775,807.
- Minimum Values: Correspondingly, the minimum values are -2,147,483,648 and -9,223,372,036,854,775,808 for 32-bit and 64-bit systems, respectively.
Syntax of Long in C++
Declaring a long variable is straightforward. The basic syntax is as follows:
long myLongVariable;
This simple declaration allows you to initialize and use long integers throughout your program.
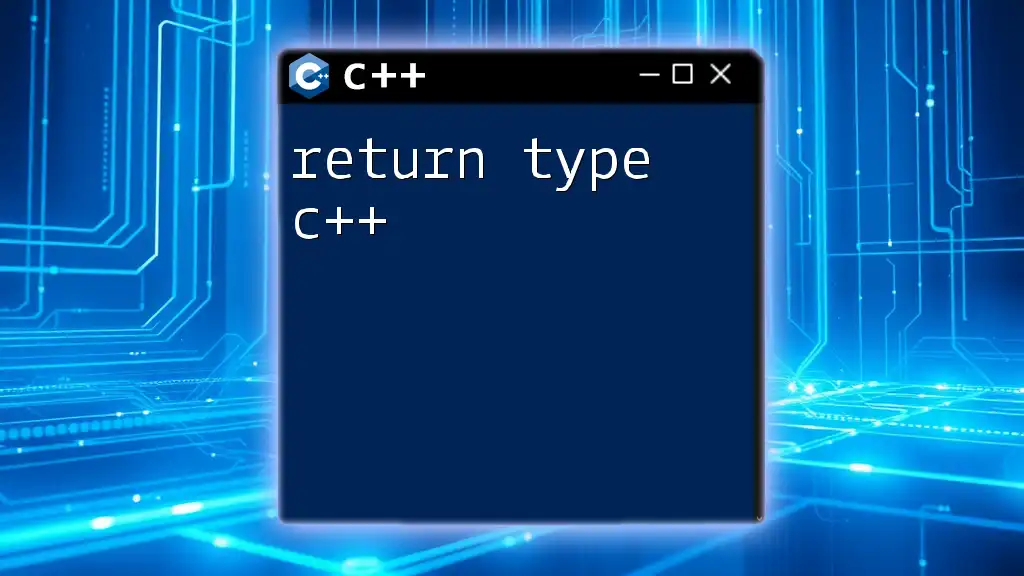
When to Use Long in C++
The `long` data type is especially useful in various programming situations. It's the preferred choice when you need to handle larger integers than what an `int` can accommodate.
Examples of Use Cases
Here’s a practical scenario where you might need to use the `long` data type:
When dealing with unique identifiers or counters, such as user IDs in a large application, you may often encounter values that exceed the limits of regular integers:
long userID = 9223372036854775807; // Maximum value for long on 64-bit systems
Utilizing `long` ensures that you can store these values safely without overflow errors.
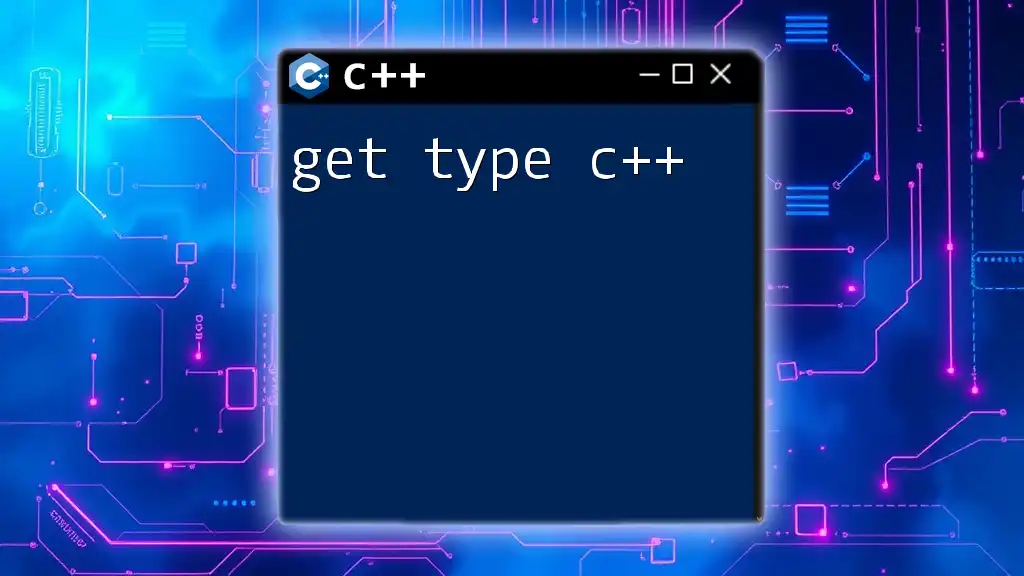
Operations with Long in C++
The `long` data type supports standard arithmetic operations, allowing you to perform calculations just like with other integer types.
Basic Arithmetic Operations
You can add, subtract, multiply, and divide long integers efficiently. Here’s an example illustrating these operations:
long a = 1000000;
long b = 2500000;
long sum = a + b; // 3500000
long difference = b - a; // 1500000
long product = a * b; // 2500000000000
long quotient = b / a; // 2
These operations reveal the intrinsic capacity of `long` to handle large values without compromising performance.
Type Conversion
When mixing data types in your calculations, it's essential to understand type conversion. C++ allows both implicit and explicit conversions. For example, if you divide a `long` by an `int`, C++ automatically promotes the `int` to a `long`.
Consider this example:
long longNumber = 1000;
int intNumber = 3;
long result = longNumber / intNumber; // result is 333. The decimal part is discarded.
The above example demonstrates how C++ handles mixed data types, making it crucial to manage data gracefully to avoid unexpected outcomes.
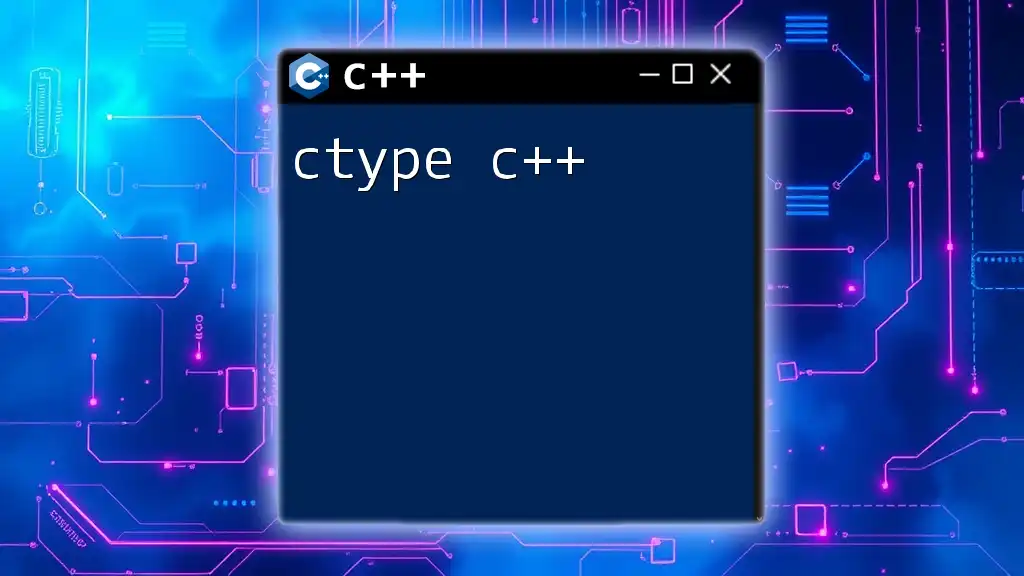
Comparing Long with Other Data Types
When programming, you often need to decide whether to use `long`, `int`, or `long long`. Understanding the distinctions between these types can inform your decision-making.
Performance Considerations
Using `long` offers some performance advantages, especially when you require handling larger values. Generally, you should opt for `long` when your application necessitates storage for large integers. However, using `long` where it’s unnecessary could lead to inefficient memory utilization, so always analyze the data requirements of your application.
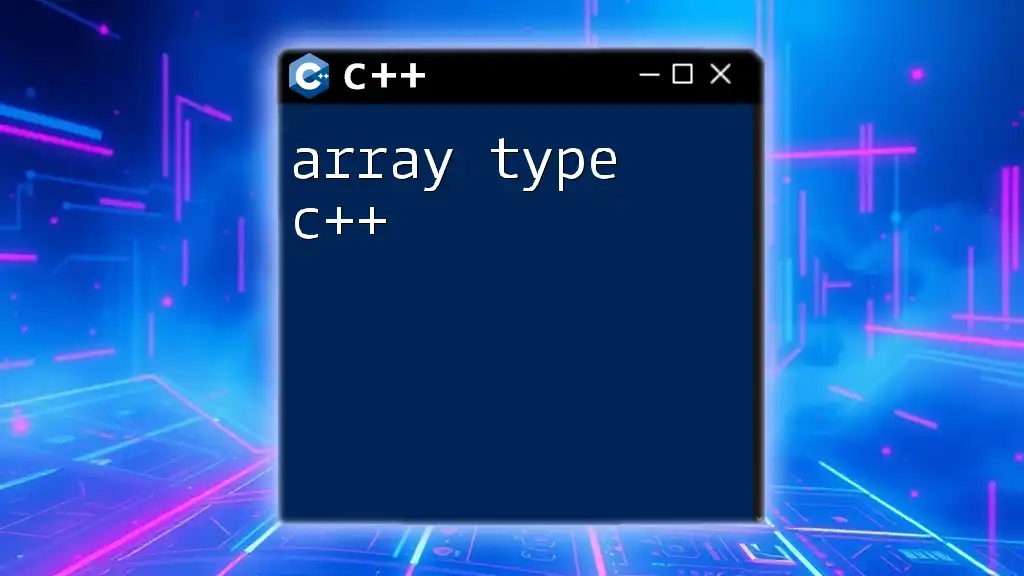
Best Practices
To make the most out of the `long` data type, consider the following best practices:
- Only use `long` when necessary: Reserve `long` for cases where `int` cannot handle your data range.
- Comment your code: When using `long`, especially in complex calculations, clear comments can provide context on why you chose `long` over other data types.
- Robustness: Ensure you handle potential overflows gracefully; using `long` does not eliminate the risk of overflow, especially in arithmetic operations.
Code Examples
Here's a well-commented code snippet to illustrate best practices with `long`:
#include <iostream>
using namespace std;
int main() {
long population = 7837000000; // World population estimate
cout << "Current world population: " << population << endl; // Output the population
long growth = 12000000; // Estimate annual growth
population += growth; // Update population for next year
cout << "Estimated next year's population: " << population << endl;
return 0; // End of program
}
This example efficiently utilizes the `long` type while maintaining clarity through comments.
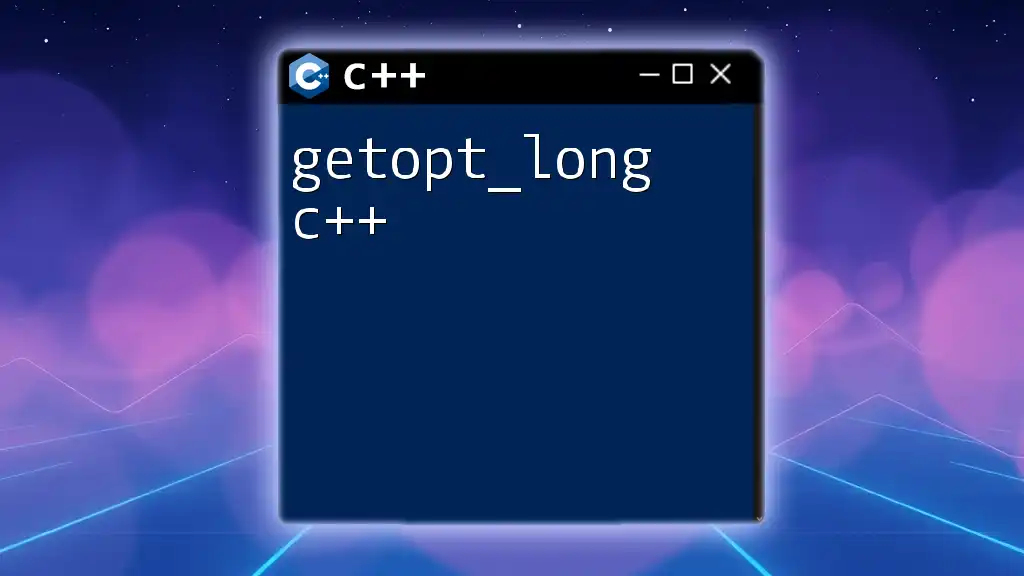
Conclusion
The long type in C++ is an essential tool for programmers aiming for precision and capability with larger integer values. Understanding its attributes, benefits, and proper usage will significantly enhance your programming efficacy. As you experiment with this data type in various scenarios, you will find it a reliable choice for handling extensive datasets and calculations seamlessly.
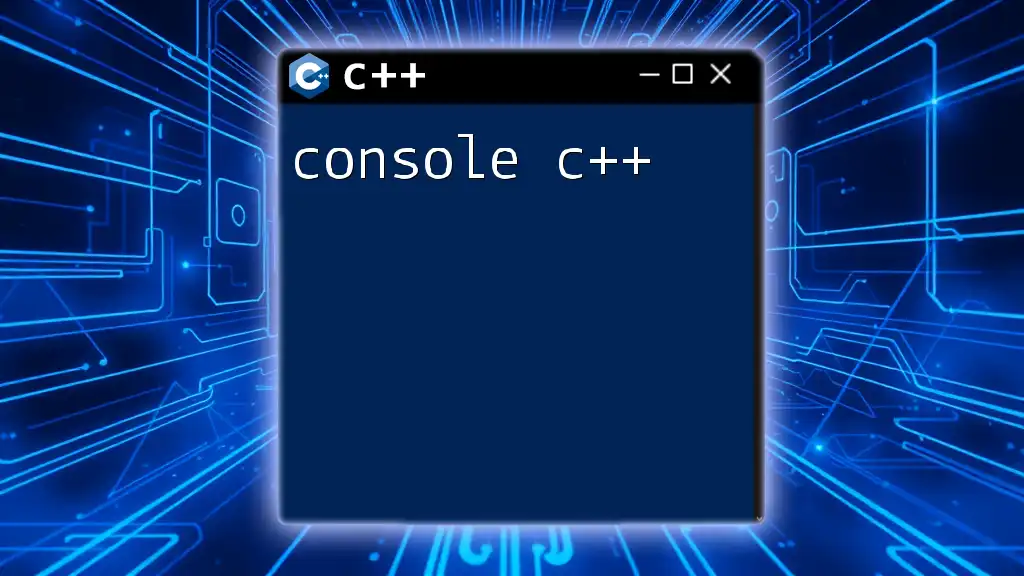
Additional Resources
For further exploration, look into C++ data type documentation and coding challenges that revolve around using the `long` data type. These resources will solidify your understanding and help you practice the concepts discussed in this guide.