Visual Studio Code (VSCode) offers a powerful debugging environment for C++ developers, allowing you to easily set breakpoints, inspect variables, and step through your code for efficient troubleshooting.
Here's a simple code snippet demonstrating a C++ program that you can debug using VSCode:
#include <iostream>
int main() {
int a = 5;
int b = 0;
// This will cause a division by zero error
int c = a / b;
std::cout << "Result: " << c << std::endl;
return 0;
}
Make sure to set breakpoints and check the variable values while debugging in VSCode!
Setting Up Your Environment
Requirements
To effectively use VSCode for C++ debugging, you'll need to ensure you have the right software and tools installed:
- Visual Studio Code: First, download and install VSCode from the official website.
- C++ Extension: Install the C++ extension for VSCode, known as "C/C++" by Microsoft. This extension adds support for debugging, IntelliSense, and code browsing.
- Compiler: Choose a compiler based on your operating system. For Windows, MinGW-w64 (GCC) and for Mac/Linux systems, you can use GCC or Clang.
VSCode Configuration
After obtaining the necessary software, it’s crucial to set up your workspace correctly:
-
Creating Your Project Structure: Start by creating a folder for your C++ project. Within this folder, create subdirectories for your source code, headers, and any resources needed.
-
Configuring VSCode Settings:
-
Open the command palette in VSCode (with `Ctrl+Shift+P`) and use "C/C++: Edit Configurations (JSON)" to access your `launch.json` file.
-
Here’s an example of a basic `launch.json` configuration:
{ "version": "0.2.0", "configurations": [ { "name": "Debug C++", "type": "cppdbg", "request": "launch", "program": "${workspaceFolder}/bin/my_program", // Adjust the path to your executable "args": [], "stopAtEntry": false, "cwd": "${workspaceFolder}", "environment": [], "externalConsole": false, "MIMode": "gdb", // or "lldb" on Mac "setupCommands": [ { "description": "Enable pretty-printing for gdb", "text": "-enable-pretty-printing", "ignoreFailures": true } ], "preLaunchTask": "build", "setupCommands": [ { "text": "-enable-pretty-printing", "ignoreFailures": true } ] } ] }
-
-
Explanation of Key Settings: The `program` option specifies the executable to run, while `args` allows you to pass command line arguments. `cwd` sets the current working directory and `MIMode` selects the debugger used.
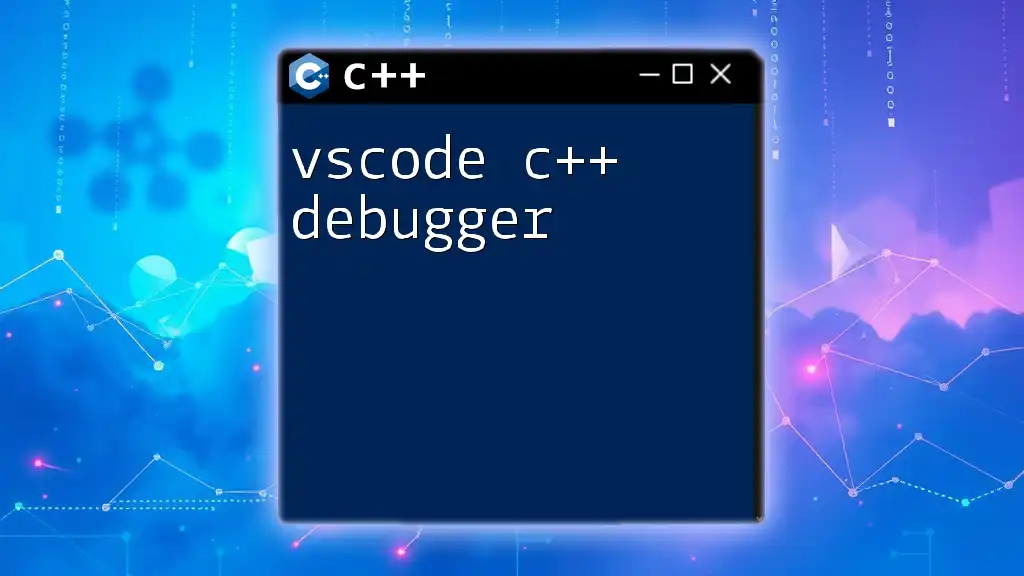
Basic Debugging Concepts
What is Debugging?
Debugging is the systematic process of identifying, isolating, and fixing bugs in your code. It’s an essential phase of the software development life cycle, as it ensures that your applications run as expected without errors or unintended behaviors. Key terms in debugging include:
- Breakpoints: These are markers set in the code where execution will pause, allowing you to inspect the program state.
- Watch Variables: These are specific variables that you want to monitor during execution to see how their values change.
- Call Stack: This is a list of method calls that shows the execution order and helps trace the flow of the program.
The Debugging Process
The debugging process typically involves several key steps:
- Identifying the Bug: Recognize symptoms in your application that indicate an error.
- Isolating the Bug: Use tools such as breakpoints to narrow down where in the code the bug occurs.
- Fixing and Verifying the Fix: After implementing a solution, test the code extensively to ensure that the bug is resolved without introducing new issues.
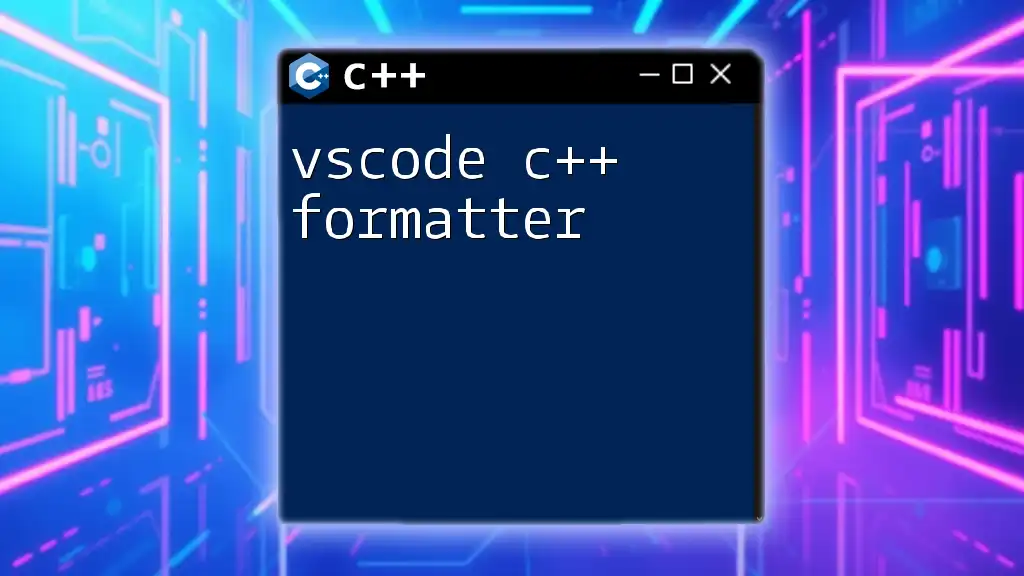
Using Debugging Features in VSCode
Setting Breakpoints
One of the most powerful features of `vscode c++ debug` functionality is the ability to set breakpoints. This allows you to pause execution at critical points in your code to examine the state:
- How to Set Breakpoints: Simply click on the left gutter in your code editor next to the line numbers where you want execution to pause.
- Adding Conditional Breakpoints: Right-click on the breakpoint and select "Edit Breakpoint" to add conditions under which the breakpoint will trigger.
Example:
if (x > 10) {
// This breakpoint is only hit if x is greater than 10
}
Navigating the Debugger
When the debugger is activated, the Debug panel will display buttons and information that help you control execution:
- Overview of the Debug Panel: Here, you can see the current call stack, watch expressions, and variable values.
- Debugger Controls: Utilize buttons such as "Continue" (F5) to run the program until the next breakpoint, "Step Over" (F10) to execute the next line without entering functions, "Step Into" (F11) to dive into function calls, and "Step Out" (Shift+F11) to exit the current function call.
Inspecting Variables and Watch Expressions
During debugging, you can inspect variable values, which helps you understand the state of your application:
- Viewing Variables: When the execution stops at a breakpoint, hover your mouse over variables to see their current values. Alternatively, monitor the "Variables" section in the debug panel.
- Adding Expressions to the Watch List: Use the "Watch" pane to add expressions or variables you wish to monitor throughout the debugging session.
Example:
int x = 5;
int y = x * 2; // Watch 'y' to see if it's calculated correctly
Using the Call Stack
Understanding the call stack is crucial for tracking function calls and understanding how you reached the current point in execution:
- Explanation of Call Stack: The call stack displays the sequence of function calls made up to the current point in the program. By analyzing the call stack, you can trace errors back to their origin.
- Navigating Call Stack Frames: In the debug panel, click on any frame to jump directly to that point in the code.
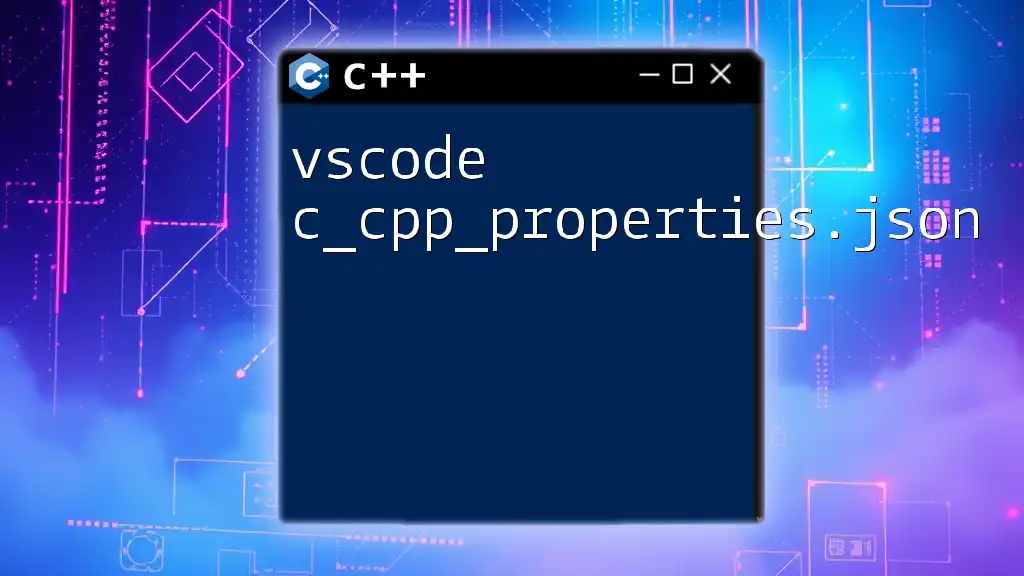
Advanced Debugging Techniques
Conditional Breakpoints and Logpoints
Conditional Breakpoints allow for more precise debugging:
- Setting Conditional Breakpoints: Right-click on a breakpoint to add conditions. For example, only halt execution if `i` is even:
for (int i = 0; i < 10; ++i) {
// Break here if i is even
}
- Implementing Logpoints: Logpoints offer a way to write messages to the debug console without stopping execution. Set a logpoint similarly to a breakpoint but choose "Log Message" instead. This is useful for printing variable states.
Remote Debugging
For situations where your application runs on a remote server, remote debugging allows you to connect to that environment directly from VSCode:
- Setting Up Remote Debugging: This will require configuration in the `launch.json` file and might involve SSH configuration depending on your remote setup.
Debugging Multi-threaded Applications
Debugging multi-threaded C++ applications can be challenging but VSCode offers tools to simplify this task:
- Challenges: Issues such as race conditions can be elusive, requiring careful inspection of thread states.
- Techniques: Utilize the debug panel to switch between thread contexts, set breakpoints in different threads, and monitor thread states.
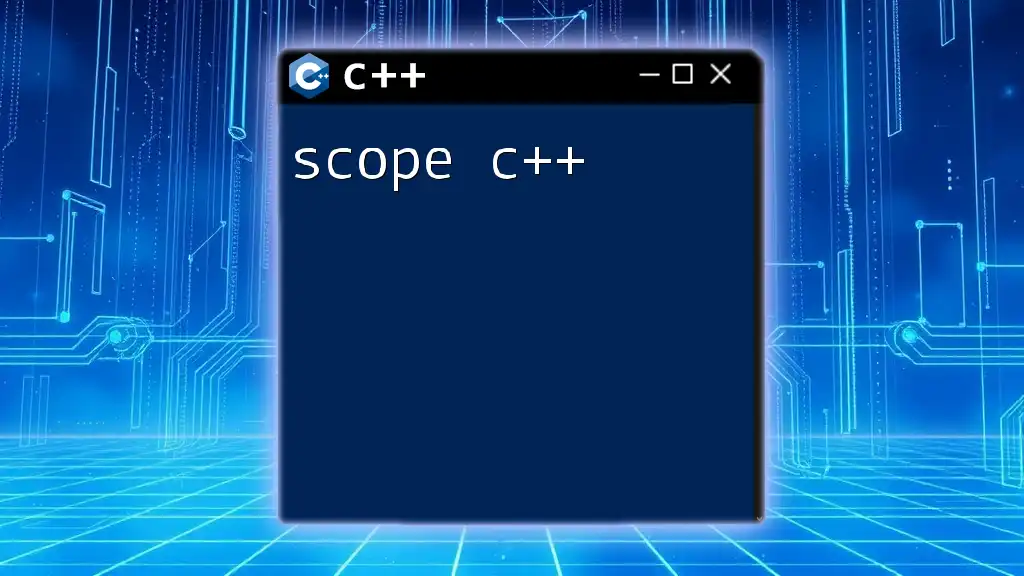
Troubleshooting Common Debugging Issues
Common Errors and Debugging Failures
While debugging in VSCode is robust, you may encounter several common issues:
- Build Configuration Problems: Ensure you have the proper build task set up to compile your C++ code successfully.
- Misconfigured `launch.json`: Verify that paths to your program and working directories are correct.
- Incorrect Breakpoints: If breakpoints aren't triggering, double-check that your code is being compiled with debug symbols.
Solutions and Workarounds
- Fixing Common Issues: Ensure that your build configurations are set to "Debug". Also, confirm that your `launch.json` file accurately reflects your workspace structure.
- Tips for a Smooth Debugging Experience: Regularly update your extensions and keep your development environment organized to minimize confusion.
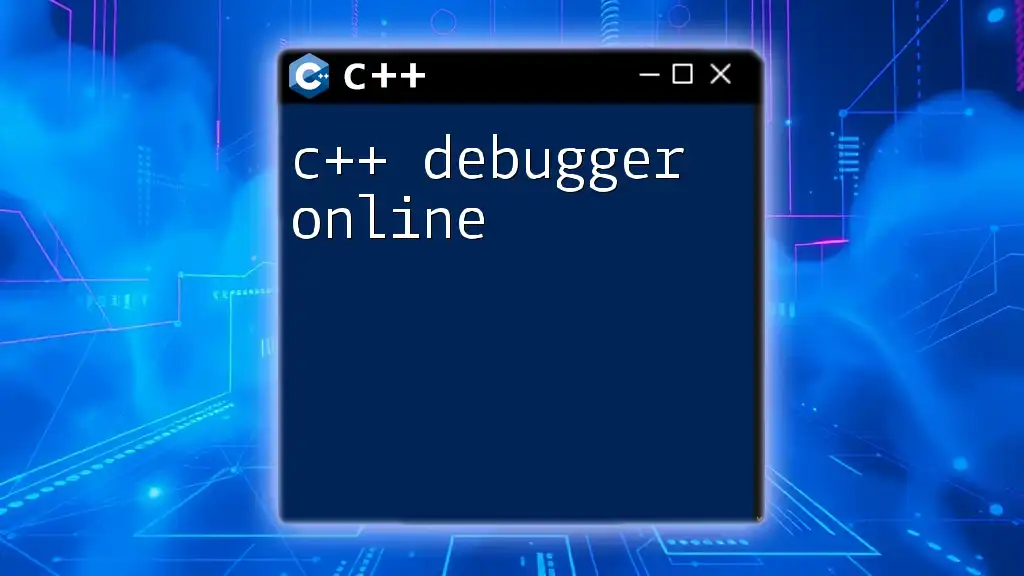
Conclusion
Throughout this guide, we explored the powerful debugging capabilities of VSCode, specifically focusing on C++. The combination of breakpoints, watch expressions, and tools like the call stack make VSCode an exceptional environment for debugging.
For a developer, mastering debugging tools is essential for effective coding and software reliability. Continue to explore and practice with the debugging features in VSCode to become more proficient and confident in your C++ development journey.
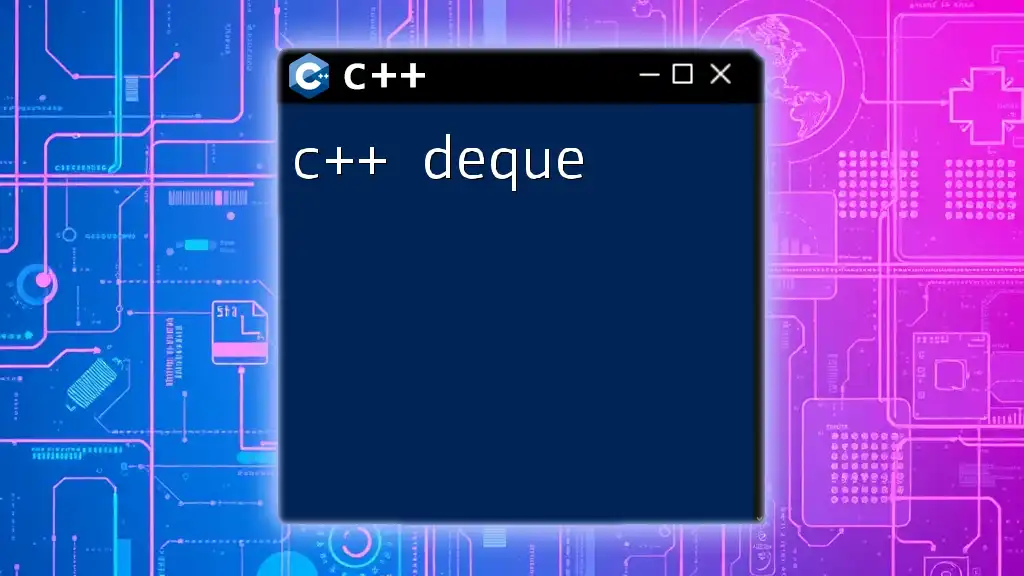
Additional Resources
To deepen your understanding of VSCode C++ debugging, consider checking out additional resources:
- Official Documentation: The Microsoft C++ extension page offers comprehensive guides and updates.
- Video Tutorials: Platforms like YouTube have numerous tutorials on VSCode debugging techniques.
- Forums and Communities: Engage with communities on sites like Stack Overflow or Reddit to find support and tips from fellow developers.