In C++, the `delete[]` operator is used to deallocate memory that was previously allocated for an array using `new[]`, ensuring proper memory management and preventing memory leaks.
Here's a code snippet that demonstrates its use:
#include <iostream>
int main() {
int* array = new int[5]; // allocate memory for an array of 5 integers
// ... use the array ...
delete[] array; // deallocate the array memory
return 0;
}
Understanding `delete` in C++
The `delete` operator in C++ is crucial for managing dynamically allocated memory. When you allocate memory using the `new` operator, C++ doesn't automatically free that memory when it goes out of scope. Instead, it remains allocated until you explicitly deallocate it using `delete`. Without proper management, this leads to memory leaks, which can degrade system performance over time.
Purpose of `delete` and its Implications
The primary purpose of the `delete` operator is to release memory back to the system. This prevents the program from consuming excessive memory resources. However, understanding how to use it correctly is essential. If you misuse `delete`, you can inadvertently lead to problems such as double deletions or memory leaks.
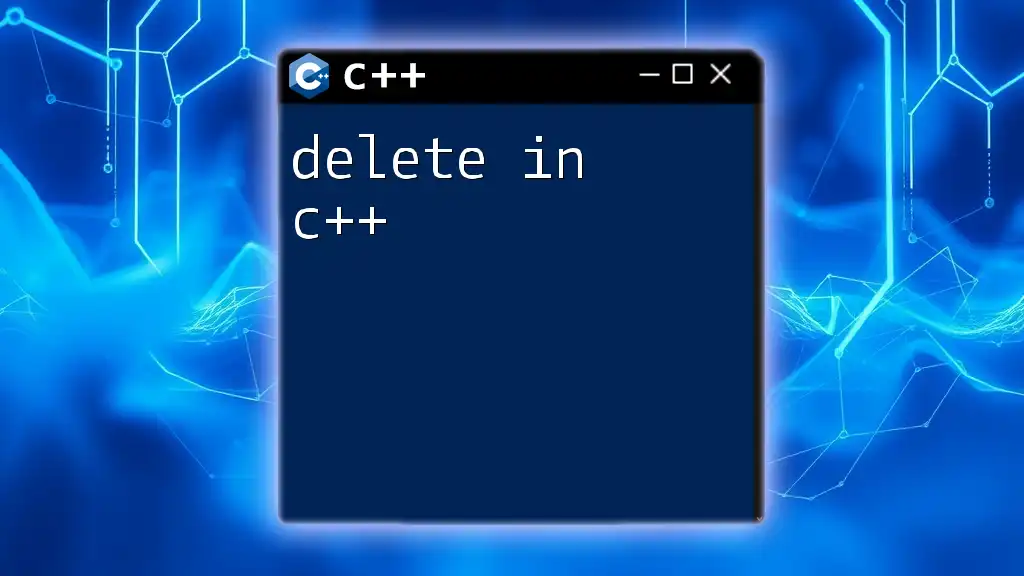
What is `delete[]`?
The `delete[]` operator is specifically designed for deallocating memory that was allocated for arrays. When you allocate memory for an array of objects, it’s crucial to use `delete[]` instead of `delete`. This distinction ensures that the destructors for all elements in the array are called, maintaining proper resource management for each object.
Use Case Scenarios for `delete[]`
- Dynamic array management: When you use `new` to create an array, you must use `delete[]` to prevent memory leaks.
- Complex objects: For objects with dynamic memory allocation within their constructors, calling the corresponding destructors is vital.
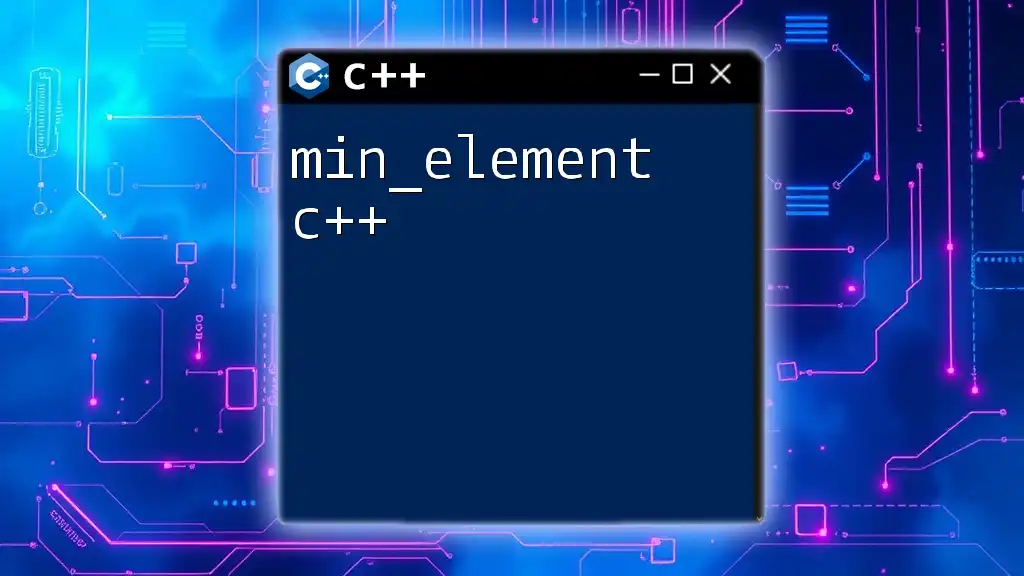
How to Use `delete[]` in C++
Syntax of `delete[]`
The syntax for using `delete[]` follows a straightforward pattern. When you want to deallocate an array, you simply use:
delete[] pointer_to_array;
This tells the compiler to release the memory allocated for that entire array.
Example Code Snippet
int* myArray = new int[5]; // Allocating memory for an array of integers
for (int i = 0; i < 5; i++) {
myArray[i] = i * 10; // Initializing array elements
}
// ... perform operations on the array
delete[] myArray; // Correctly deallocating the array
In this example, an array of integers is dynamically allocated and later deallocated using `delete[]`. This ensures that the memory is properly freed, preventing leaks.
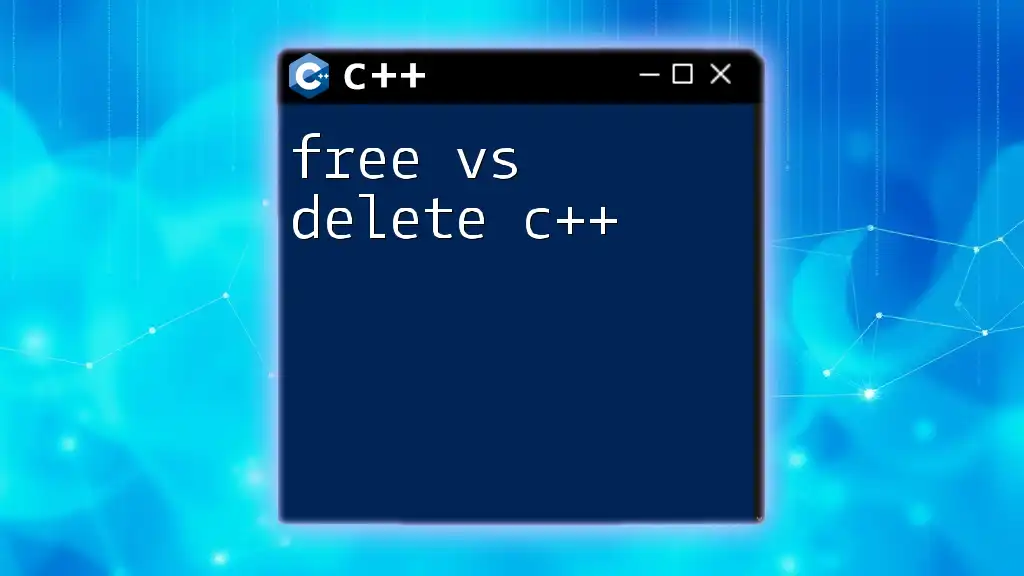
The Role of Constructors and Destructors
When using classes that manage resources, it’s essential to understand the implications of constructors and destructors.
Implicit vs. Explicit Calls
When you allocate memory for an array of objects, the constructor for each object is called automatically. Conversely, when deallocating, `delete[]` will call the destructor for each object in the array. This is critical for classes that manage resources like file handles or memory.
Example of Class with Destructor
class MyClass {
public:
MyClass() {
// Constructor code to initialize resources
}
~MyClass() {
// Destructor code to free resources
}
};
MyClass* objArray = new MyClass[10]; // Allocating array of objects
delete[] objArray; // Calls the destructor for each object
In this example, allocating an array of `MyClass` objects automatically invokes the constructor for each object. When `delete[]` is called, the destructor is executed for every instance, ensuring that any resources are properly released.
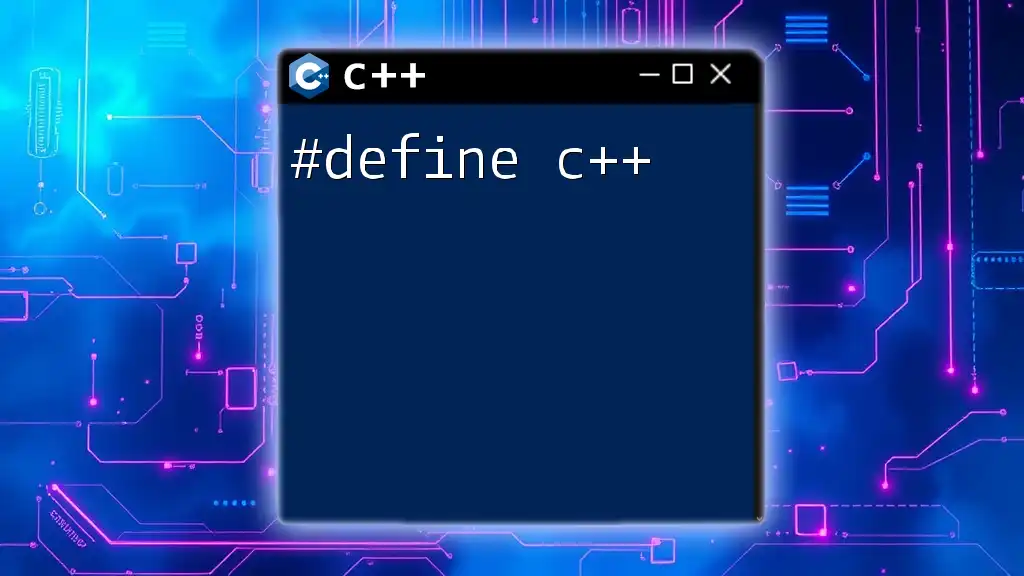
Common Pitfalls When Using `delete[]`
Managing memory comes with common pitfalls that developers must navigate carefully.
Memory Leaks
A memory leak occurs when allocated memory is not released. This can happen if you forget to use `delete[]`, leading to wasted resources. Tools like Valgrind can help identify memory leaks in your code.
Double Deletion
Attempting to delete the same memory twice can lead to undefined behavior, often resulting in program crashes. This error is more prevalent in complex applications where dynamic memory allocation is extensively used.
Using `delete` Instead of `delete[]`
Using `delete` on an array leads to undefined behavior because it only calls the destructor for the first element. As such, using `delete` on `new[]` can lead to resource leaks and potentially corrupt memory. An example of incorrect usage:
int* myArray = new int[5];
delete myArray; // Incorrect: Only deallocates the first element
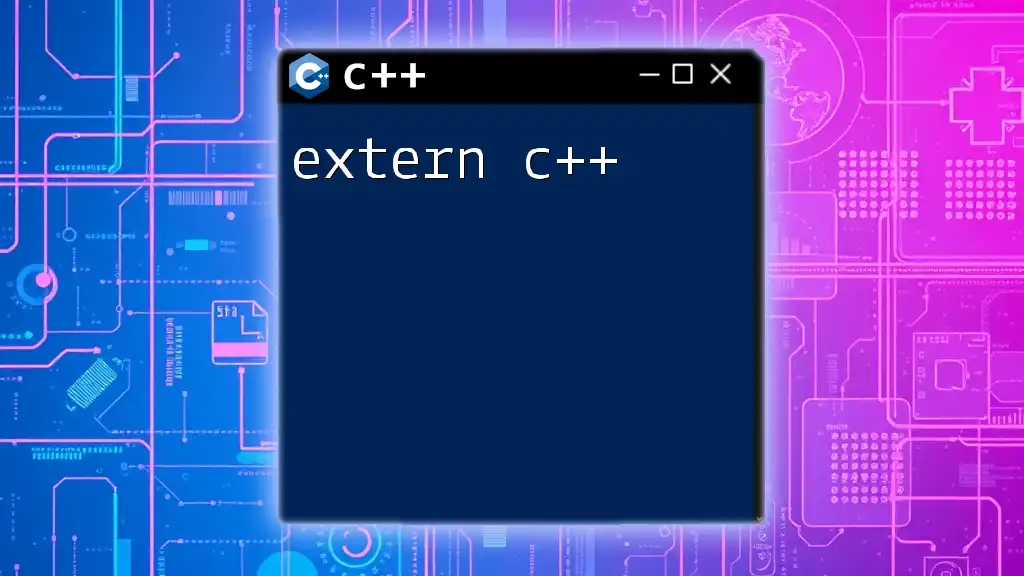
Best Practices for Memory Management
Always Pair `new` with `delete[]`
Always ensure that the use of `new` for array allocation is paired with `delete[]`. This practice maintains the integrity of memory management in your program, preventing leaks and undefined behaviors.
Using Smart Pointers
Smart pointers such as `std::unique_ptr` and `std::shared_ptr` can simplify memory management significantly. They automatically handle deallocation when they go out of scope, providing a safer alternative to raw pointers.
Example of Using `std::unique_ptr`
#include <memory>
std::unique_ptr<int[]> mySmartArray(new int[5]);
// No need to manually delete; memory will be cleaned up automatically
By using smart pointers, you ensure that memory is always released without explicitly calling `delete[]`, enhancing safety and maintainability in your code.
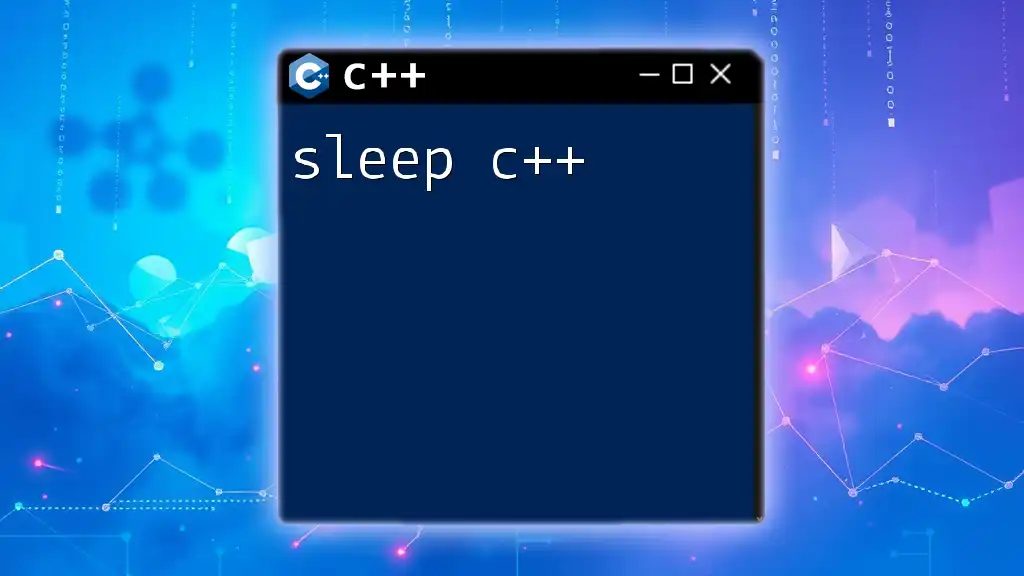
Conclusion
Understanding how to correctly use `delete[]` in C++ is vital for proper memory management. Misusing this operator can lead to severe issues such as memory leaks and program crashes. As you gain familiarity with dynamic memory and its implications, ensure you practice correct pairing of `new` and `delete[]`. Remember to explore the use of smart pointers in modern C++ for a more robust approach to memory management.
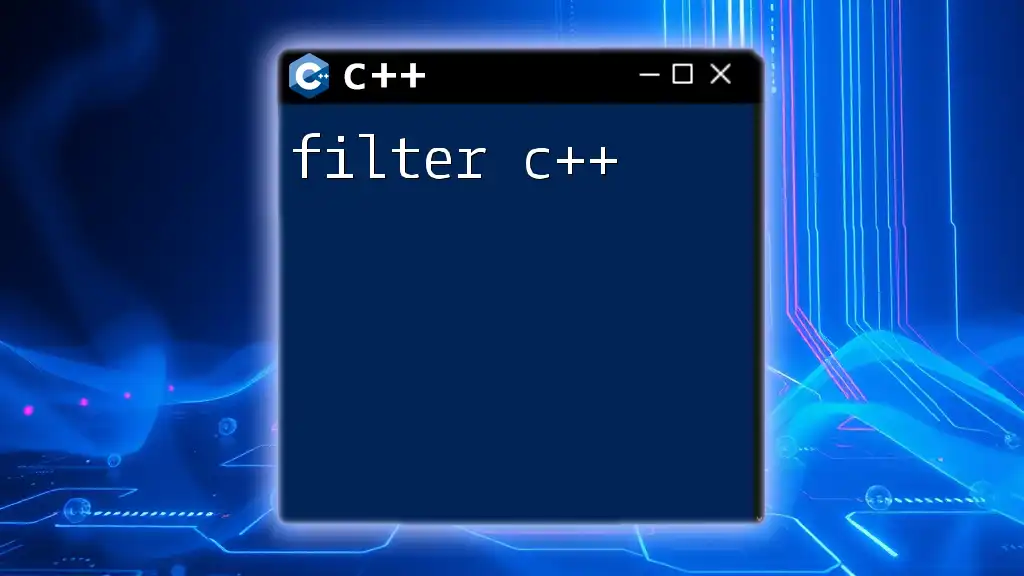
FAQ Section
You may encounter common queries regarding `delete[]`. Understanding the answers to these questions can clarify any confusion surrounding memory management practices in C++.
Further Reading
To deepen your knowledge of memory management, consider exploring additional resources, including documentation and advanced courses that focus on C++ best practices. By investing time in these areas, you can build a stronger foundation and avoid common pitfalls in memory management.