The `#ifdef DEBUG` directive in C++ is used to conditionally compile code segments, enabling or disabling debugging code based on whether the `DEBUG` macro is defined.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#define DEBUG // Uncomment this line to enable debugging
int main() {
std::cout << "Starting program..." << std::endl;
#ifdef DEBUG
std::cout << "Debug mode is ON" << std::endl;
#endif
// Your main code here
return 0;
}
Understanding Preprocessor Directives
What Are Preprocessor Directives?
Preprocessor directives are commands in C++ that instruct the compiler to process the code before the actual compilation begins. These directives allow for various manipulations of the codebase that can streamline development, especially in debugging scenarios. The most common preprocessor directives include:
- `#define`: Used to define constants or macros.
- `#include`: For including header files.
- `#if`/`#endif`: To include or exclude parts of code conditionally.
Importance of Debugging in C++
Debugging is an essential part of software development. It not only helps programmers identify and fix errors or bugs in their code but also plays a vital role in improving code quality. Proper debugging can significantly enhance performance and reliability of the software, ensuring a better end-user experience. Without effective debugging techniques, developers may overlook critical issues, potentially leading to significant problems down the line.
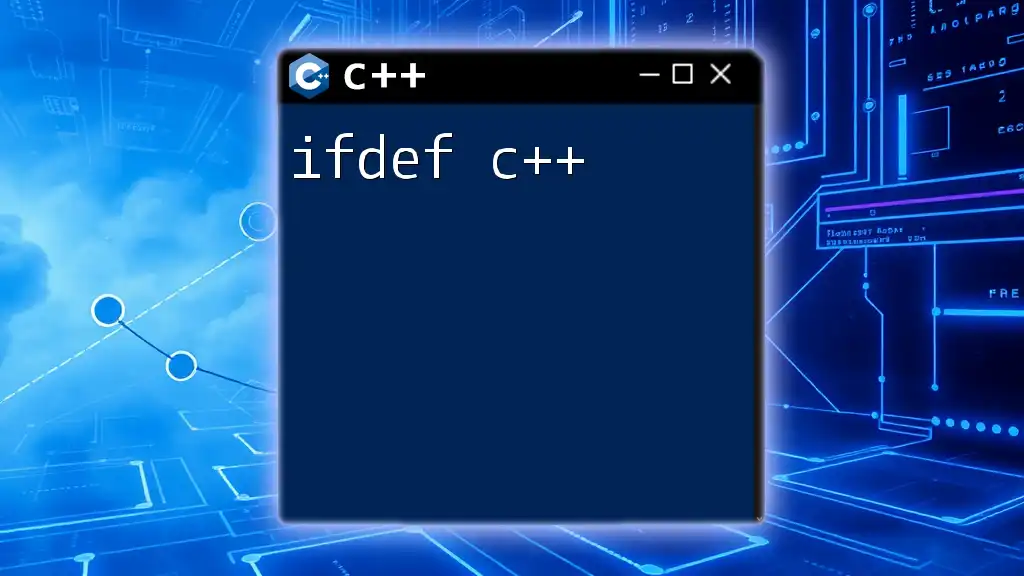
The `#ifdef` Directive
What is `#ifdef`?
The `#ifdef` directive is a conditional compilation method that allows programmers to compile specific sections of code only if a particular condition is met—specifically, if a given macro is defined. This provides flexibility in code management and helps isolate debugging or testing code from the production code.
Syntax of `#ifdef`
The syntax for the `#ifdef` directive is straightforward:
#ifdef DEBUG
// Code to include if DEBUG is defined
#endif
In this construct, if the `DEBUG` macro is defined before this section of the code, the compiler includes all lines between `#ifdef DEBUG` and `#endif`. If `DEBUG` is not defined, those lines are ignored during compilation.
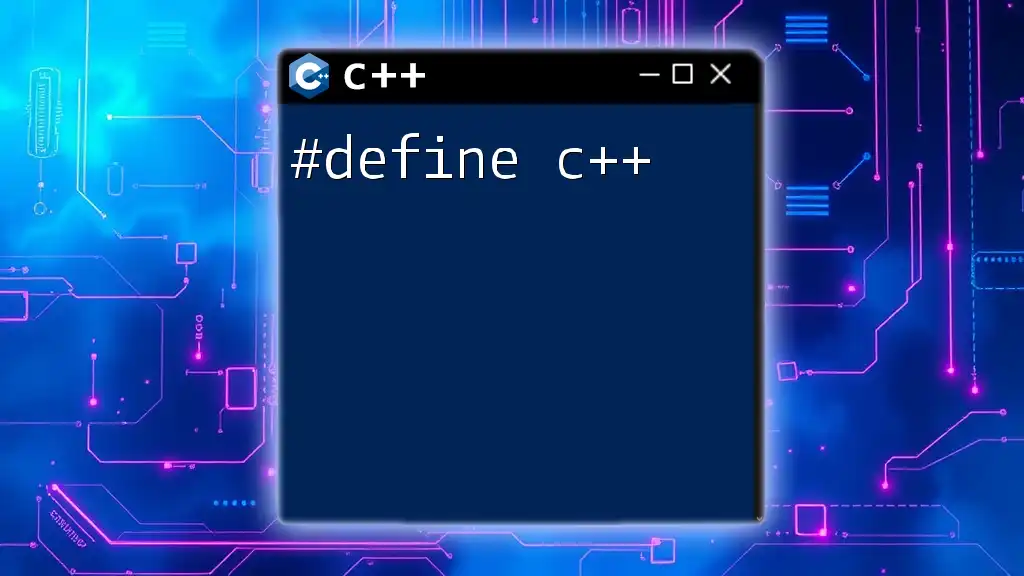
Implementing `#ifdef debug` in C++
Defining the Debug Macro
Before using the `#ifdef` directive, it is imperative to define the macro itself. This can typically be done with the `#define` preprocessor command:
#define DEBUG
The ideal place to include this line might be in a header file or at the top of the main source file. This way, developers can easily toggle the debugging environment by simply adding or removing this definition.
Using `#ifdef debug` in Your Code
Using `#ifdef debug` is an excellent way to manage debug information within your C++ programs. For instance, consider the following sample code:
#include <iostream>
#define DEBUG
int main() {
std::cout << "Program started." << std::endl;
#ifdef DEBUG
std::cout << "Debugging information: Program is running smoothly." << std::endl;
#endif
// Main program logic here
return 0;
}
In this example, when the program is compiled with `DEBUG` defined, the output will include the message: "Debugging information: Program is running smoothly." If you were to remove or comment out the `#define DEBUG` line, that debugging message would no longer be printed, reducing unnecessary output in production.
Turning Debugging On and Off
A practical application of `#ifdef debug` is switching debugging information on and off without modifying the core logic of the program. This can be accomplished by using the `#ifndef` directive to exclude debug information from production builds:
#ifndef DEBUG
std::cout << "In production mode. No debug info." << std::endl;
#endif
This allows you to selectively include or exclude debug lines based on the defined condition, assisting in maintaining clean and manageable code.
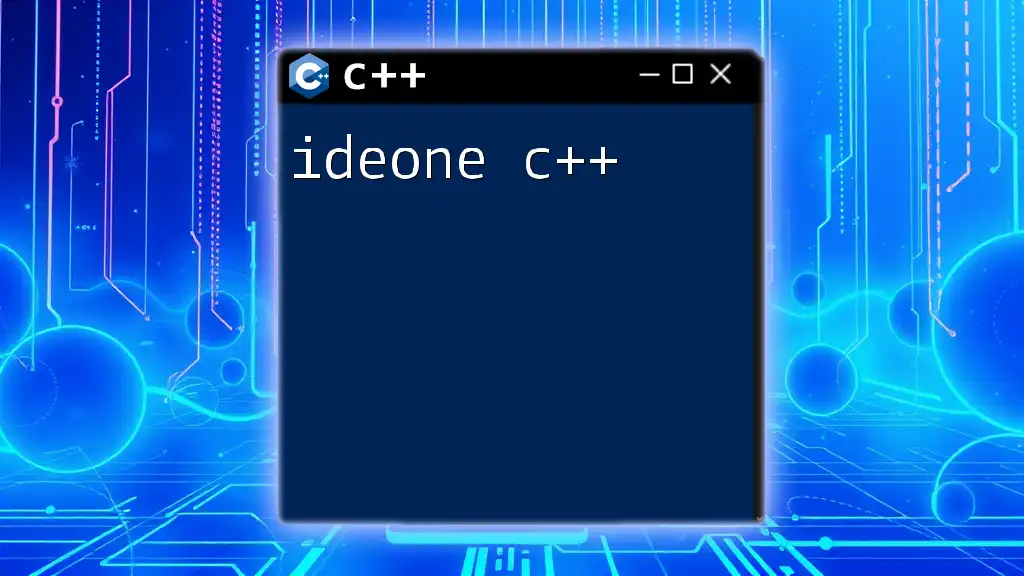
Best Practices for Using `#ifdef` for Debugging
Keep Debug Code Separate
It is a best practice to keep debugging code separate from production code. Cluttered code can lead to confusion and increase the likelihood of errors. One effective strategy is to group all debug-related code in a dedicated section of the project or within specific header files.
Avoiding Debug Code in Production
Ensuring that debug code does not inadvertently end up in production builds is crucial for performance and security. Utilize build scripts or IDE settings to help manage which macros are defined based on the build type. For example, you can use compiler flags to control whether or not `DEBUG` is defined during the build process.
Documenting Debug Code
Well-documented code ensures future maintainability and clarity. Make it a habit to provide comments in your debug code, explaining its purpose and any important functions. For example:
#ifdef DEBUG
// This log outputs the status of critical variables.
std::cout << "Variable X is: " << x << std::endl;
#endif
Providing context can significantly help other developers understand your intentions and the reasoning behind your debugging strategy.
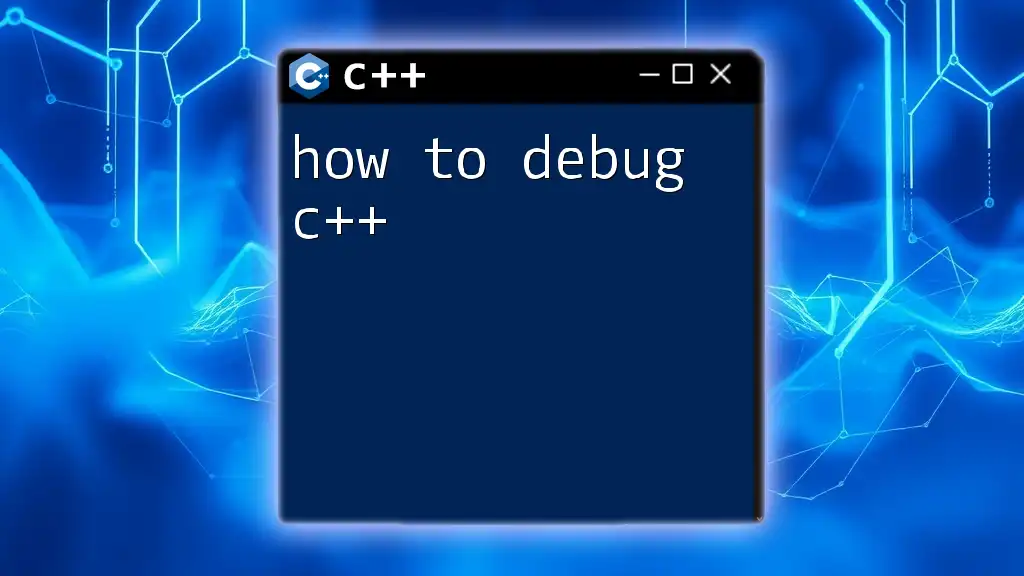
Advanced Techniques
Nested `#ifdef` Directives
Complex situations may arise where you need to manage multiple debug levels or features. In such cases, nested `#ifdef` directives can be used. For example:
#ifdef DEBUG
#ifdef VERBOSE
std::cout << "Verbose debug information." << std::endl;
#endif
#endif
This structure allows different levels of debugging output based on multiple defined macros and helps in finer control over the debugging information presented.
Using `#pragma message`
Another advanced technique is utilizing `#pragma message`, which can provide compile-time messages about the state of your program:
#ifdef DEBUG
#pragma message("Debug mode is enabled.")
#endif
This can be especially useful for logging the current build configuration directly in the compiler output, reinforcing awareness of debug states.
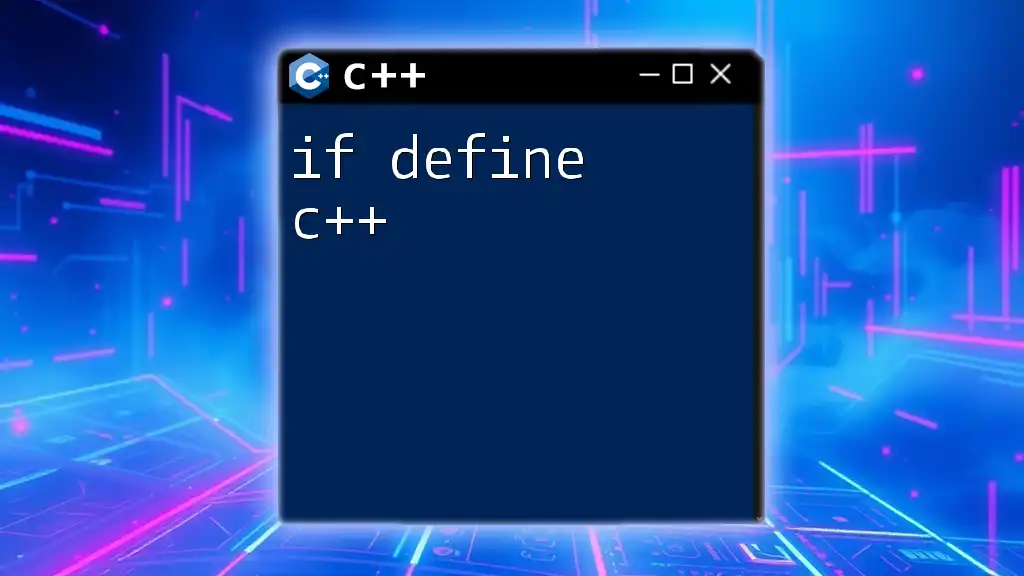
Conclusion
The `#ifdef debug` directive is an invaluable tool for C++ developers aiming to enhance their debugging practices. By implementing conditional compilation, separating debug code from production code, and keeping thorough documentation, developers can ensure better code quality and integrity in their applications.
Incorporating such techniques allows for a cleaner, more manageable codebase, minimizing the struggle of debugging while maximizing productivity. Embrace these strategies in your coding practices, and remember that effective debugging is not just about fixing errors—it's about understanding and improving your code.