In C++, `stdout` is a predefined output stream used for displaying output to the console, often accessed through the `cout` object from the `<iostream>` library.
Here’s a simple code snippet demonstrating how to use `stdout` with `cout`:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is stdout?
In C++, stdout refers to the standard output stream, which is a default output destination for a program. By convention, when you print output without specifying a file or another output stream, the content is displayed on the console window, or terminal, from which the program is run. The significance of stdout lies in its role as the primary means for applications to communicate output to the user, whether it's for regular informational messages, debugging purposes, or results of computations.
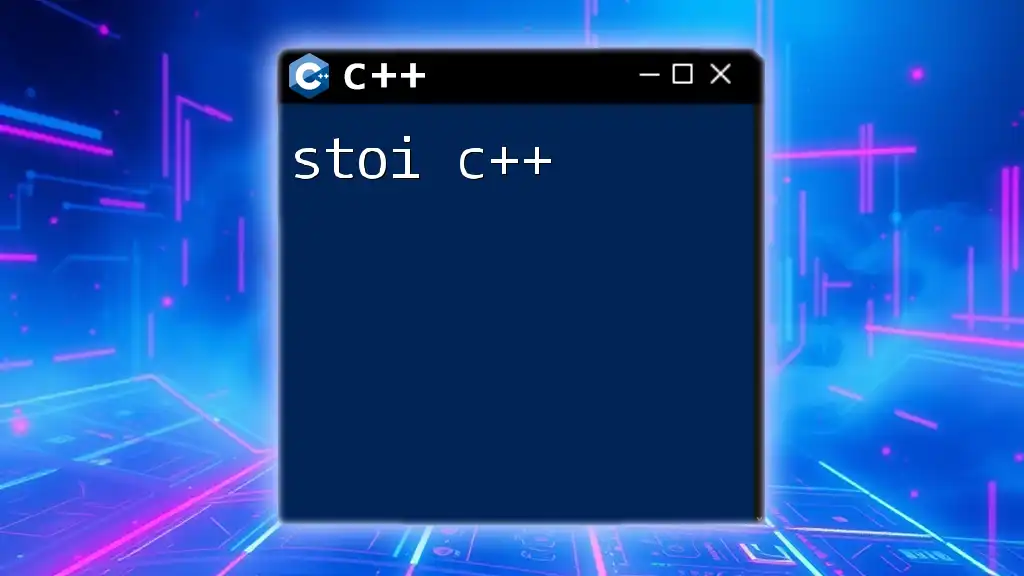
How stdout Works in C++
The mechanism behind stdout in C++ is built upon the C standard library. When you use `std::cout`, you are accessing a stream object linked directly to the operating system's standard output device, typically the console screen. It's essential to understand that stdout works through a buffering system managed by the operating system. When you send data to stdout, it may not appear immediately on your screen; instead, it can be temporarily stored in a buffer until it's full or explicitly flushed.
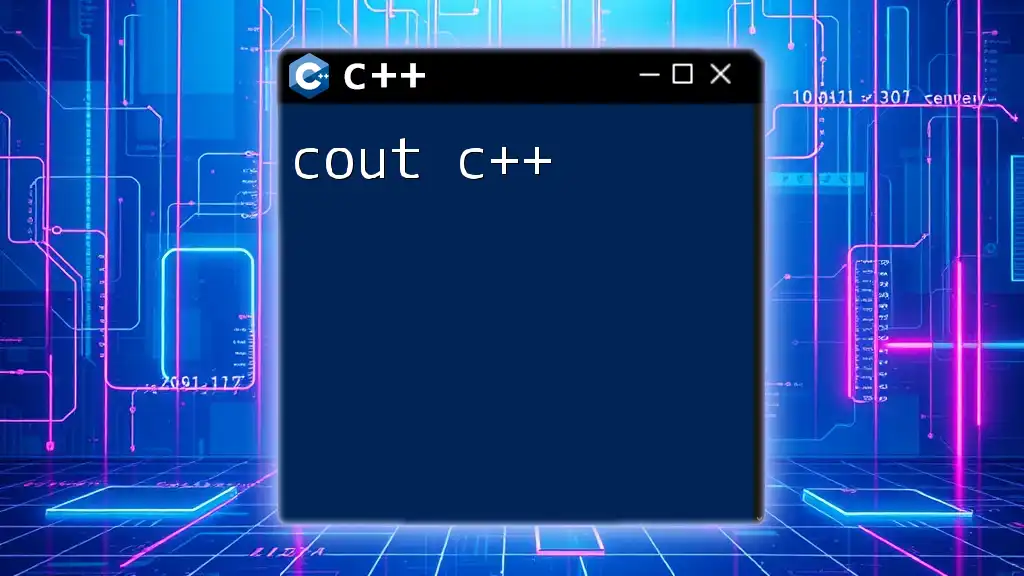
Basic Usage of stdout in C++
Printing to stdout
One of the most common ways to output data to stdout in C++ is by using the `cout` stream, which is defined in the `<iostream>` header file. The syntax to output data is straightforward:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, `std::cout` initiates the output stream. The `<<` operator, known as the stream insertion operator, directs the string `"Hello, World!"` to the output stream. The `std::endl` manipulator is used to insert a newline character and flush the buffer, ensuring that the message appears immediately.
Common Output Manipulators
C++ provides several output manipulators that enhance the formatting of the output. These include `std::endl`, which not only adds a newline character but also flushes the output buffer, and `std::setw`, which sets the width of the next output field.
Here's an example demonstrating `std::setw`:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(10) << "Value" << std::endl;
std::cout << std::setw(10) << 42 << std::endl;
return 0;
}
In this snippet, `std::setw(10)` specifies that the output will occupy at least 10 spaces. This enhances readability, especially when displaying columns of data.
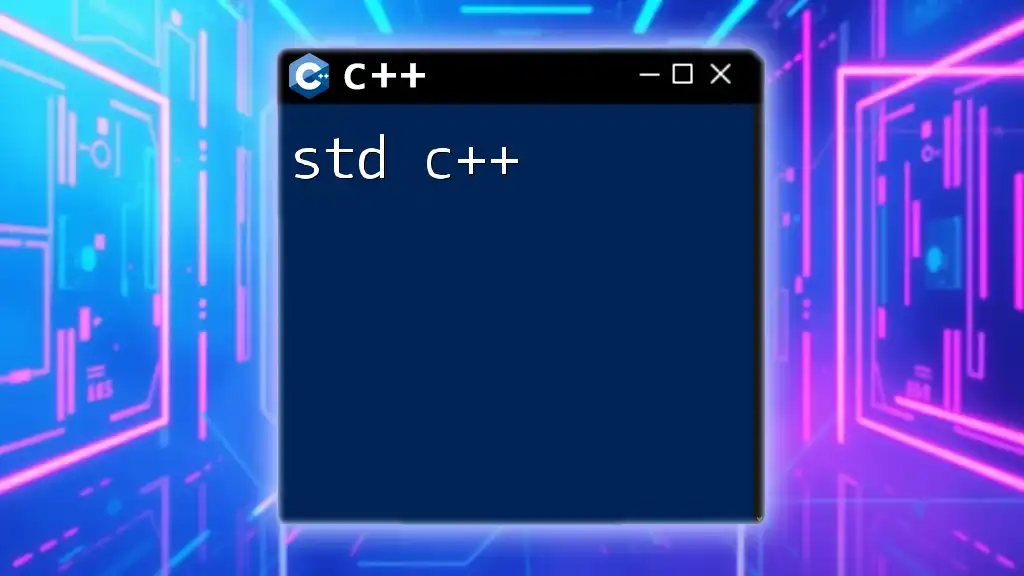
Advanced Output Techniques
Formatting Output with std::ostream
You can further customize the output using format flags available in `<iomanip>`. These flags control various formatting options, allowing you to format numbers, adjust precision, and change base representations.
Here’s an example:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::showbase << std::hex << 255 << std::endl;
return 0;
}
In this example, `std::showbase` makes sure that the output displays a prefix indicating the base of the number. When used with `std::hex`, the output will show `0xff`, illustrating how you can represent numbers in hexadecimal format.
Output Streams and Buffers
Understanding Buffers
Output streams like `std::cout` use a buffering system to increase performance. When you print output, it's initially written to a buffer rather than immediately displayed, which reduces the number of system calls and improves execution speed.
Flushing the Buffer
The output buffer is automatically flushed under certain conditions—when it reaches a specific size or when `std::endl` is used. However, you can manually flush it whenever needed, which is particularly useful in situations requiring real-time output:
#include <iostream>
int main() {
std::cout << "Hello, ";
std::cout.flush(); // manually flushes the buffer
std::cout << "World!" << std::endl;
return 0;
}
In this code, `std::flush` forces the buffer to clear, ensuring that `Hello, ` is visible to the user before "World!" is printed.
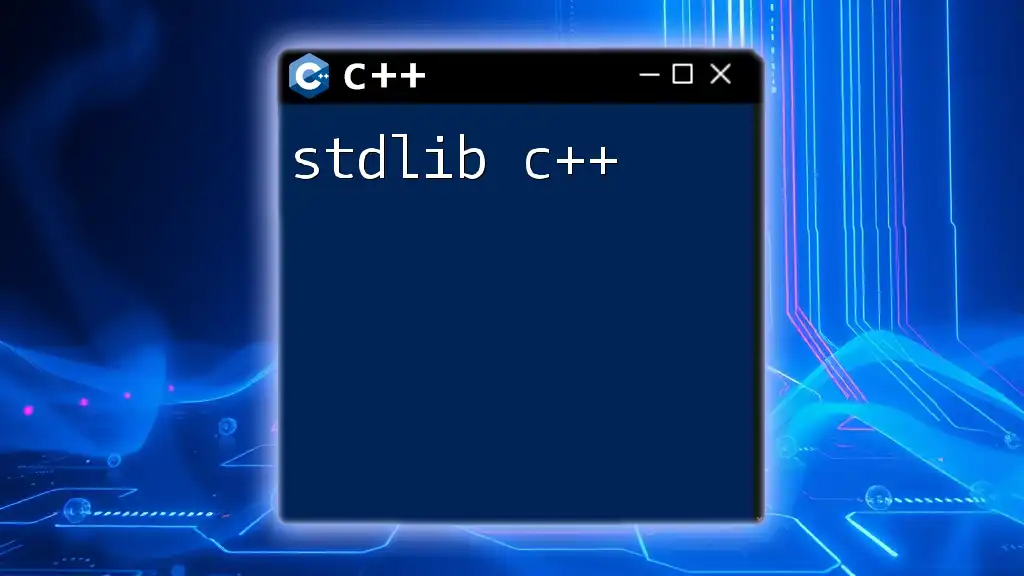
Redirecting stdout
What is Redirection?
Redirection is a powerful feature in C++ that allows you to change the destination of your output stream. Instead of printing to the console, you might want to save the output in a file or send it to another process. This flexibility is particularly useful for logging, data analysis, and batch processing.
How to Redirect stdout in C++
To redirect stdout to a file, you can use `std::ofstream` along with stream buffers. Here’s how you can accomplish this:
#include <iostream>
#include <fstream>
int main() {
std::ofstream out("output.txt");
std::streambuf* coutbuf = std::cout.rdbuf(); // save old buf
std::cout.rdbuf(out.rdbuf()); // redirect std::cout to out.txt
std::cout << "This will go to the file!" << std::endl;
std::cout.rdbuf(coutbuf); // reset to standard output
return 0;
}
In this example, we first open a file named `"output.txt"` for writing and then redirect `std::cout` to this file by changing its buffer. After printing the message, we restore the original stdout buffer, ensuring that subsequent output goes back to the console.
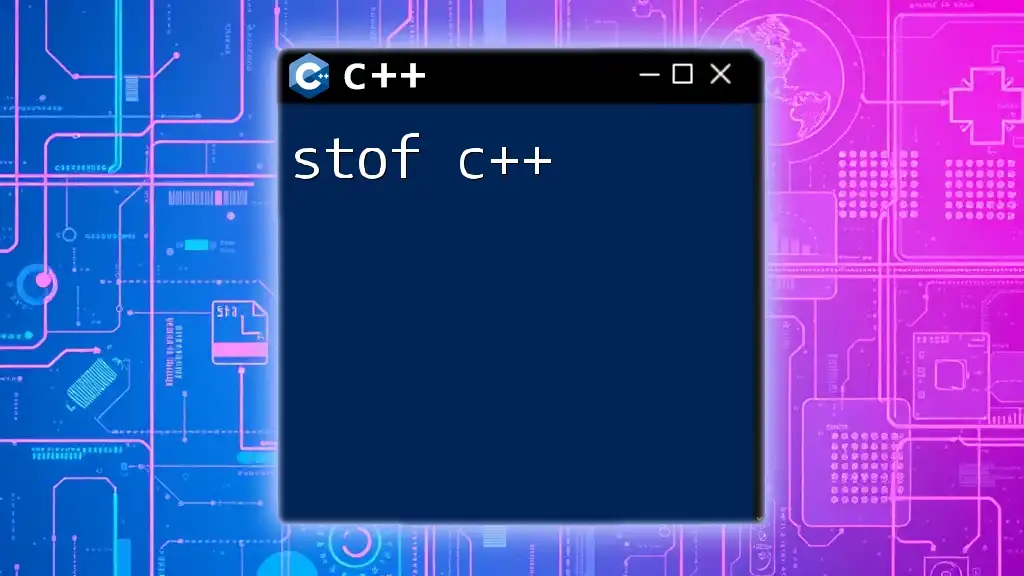
Debugging Output with stdout
Using stdout for Debugging Purposes
Using stdout for debugging is a common practice. By printing debug statements to the console, programmers can gain insights into the execution flow and variable states in their applications.
Here’s an example of a simple debugging function:
#include <iostream>
void debug(const std::string& message) {
std::cout << "[DEBUG] " << message << std::endl;
}
int main() {
debug("Application has started.");
return 0;
}
In this snippet, the `debug` function prefixes debug messages with `"[DEBUG]"`, helping you quickly identify them in your output stream. This approach can help significantly during the development and troubleshooting of applications.
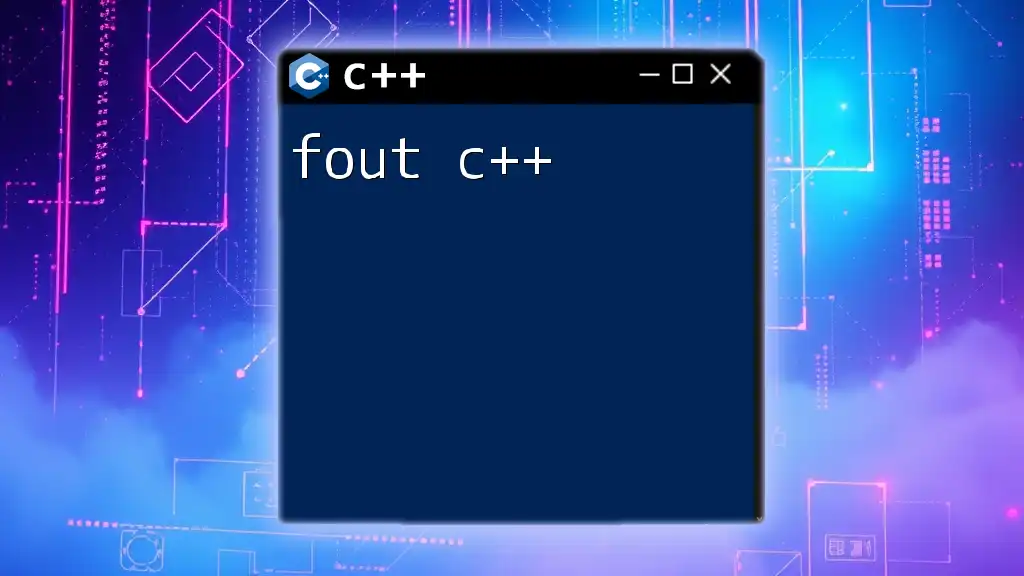
Conclusion
stdout in C++ is a fundamental concept that enables interactive communication between your program and its users. Understanding how to utilize this stream effectively—through various output techniques, formatting options, and redirection capabilities—will elevate your programming skills. Whether for simple output tasks or complex debugging scenarios, mastering stdout allows you to create more robust and user-friendly applications. As you continue your exploration of C++, remember that practice is key, and incorporating stdout effectively in your projects will enhance your coding proficiency.
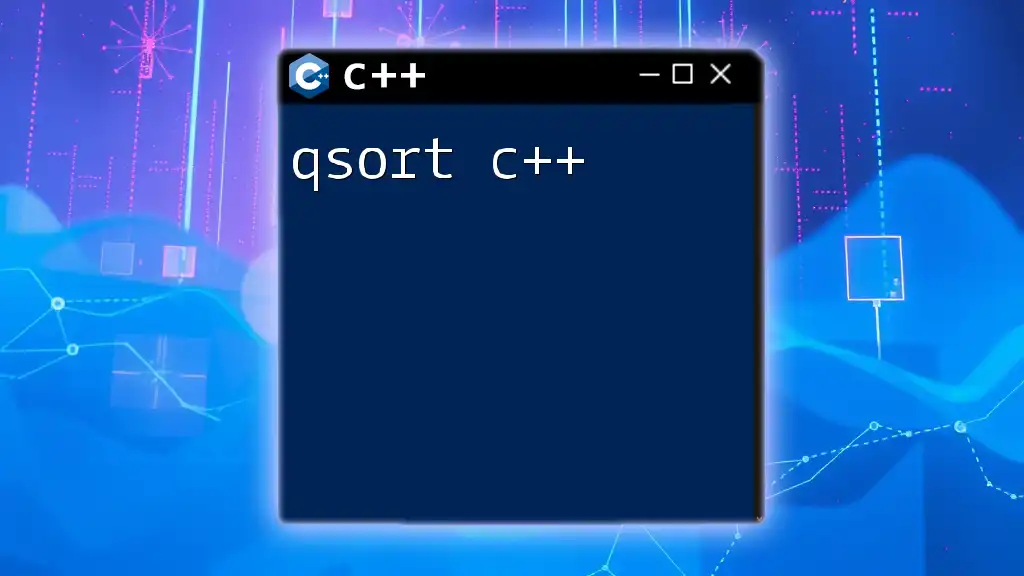
Additional Resources
For further reading, consider exploring recommended books and online tutorials focused on C++ programming. Many forums and communities can also provide valuable insights and discussions about best practices and advanced topics related to stdout in C++. Engaging with these resources will expand your knowledge and help you become a more adept C++ programmer.