The command `./a.out` is used in the terminal to execute a compiled C++ program named `a.out`, which is the default output filename generated by the g++ compiler when no output filename is specified.
Here's a simple example of a C++ program and how to compile and run it:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile and run this program, you would use the following commands in your terminal:
g++ -o myprogram myprogram.cpp
./myprogram
Understanding the Output File: a.out
What is a.out?
a.out is the default name for the executable file generated by the GNU Compiler Collection (GCC) when you compile a C++ program without specifying an output file name. The name itself stands for "assembler output." It has its origins in Unix systems, where it became a standard among developers. While a.out might seem like a relic from the past, it remains relevant today, especially for quick tests and simple programs.
How is a.out Generated?
When you write a C++ program and compile it using the GNU compiler with the command `g++ source.cpp`, the compiler processes your source code, converting it into machine-readable instructions. The end result of this complex translation is an executable file named a.out. This command can be broken down as follows:
- g++: The C++ compiler command.
- source.cpp: The source file you want to compile.
After the compilation process is successful, you will find a.out in the same directory as your source code—this is your compiled program, ready to be executed.
Example of Creating a.out
To illustrate what happens at this stage, let’s assume you have a simple file named `hello.cpp`. You can compile it as follows:
g++ hello.cpp
Upon successful compilation, you will see an a.out file created in the directory. You can check it by running `ls` in your terminal.
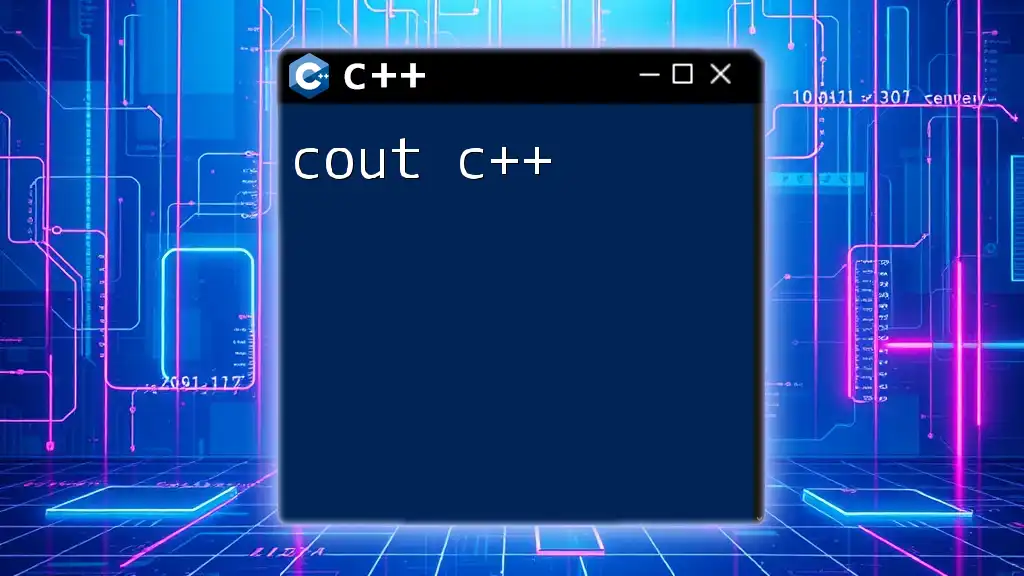
Running Your Program: Using ./a.out
The Importance of the `./` Syntax
When executing programs in Unix/Linux systems, you often need to specify the current directory with `./` to indicate that the program should be run from the directory you are currently in. This is a security measure to prevent accidentally running scripts or executables from other locations that could pose risks.
How to Execute a.out
To run your compiled program, you type the following command in your terminal:
./a.out
This command will execute a.out, and you will see the program's output directly in your terminal.
Example: Full Workflow
Writing a Sample Program
Here is a simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This program prints "Hello, World!" to the console. It serves as a classic starting point for learning C++.
Compiling and Running the Program
To compile and run the above program, follow these commands:
g++ hello.cpp
./a.out
When you execute a.out, you should see:
Hello, World!
This output verifies that the program has compiled and executed successfully.
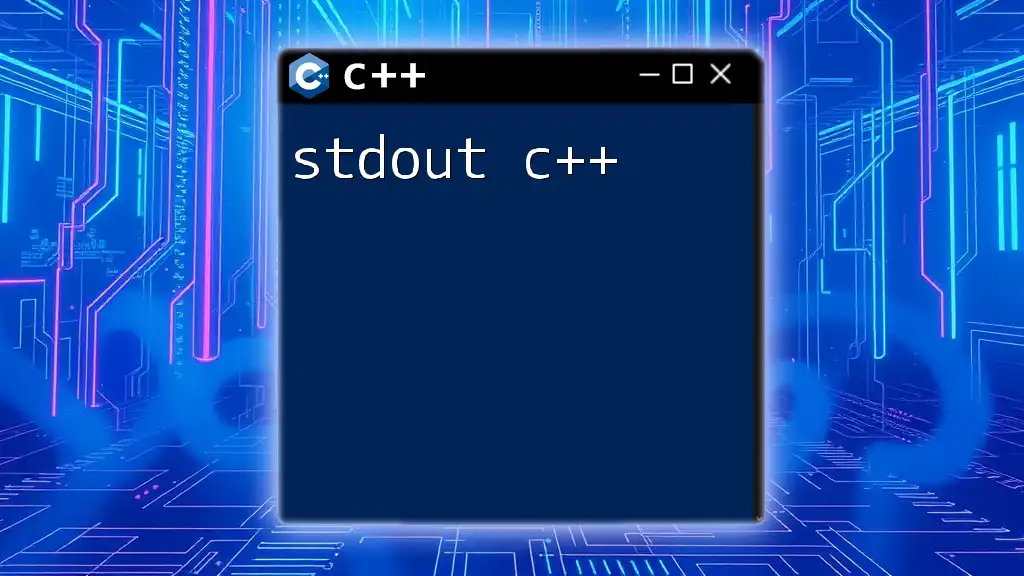
Customizing the Output Executable Name
Understanding Different Executable Names
Using a.out can quickly become inconvenient, especially for larger projects or multiple sources. Developers often prefer custom names for improved organization and clarity. It allows better identification of executables relative to their source files.
Using the -o Option in g++
To customize the name of the output executable, you can use the `-o` option. This is particularly helpful for managing multiple executables. The command looks like this:
g++ hello.cpp -o hello_program
Here, hello_program is the custom name you are assigning to your executable.
Example of Running Custom Executables
Once you compile with a custom name, you can run it with:
./hello_program
This method provides clarity over which program is being executed, especially in multiple-file projects.
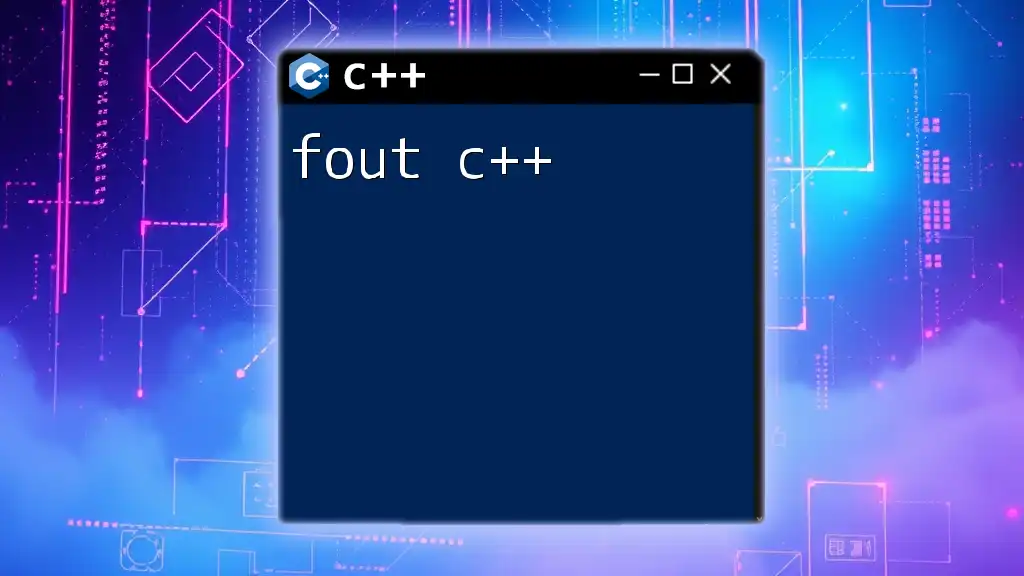
Troubleshooting Common Issues
Running Permission Errors
Sometimes, you may encounter "Permission denied" errors when trying to run a.out or your custom executable. This occurs if the file does not have the necessary execute permissions. You can resolve this by using the `chmod` command:
chmod +x a.out
This command adds executable permissions to the file, allowing you to run it without issue.
Debugging Compilation Errors
If your program fails to compile, you might see error messages in your terminal. Common issues include mismatched brackets or syntax errors. Read the compilation output carefully, as it usually points to the line number where the error originates, allowing for easier debugging.
Runtime Exceptions
Even if your program compiles without error, it may still encounter issues during execution. Examples include division by zero or underflow/overflow errors. To aid in debugging such runtime exceptions, you can use debugging tools like `gdb` (GNU Debugger) to step through your code and identify the problem.
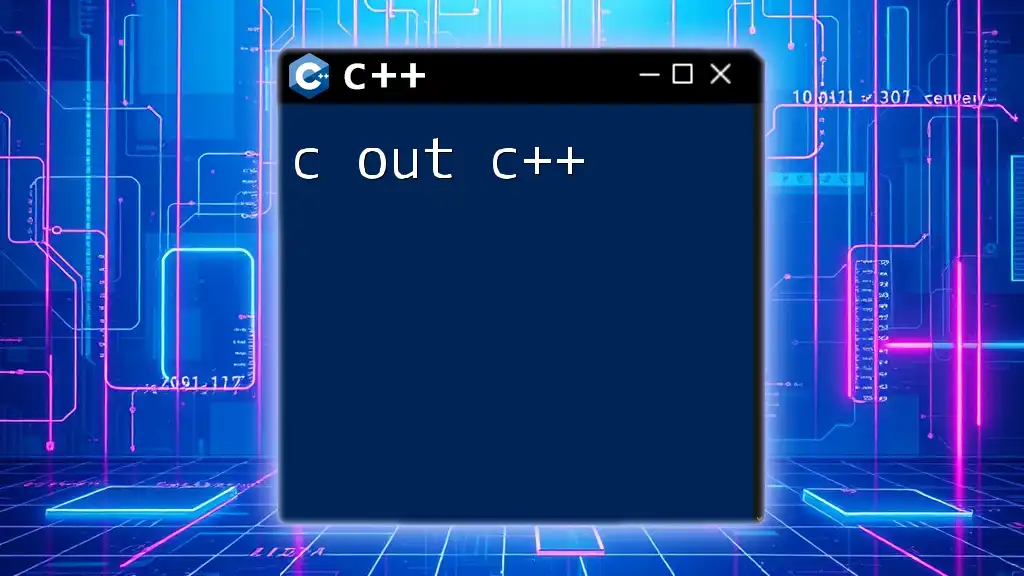
Conclusion
Mastering the command `./a.out c++` is essential for beginners learning C++. Understanding the compilation process and how to execute the resulting executable will empower developers to create and run their programs effectively. As you progress, remember to explore custom executable naming and regularly troubleshoot common issues to enhance your development skills.
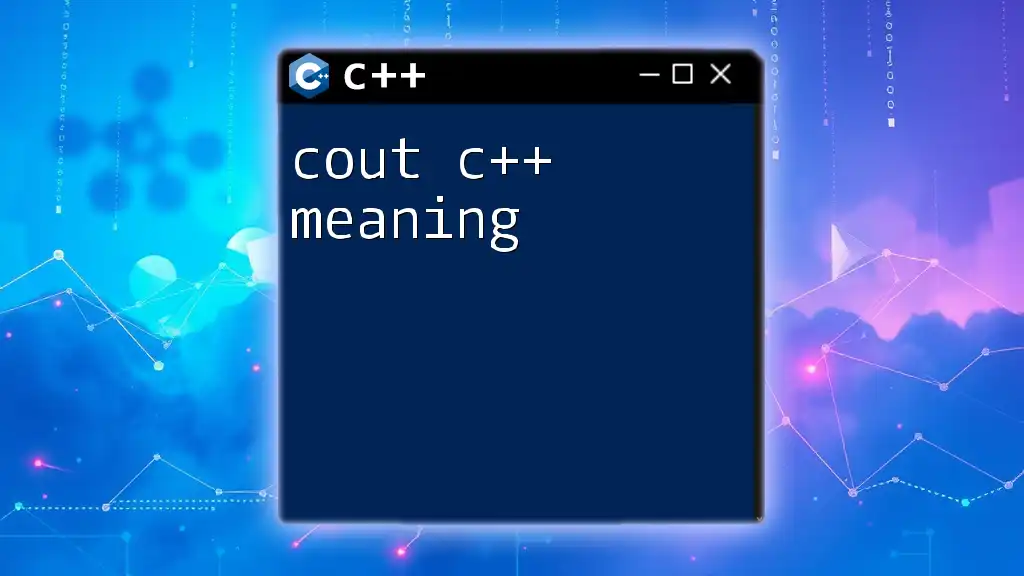
Call to Action
If you're eager to delve deeper into C++ programming, consider exploring more in-depth tutorials and resources available on our website. Your programming journey is just beginning, and there's so much more to learn!