A segmentation fault (segfault) in C++ occurs when a program attempts to access an invalid memory location, often resulting from dereferencing a null or uninitialized pointer.
Here’s a simple code snippet that demonstrates a segfault:
#include <iostream>
int main() {
int* ptr = nullptr; // Uninitialized pointer
std::cout << *ptr; // Dereferencing a null pointer causes a segmentation fault
return 0;
}
What is a Segmentation Fault?
A segmentation fault, often abbreviated as segfault, is a specific kind of error that occurs when a C++ program attempts to access a memory location that it's not allowed to access. This is a critical issue that usually results in the termination of the program and can be a frustrating problem for developers to debug. Understanding segmentation faults is vital for writing robust and efficient C++ code.
Common Causes of Segmentation Faults
Most segmentation faults arise in a few typical scenarios. Understanding these causes can help developers avoid them in the first place.

Understanding Memory Management in C++
Memory Basics in C++
C++ has two primary types of memory allocation: stack memory and heap memory. The stack is used for static memory allocation, which means that the amount of memory is determined at compile time. The heap, on the other hand, is used for dynamic memory allocation, allowing for memory allocation during runtime.
Role of Pointers in Memory Management
Pointers are variables that store memory addresses. They allow for flexible memory management in C++, but improper use of pointers can lead to severe consequences, including segmentation faults. It is crucial to understand how to use pointers safely to prevent these issues.
The Concept of Undefined Behavior
C++ is known for its undefined behavior, which means that the C++ standard does not specify what should happen in certain situations. Some operations, such as dereferencing a null pointer or accessing an out-of-bounds array, yield unpredictable results, which can include segmentation faults. Understanding and being vigilant about undefined behavior is essential for writing reliable C++ code.

Common Causes of Segmentation Faults
Dereferencing Null Pointers
One of the most common causes of a segmentation fault is dereferencing a null pointer. A null pointer is a pointer that does not point to any valid memory location.
For instance, consider the following code:
int* ptr = nullptr;
std::cout << *ptr; // Causes segmentation fault
Here, the attempt to dereference `ptr`, which is null, will lead to a segmentation fault.
Prevention Techniques: Always check if a pointer is `nullptr` before dereferencing it. Using smart pointers, introduced in C++11, can help manage memory better and reduce the risk.
Accessing Invalid Memory Locations
Another common source of segfaults is accessing an invalid memory location, particularly through out-of-bounds array indexing.
Here’s an example:
int arr[5] = {1, 2, 3, 4, 5};
std::cout << arr[10]; // Causes segmentation fault
Accessing `arr[10]` is illegal because it exceeds the bounds of the array.
Solutions and Best Practices: Always ensure that any index used to access an array is within the allocated range. Consider using data structures like `std::vector` in C++ that perform bounds checking when accessed.
Dangling Pointers
A dangling pointer occurs when an object is deleted, or the pointer goes out of scope, but the pointer still holds the address of the deallocated memory.
Consider this example:
int* ptr;
{
int temp = 42;
ptr = &temp; // temp goes out of scope, ptr becomes dangling
}
std::cout << *ptr; // Causes segmentation fault
In this code, once `temp` goes out of scope, `ptr` becomes a dangling pointer. Attempting to dereference it will cause a segmentation fault.
How to Avoid Dangling Pointers: One strategy is to use smart pointers (e.g., `std::shared_ptr`, `std::unique_ptr`) which automatically manage memory.
Stack Overflow
Stack overflow occurs when too much memory is used on the call stack, often due to excessively deep recursion.
Example of a problematic recursive function:
void recursiveFunction() {
recursiveFunction(); // Stack overflow
}
Here, `recursiveFunction` calls itself indefinitely until the stack limit is reached, resulting in a segmentation fault.
Strategies for Preventing Stack Overflow: Be cautious with recursion; ensure a base case or limit depth.
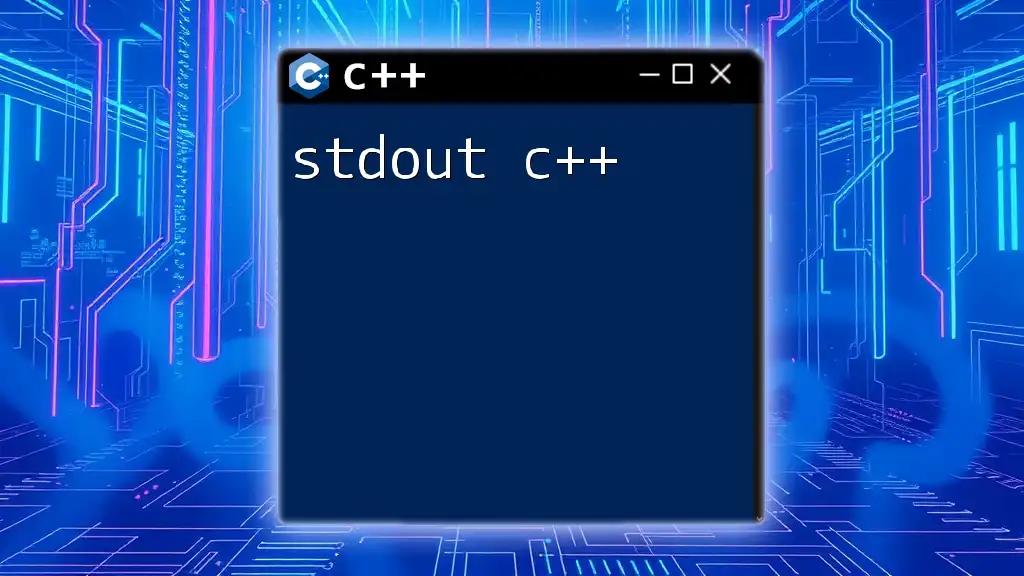
Debugging Segmentation Faults
Using Debuggers
Using debug tools like GDB (GNU Debugger) can significantly aid in identifying the source of segmentation faults. Common GDB commands to debug segfaults include `run`, `bt` (backtrace), and `list`. These allow you to see where the segfault occurred.
Printing Stack Traces
Enabling stack traces can provide valuable insight into what functions were called leading up to a segmentation fault, helping to locate the problematic code more easily.
Utilizing Static Analysis Tools
Static analysis tools like Valgrind are invaluable for detecting memory issues. A simple command:
valgrind ./your_program
This command will run your program and report memory leaks, invalid memory accesses, and other related errors. Using these tools can help prevent segment faults before your code runs.
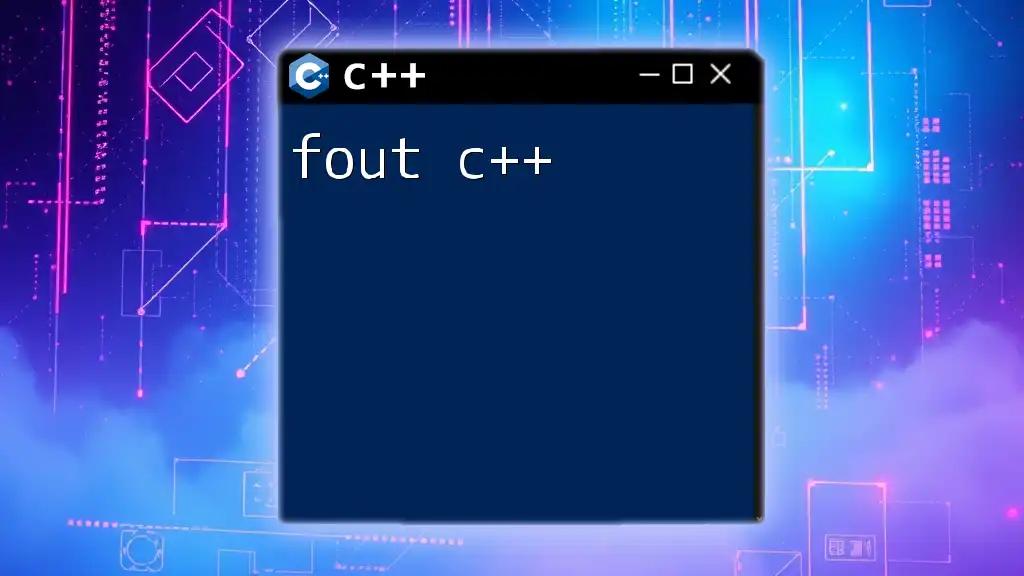
Handling Segmentation Faults
Error Handling Techniques in C++
C++ provides mechanisms to handle errors gracefully. Using exceptions can help manage and recover from runtime errors, including segmentation faults.
Here's a simple example of exception handling:
try {
// code that may cause a segmentation fault
} catch (const std::exception& e) {
std::cerr << "Caught exception: " << e.what() << '\n';
}
This approach allows you to catch and deal with potential issues without crashing your program.
Best Practices for Preventing Segfaults
To prevent segmentation faults, consider adopting these best practices:
- Always initialize pointers before use.
- Check that pointers are valid before dereferencing.
- Use `std::vector` and other standard libraries that manage memory and provide bounds checking.
- Prefer smart pointers over raw pointers to manage memory effectively.
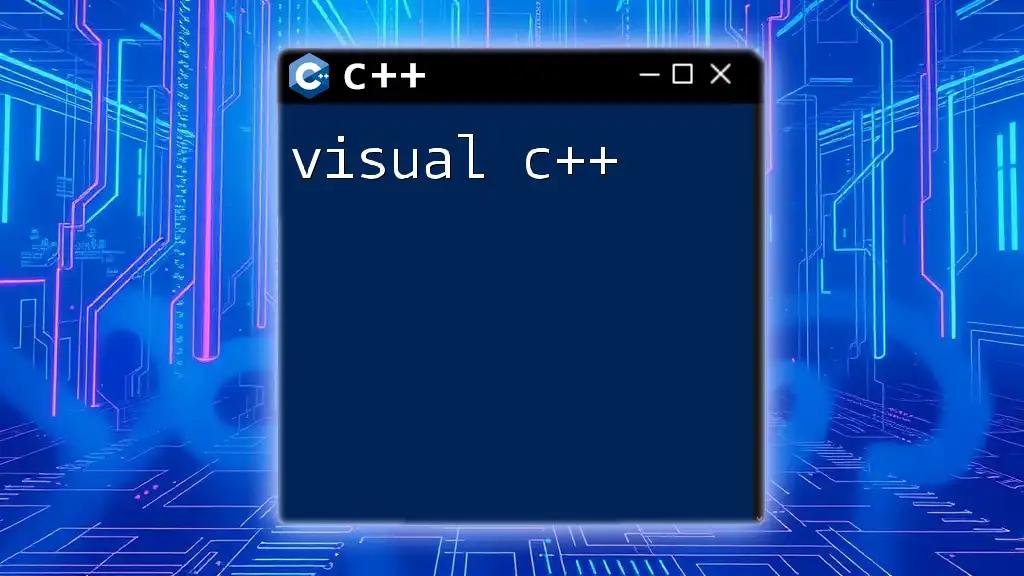
Conclusion
Understanding segmentation faults in C++ is crucial for writing reliable and efficient code. By familiarizing yourself with the causes and debugging techniques, you can develop a strong foundation in memory management and circumvent common pitfalls. Practice safe C++ coding techniques today, and avoid the frustrations of segfaults in your applications.

Additional Resources
For those looking to delve deeper into C++ and memory management, consider exploring recommended books on C++ programming and online tutorials that can offer further insight into sophisticated memory handling techniques. The journey of mastering C++ entails consistent practice and learning, especially about intricate issues like segmentation faults.