"Absolute C++" refers to a comprehensive approach to learning C++ programming that emphasizes clarity and simplicity, making it accessible for beginners and effective for seasoned programmers.
Here's a simple code snippet demonstrating a basic "Hello World" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Absolute C++?
Absolute C++ is both a concept and a comprehensive educational resource designed to help beginners and intermediate programmers master the intricacies of the C++ programming language. It serves as a guiding light through the complexities of C++, whether you are just starting or looking to deepen your understanding of the language. The book, often utilized as a textbook in academic settings, employs a structured approach tailored for effective learning.
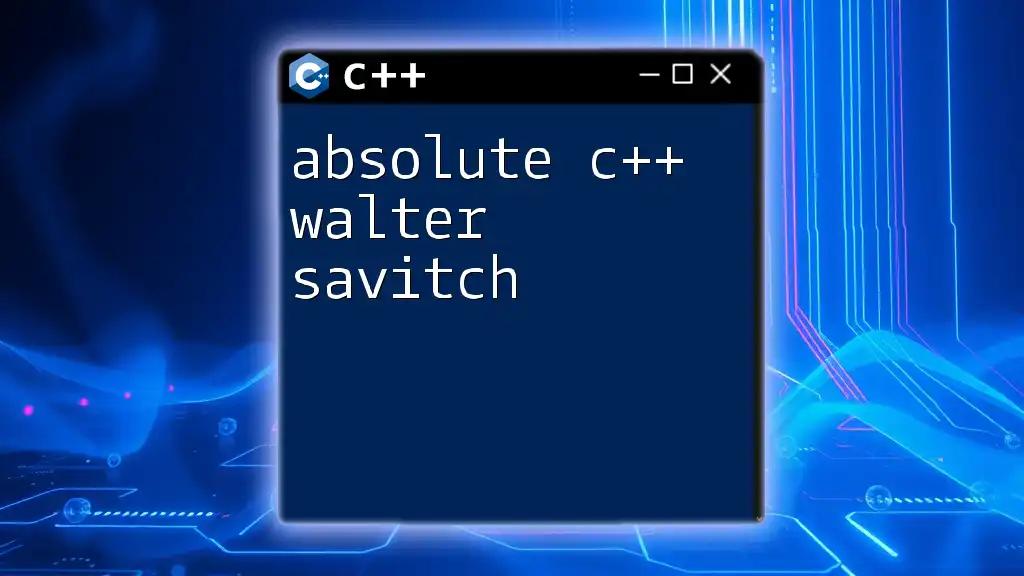
Key Features of Absolute C++
Structured Learning Approach
The brilliance of Absolute C++ lies in its step-by-step methodology. The content is organized in a logical flow, beginning with fundamental concepts and gradually introducing more complex topics. This structure helps build a solid foundation, ensuring that readers acquire the necessary skills before moving on to advanced features.
Hands-on Examples
Absolute C++ emphasizes hands-on learning, a crucial factor in programming education. The book contains numerous practical coding exercises that allow learners to apply what they have learned. This way, you can test your understanding and reinforce the concepts, making it easier to recall information when you need it.
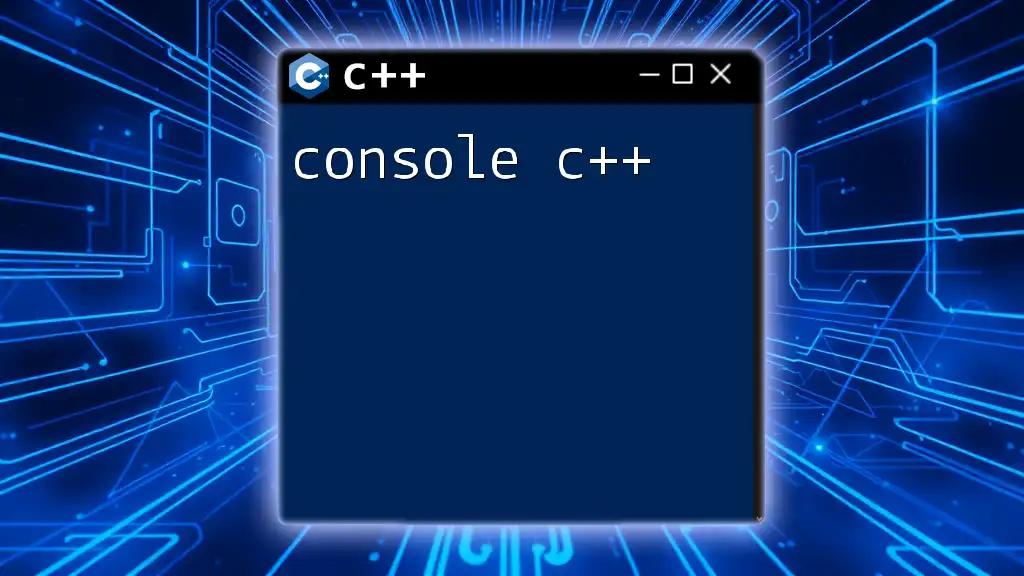
Getting Started with C++
Setting Up Your Environment
Before diving into the world of C++, it's essential to have a proper development environment set up. Here's how you can do that:
- Installation of C++ Compilers: Popular compilers like GCC, Clang, or MSVC can be installed based on your OS. For Windows, Visual Studio is a widely-used and robust choice.
- Recommended Integrated Development Environments (IDEs): IDEs like Code::Blocks, Eclipse CDT, and Visual Studio provide user-friendly interfaces and debugging tools that enhance the coding experience.
Your First C++ Program
Creating your first C++ program is a moment of pride. Let’s start with a simple "Hello, World!" example that illustrates the basic structure of a C++ program.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this code snippet:
- `#include <iostream>` allows you to use the standard input-output library.
- `using namespace std;` tells the compiler to use the standard namespace, so you don’t have to prefix `std::` before types like `cout`.
- The `main()` function acts as the entry point of the program, where execution begins.
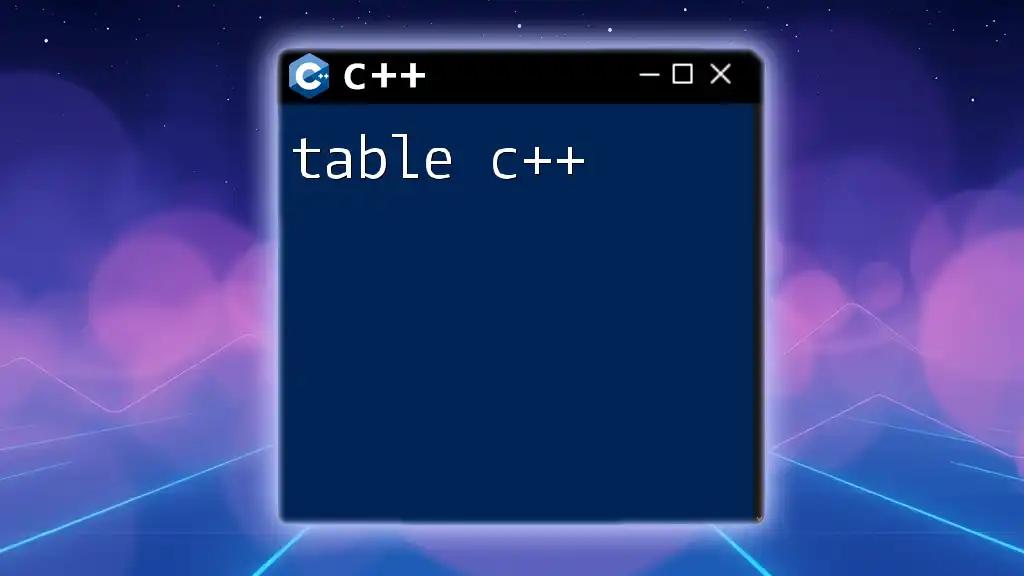
Basic C++ Concepts
Variables and Data Types
Understanding variables and data types is crucial for any programming language. C++ offers several primary data types that you'll frequently use, such as:
- int (Integer numbers): e.g., `int age = 30;`
- float (Floating-point numbers): e.g., `float height = 5.9;`
- char (Single characters): e.g., `char initial = 'A';`
- string (Strings of characters): e.g., `string name = "Alice";`
Control Structures
Control structures dictate the flow of execution in your program. C++ provides conditional statements (like `if` and `switch`) as well as looping mechanisms (like `for`, `while`, and `do-while`).
For instance, using an if-else statement allows you to make decisions based on certain conditions:
if (age > 18) {
cout << "You are an adult." << endl;
} else {
cout << "You are not an adult." << endl;
}
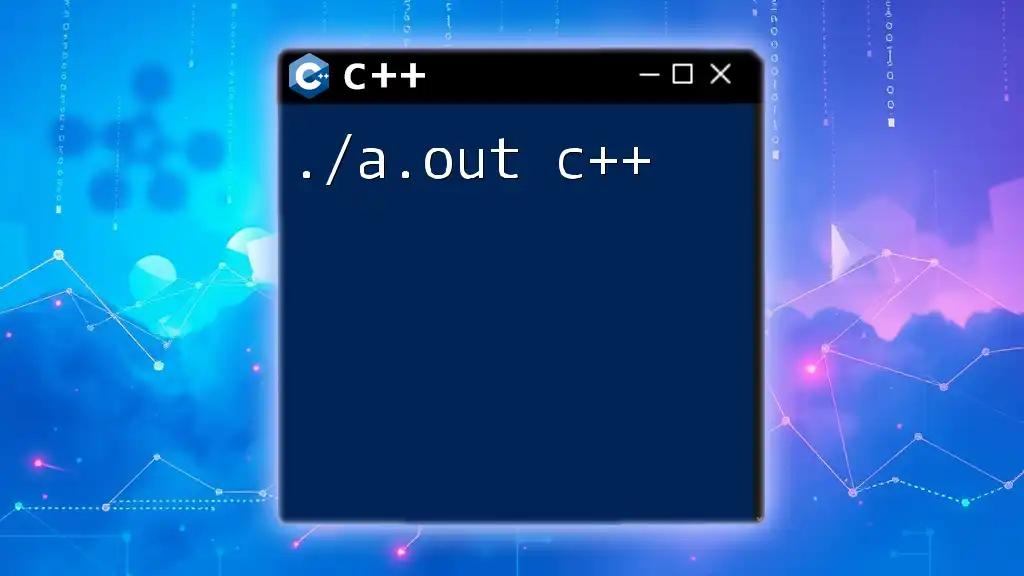
Object-Oriented Programming in C++
Core Principles
C++ is well-known for its support of Object-Oriented Programming (OOP), which includes core principles such as encapsulation, inheritance, and polymorphism. These principles allow you to design your code more efficiently and promote code reusability.
Creating Classes and Objects
Applying OOP begins with defining classes and creating objects. Here's how you can define a simple class called `Dog` and create an object of that class:
class Dog {
public:
string name;
void bark() {
cout << name << " says Woof!" << endl;
}
};
Dog myDog;
myDog.name = "Buddy";
myDog.bark();
In this code, the `Dog` class has a member variable `name` and a member function `bark()`. When we create an object `myDog` and set its `name`, calling `bark()` displays the message.
Constructors and Destructors
Constructors are special functions invoked when an object is created, allowing it to initialize its member variables. Conversely, destructors are called when an object is destroyed, providing a chance to release resources effectively.
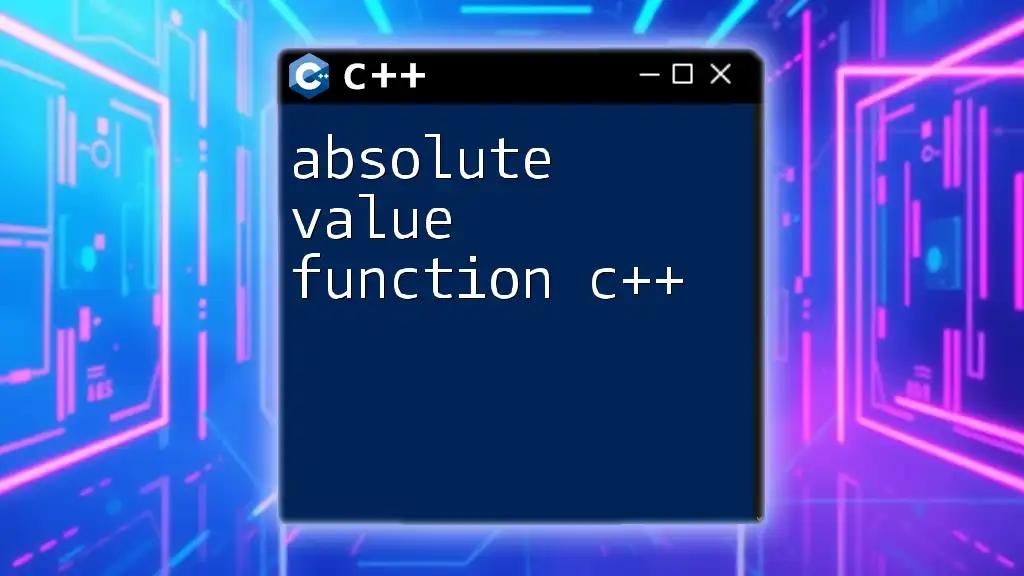
Advanced C++ Features
Templates
Templates enable code reusability by creating functions and classes adaptable to different data types. For example, a function template for swapping two values can be defined as:
template <typename T>
void swap(T &a, T &b) {
T temp = a;
a = b;
b = temp;
}
This flexibility means the same function can swap integers, floats, and even custom objects.
Standard Template Library (STL)
The Standard Template Library (STL) is a vital component of C++, providing pre-built classes and functions for various data structures. Important components include vectors, maps, and iterators, significantly simplifying your coding tasks. A quick example of using a vector in C++:
#include <vector>
using namespace std;
vector<int> numbers = {1, 2, 3, 4, 5};
Vectors dynamically store elements, making it easy to manipulate collections of data.
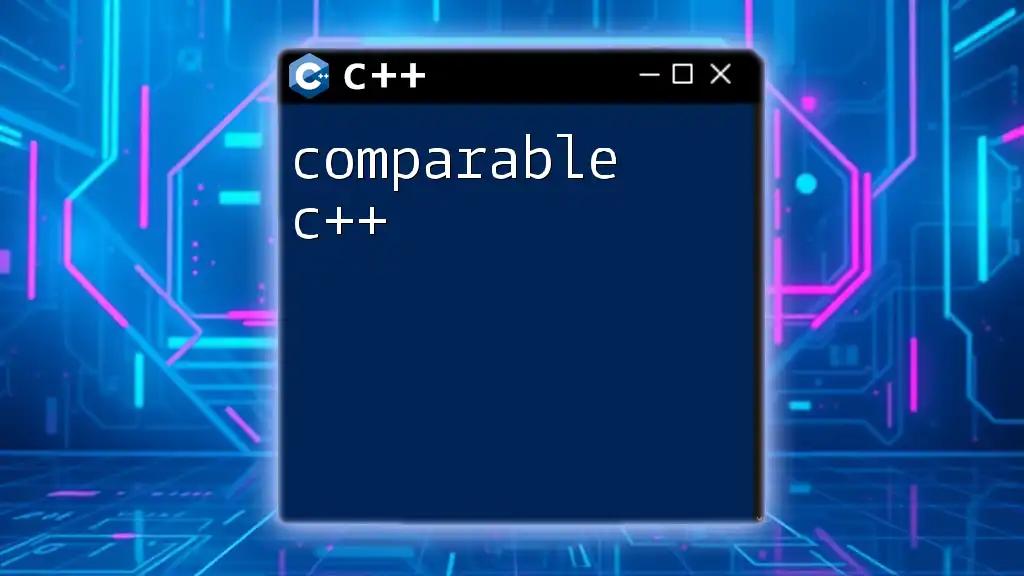
Debugging and Best Practices
Common Errors in C++
Even experienced C++ programmers encounter errors. Common issues include syntax errors, type mismatches, and runtime errors. Mastering the debugging process involves understanding compiler feedback, using debug modes, and employing print statements to trace the code's execution flow.
Coding Best Practices
To write clean and maintainable C++ code, adhere to the following guidelines:
- Comment your code effectively. Use comments to explain the why behind complex logic.
- Follow a consistent naming convention for functions, classes, and variables.
- Keep functions short and focused on a single task to enhance readability and maintainability.
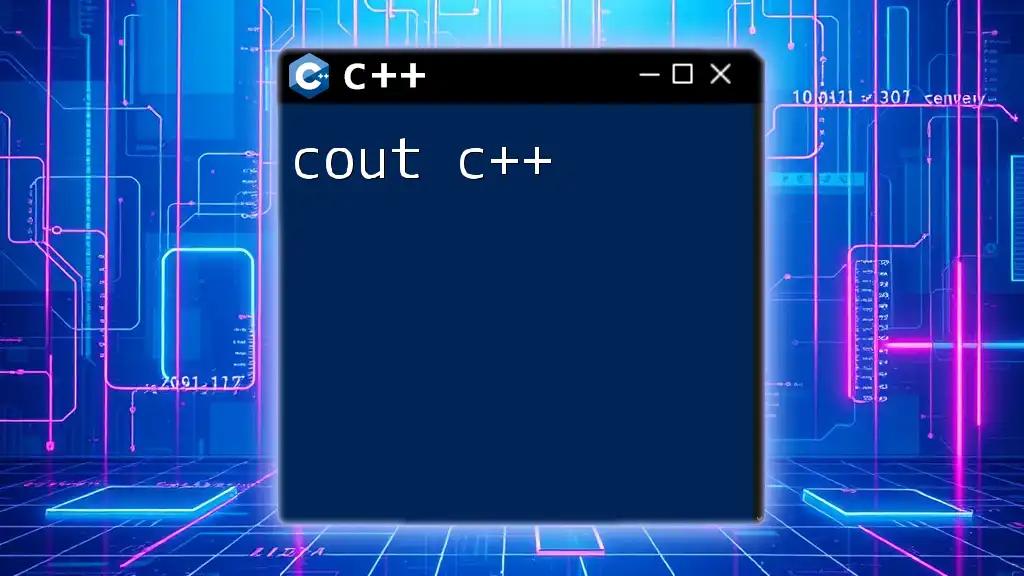
Conclusion
Mastering C++ is a journey that requires dedication and practice. Resources like Absolute C++ provide a structured framework that is invaluable in navigating this complex language. Regular coding practice is essential to reinforce what you've learned. Seek opportunities to apply your skills in projects or workshops to deepen your understanding of C++ and become a proficient programmer.
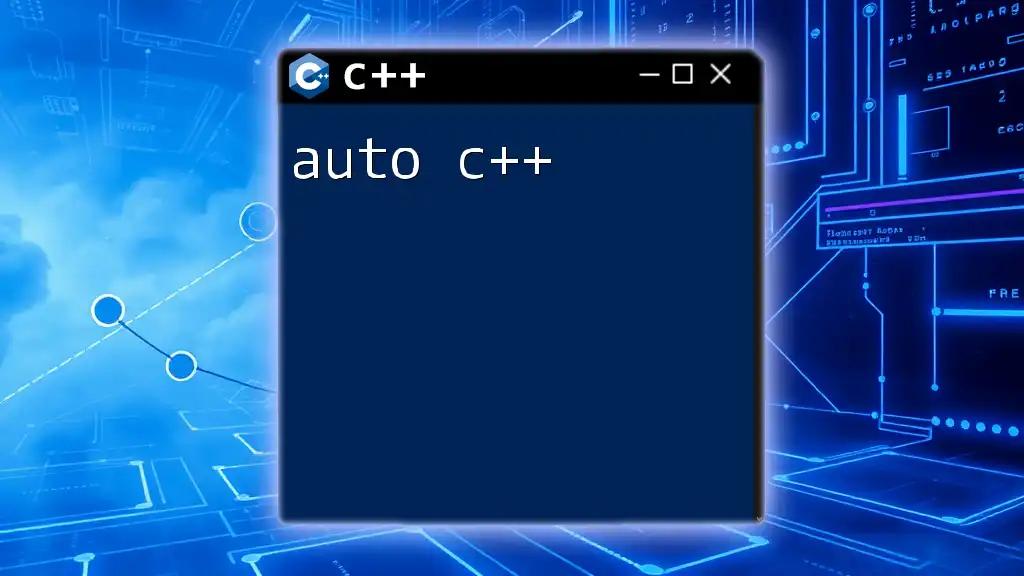
Additional Resources
For ongoing learning, several resources can complement your education:
- Online tutorials and coding platforms.
- C++ programming forums like Stack Overflow for community support.
- Books focused on advanced C++ topics and design patterns.
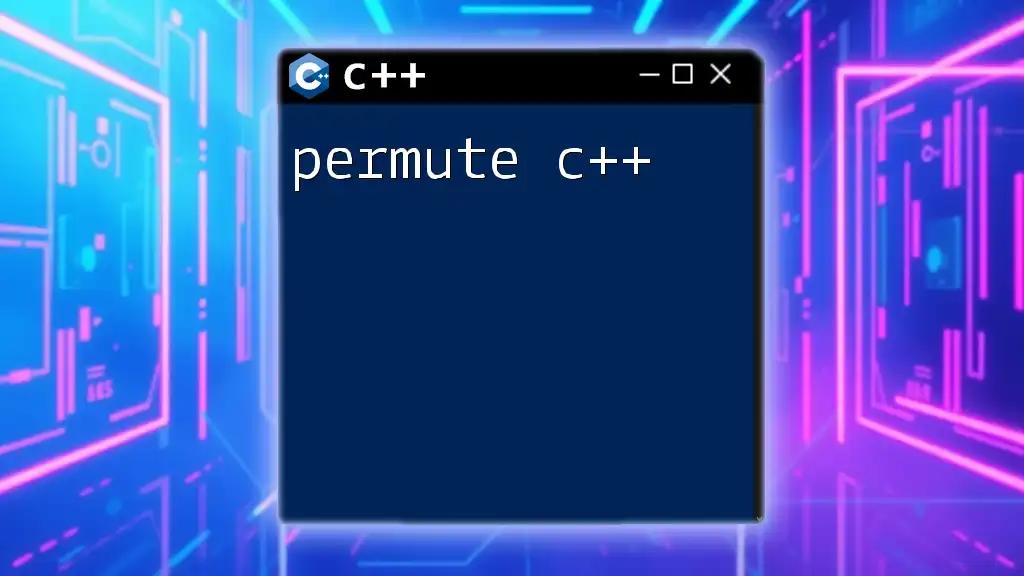
FAQs
What is the best way to learn C++?
Engaging in hands-on practice while utilizing structured resources like Absolute C++ can significantly enhance your learning experience.
How do I handle errors in C++?
Error handling becomes easier by familiarizing yourself with common errors, utilizing debug tools, and practicing error resolution techniques.
By adhering to the guidelines and applying the concepts presented, you're well on your way to becoming proficient in C++ programming through the powerful insights of Absolute C++.