The `cout` object in C++ is used to output data to the standard console, and it plays a crucial role in displaying information to users.
Here's a simple code snippet demonstrating how to use `cout`:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Output in C++
What is Output?
In programming, the term output refers to the data sent from a program to a display device, file, or other output medium. It allows users to see the results of their program's computations and actions. In C++, output is vital for effective user interaction and the verification of program flow and logic.
The Role of `cout` in C++
The `cout` object is a predefined object in C++ that is part of the iostream library. It serves as the standard output stream used to send data to the console. The use of `cout` is essential for any C++ programmer, as it helps in displaying messages, results, and debugging information to users.

Basics of Using cout
Setting Up a C++ Program
To effectively use `cout`, you need to set up a basic C++ program. This includes including the iostream header file that declares the `cout` object, among others. Here's a simple structure of a C++ program that exemplifies this:
#include <iostream>
int main() {
// Code will go here
return 0;
}
Syntax of cout
The syntax for using `cout` is straightforward. It employs the insertion operator (`<<`) to send data to the output stream. A typical use case is as follows:
std::cout << "Hello, World!" << std::endl;
Here, the phrase "Hello, World!" is sent to the console followed by an end-of-line character due to `std::endl`, which not only moves the cursor to the next line but also flushes the output buffer.
Outputting Text with cout
You can easily output strings and characters using `cout`. For instance:
std::cout << "Welcome to C++ programming!" << std::endl;
This command sends the text "Welcome to C++ programming!" to the console, serving as a greeting for anyone running your program.
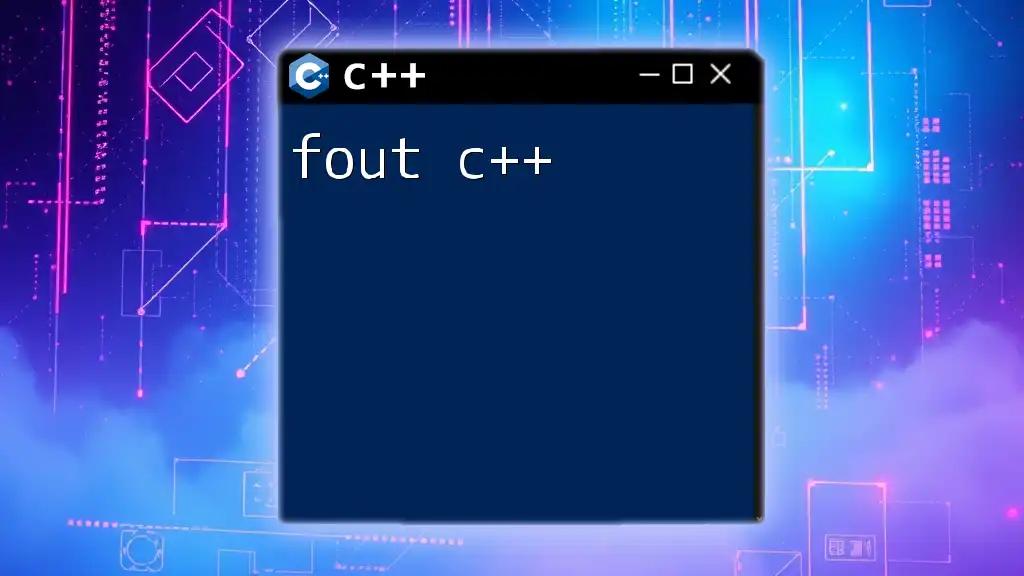
Advanced Usage of cout
Outputting Different Data Types
`cout` is capable of handling various data types, making it a flexible tool for display purposes. Below are examples that demonstrate how to output integers, floats, and characters:
int a = 10;
std::cout << "Integer: " << a << std::endl;
float b = 5.75;
std::cout << "Float: " << b << std::endl;
char c = 'A';
std::cout << "Character: " << c << std::endl;
Here, each variable is sent to the console with an accompanying message. This versatility is one of the strong points of `cout`.
Manipulators in C++
Manipulators are special functions in C++ that can modify the behavior of the output stream. Some common manipulators are `std::endl`, `std::fixed`, and `std::setprecision`. The `std::endl` cannot only print a newline but also flush the stream, ensuring all previous output is presented before moving on.
For instance, using `std::fixed` and `std::setprecision` helps format floating-point output:
#include <iomanip> // Required for std::setw and std::setprecision
std::cout << std::fixed << std::setprecision(2) << b << std::endl;
The above line will ensure that floating-point numbers are output with two decimal places, enhancing the clarity of your output.
Formatting Output with cout
C++ also provides ways to format the output visually. Functions like `std::setw` and `std::setfill` can make your console output look better. Below is an example that shows how to format the appearance of numbers:
std::cout << std::setw(10) << std::setfill('*') << a << std::endl;
In this example, `a` will be printed in a field that is at least 10 characters wide, filled with asterisks if the number is shorter. This approach is particularly useful for aligning columns of data.
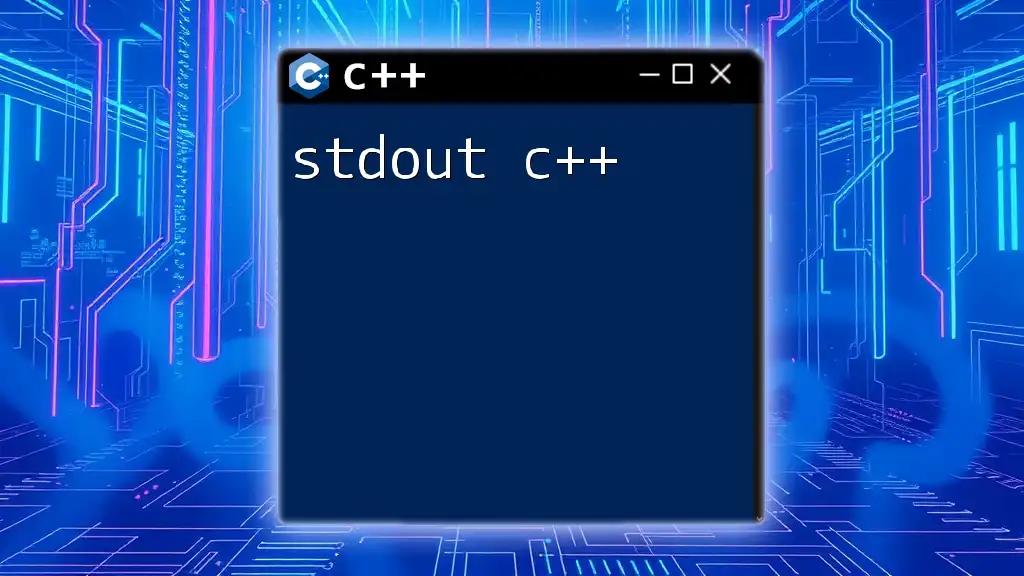
Common Issues with cout
Common Errors When Using cout
When using `cout`, there are some common pitfalls. One frequent mistake is to forget to include the `<iostream>` header file, which will lead to compilation errors. Always ensure your program starts with the necessary includes:
#include <iostream> // Make sure to include this
Debugging Output Issues
If you encounter issues with `cout`, such as missing output or unexpected results, consider checking the order of your output statements. Additionally, ensure that you are using the correct data types, as outputting a variable not properly initialized may lead to undefined behavior.
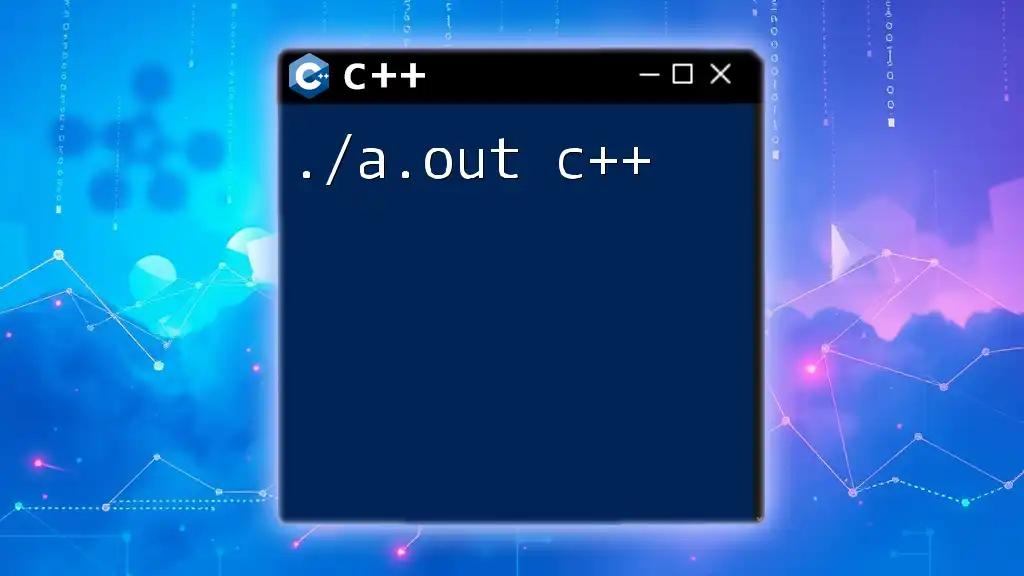
Enhancements with cout
Chaining Multiple Outputs
One powerful feature of `cout` is the ability to chain multiple outputs in a single statement using the insertion operator. This flexibility allows you to write more concise code:
std::cout << "Value of a: " << a << ", Value of b: " << b << std::endl;
In the example above, both variables `a` and `b` are printed on the same line, separated by a comma, simplifying your output code.
Using cout with Conditional Logic
`cout` can also be employed smoothly with conditional logic. This capability adds dynamic output based on program conditions. Here's an illustrative example:
if (a > b) {
std::cout << "A is greater than B." << std::endl;
}
In this scenario, only the output indicating that "A is greater than B." will be displayed if the condition holds true, demonstrating how powerful `cout` can be within control structures.
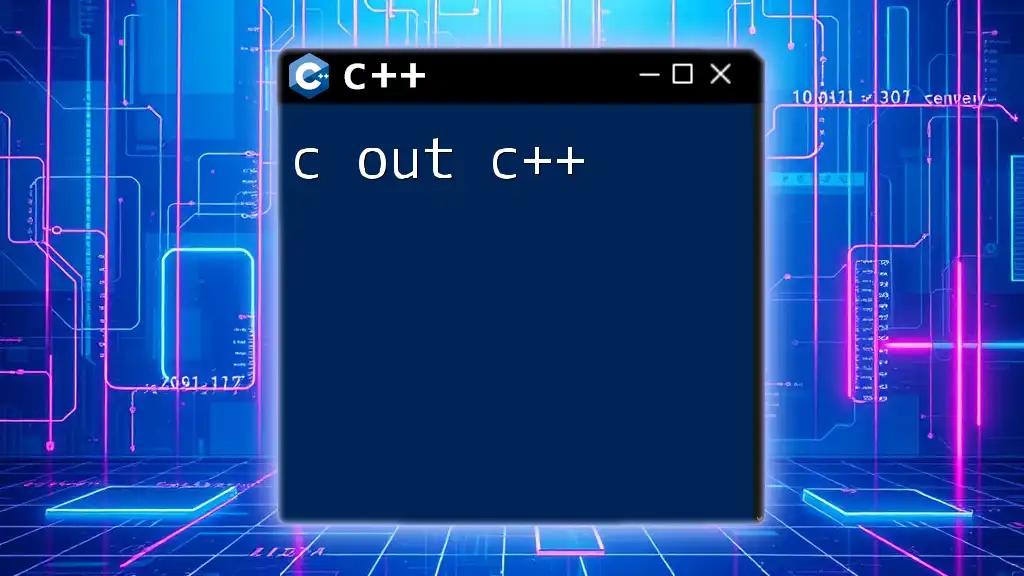
Comparing cout with Other Output Methods
Alternative Methods for Output
While `cout` is one of the most popular ways to display output in C++, there are other methods like `printf` and `puts` originating from the C language. Although these methods can be efficient, they lack the type safety and ease of use provided by `cout`.
Advantages and Disadvantages of cout
-
Pros of `cout`:
- Type safety: Automatic type conversions
- Easier syntax for formatting using manipulators
- Seamless integration with other C++ features
-
Cons of `cout`:
- May be slower due to type checking and stream formatting
By comparing these methods, you'll understand that while `cout` may have some overhead, its benefits outweigh the small performance penalties in most scenarios.

Conclusion
In conclusion, mastering `cout` is fundamental for any aspiring C++ programmer. It serves as the primary means to communicate results and data from a program to the user, making it a crucial tool in your programming toolkit. The capabilities and flexibility offered by `cout` allow you to produce clean, easily readable output, essential for effective software development.
By exploring and practicing the various aspects of `cout`, you'll not only improve your output handling but also deepen your understanding of C++. Happy coding!
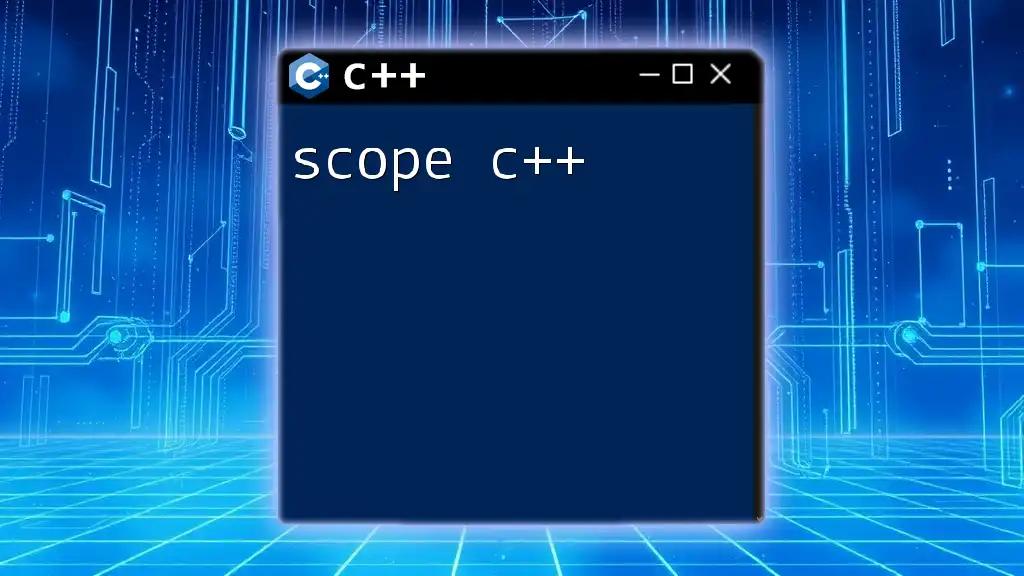
Additional Resources
For further practice and to enhance your skills, consider looking into online compilers that allow you to experiment with C++ code snippets directly. Additionally, recommended C++ programming books and educational platforms can provide structured learning paths to further bolster your knowledge and mastery of C++.